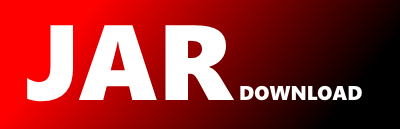
io.ciera.tool.sql.ooaofooa.domain.impl.FunctionParameterImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.domain.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.domain.DataType;
import io.ciera.tool.sql.ooaofooa.domain.Dimensions;
import io.ciera.tool.sql.ooaofooa.domain.DimensionsSet;
import io.ciera.tool.sql.ooaofooa.domain.FunctionParameter;
import io.ciera.tool.sql.ooaofooa.domain.S_SYNC;
import io.ciera.tool.sql.ooaofooa.domain.impl.DataTypeImpl;
import io.ciera.tool.sql.ooaofooa.domain.impl.DimensionsSetImpl;
import io.ciera.tool.sql.ooaofooa.domain.impl.FunctionParameterImpl;
import io.ciera.tool.sql.ooaofooa.domain.impl.S_SYNCImpl;
import io.ciera.tool.sql.ooaofooa.message.FunctionArgument;
import io.ciera.tool.sql.ooaofooa.message.FunctionArgumentSet;
import io.ciera.tool.sql.ooaofooa.message.impl.FunctionArgumentSetImpl;
import io.ciera.tool.sql.ooaofooa.value.ParameterValue;
import io.ciera.tool.sql.ooaofooa.value.ParameterValueSet;
import io.ciera.tool.sql.ooaofooa.value.impl.ParameterValueSetImpl;
public class FunctionParameterImpl extends ModelInstance implements FunctionParameter {
public static final String KEY_LETTERS = "S_SPARM";
public static final FunctionParameter EMPTY_FUNCTIONPARAMETER = new EmptyFunctionParameter();
private Sql context;
// constructors
private FunctionParameterImpl( Sql context ) {
this.context = context;
m_SParm_ID = UniqueId.random();
ref_Sync_ID = UniqueId.random();
m_Name = "";
ref_DT_ID = UniqueId.random();
m_By_Ref = 0;
m_Dimensions = "";
ref_Previous_SParm_ID = UniqueId.random();
m_Descrip = "";
R1016_represents_FunctionArgument_set = new FunctionArgumentSetImpl();
R24_is_defined_for_S_SYNC_inst = S_SYNCImpl.EMPTY_S_SYNC;
R26_is_typed_by__DataType_inst = DataTypeImpl.EMPTY_DATATYPE;
R52_may_have_Dimensions_set = new DimensionsSetImpl();
R54_precedes_FunctionParameter_inst = FunctionParameterImpl.EMPTY_FUNCTIONPARAMETER;
R54_succeeds_FunctionParameter_inst = FunctionParameterImpl.EMPTY_FUNCTIONPARAMETER;
R832_ParameterValue_set = new ParameterValueSetImpl();
}
private FunctionParameterImpl( Sql context, UniqueId instanceId, UniqueId m_SParm_ID, UniqueId ref_Sync_ID, String m_Name, UniqueId ref_DT_ID, int m_By_Ref, String m_Dimensions, UniqueId ref_Previous_SParm_ID, String m_Descrip ) {
super(instanceId);
this.context = context;
this.m_SParm_ID = m_SParm_ID;
this.ref_Sync_ID = ref_Sync_ID;
this.m_Name = m_Name;
this.ref_DT_ID = ref_DT_ID;
this.m_By_Ref = m_By_Ref;
this.m_Dimensions = m_Dimensions;
this.ref_Previous_SParm_ID = ref_Previous_SParm_ID;
this.m_Descrip = m_Descrip;
R1016_represents_FunctionArgument_set = new FunctionArgumentSetImpl();
R24_is_defined_for_S_SYNC_inst = S_SYNCImpl.EMPTY_S_SYNC;
R26_is_typed_by__DataType_inst = DataTypeImpl.EMPTY_DATATYPE;
R52_may_have_Dimensions_set = new DimensionsSetImpl();
R54_precedes_FunctionParameter_inst = FunctionParameterImpl.EMPTY_FUNCTIONPARAMETER;
R54_succeeds_FunctionParameter_inst = FunctionParameterImpl.EMPTY_FUNCTIONPARAMETER;
R832_ParameterValue_set = new ParameterValueSetImpl();
}
public static FunctionParameter create( Sql context ) throws XtumlException {
FunctionParameter newFunctionParameter = new FunctionParameterImpl( context );
if ( context.addInstance( newFunctionParameter ) ) {
newFunctionParameter.getRunContext().addChange(new InstanceCreatedDelta(newFunctionParameter, KEY_LETTERS));
return newFunctionParameter;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static FunctionParameter create( Sql context, UniqueId m_SParm_ID, UniqueId ref_Sync_ID, String m_Name, UniqueId ref_DT_ID, int m_By_Ref, String m_Dimensions, UniqueId ref_Previous_SParm_ID, String m_Descrip ) throws XtumlException {
return create(context, UniqueId.random(), m_SParm_ID, ref_Sync_ID, m_Name, ref_DT_ID, m_By_Ref, m_Dimensions, ref_Previous_SParm_ID, m_Descrip);
}
public static FunctionParameter create( Sql context, UniqueId instanceId, UniqueId m_SParm_ID, UniqueId ref_Sync_ID, String m_Name, UniqueId ref_DT_ID, int m_By_Ref, String m_Dimensions, UniqueId ref_Previous_SParm_ID, String m_Descrip ) throws XtumlException {
FunctionParameter newFunctionParameter = new FunctionParameterImpl( context, instanceId, m_SParm_ID, ref_Sync_ID, m_Name, ref_DT_ID, m_By_Ref, m_Dimensions, ref_Previous_SParm_ID, m_Descrip );
if ( context.addInstance( newFunctionParameter ) ) {
return newFunctionParameter;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId m_SParm_ID;
@Override
public void setSParm_ID(UniqueId m_SParm_ID) throws XtumlException {
checkLiving();
if (m_SParm_ID.inequality( this.m_SParm_ID)) {
final UniqueId oldValue = this.m_SParm_ID;
this.m_SParm_ID = m_SParm_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_SParm_ID", oldValue, this.m_SParm_ID));
if ( !R54_precedes_FunctionParameter().isEmpty() ) R54_precedes_FunctionParameter().setPrevious_SParm_ID( m_SParm_ID );
if ( !R1016_represents_FunctionArgument().isEmpty() ) R1016_represents_FunctionArgument().setSParm_ID( m_SParm_ID );
if ( !R52_may_have_Dimensions().isEmpty() ) R52_may_have_Dimensions().setSParm_ID( m_SParm_ID );
if ( !R832_ParameterValue().isEmpty() ) R832_ParameterValue().setSParm_ID( m_SParm_ID );
}
}
@Override
public UniqueId getSParm_ID() throws XtumlException {
checkLiving();
return m_SParm_ID;
}
private UniqueId ref_Sync_ID;
@Override
public UniqueId getSync_ID() throws XtumlException {
checkLiving();
return ref_Sync_ID;
}
@Override
public void setSync_ID(UniqueId ref_Sync_ID) throws XtumlException {
checkLiving();
if (ref_Sync_ID.inequality( this.ref_Sync_ID)) {
final UniqueId oldValue = this.ref_Sync_ID;
this.ref_Sync_ID = ref_Sync_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Sync_ID", oldValue, this.ref_Sync_ID));
}
}
private String m_Name;
@Override
public void setName(String m_Name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Name, this.m_Name)) {
final String oldValue = this.m_Name;
this.m_Name = m_Name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Name", oldValue, this.m_Name));
}
}
@Override
public String getName() throws XtumlException {
checkLiving();
return m_Name;
}
private UniqueId ref_DT_ID;
@Override
public void setDT_ID(UniqueId ref_DT_ID) throws XtumlException {
checkLiving();
if (ref_DT_ID.inequality( this.ref_DT_ID)) {
final UniqueId oldValue = this.ref_DT_ID;
this.ref_DT_ID = ref_DT_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_DT_ID", oldValue, this.ref_DT_ID));
}
}
@Override
public UniqueId getDT_ID() throws XtumlException {
checkLiving();
return ref_DT_ID;
}
private int m_By_Ref;
@Override
public void setBy_Ref(int m_By_Ref) throws XtumlException {
checkLiving();
if (m_By_Ref != this.m_By_Ref) {
final int oldValue = this.m_By_Ref;
this.m_By_Ref = m_By_Ref;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_By_Ref", oldValue, this.m_By_Ref));
}
}
@Override
public int getBy_Ref() throws XtumlException {
checkLiving();
return m_By_Ref;
}
private String m_Dimensions;
@Override
public String getDimensions() throws XtumlException {
checkLiving();
return m_Dimensions;
}
@Override
public void setDimensions(String m_Dimensions) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Dimensions, this.m_Dimensions)) {
final String oldValue = this.m_Dimensions;
this.m_Dimensions = m_Dimensions;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Dimensions", oldValue, this.m_Dimensions));
}
}
private UniqueId ref_Previous_SParm_ID;
@Override
public UniqueId getPrevious_SParm_ID() throws XtumlException {
checkLiving();
return ref_Previous_SParm_ID;
}
@Override
public void setPrevious_SParm_ID(UniqueId ref_Previous_SParm_ID) throws XtumlException {
checkLiving();
if (ref_Previous_SParm_ID.inequality( this.ref_Previous_SParm_ID)) {
final UniqueId oldValue = this.ref_Previous_SParm_ID;
this.ref_Previous_SParm_ID = ref_Previous_SParm_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Previous_SParm_ID", oldValue, this.ref_Previous_SParm_ID));
}
}
private String m_Descrip;
@Override
public void setDescrip(String m_Descrip) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Descrip, this.m_Descrip)) {
final String oldValue = this.m_Descrip;
this.m_Descrip = m_Descrip;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Descrip", oldValue, this.m_Descrip));
}
}
@Override
public String getDescrip() throws XtumlException {
checkLiving();
return m_Descrip;
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getSParm_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private FunctionArgumentSet R1016_represents_FunctionArgument_set;
@Override
public void addR1016_represents_FunctionArgument( FunctionArgument inst ) {
R1016_represents_FunctionArgument_set.add(inst);
}
@Override
public void removeR1016_represents_FunctionArgument( FunctionArgument inst ) {
R1016_represents_FunctionArgument_set.remove(inst);
}
@Override
public FunctionArgumentSet R1016_represents_FunctionArgument() throws XtumlException {
return R1016_represents_FunctionArgument_set;
}
private S_SYNC R24_is_defined_for_S_SYNC_inst;
@Override
public void setR24_is_defined_for_S_SYNC( S_SYNC inst ) {
R24_is_defined_for_S_SYNC_inst = inst;
}
@Override
public S_SYNC R24_is_defined_for_S_SYNC() throws XtumlException {
return R24_is_defined_for_S_SYNC_inst;
}
private DataType R26_is_typed_by__DataType_inst;
@Override
public void setR26_is_typed_by__DataType( DataType inst ) {
R26_is_typed_by__DataType_inst = inst;
}
@Override
public DataType R26_is_typed_by__DataType() throws XtumlException {
return R26_is_typed_by__DataType_inst;
}
private DimensionsSet R52_may_have_Dimensions_set;
@Override
public void addR52_may_have_Dimensions( Dimensions inst ) {
R52_may_have_Dimensions_set.add(inst);
}
@Override
public void removeR52_may_have_Dimensions( Dimensions inst ) {
R52_may_have_Dimensions_set.remove(inst);
}
@Override
public DimensionsSet R52_may_have_Dimensions() throws XtumlException {
return R52_may_have_Dimensions_set;
}
private FunctionParameter R54_precedes_FunctionParameter_inst;
@Override
public void setR54_precedes_FunctionParameter( FunctionParameter inst ) {
R54_precedes_FunctionParameter_inst = inst;
}
@Override
public FunctionParameter R54_precedes_FunctionParameter() throws XtumlException {
return R54_precedes_FunctionParameter_inst;
}
private FunctionParameter R54_succeeds_FunctionParameter_inst;
@Override
public void setR54_succeeds_FunctionParameter( FunctionParameter inst ) {
R54_succeeds_FunctionParameter_inst = inst;
}
@Override
public FunctionParameter R54_succeeds_FunctionParameter() throws XtumlException {
return R54_succeeds_FunctionParameter_inst;
}
private ParameterValueSet R832_ParameterValue_set;
@Override
public void addR832_ParameterValue( ParameterValue inst ) {
R832_ParameterValue_set.add(inst);
}
@Override
public void removeR832_ParameterValue( ParameterValue inst ) {
R832_ParameterValue_set.remove(inst);
}
@Override
public ParameterValueSet R832_ParameterValue() throws XtumlException {
return R832_ParameterValue_set;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public FunctionParameter self() {
return this;
}
@Override
public FunctionParameter oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_FUNCTIONPARAMETER;
}
}
class EmptyFunctionParameter extends ModelInstance implements FunctionParameter {
// attributes
public void setSParm_ID( UniqueId m_SParm_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getSParm_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public UniqueId getSync_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setSync_ID( UniqueId ref_Sync_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setName( String m_Name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getName() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setDT_ID( UniqueId ref_DT_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getDT_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setBy_Ref( int m_By_Ref ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getBy_Ref() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getDimensions() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setDimensions( String m_Dimensions ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getPrevious_SParm_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setPrevious_SParm_ID( UniqueId ref_Previous_SParm_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setDescrip( String m_Descrip ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getDescrip() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
// operations
// selections
@Override
public FunctionArgumentSet R1016_represents_FunctionArgument() {
return (new FunctionArgumentSetImpl());
}
@Override
public S_SYNC R24_is_defined_for_S_SYNC() {
return S_SYNCImpl.EMPTY_S_SYNC;
}
@Override
public DataType R26_is_typed_by__DataType() {
return DataTypeImpl.EMPTY_DATATYPE;
}
@Override
public DimensionsSet R52_may_have_Dimensions() {
return (new DimensionsSetImpl());
}
@Override
public FunctionParameter R54_precedes_FunctionParameter() {
return FunctionParameterImpl.EMPTY_FUNCTIONPARAMETER;
}
@Override
public FunctionParameter R54_succeeds_FunctionParameter() {
return FunctionParameterImpl.EMPTY_FUNCTIONPARAMETER;
}
@Override
public ParameterValueSet R832_ParameterValue() {
return (new ParameterValueSetImpl());
}
@Override
public String getKeyLetters() {
return FunctionParameterImpl.KEY_LETTERS;
}
@Override
public FunctionParameter self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public FunctionParameter oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return FunctionParameterImpl.EMPTY_FUNCTIONPARAMETER;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy