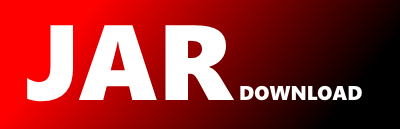
io.ciera.tool.sql.ooaofooa.elementpackaging.impl.EP_PKGImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.elementpackaging.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.domain.SystemModel;
import io.ciera.tool.sql.ooaofooa.domain.impl.SystemModelImpl;
import io.ciera.tool.sql.ooaofooa.elementpackaging.EP_PKG;
import io.ciera.tool.sql.ooaofooa.elementpackaging.PackageReference;
import io.ciera.tool.sql.ooaofooa.elementpackaging.PackageReferenceSet;
import io.ciera.tool.sql.ooaofooa.elementpackaging.impl.PackageReferenceImpl;
import io.ciera.tool.sql.ooaofooa.elementpackaging.impl.PackageReferenceSetImpl;
import io.ciera.tool.sql.ooaofooa.instance.ComponentInstance;
import io.ciera.tool.sql.ooaofooa.instance.impl.ComponentInstanceImpl;
import io.ciera.tool.sql.ooaofooa.interaction.PackageParticipant;
import io.ciera.tool.sql.ooaofooa.interaction.PackageParticipantSet;
import io.ciera.tool.sql.ooaofooa.interaction.impl.PackageParticipantSetImpl;
import io.ciera.tool.sql.ooaofooa.packageableelement.ElementVisibility;
import io.ciera.tool.sql.ooaofooa.packageableelement.ElementVisibilitySet;
import io.ciera.tool.sql.ooaofooa.packageableelement.PackageableElement;
import io.ciera.tool.sql.ooaofooa.packageableelement.PackageableElementSet;
import io.ciera.tool.sql.ooaofooa.packageableelement.SearchResultSet;
import io.ciera.tool.sql.ooaofooa.packageableelement.SearchResultSetSet;
import io.ciera.tool.sql.ooaofooa.packageableelement.impl.ElementVisibilitySetImpl;
import io.ciera.tool.sql.ooaofooa.packageableelement.impl.PackageableElementImpl;
import io.ciera.tool.sql.ooaofooa.packageableelement.impl.PackageableElementSetImpl;
import io.ciera.tool.sql.ooaofooa.packageableelement.impl.SearchResultSetSetImpl;
public class EP_PKGImpl extends ModelInstance implements EP_PKG {
public static final String KEY_LETTERS = "EP_PKG";
public static final EP_PKG EMPTY_EP_PKG = new EmptyEP_PKG();
private Sql context;
// constructors
private EP_PKGImpl( Sql context ) {
this.context = context;
ref_Package_ID = UniqueId.random();
ref_Sys_ID = UniqueId.random();
ref_Direct_Sys_ID = UniqueId.random();
m_Name = "";
m_Descrip = "";
m_Num_Rng = 0;
R1401_directly_contained_under_SystemModel_inst = SystemModelImpl.EMPTY_SYSTEMMODEL;
R1402_is_referenced_by_PackageReference_set = new PackageReferenceSetImpl();
R1402_refers_to_PackageReference_inst = PackageReferenceImpl.EMPTY_PACKAGEREFERENCE;
R1405_SystemModel_inst = SystemModelImpl.EMPTY_SYSTEMMODEL;
R2970_is_being_verified_by_ComponentInstance_inst = ComponentInstanceImpl.EMPTY_COMPONENTINSTANCE;
R8000_contains_PackageableElement_set = new PackageableElementSetImpl();
R8001_is_a_PackageableElement_inst = PackageableElementImpl.EMPTY_PACKAGEABLEELEMENT;
R8002_has_visibility_of_ElementVisibility_set = new ElementVisibilitySetImpl();
R8005_holds_SearchResultSet_set = new SearchResultSetSetImpl();
R956_represents_participant_of_PackageParticipant_set = new PackageParticipantSetImpl();
}
private EP_PKGImpl( Sql context, UniqueId instanceId, UniqueId ref_Package_ID, UniqueId ref_Sys_ID, UniqueId ref_Direct_Sys_ID, String m_Name, String m_Descrip, int m_Num_Rng ) {
super(instanceId);
this.context = context;
this.ref_Package_ID = ref_Package_ID;
this.ref_Sys_ID = ref_Sys_ID;
this.ref_Direct_Sys_ID = ref_Direct_Sys_ID;
this.m_Name = m_Name;
this.m_Descrip = m_Descrip;
this.m_Num_Rng = m_Num_Rng;
R1401_directly_contained_under_SystemModel_inst = SystemModelImpl.EMPTY_SYSTEMMODEL;
R1402_is_referenced_by_PackageReference_set = new PackageReferenceSetImpl();
R1402_refers_to_PackageReference_inst = PackageReferenceImpl.EMPTY_PACKAGEREFERENCE;
R1405_SystemModel_inst = SystemModelImpl.EMPTY_SYSTEMMODEL;
R2970_is_being_verified_by_ComponentInstance_inst = ComponentInstanceImpl.EMPTY_COMPONENTINSTANCE;
R8000_contains_PackageableElement_set = new PackageableElementSetImpl();
R8001_is_a_PackageableElement_inst = PackageableElementImpl.EMPTY_PACKAGEABLEELEMENT;
R8002_has_visibility_of_ElementVisibility_set = new ElementVisibilitySetImpl();
R8005_holds_SearchResultSet_set = new SearchResultSetSetImpl();
R956_represents_participant_of_PackageParticipant_set = new PackageParticipantSetImpl();
}
public static EP_PKG create( Sql context ) throws XtumlException {
EP_PKG newEP_PKG = new EP_PKGImpl( context );
if ( context.addInstance( newEP_PKG ) ) {
newEP_PKG.getRunContext().addChange(new InstanceCreatedDelta(newEP_PKG, KEY_LETTERS));
return newEP_PKG;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static EP_PKG create( Sql context, UniqueId ref_Package_ID, UniqueId ref_Sys_ID, UniqueId ref_Direct_Sys_ID, String m_Name, String m_Descrip, int m_Num_Rng ) throws XtumlException {
return create(context, UniqueId.random(), ref_Package_ID, ref_Sys_ID, ref_Direct_Sys_ID, m_Name, m_Descrip, m_Num_Rng);
}
public static EP_PKG create( Sql context, UniqueId instanceId, UniqueId ref_Package_ID, UniqueId ref_Sys_ID, UniqueId ref_Direct_Sys_ID, String m_Name, String m_Descrip, int m_Num_Rng ) throws XtumlException {
EP_PKG newEP_PKG = new EP_PKGImpl( context, instanceId, ref_Package_ID, ref_Sys_ID, ref_Direct_Sys_ID, m_Name, m_Descrip, m_Num_Rng );
if ( context.addInstance( newEP_PKG ) ) {
return newEP_PKG;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId ref_Package_ID;
@Override
public void setPackage_ID(UniqueId ref_Package_ID) throws XtumlException {
checkLiving();
if (ref_Package_ID.inequality( this.ref_Package_ID)) {
final UniqueId oldValue = this.ref_Package_ID;
this.ref_Package_ID = ref_Package_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Package_ID", oldValue, this.ref_Package_ID));
if ( !R1402_is_referenced_by_PackageReference().isEmpty() ) R1402_is_referenced_by_PackageReference().setReferred_Package_ID( ref_Package_ID );
if ( !R8002_has_visibility_of_ElementVisibility().isEmpty() ) R8002_has_visibility_of_ElementVisibility().setPackage_ID( ref_Package_ID );
if ( !R956_represents_participant_of_PackageParticipant().isEmpty() ) R956_represents_participant_of_PackageParticipant().setPackage_ID( ref_Package_ID );
if ( !R2970_is_being_verified_by_ComponentInstance().isEmpty() ) R2970_is_being_verified_by_ComponentInstance().setPackage_ID( ref_Package_ID );
if ( !R8000_contains_PackageableElement().isEmpty() ) R8000_contains_PackageableElement().setPackage_ID( ref_Package_ID );
if ( !R8005_holds_SearchResultSet().isEmpty() ) R8005_holds_SearchResultSet().setPackage_ID( ref_Package_ID );
if ( !R1402_refers_to_PackageReference().isEmpty() ) R1402_refers_to_PackageReference().setReferring_Package_ID( ref_Package_ID );
}
}
@Override
public UniqueId getPackage_ID() throws XtumlException {
checkLiving();
return ref_Package_ID;
}
private UniqueId ref_Sys_ID;
@Override
public UniqueId getSys_ID() throws XtumlException {
checkLiving();
return ref_Sys_ID;
}
@Override
public void setSys_ID(UniqueId ref_Sys_ID) throws XtumlException {
checkLiving();
if (ref_Sys_ID.inequality( this.ref_Sys_ID)) {
final UniqueId oldValue = this.ref_Sys_ID;
this.ref_Sys_ID = ref_Sys_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Sys_ID", oldValue, this.ref_Sys_ID));
}
}
private UniqueId ref_Direct_Sys_ID;
@Override
public void setDirect_Sys_ID(UniqueId ref_Direct_Sys_ID) throws XtumlException {
checkLiving();
if (ref_Direct_Sys_ID.inequality( this.ref_Direct_Sys_ID)) {
final UniqueId oldValue = this.ref_Direct_Sys_ID;
this.ref_Direct_Sys_ID = ref_Direct_Sys_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Direct_Sys_ID", oldValue, this.ref_Direct_Sys_ID));
}
}
@Override
public UniqueId getDirect_Sys_ID() throws XtumlException {
checkLiving();
return ref_Direct_Sys_ID;
}
private String m_Name;
@Override
public void setName(String m_Name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Name, this.m_Name)) {
final String oldValue = this.m_Name;
this.m_Name = m_Name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Name", oldValue, this.m_Name));
}
}
@Override
public String getName() throws XtumlException {
checkLiving();
return m_Name;
}
private String m_Descrip;
@Override
public void setDescrip(String m_Descrip) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Descrip, this.m_Descrip)) {
final String oldValue = this.m_Descrip;
this.m_Descrip = m_Descrip;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Descrip", oldValue, this.m_Descrip));
}
}
@Override
public String getDescrip() throws XtumlException {
checkLiving();
return m_Descrip;
}
private int m_Num_Rng;
@Override
public int getNum_Rng() throws XtumlException {
checkLiving();
return m_Num_Rng;
}
@Override
public void setNum_Rng(int m_Num_Rng) throws XtumlException {
checkLiving();
if (m_Num_Rng != this.m_Num_Rng) {
final int oldValue = this.m_Num_Rng;
this.m_Num_Rng = m_Num_Rng;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Num_Rng", oldValue, this.m_Num_Rng));
}
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getPackage_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private SystemModel R1401_directly_contained_under_SystemModel_inst;
@Override
public void setR1401_directly_contained_under_SystemModel( SystemModel inst ) {
R1401_directly_contained_under_SystemModel_inst = inst;
}
@Override
public SystemModel R1401_directly_contained_under_SystemModel() throws XtumlException {
return R1401_directly_contained_under_SystemModel_inst;
}
private PackageReferenceSet R1402_is_referenced_by_PackageReference_set;
@Override
public void addR1402_is_referenced_by_PackageReference( PackageReference inst ) {
R1402_is_referenced_by_PackageReference_set.add(inst);
}
@Override
public void removeR1402_is_referenced_by_PackageReference( PackageReference inst ) {
R1402_is_referenced_by_PackageReference_set.remove(inst);
}
@Override
public PackageReferenceSet R1402_is_referenced_by_PackageReference() throws XtumlException {
return R1402_is_referenced_by_PackageReference_set;
}
private PackageReference R1402_refers_to_PackageReference_inst;
@Override
public void setR1402_refers_to_PackageReference( PackageReference inst ) {
R1402_refers_to_PackageReference_inst = inst;
}
@Override
public PackageReference R1402_refers_to_PackageReference() throws XtumlException {
return R1402_refers_to_PackageReference_inst;
}
private SystemModel R1405_SystemModel_inst;
@Override
public void setR1405_SystemModel( SystemModel inst ) {
R1405_SystemModel_inst = inst;
}
@Override
public SystemModel R1405_SystemModel() throws XtumlException {
return R1405_SystemModel_inst;
}
private ComponentInstance R2970_is_being_verified_by_ComponentInstance_inst;
@Override
public void setR2970_is_being_verified_by_ComponentInstance( ComponentInstance inst ) {
R2970_is_being_verified_by_ComponentInstance_inst = inst;
}
@Override
public ComponentInstance R2970_is_being_verified_by_ComponentInstance() throws XtumlException {
return R2970_is_being_verified_by_ComponentInstance_inst;
}
private PackageableElementSet R8000_contains_PackageableElement_set;
@Override
public void addR8000_contains_PackageableElement( PackageableElement inst ) {
R8000_contains_PackageableElement_set.add(inst);
}
@Override
public void removeR8000_contains_PackageableElement( PackageableElement inst ) {
R8000_contains_PackageableElement_set.remove(inst);
}
@Override
public PackageableElementSet R8000_contains_PackageableElement() throws XtumlException {
return R8000_contains_PackageableElement_set;
}
private PackageableElement R8001_is_a_PackageableElement_inst;
@Override
public void setR8001_is_a_PackageableElement( PackageableElement inst ) {
R8001_is_a_PackageableElement_inst = inst;
}
@Override
public PackageableElement R8001_is_a_PackageableElement() throws XtumlException {
return R8001_is_a_PackageableElement_inst;
}
private ElementVisibilitySet R8002_has_visibility_of_ElementVisibility_set;
@Override
public void addR8002_has_visibility_of_ElementVisibility( ElementVisibility inst ) {
R8002_has_visibility_of_ElementVisibility_set.add(inst);
}
@Override
public void removeR8002_has_visibility_of_ElementVisibility( ElementVisibility inst ) {
R8002_has_visibility_of_ElementVisibility_set.remove(inst);
}
@Override
public ElementVisibilitySet R8002_has_visibility_of_ElementVisibility() throws XtumlException {
return R8002_has_visibility_of_ElementVisibility_set;
}
private SearchResultSetSet R8005_holds_SearchResultSet_set;
@Override
public void addR8005_holds_SearchResultSet( SearchResultSet inst ) {
R8005_holds_SearchResultSet_set.add(inst);
}
@Override
public void removeR8005_holds_SearchResultSet( SearchResultSet inst ) {
R8005_holds_SearchResultSet_set.remove(inst);
}
@Override
public SearchResultSetSet R8005_holds_SearchResultSet() throws XtumlException {
return R8005_holds_SearchResultSet_set;
}
private PackageParticipantSet R956_represents_participant_of_PackageParticipant_set;
@Override
public void addR956_represents_participant_of_PackageParticipant( PackageParticipant inst ) {
R956_represents_participant_of_PackageParticipant_set.add(inst);
}
@Override
public void removeR956_represents_participant_of_PackageParticipant( PackageParticipant inst ) {
R956_represents_participant_of_PackageParticipant_set.remove(inst);
}
@Override
public PackageParticipantSet R956_represents_participant_of_PackageParticipant() throws XtumlException {
return R956_represents_participant_of_PackageParticipant_set;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public EP_PKG self() {
return this;
}
@Override
public EP_PKG oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_EP_PKG;
}
}
class EmptyEP_PKG extends ModelInstance implements EP_PKG {
// attributes
public void setPackage_ID( UniqueId ref_Package_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getPackage_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public UniqueId getSys_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setSys_ID( UniqueId ref_Sys_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setDirect_Sys_ID( UniqueId ref_Direct_Sys_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getDirect_Sys_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setName( String m_Name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getName() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setDescrip( String m_Descrip ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getDescrip() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public int getNum_Rng() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setNum_Rng( int m_Num_Rng ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
// operations
// selections
@Override
public SystemModel R1401_directly_contained_under_SystemModel() {
return SystemModelImpl.EMPTY_SYSTEMMODEL;
}
@Override
public PackageReferenceSet R1402_is_referenced_by_PackageReference() {
return (new PackageReferenceSetImpl());
}
@Override
public PackageReference R1402_refers_to_PackageReference() {
return PackageReferenceImpl.EMPTY_PACKAGEREFERENCE;
}
@Override
public SystemModel R1405_SystemModel() {
return SystemModelImpl.EMPTY_SYSTEMMODEL;
}
@Override
public ComponentInstance R2970_is_being_verified_by_ComponentInstance() {
return ComponentInstanceImpl.EMPTY_COMPONENTINSTANCE;
}
@Override
public PackageableElementSet R8000_contains_PackageableElement() {
return (new PackageableElementSetImpl());
}
@Override
public PackageableElement R8001_is_a_PackageableElement() {
return PackageableElementImpl.EMPTY_PACKAGEABLEELEMENT;
}
@Override
public ElementVisibilitySet R8002_has_visibility_of_ElementVisibility() {
return (new ElementVisibilitySetImpl());
}
@Override
public SearchResultSetSet R8005_holds_SearchResultSet() {
return (new SearchResultSetSetImpl());
}
@Override
public PackageParticipantSet R956_represents_participant_of_PackageParticipant() {
return (new PackageParticipantSetImpl());
}
@Override
public String getKeyLetters() {
return EP_PKGImpl.KEY_LETTERS;
}
@Override
public EP_PKG self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public EP_PKG oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return EP_PKGImpl.EMPTY_EP_PKG;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy