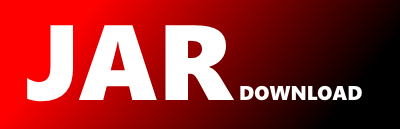
io.ciera.tool.sql.ooaofooa.domain.impl.S_SYNCImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.domain.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.body.FunctionBody;
import io.ciera.tool.sql.ooaofooa.body.impl.FunctionBodyImpl;
import io.ciera.tool.sql.ooaofooa.domain.DataType;
import io.ciera.tool.sql.ooaofooa.domain.Dimensions;
import io.ciera.tool.sql.ooaofooa.domain.DimensionsSet;
import io.ciera.tool.sql.ooaofooa.domain.FunctionParameter;
import io.ciera.tool.sql.ooaofooa.domain.FunctionParameterSet;
import io.ciera.tool.sql.ooaofooa.domain.S_SYNC;
import io.ciera.tool.sql.ooaofooa.domain.impl.DataTypeImpl;
import io.ciera.tool.sql.ooaofooa.domain.impl.DimensionsSetImpl;
import io.ciera.tool.sql.ooaofooa.domain.impl.FunctionParameterSetImpl;
import io.ciera.tool.sql.ooaofooa.invocation.FunctionInvocation;
import io.ciera.tool.sql.ooaofooa.invocation.FunctionInvocationSet;
import io.ciera.tool.sql.ooaofooa.invocation.impl.FunctionInvocationSetImpl;
import io.ciera.tool.sql.ooaofooa.message.FunctionMessage;
import io.ciera.tool.sql.ooaofooa.message.FunctionMessageSet;
import io.ciera.tool.sql.ooaofooa.message.impl.FunctionMessageSetImpl;
import io.ciera.tool.sql.ooaofooa.packageableelement.PackageableElement;
import io.ciera.tool.sql.ooaofooa.packageableelement.impl.PackageableElementImpl;
import io.ciera.tool.sql.ooaofooa.value.FunctionValue;
import io.ciera.tool.sql.ooaofooa.value.FunctionValueSet;
import io.ciera.tool.sql.ooaofooa.value.impl.FunctionValueSetImpl;
import ooaofooa.datatypes.ActionDialect;
import ooaofooa.datatypes.ParseStatus;
public class S_SYNCImpl extends ModelInstance implements S_SYNC {
public static final String KEY_LETTERS = "S_SYNC";
public static final S_SYNC EMPTY_S_SYNC = new EmptyS_SYNC();
private Sql context;
// constructors
private S_SYNCImpl( Sql context ) {
this.context = context;
ref_Sync_ID = UniqueId.random();
m_Dom_IDdeprecated = UniqueId.random();
m_Name = "";
m_Descrip = "";
m_Action_Semantics = "";
m_Action_Semantics_internal = "";
ref_DT_ID = UniqueId.random();
m_Suc_Pars = ParseStatus.UNINITIALIZED_ENUM;
m_Return_Dimensions = "";
m_Dialect = ActionDialect.UNINITIALIZED_ENUM;
m_Numb = 0;
R1010_is_invoked_by_FunctionMessage_set = new FunctionMessageSetImpl();
R24_defines_FunctionParameter_set = new FunctionParameterSetImpl();
R25_has_return_type_of_DataType_inst = DataTypeImpl.EMPTY_DATATYPE;
R51_return_value_may_have_Dimensions_set = new DimensionsSetImpl();
R675_FunctionInvocation_set = new FunctionInvocationSetImpl();
R695_FunctionBody_inst = FunctionBodyImpl.EMPTY_FUNCTIONBODY;
R8001_is_a_PackageableElement_inst = PackageableElementImpl.EMPTY_PACKAGEABLEELEMENT;
R827_FunctionValue_set = new FunctionValueSetImpl();
}
private S_SYNCImpl( Sql context, UniqueId instanceId, UniqueId ref_Sync_ID, UniqueId m_Dom_IDdeprecated, String m_Name, String m_Descrip, String m_Action_Semantics, String m_Action_Semantics_internal, UniqueId ref_DT_ID, ParseStatus m_Suc_Pars, String m_Return_Dimensions, ActionDialect m_Dialect, int m_Numb ) {
super(instanceId);
this.context = context;
this.ref_Sync_ID = ref_Sync_ID;
this.m_Dom_IDdeprecated = m_Dom_IDdeprecated;
this.m_Name = m_Name;
this.m_Descrip = m_Descrip;
this.m_Action_Semantics = m_Action_Semantics;
this.m_Action_Semantics_internal = m_Action_Semantics_internal;
this.ref_DT_ID = ref_DT_ID;
this.m_Suc_Pars = m_Suc_Pars;
this.m_Return_Dimensions = m_Return_Dimensions;
this.m_Dialect = m_Dialect;
this.m_Numb = m_Numb;
R1010_is_invoked_by_FunctionMessage_set = new FunctionMessageSetImpl();
R24_defines_FunctionParameter_set = new FunctionParameterSetImpl();
R25_has_return_type_of_DataType_inst = DataTypeImpl.EMPTY_DATATYPE;
R51_return_value_may_have_Dimensions_set = new DimensionsSetImpl();
R675_FunctionInvocation_set = new FunctionInvocationSetImpl();
R695_FunctionBody_inst = FunctionBodyImpl.EMPTY_FUNCTIONBODY;
R8001_is_a_PackageableElement_inst = PackageableElementImpl.EMPTY_PACKAGEABLEELEMENT;
R827_FunctionValue_set = new FunctionValueSetImpl();
}
public static S_SYNC create( Sql context ) throws XtumlException {
S_SYNC newS_SYNC = new S_SYNCImpl( context );
if ( context.addInstance( newS_SYNC ) ) {
newS_SYNC.getRunContext().addChange(new InstanceCreatedDelta(newS_SYNC, KEY_LETTERS));
return newS_SYNC;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static S_SYNC create( Sql context, UniqueId ref_Sync_ID, UniqueId m_Dom_IDdeprecated, String m_Name, String m_Descrip, String m_Action_Semantics, String m_Action_Semantics_internal, UniqueId ref_DT_ID, ParseStatus m_Suc_Pars, String m_Return_Dimensions, ActionDialect m_Dialect, int m_Numb ) throws XtumlException {
return create(context, UniqueId.random(), ref_Sync_ID, m_Dom_IDdeprecated, m_Name, m_Descrip, m_Action_Semantics, m_Action_Semantics_internal, ref_DT_ID, m_Suc_Pars, m_Return_Dimensions, m_Dialect, m_Numb);
}
public static S_SYNC create( Sql context, UniqueId instanceId, UniqueId ref_Sync_ID, UniqueId m_Dom_IDdeprecated, String m_Name, String m_Descrip, String m_Action_Semantics, String m_Action_Semantics_internal, UniqueId ref_DT_ID, ParseStatus m_Suc_Pars, String m_Return_Dimensions, ActionDialect m_Dialect, int m_Numb ) throws XtumlException {
S_SYNC newS_SYNC = new S_SYNCImpl( context, instanceId, ref_Sync_ID, m_Dom_IDdeprecated, m_Name, m_Descrip, m_Action_Semantics, m_Action_Semantics_internal, ref_DT_ID, m_Suc_Pars, m_Return_Dimensions, m_Dialect, m_Numb );
if ( context.addInstance( newS_SYNC ) ) {
return newS_SYNC;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId ref_Sync_ID;
@Override
public UniqueId getSync_ID() throws XtumlException {
checkLiving();
return ref_Sync_ID;
}
@Override
public void setSync_ID(UniqueId ref_Sync_ID) throws XtumlException {
checkLiving();
if (ref_Sync_ID.inequality( this.ref_Sync_ID)) {
final UniqueId oldValue = this.ref_Sync_ID;
this.ref_Sync_ID = ref_Sync_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Sync_ID", oldValue, this.ref_Sync_ID));
if ( !R675_FunctionInvocation().isEmpty() ) R675_FunctionInvocation().setSync_ID( ref_Sync_ID );
if ( !R695_FunctionBody().isEmpty() ) R695_FunctionBody().setSync_ID( ref_Sync_ID );
if ( !R827_FunctionValue().isEmpty() ) R827_FunctionValue().setSync_ID( ref_Sync_ID );
if ( !R51_return_value_may_have_Dimensions().isEmpty() ) R51_return_value_may_have_Dimensions().setSync_ID( ref_Sync_ID );
if ( !R24_defines_FunctionParameter().isEmpty() ) R24_defines_FunctionParameter().setSync_ID( ref_Sync_ID );
if ( !R1010_is_invoked_by_FunctionMessage().isEmpty() ) R1010_is_invoked_by_FunctionMessage().setSync_ID( ref_Sync_ID );
}
}
private UniqueId m_Dom_IDdeprecated;
@Override
public void setDom_IDdeprecated(UniqueId m_Dom_IDdeprecated) throws XtumlException {
checkLiving();
if (m_Dom_IDdeprecated.inequality( this.m_Dom_IDdeprecated)) {
final UniqueId oldValue = this.m_Dom_IDdeprecated;
this.m_Dom_IDdeprecated = m_Dom_IDdeprecated;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Dom_IDdeprecated", oldValue, this.m_Dom_IDdeprecated));
}
}
@Override
public UniqueId getDom_IDdeprecated() throws XtumlException {
checkLiving();
return m_Dom_IDdeprecated;
}
private String m_Name;
@Override
public void setName(String m_Name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Name, this.m_Name)) {
final String oldValue = this.m_Name;
this.m_Name = m_Name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Name", oldValue, this.m_Name));
}
}
@Override
public String getName() throws XtumlException {
checkLiving();
return m_Name;
}
private String m_Descrip;
@Override
public String getDescrip() throws XtumlException {
checkLiving();
return m_Descrip;
}
@Override
public void setDescrip(String m_Descrip) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Descrip, this.m_Descrip)) {
final String oldValue = this.m_Descrip;
this.m_Descrip = m_Descrip;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Descrip", oldValue, this.m_Descrip));
}
}
private String m_Action_Semantics;
@Override
public void setAction_Semantics(String m_Action_Semantics) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Action_Semantics, this.m_Action_Semantics)) {
final String oldValue = this.m_Action_Semantics;
this.m_Action_Semantics = m_Action_Semantics;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Action_Semantics", oldValue, this.m_Action_Semantics));
}
}
@Override
public String getAction_Semantics() throws XtumlException {
checkLiving();
return m_Action_Semantics;
}
private String m_Action_Semantics_internal;
@Override
public void setAction_Semantics_internal(String m_Action_Semantics_internal) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Action_Semantics_internal, this.m_Action_Semantics_internal)) {
final String oldValue = this.m_Action_Semantics_internal;
this.m_Action_Semantics_internal = m_Action_Semantics_internal;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Action_Semantics_internal", oldValue, this.m_Action_Semantics_internal));
}
}
@Override
public String getAction_Semantics_internal() throws XtumlException {
checkLiving();
return m_Action_Semantics_internal;
}
private UniqueId ref_DT_ID;
@Override
public void setDT_ID(UniqueId ref_DT_ID) throws XtumlException {
checkLiving();
if (ref_DT_ID.inequality( this.ref_DT_ID)) {
final UniqueId oldValue = this.ref_DT_ID;
this.ref_DT_ID = ref_DT_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_DT_ID", oldValue, this.ref_DT_ID));
}
}
@Override
public UniqueId getDT_ID() throws XtumlException {
checkLiving();
return ref_DT_ID;
}
private ParseStatus m_Suc_Pars;
@Override
public void setSuc_Pars(ParseStatus m_Suc_Pars) throws XtumlException {
checkLiving();
if (m_Suc_Pars.inequality( this.m_Suc_Pars)) {
final ParseStatus oldValue = this.m_Suc_Pars;
this.m_Suc_Pars = m_Suc_Pars;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Suc_Pars", oldValue, this.m_Suc_Pars));
}
}
@Override
public ParseStatus getSuc_Pars() throws XtumlException {
checkLiving();
return m_Suc_Pars;
}
private String m_Return_Dimensions;
@Override
public void setReturn_Dimensions(String m_Return_Dimensions) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Return_Dimensions, this.m_Return_Dimensions)) {
final String oldValue = this.m_Return_Dimensions;
this.m_Return_Dimensions = m_Return_Dimensions;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Return_Dimensions", oldValue, this.m_Return_Dimensions));
}
}
@Override
public String getReturn_Dimensions() throws XtumlException {
checkLiving();
return m_Return_Dimensions;
}
private ActionDialect m_Dialect;
@Override
public ActionDialect getDialect() throws XtumlException {
checkLiving();
return m_Dialect;
}
@Override
public void setDialect(ActionDialect m_Dialect) throws XtumlException {
checkLiving();
if (m_Dialect.inequality( this.m_Dialect)) {
final ActionDialect oldValue = this.m_Dialect;
this.m_Dialect = m_Dialect;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Dialect", oldValue, this.m_Dialect));
}
}
private int m_Numb;
@Override
public void setNumb(int m_Numb) throws XtumlException {
checkLiving();
if (m_Numb != this.m_Numb) {
final int oldValue = this.m_Numb;
this.m_Numb = m_Numb;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Numb", oldValue, this.m_Numb));
}
}
@Override
public int getNumb() throws XtumlException {
checkLiving();
return m_Numb;
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getSync_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private FunctionMessageSet R1010_is_invoked_by_FunctionMessage_set;
@Override
public void addR1010_is_invoked_by_FunctionMessage( FunctionMessage inst ) {
R1010_is_invoked_by_FunctionMessage_set.add(inst);
}
@Override
public void removeR1010_is_invoked_by_FunctionMessage( FunctionMessage inst ) {
R1010_is_invoked_by_FunctionMessage_set.remove(inst);
}
@Override
public FunctionMessageSet R1010_is_invoked_by_FunctionMessage() throws XtumlException {
return R1010_is_invoked_by_FunctionMessage_set;
}
private FunctionParameterSet R24_defines_FunctionParameter_set;
@Override
public void addR24_defines_FunctionParameter( FunctionParameter inst ) {
R24_defines_FunctionParameter_set.add(inst);
}
@Override
public void removeR24_defines_FunctionParameter( FunctionParameter inst ) {
R24_defines_FunctionParameter_set.remove(inst);
}
@Override
public FunctionParameterSet R24_defines_FunctionParameter() throws XtumlException {
return R24_defines_FunctionParameter_set;
}
private DataType R25_has_return_type_of_DataType_inst;
@Override
public void setR25_has_return_type_of_DataType( DataType inst ) {
R25_has_return_type_of_DataType_inst = inst;
}
@Override
public DataType R25_has_return_type_of_DataType() throws XtumlException {
return R25_has_return_type_of_DataType_inst;
}
private DimensionsSet R51_return_value_may_have_Dimensions_set;
@Override
public void addR51_return_value_may_have_Dimensions( Dimensions inst ) {
R51_return_value_may_have_Dimensions_set.add(inst);
}
@Override
public void removeR51_return_value_may_have_Dimensions( Dimensions inst ) {
R51_return_value_may_have_Dimensions_set.remove(inst);
}
@Override
public DimensionsSet R51_return_value_may_have_Dimensions() throws XtumlException {
return R51_return_value_may_have_Dimensions_set;
}
private FunctionInvocationSet R675_FunctionInvocation_set;
@Override
public void addR675_FunctionInvocation( FunctionInvocation inst ) {
R675_FunctionInvocation_set.add(inst);
}
@Override
public void removeR675_FunctionInvocation( FunctionInvocation inst ) {
R675_FunctionInvocation_set.remove(inst);
}
@Override
public FunctionInvocationSet R675_FunctionInvocation() throws XtumlException {
return R675_FunctionInvocation_set;
}
private FunctionBody R695_FunctionBody_inst;
@Override
public void setR695_FunctionBody( FunctionBody inst ) {
R695_FunctionBody_inst = inst;
}
@Override
public FunctionBody R695_FunctionBody() throws XtumlException {
return R695_FunctionBody_inst;
}
private PackageableElement R8001_is_a_PackageableElement_inst;
@Override
public void setR8001_is_a_PackageableElement( PackageableElement inst ) {
R8001_is_a_PackageableElement_inst = inst;
}
@Override
public PackageableElement R8001_is_a_PackageableElement() throws XtumlException {
return R8001_is_a_PackageableElement_inst;
}
private FunctionValueSet R827_FunctionValue_set;
@Override
public void addR827_FunctionValue( FunctionValue inst ) {
R827_FunctionValue_set.add(inst);
}
@Override
public void removeR827_FunctionValue( FunctionValue inst ) {
R827_FunctionValue_set.remove(inst);
}
@Override
public FunctionValueSet R827_FunctionValue() throws XtumlException {
return R827_FunctionValue_set;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public S_SYNC self() {
return this;
}
@Override
public S_SYNC oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_S_SYNC;
}
}
class EmptyS_SYNC extends ModelInstance implements S_SYNC {
// attributes
public UniqueId getSync_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setSync_ID( UniqueId ref_Sync_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setDom_IDdeprecated( UniqueId m_Dom_IDdeprecated ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getDom_IDdeprecated() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setName( String m_Name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getName() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getDescrip() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setDescrip( String m_Descrip ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setAction_Semantics( String m_Action_Semantics ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getAction_Semantics() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setAction_Semantics_internal( String m_Action_Semantics_internal ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getAction_Semantics_internal() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setDT_ID( UniqueId ref_DT_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getDT_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setSuc_Pars( ParseStatus m_Suc_Pars ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public ParseStatus getSuc_Pars() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setReturn_Dimensions( String m_Return_Dimensions ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getReturn_Dimensions() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public ActionDialect getDialect() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setDialect( ActionDialect m_Dialect ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setNumb( int m_Numb ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getNumb() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
// operations
// selections
@Override
public FunctionMessageSet R1010_is_invoked_by_FunctionMessage() {
return (new FunctionMessageSetImpl());
}
@Override
public FunctionParameterSet R24_defines_FunctionParameter() {
return (new FunctionParameterSetImpl());
}
@Override
public DataType R25_has_return_type_of_DataType() {
return DataTypeImpl.EMPTY_DATATYPE;
}
@Override
public DimensionsSet R51_return_value_may_have_Dimensions() {
return (new DimensionsSetImpl());
}
@Override
public FunctionInvocationSet R675_FunctionInvocation() {
return (new FunctionInvocationSetImpl());
}
@Override
public FunctionBody R695_FunctionBody() {
return FunctionBodyImpl.EMPTY_FUNCTIONBODY;
}
@Override
public PackageableElement R8001_is_a_PackageableElement() {
return PackageableElementImpl.EMPTY_PACKAGEABLEELEMENT;
}
@Override
public FunctionValueSet R827_FunctionValue() {
return (new FunctionValueSetImpl());
}
@Override
public String getKeyLetters() {
return S_SYNCImpl.KEY_LETTERS;
}
@Override
public S_SYNC self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public S_SYNC oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return S_SYNCImpl.EMPTY_S_SYNC;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy