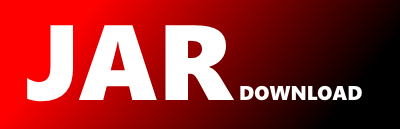
io.ciera.tool.sql.ooaofooa.deployment.impl.DeploymentImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.deployment.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.deployment.Deployment;
import io.ciera.tool.sql.ooaofooa.deployment.Terminator;
import io.ciera.tool.sql.ooaofooa.deployment.TerminatorSet;
import io.ciera.tool.sql.ooaofooa.deployment.impl.TerminatorSetImpl;
import io.ciera.tool.sql.ooaofooa.packageableelement.PackageableElement;
import io.ciera.tool.sql.ooaofooa.packageableelement.impl.PackageableElementImpl;
public class DeploymentImpl extends ModelInstance implements Deployment {
public static final String KEY_LETTERS = "D_DEPL";
public static final Deployment EMPTY_DEPLOYMENT = new EmptyDeployment();
private Sql context;
// constructors
private DeploymentImpl( Sql context ) {
this.context = context;
ref_Deployment_ID = UniqueId.random();
m_Name = "";
m_Descrip = "";
m_Key_Lett = "";
R1650_Terminator_set = new TerminatorSetImpl();
R8001_is_a_PackageableElement_inst = PackageableElementImpl.EMPTY_PACKAGEABLEELEMENT;
}
private DeploymentImpl( Sql context, UniqueId instanceId, UniqueId ref_Deployment_ID, String m_Name, String m_Descrip, String m_Key_Lett ) {
super(instanceId);
this.context = context;
this.ref_Deployment_ID = ref_Deployment_ID;
this.m_Name = m_Name;
this.m_Descrip = m_Descrip;
this.m_Key_Lett = m_Key_Lett;
R1650_Terminator_set = new TerminatorSetImpl();
R8001_is_a_PackageableElement_inst = PackageableElementImpl.EMPTY_PACKAGEABLEELEMENT;
}
public static Deployment create( Sql context ) throws XtumlException {
Deployment newDeployment = new DeploymentImpl( context );
if ( context.addInstance( newDeployment ) ) {
newDeployment.getRunContext().addChange(new InstanceCreatedDelta(newDeployment, KEY_LETTERS));
return newDeployment;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static Deployment create( Sql context, UniqueId ref_Deployment_ID, String m_Name, String m_Descrip, String m_Key_Lett ) throws XtumlException {
return create(context, UniqueId.random(), ref_Deployment_ID, m_Name, m_Descrip, m_Key_Lett);
}
public static Deployment create( Sql context, UniqueId instanceId, UniqueId ref_Deployment_ID, String m_Name, String m_Descrip, String m_Key_Lett ) throws XtumlException {
Deployment newDeployment = new DeploymentImpl( context, instanceId, ref_Deployment_ID, m_Name, m_Descrip, m_Key_Lett );
if ( context.addInstance( newDeployment ) ) {
return newDeployment;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId ref_Deployment_ID;
@Override
public void setDeployment_ID(UniqueId ref_Deployment_ID) throws XtumlException {
checkLiving();
if (ref_Deployment_ID.inequality( this.ref_Deployment_ID)) {
final UniqueId oldValue = this.ref_Deployment_ID;
this.ref_Deployment_ID = ref_Deployment_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Deployment_ID", oldValue, this.ref_Deployment_ID));
if ( !R1650_Terminator().isEmpty() ) R1650_Terminator().setDeployment_ID( ref_Deployment_ID );
}
}
@Override
public UniqueId getDeployment_ID() throws XtumlException {
checkLiving();
return ref_Deployment_ID;
}
private String m_Name;
@Override
public String getName() throws XtumlException {
checkLiving();
return m_Name;
}
@Override
public void setName(String m_Name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Name, this.m_Name)) {
final String oldValue = this.m_Name;
this.m_Name = m_Name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Name", oldValue, this.m_Name));
}
}
private String m_Descrip;
@Override
public void setDescrip(String m_Descrip) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Descrip, this.m_Descrip)) {
final String oldValue = this.m_Descrip;
this.m_Descrip = m_Descrip;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Descrip", oldValue, this.m_Descrip));
}
}
@Override
public String getDescrip() throws XtumlException {
checkLiving();
return m_Descrip;
}
private String m_Key_Lett;
@Override
public String getKey_Lett() throws XtumlException {
checkLiving();
return m_Key_Lett;
}
@Override
public void setKey_Lett(String m_Key_Lett) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Key_Lett, this.m_Key_Lett)) {
final String oldValue = this.m_Key_Lett;
this.m_Key_Lett = m_Key_Lett;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Key_Lett", oldValue, this.m_Key_Lett));
}
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getDeployment_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private TerminatorSet R1650_Terminator_set;
@Override
public void addR1650_Terminator( Terminator inst ) {
R1650_Terminator_set.add(inst);
}
@Override
public void removeR1650_Terminator( Terminator inst ) {
R1650_Terminator_set.remove(inst);
}
@Override
public TerminatorSet R1650_Terminator() throws XtumlException {
return R1650_Terminator_set;
}
private PackageableElement R8001_is_a_PackageableElement_inst;
@Override
public void setR8001_is_a_PackageableElement( PackageableElement inst ) {
R8001_is_a_PackageableElement_inst = inst;
}
@Override
public PackageableElement R8001_is_a_PackageableElement() throws XtumlException {
return R8001_is_a_PackageableElement_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public Deployment self() {
return this;
}
@Override
public Deployment oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_DEPLOYMENT;
}
}
class EmptyDeployment extends ModelInstance implements Deployment {
// attributes
public void setDeployment_ID( UniqueId ref_Deployment_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getDeployment_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getName() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setName( String m_Name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setDescrip( String m_Descrip ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getDescrip() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getKey_Lett() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setKey_Lett( String m_Key_Lett ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
// operations
// selections
@Override
public TerminatorSet R1650_Terminator() {
return (new TerminatorSetImpl());
}
@Override
public PackageableElement R8001_is_a_PackageableElement() {
return PackageableElementImpl.EMPTY_PACKAGEABLEELEMENT;
}
@Override
public String getKeyLetters() {
return DeploymentImpl.KEY_LETTERS;
}
@Override
public Deployment self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public Deployment oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return DeploymentImpl.EMPTY_DEPLOYMENT;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy