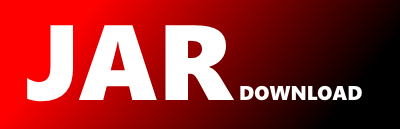
io.ciera.tool.sql.ooaofooa.domain.impl.ExternalEntityInModelImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.domain.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.domain.ExternalEntity;
import io.ciera.tool.sql.ooaofooa.domain.ExternalEntityInModel;
import io.ciera.tool.sql.ooaofooa.domain.impl.ExternalEntityImpl;
public class ExternalEntityInModelImpl extends ModelInstance implements ExternalEntityInModel {
public static final String KEY_LETTERS = "S_EEM";
public static final ExternalEntityInModel EMPTY_EXTERNALENTITYINMODEL = new EmptyExternalEntityInModel();
private Sql context;
// constructors
private ExternalEntityInModelImpl( Sql context ) {
this.context = context;
m_EEmod_ID = UniqueId.random();
ref_EE_ID = UniqueId.random();
m_Modl_Typ = 0;
m_SS_IDdeprecated = UniqueId.random();
R9_is_a_presence_in_subsystem_model_of_ExternalEntity_inst = ExternalEntityImpl.EMPTY_EXTERNALENTITY;
}
private ExternalEntityInModelImpl( Sql context, UniqueId instanceId, UniqueId m_EEmod_ID, UniqueId ref_EE_ID, int m_Modl_Typ, UniqueId m_SS_IDdeprecated ) {
super(instanceId);
this.context = context;
this.m_EEmod_ID = m_EEmod_ID;
this.ref_EE_ID = ref_EE_ID;
this.m_Modl_Typ = m_Modl_Typ;
this.m_SS_IDdeprecated = m_SS_IDdeprecated;
R9_is_a_presence_in_subsystem_model_of_ExternalEntity_inst = ExternalEntityImpl.EMPTY_EXTERNALENTITY;
}
public static ExternalEntityInModel create( Sql context ) throws XtumlException {
ExternalEntityInModel newExternalEntityInModel = new ExternalEntityInModelImpl( context );
if ( context.addInstance( newExternalEntityInModel ) ) {
newExternalEntityInModel.getRunContext().addChange(new InstanceCreatedDelta(newExternalEntityInModel, KEY_LETTERS));
return newExternalEntityInModel;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static ExternalEntityInModel create( Sql context, UniqueId m_EEmod_ID, UniqueId ref_EE_ID, int m_Modl_Typ, UniqueId m_SS_IDdeprecated ) throws XtumlException {
return create(context, UniqueId.random(), m_EEmod_ID, ref_EE_ID, m_Modl_Typ, m_SS_IDdeprecated);
}
public static ExternalEntityInModel create( Sql context, UniqueId instanceId, UniqueId m_EEmod_ID, UniqueId ref_EE_ID, int m_Modl_Typ, UniqueId m_SS_IDdeprecated ) throws XtumlException {
ExternalEntityInModel newExternalEntityInModel = new ExternalEntityInModelImpl( context, instanceId, m_EEmod_ID, ref_EE_ID, m_Modl_Typ, m_SS_IDdeprecated );
if ( context.addInstance( newExternalEntityInModel ) ) {
return newExternalEntityInModel;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId m_EEmod_ID;
@Override
public void setEEmod_ID(UniqueId m_EEmod_ID) throws XtumlException {
checkLiving();
if (m_EEmod_ID.inequality( this.m_EEmod_ID)) {
final UniqueId oldValue = this.m_EEmod_ID;
this.m_EEmod_ID = m_EEmod_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_EEmod_ID", oldValue, this.m_EEmod_ID));
}
}
@Override
public UniqueId getEEmod_ID() throws XtumlException {
checkLiving();
return m_EEmod_ID;
}
private UniqueId ref_EE_ID;
@Override
public UniqueId getEE_ID() throws XtumlException {
checkLiving();
return ref_EE_ID;
}
@Override
public void setEE_ID(UniqueId ref_EE_ID) throws XtumlException {
checkLiving();
if (ref_EE_ID.inequality( this.ref_EE_ID)) {
final UniqueId oldValue = this.ref_EE_ID;
this.ref_EE_ID = ref_EE_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_EE_ID", oldValue, this.ref_EE_ID));
}
}
private int m_Modl_Typ;
@Override
public void setModl_Typ(int m_Modl_Typ) throws XtumlException {
checkLiving();
if (m_Modl_Typ != this.m_Modl_Typ) {
final int oldValue = this.m_Modl_Typ;
this.m_Modl_Typ = m_Modl_Typ;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Modl_Typ", oldValue, this.m_Modl_Typ));
}
}
@Override
public int getModl_Typ() throws XtumlException {
checkLiving();
return m_Modl_Typ;
}
private UniqueId m_SS_IDdeprecated;
@Override
public UniqueId getSS_IDdeprecated() throws XtumlException {
checkLiving();
return m_SS_IDdeprecated;
}
@Override
public void setSS_IDdeprecated(UniqueId m_SS_IDdeprecated) throws XtumlException {
checkLiving();
if (m_SS_IDdeprecated.inequality( this.m_SS_IDdeprecated)) {
final UniqueId oldValue = this.m_SS_IDdeprecated;
this.m_SS_IDdeprecated = m_SS_IDdeprecated;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_SS_IDdeprecated", oldValue, this.m_SS_IDdeprecated));
}
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getEEmod_ID(), getEE_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private ExternalEntity R9_is_a_presence_in_subsystem_model_of_ExternalEntity_inst;
@Override
public void setR9_is_a_presence_in_subsystem_model_of_ExternalEntity( ExternalEntity inst ) {
R9_is_a_presence_in_subsystem_model_of_ExternalEntity_inst = inst;
}
@Override
public ExternalEntity R9_is_a_presence_in_subsystem_model_of_ExternalEntity() throws XtumlException {
return R9_is_a_presence_in_subsystem_model_of_ExternalEntity_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public ExternalEntityInModel self() {
return this;
}
@Override
public ExternalEntityInModel oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_EXTERNALENTITYINMODEL;
}
}
class EmptyExternalEntityInModel extends ModelInstance implements ExternalEntityInModel {
// attributes
public void setEEmod_ID( UniqueId m_EEmod_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getEEmod_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public UniqueId getEE_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setEE_ID( UniqueId ref_EE_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setModl_Typ( int m_Modl_Typ ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getModl_Typ() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public UniqueId getSS_IDdeprecated() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setSS_IDdeprecated( UniqueId m_SS_IDdeprecated ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
// operations
// selections
@Override
public ExternalEntity R9_is_a_presence_in_subsystem_model_of_ExternalEntity() {
return ExternalEntityImpl.EMPTY_EXTERNALENTITY;
}
@Override
public String getKeyLetters() {
return ExternalEntityInModelImpl.KEY_LETTERS;
}
@Override
public ExternalEntityInModel self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public ExternalEntityInModel oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return ExternalEntityInModelImpl.EMPTY_EXTERNALENTITYINMODEL;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy