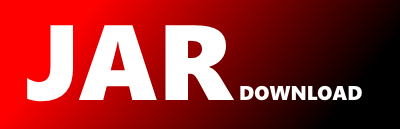
io.ciera.tool.sql.ooaofooa.instance.impl.I_INSImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.instance.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.instance.AttributeValue;
import io.ciera.tool.sql.ooaofooa.instance.AttributeValueSet;
import io.ciera.tool.sql.ooaofooa.instance.ComponentInstance;
import io.ciera.tool.sql.ooaofooa.instance.I_INS;
import io.ciera.tool.sql.ooaofooa.instance.LinkParticipation;
import io.ciera.tool.sql.ooaofooa.instance.LinkParticipationSet;
import io.ciera.tool.sql.ooaofooa.instance.Monitor;
import io.ciera.tool.sql.ooaofooa.instance.PendingEvent;
import io.ciera.tool.sql.ooaofooa.instance.PendingEventSet;
import io.ciera.tool.sql.ooaofooa.instance.StackFrame;
import io.ciera.tool.sql.ooaofooa.instance.StackFrameSet;
import io.ciera.tool.sql.ooaofooa.instance.impl.AttributeValueSetImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.ComponentInstanceImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.LinkParticipationSetImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.MonitorImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.PendingEventSetImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.StackFrameSetImpl;
import io.ciera.tool.sql.ooaofooa.statemachine.StateMachineState;
import io.ciera.tool.sql.ooaofooa.statemachine.Transition;
import io.ciera.tool.sql.ooaofooa.statemachine.impl.StateMachineStateImpl;
import io.ciera.tool.sql.ooaofooa.statemachine.impl.TransitionImpl;
public class I_INSImpl extends ModelInstance implements I_INS {
public static final String KEY_LETTERS = "I_INS";
public static final I_INS EMPTY_I_INS = new EmptyI_INS();
private Sql context;
// constructors
private I_INSImpl( Sql context ) {
this.context = context;
m_Inst_ID = UniqueId.random();
m_Name = "";
ref_SM_ID = UniqueId.random();
ref_SMstt_ID = UniqueId.random();
ref_Execution_Engine_ID = UniqueId.random();
ref_Trans_ID = UniqueId.random();
m_CIE_ID = UniqueId.random();
m_Label = "";
m_Default_Name = "";
R2907_has_waiting_PendingEvent_set = new PendingEventSetImpl();
R2909_has_characteristic_abstracted_by_AttributeValue_set = new AttributeValueSetImpl();
R2915_occupies_StateMachineState_inst = StateMachineStateImpl.EMPTY_STATEMACHINESTATE;
R2935_is_target_of_PendingEvent_set = new PendingEventSetImpl();
R2937_sends_PendingEvent_set = new PendingEventSetImpl();
R2949_monitored_by_Monitor_inst = MonitorImpl.EMPTY_MONITOR;
R2953_entered_last_state_via_Transition_inst = TransitionImpl.EMPTY_TRANSITION;
R2954_provides_context_for_StackFrame_set = new StackFrameSetImpl();
R2957_created_by_ComponentInstance_inst = ComponentInstanceImpl.EMPTY_COMPONENTINSTANCE;
R2958_LinkParticipation_set = new LinkParticipationSetImpl();
}
private I_INSImpl( Sql context, UniqueId instanceId, UniqueId m_Inst_ID, String m_Name, UniqueId ref_SM_ID, UniqueId ref_SMstt_ID, UniqueId ref_Execution_Engine_ID, UniqueId ref_Trans_ID, UniqueId m_CIE_ID, String m_Label, String m_Default_Name ) {
super(instanceId);
this.context = context;
this.m_Inst_ID = m_Inst_ID;
this.m_Name = m_Name;
this.ref_SM_ID = ref_SM_ID;
this.ref_SMstt_ID = ref_SMstt_ID;
this.ref_Execution_Engine_ID = ref_Execution_Engine_ID;
this.ref_Trans_ID = ref_Trans_ID;
this.m_CIE_ID = m_CIE_ID;
this.m_Label = m_Label;
this.m_Default_Name = m_Default_Name;
R2907_has_waiting_PendingEvent_set = new PendingEventSetImpl();
R2909_has_characteristic_abstracted_by_AttributeValue_set = new AttributeValueSetImpl();
R2915_occupies_StateMachineState_inst = StateMachineStateImpl.EMPTY_STATEMACHINESTATE;
R2935_is_target_of_PendingEvent_set = new PendingEventSetImpl();
R2937_sends_PendingEvent_set = new PendingEventSetImpl();
R2949_monitored_by_Monitor_inst = MonitorImpl.EMPTY_MONITOR;
R2953_entered_last_state_via_Transition_inst = TransitionImpl.EMPTY_TRANSITION;
R2954_provides_context_for_StackFrame_set = new StackFrameSetImpl();
R2957_created_by_ComponentInstance_inst = ComponentInstanceImpl.EMPTY_COMPONENTINSTANCE;
R2958_LinkParticipation_set = new LinkParticipationSetImpl();
}
public static I_INS create( Sql context ) throws XtumlException {
I_INS newI_INS = new I_INSImpl( context );
if ( context.addInstance( newI_INS ) ) {
newI_INS.getRunContext().addChange(new InstanceCreatedDelta(newI_INS, KEY_LETTERS));
return newI_INS;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static I_INS create( Sql context, UniqueId m_Inst_ID, String m_Name, UniqueId ref_SM_ID, UniqueId ref_SMstt_ID, UniqueId ref_Execution_Engine_ID, UniqueId ref_Trans_ID, UniqueId m_CIE_ID, String m_Label, String m_Default_Name ) throws XtumlException {
return create(context, UniqueId.random(), m_Inst_ID, m_Name, ref_SM_ID, ref_SMstt_ID, ref_Execution_Engine_ID, ref_Trans_ID, m_CIE_ID, m_Label, m_Default_Name);
}
public static I_INS create( Sql context, UniqueId instanceId, UniqueId m_Inst_ID, String m_Name, UniqueId ref_SM_ID, UniqueId ref_SMstt_ID, UniqueId ref_Execution_Engine_ID, UniqueId ref_Trans_ID, UniqueId m_CIE_ID, String m_Label, String m_Default_Name ) throws XtumlException {
I_INS newI_INS = new I_INSImpl( context, instanceId, m_Inst_ID, m_Name, ref_SM_ID, ref_SMstt_ID, ref_Execution_Engine_ID, ref_Trans_ID, m_CIE_ID, m_Label, m_Default_Name );
if ( context.addInstance( newI_INS ) ) {
return newI_INS;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId m_Inst_ID;
@Override
public void setInst_ID(UniqueId m_Inst_ID) throws XtumlException {
checkLiving();
if (m_Inst_ID.inequality( this.m_Inst_ID)) {
final UniqueId oldValue = this.m_Inst_ID;
this.m_Inst_ID = m_Inst_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Inst_ID", oldValue, this.m_Inst_ID));
if ( !R2949_monitored_by_Monitor().isEmpty() ) R2949_monitored_by_Monitor().setInst_ID( m_Inst_ID );
if ( !R2954_provides_context_for_StackFrame().isEmpty() ) R2954_provides_context_for_StackFrame().setInst_ID( m_Inst_ID );
if ( !R2907_has_waiting_PendingEvent().isEmpty() ) R2907_has_waiting_PendingEvent().setTarget_Inst_ID( m_Inst_ID );
if ( !R2958_LinkParticipation().isEmpty() ) R2958_LinkParticipation().setInst_ID( m_Inst_ID );
if ( !R2937_sends_PendingEvent().isEmpty() ) R2937_sends_PendingEvent().setSent_By_Inst_ID( m_Inst_ID );
if ( !R2935_is_target_of_PendingEvent().isEmpty() ) R2935_is_target_of_PendingEvent().setTarget_Inst_ID( m_Inst_ID );
if ( !R2909_has_characteristic_abstracted_by_AttributeValue().isEmpty() ) R2909_has_characteristic_abstracted_by_AttributeValue().setInst_ID( m_Inst_ID );
}
}
@Override
public UniqueId getInst_ID() throws XtumlException {
checkLiving();
return m_Inst_ID;
}
private String m_Name;
@Override
public String getName() throws XtumlException {
checkLiving();
return m_Name;
}
@Override
public void setName(String m_Name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Name, this.m_Name)) {
final String oldValue = this.m_Name;
this.m_Name = m_Name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Name", oldValue, this.m_Name));
}
}
private UniqueId ref_SM_ID;
@Override
public UniqueId getSM_ID() throws XtumlException {
checkLiving();
return ref_SM_ID;
}
@Override
public void setSM_ID(UniqueId ref_SM_ID) throws XtumlException {
checkLiving();
if (ref_SM_ID.inequality( this.ref_SM_ID)) {
final UniqueId oldValue = this.ref_SM_ID;
this.ref_SM_ID = ref_SM_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_SM_ID", oldValue, this.ref_SM_ID));
}
}
private UniqueId ref_SMstt_ID;
@Override
public UniqueId getSMstt_ID() throws XtumlException {
checkLiving();
return ref_SMstt_ID;
}
@Override
public void setSMstt_ID(UniqueId ref_SMstt_ID) throws XtumlException {
checkLiving();
if (ref_SMstt_ID.inequality( this.ref_SMstt_ID)) {
final UniqueId oldValue = this.ref_SMstt_ID;
this.ref_SMstt_ID = ref_SMstt_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_SMstt_ID", oldValue, this.ref_SMstt_ID));
}
}
private UniqueId ref_Execution_Engine_ID;
@Override
public void setExecution_Engine_ID(UniqueId ref_Execution_Engine_ID) throws XtumlException {
checkLiving();
if (ref_Execution_Engine_ID.inequality( this.ref_Execution_Engine_ID)) {
final UniqueId oldValue = this.ref_Execution_Engine_ID;
this.ref_Execution_Engine_ID = ref_Execution_Engine_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Execution_Engine_ID", oldValue, this.ref_Execution_Engine_ID));
}
}
@Override
public UniqueId getExecution_Engine_ID() throws XtumlException {
checkLiving();
return ref_Execution_Engine_ID;
}
private UniqueId ref_Trans_ID;
@Override
public UniqueId getTrans_ID() throws XtumlException {
checkLiving();
return ref_Trans_ID;
}
@Override
public void setTrans_ID(UniqueId ref_Trans_ID) throws XtumlException {
checkLiving();
if (ref_Trans_ID.inequality( this.ref_Trans_ID)) {
final UniqueId oldValue = this.ref_Trans_ID;
this.ref_Trans_ID = ref_Trans_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Trans_ID", oldValue, this.ref_Trans_ID));
}
}
private UniqueId m_CIE_ID;
@Override
public void setCIE_ID(UniqueId m_CIE_ID) throws XtumlException {
checkLiving();
if (m_CIE_ID.inequality( this.m_CIE_ID)) {
final UniqueId oldValue = this.m_CIE_ID;
this.m_CIE_ID = m_CIE_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_CIE_ID", oldValue, this.m_CIE_ID));
}
}
@Override
public UniqueId getCIE_ID() throws XtumlException {
checkLiving();
return m_CIE_ID;
}
private String m_Label;
@Override
public String getLabel() throws XtumlException {
checkLiving();
return m_Label;
}
@Override
public void setLabel(String m_Label) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Label, this.m_Label)) {
final String oldValue = this.m_Label;
this.m_Label = m_Label;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Label", oldValue, this.m_Label));
}
}
private String m_Default_Name;
@Override
public void setDefault_Name(String m_Default_Name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Default_Name, this.m_Default_Name)) {
final String oldValue = this.m_Default_Name;
this.m_Default_Name = m_Default_Name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Default_Name", oldValue, this.m_Default_Name));
}
}
@Override
public String getDefault_Name() throws XtumlException {
checkLiving();
return m_Default_Name;
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getInst_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private PendingEventSet R2907_has_waiting_PendingEvent_set;
@Override
public void addR2907_has_waiting_PendingEvent( PendingEvent inst ) {
R2907_has_waiting_PendingEvent_set.add(inst);
}
@Override
public void removeR2907_has_waiting_PendingEvent( PendingEvent inst ) {
R2907_has_waiting_PendingEvent_set.remove(inst);
}
@Override
public PendingEventSet R2907_has_waiting_PendingEvent() throws XtumlException {
return R2907_has_waiting_PendingEvent_set;
}
private AttributeValueSet R2909_has_characteristic_abstracted_by_AttributeValue_set;
@Override
public void addR2909_has_characteristic_abstracted_by_AttributeValue( AttributeValue inst ) {
R2909_has_characteristic_abstracted_by_AttributeValue_set.add(inst);
}
@Override
public void removeR2909_has_characteristic_abstracted_by_AttributeValue( AttributeValue inst ) {
R2909_has_characteristic_abstracted_by_AttributeValue_set.remove(inst);
}
@Override
public AttributeValueSet R2909_has_characteristic_abstracted_by_AttributeValue() throws XtumlException {
return R2909_has_characteristic_abstracted_by_AttributeValue_set;
}
private StateMachineState R2915_occupies_StateMachineState_inst;
@Override
public void setR2915_occupies_StateMachineState( StateMachineState inst ) {
R2915_occupies_StateMachineState_inst = inst;
}
@Override
public StateMachineState R2915_occupies_StateMachineState() throws XtumlException {
return R2915_occupies_StateMachineState_inst;
}
private PendingEventSet R2935_is_target_of_PendingEvent_set;
@Override
public void addR2935_is_target_of_PendingEvent( PendingEvent inst ) {
R2935_is_target_of_PendingEvent_set.add(inst);
}
@Override
public void removeR2935_is_target_of_PendingEvent( PendingEvent inst ) {
R2935_is_target_of_PendingEvent_set.remove(inst);
}
@Override
public PendingEventSet R2935_is_target_of_PendingEvent() throws XtumlException {
return R2935_is_target_of_PendingEvent_set;
}
private PendingEventSet R2937_sends_PendingEvent_set;
@Override
public void addR2937_sends_PendingEvent( PendingEvent inst ) {
R2937_sends_PendingEvent_set.add(inst);
}
@Override
public void removeR2937_sends_PendingEvent( PendingEvent inst ) {
R2937_sends_PendingEvent_set.remove(inst);
}
@Override
public PendingEventSet R2937_sends_PendingEvent() throws XtumlException {
return R2937_sends_PendingEvent_set;
}
private Monitor R2949_monitored_by_Monitor_inst;
@Override
public void setR2949_monitored_by_Monitor( Monitor inst ) {
R2949_monitored_by_Monitor_inst = inst;
}
@Override
public Monitor R2949_monitored_by_Monitor() throws XtumlException {
return R2949_monitored_by_Monitor_inst;
}
private Transition R2953_entered_last_state_via_Transition_inst;
@Override
public void setR2953_entered_last_state_via_Transition( Transition inst ) {
R2953_entered_last_state_via_Transition_inst = inst;
}
@Override
public Transition R2953_entered_last_state_via_Transition() throws XtumlException {
return R2953_entered_last_state_via_Transition_inst;
}
private StackFrameSet R2954_provides_context_for_StackFrame_set;
@Override
public void addR2954_provides_context_for_StackFrame( StackFrame inst ) {
R2954_provides_context_for_StackFrame_set.add(inst);
}
@Override
public void removeR2954_provides_context_for_StackFrame( StackFrame inst ) {
R2954_provides_context_for_StackFrame_set.remove(inst);
}
@Override
public StackFrameSet R2954_provides_context_for_StackFrame() throws XtumlException {
return R2954_provides_context_for_StackFrame_set;
}
private ComponentInstance R2957_created_by_ComponentInstance_inst;
@Override
public void setR2957_created_by_ComponentInstance( ComponentInstance inst ) {
R2957_created_by_ComponentInstance_inst = inst;
}
@Override
public ComponentInstance R2957_created_by_ComponentInstance() throws XtumlException {
return R2957_created_by_ComponentInstance_inst;
}
private LinkParticipationSet R2958_LinkParticipation_set;
@Override
public void addR2958_LinkParticipation( LinkParticipation inst ) {
R2958_LinkParticipation_set.add(inst);
}
@Override
public void removeR2958_LinkParticipation( LinkParticipation inst ) {
R2958_LinkParticipation_set.remove(inst);
}
@Override
public LinkParticipationSet R2958_LinkParticipation() throws XtumlException {
return R2958_LinkParticipation_set;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public I_INS self() {
return this;
}
@Override
public I_INS oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_I_INS;
}
}
class EmptyI_INS extends ModelInstance implements I_INS {
// attributes
public void setInst_ID( UniqueId m_Inst_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getInst_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getName() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setName( String m_Name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getSM_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setSM_ID( UniqueId ref_SM_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getSMstt_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setSMstt_ID( UniqueId ref_SMstt_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setExecution_Engine_ID( UniqueId ref_Execution_Engine_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getExecution_Engine_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public UniqueId getTrans_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setTrans_ID( UniqueId ref_Trans_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setCIE_ID( UniqueId m_CIE_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getCIE_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getLabel() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setLabel( String m_Label ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setDefault_Name( String m_Default_Name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getDefault_Name() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
// operations
// selections
@Override
public PendingEventSet R2907_has_waiting_PendingEvent() {
return (new PendingEventSetImpl());
}
@Override
public AttributeValueSet R2909_has_characteristic_abstracted_by_AttributeValue() {
return (new AttributeValueSetImpl());
}
@Override
public StateMachineState R2915_occupies_StateMachineState() {
return StateMachineStateImpl.EMPTY_STATEMACHINESTATE;
}
@Override
public PendingEventSet R2935_is_target_of_PendingEvent() {
return (new PendingEventSetImpl());
}
@Override
public PendingEventSet R2937_sends_PendingEvent() {
return (new PendingEventSetImpl());
}
@Override
public Monitor R2949_monitored_by_Monitor() {
return MonitorImpl.EMPTY_MONITOR;
}
@Override
public Transition R2953_entered_last_state_via_Transition() {
return TransitionImpl.EMPTY_TRANSITION;
}
@Override
public StackFrameSet R2954_provides_context_for_StackFrame() {
return (new StackFrameSetImpl());
}
@Override
public ComponentInstance R2957_created_by_ComponentInstance() {
return ComponentInstanceImpl.EMPTY_COMPONENTINSTANCE;
}
@Override
public LinkParticipationSet R2958_LinkParticipation() {
return (new LinkParticipationSetImpl());
}
@Override
public String getKeyLetters() {
return I_INSImpl.KEY_LETTERS;
}
@Override
public I_INS self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public I_INS oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return I_INSImpl.EMPTY_I_INS;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy