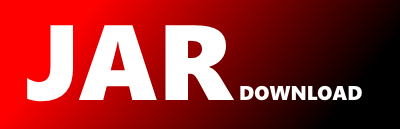
io.ciera.tool.sql.ooaofooa.instance.impl.PendingEventImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.instance.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.instance.ComponentInstance;
import io.ciera.tool.sql.ooaofooa.instance.DataItemValue;
import io.ciera.tool.sql.ooaofooa.instance.DataItemValueSet;
import io.ciera.tool.sql.ooaofooa.instance.EventQueueEntry;
import io.ciera.tool.sql.ooaofooa.instance.I_INS;
import io.ciera.tool.sql.ooaofooa.instance.PendingEvent;
import io.ciera.tool.sql.ooaofooa.instance.SelfQueueEntry;
import io.ciera.tool.sql.ooaofooa.instance.Timer;
import io.ciera.tool.sql.ooaofooa.instance.impl.ComponentInstanceImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.DataItemValueSetImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.EventQueueEntryImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.I_INSImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.PendingEventImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.SelfQueueEntryImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.TimerImpl;
import io.ciera.tool.sql.ooaofooa.statemachine.StateMachineEvent;
import io.ciera.tool.sql.ooaofooa.statemachine.impl.StateMachineEventImpl;
public class PendingEventImpl extends ModelInstance implements PendingEvent {
public static final String KEY_LETTERS = "I_EVI";
public static final PendingEvent EMPTY_PENDINGEVENT = new EmptyPendingEvent();
private Sql context;
// constructors
private PendingEventImpl( Sql context ) {
this.context = context;
m_Event_ID = UniqueId.random();
m_isExecuting = false;
m_isCreation = false;
ref_SMevt_ID = UniqueId.random();
ref_Target_Inst_ID = UniqueId.random();
ref_nextEvent_ID = UniqueId.random();
ref_Sent_By_Inst_ID = UniqueId.random();
ref_next_self_Event_ID = UniqueId.random();
m_Sent_By_CIE_ID = UniqueId.random();
m_CIE_ID = UniqueId.random();
ref_Execution_Engine_ID = UniqueId.random();
ref_Originating_Execution_Engine_ID = UniqueId.random();
m_Label = "";
R2906_is_instance_of_StateMachineEvent_inst = StateMachineEventImpl.EMPTY_STATEMACHINEEVENT;
R2907_is_pending_for_I_INS_inst = I_INSImpl.EMPTY_I_INS;
R2908_will_be_processed_after_PendingEvent_inst = PendingEventImpl.EMPTY_PENDINGEVENT;
R2908_will_be_processed_before_PendingEvent_inst = PendingEventImpl.EMPTY_PENDINGEVENT;
R2933_DataItemValue_set = new DataItemValueSetImpl();
R2935_targets_I_INS_inst = I_INSImpl.EMPTY_I_INS;
R2937_was_sent_from_I_INS_inst = I_INSImpl.EMPTY_I_INS;
R2939_will_be_processed_after_PendingEvent_inst = PendingEventImpl.EMPTY_PENDINGEVENT;
R2939_will_be_processed_before_PendingEvent_inst = PendingEventImpl.EMPTY_PENDINGEVENT;
R2940_delivered_by_Timer_inst = TimerImpl.EMPTY_TIMER;
R2944_EventQueueEntry_inst = EventQueueEntryImpl.EMPTY_EVENTQUEUEENTRY;
R2946_SelfQueueEntry_inst = SelfQueueEntryImpl.EMPTY_SELFQUEUEENTRY;
R2964_is_pending_in_ComponentInstance_inst = ComponentInstanceImpl.EMPTY_COMPONENTINSTANCE;
R2976_originates_from_ComponentInstance_inst = ComponentInstanceImpl.EMPTY_COMPONENTINSTANCE;
}
private PendingEventImpl( Sql context, UniqueId instanceId, UniqueId m_Event_ID, boolean m_isExecuting, boolean m_isCreation, UniqueId ref_SMevt_ID, UniqueId ref_Target_Inst_ID, UniqueId ref_nextEvent_ID, UniqueId ref_Sent_By_Inst_ID, UniqueId ref_next_self_Event_ID, UniqueId m_Sent_By_CIE_ID, UniqueId m_CIE_ID, UniqueId ref_Execution_Engine_ID, UniqueId ref_Originating_Execution_Engine_ID, String m_Label ) {
super(instanceId);
this.context = context;
this.m_Event_ID = m_Event_ID;
this.m_isExecuting = m_isExecuting;
this.m_isCreation = m_isCreation;
this.ref_SMevt_ID = ref_SMevt_ID;
this.ref_Target_Inst_ID = ref_Target_Inst_ID;
this.ref_nextEvent_ID = ref_nextEvent_ID;
this.ref_Sent_By_Inst_ID = ref_Sent_By_Inst_ID;
this.ref_next_self_Event_ID = ref_next_self_Event_ID;
this.m_Sent_By_CIE_ID = m_Sent_By_CIE_ID;
this.m_CIE_ID = m_CIE_ID;
this.ref_Execution_Engine_ID = ref_Execution_Engine_ID;
this.ref_Originating_Execution_Engine_ID = ref_Originating_Execution_Engine_ID;
this.m_Label = m_Label;
R2906_is_instance_of_StateMachineEvent_inst = StateMachineEventImpl.EMPTY_STATEMACHINEEVENT;
R2907_is_pending_for_I_INS_inst = I_INSImpl.EMPTY_I_INS;
R2908_will_be_processed_after_PendingEvent_inst = PendingEventImpl.EMPTY_PENDINGEVENT;
R2908_will_be_processed_before_PendingEvent_inst = PendingEventImpl.EMPTY_PENDINGEVENT;
R2933_DataItemValue_set = new DataItemValueSetImpl();
R2935_targets_I_INS_inst = I_INSImpl.EMPTY_I_INS;
R2937_was_sent_from_I_INS_inst = I_INSImpl.EMPTY_I_INS;
R2939_will_be_processed_after_PendingEvent_inst = PendingEventImpl.EMPTY_PENDINGEVENT;
R2939_will_be_processed_before_PendingEvent_inst = PendingEventImpl.EMPTY_PENDINGEVENT;
R2940_delivered_by_Timer_inst = TimerImpl.EMPTY_TIMER;
R2944_EventQueueEntry_inst = EventQueueEntryImpl.EMPTY_EVENTQUEUEENTRY;
R2946_SelfQueueEntry_inst = SelfQueueEntryImpl.EMPTY_SELFQUEUEENTRY;
R2964_is_pending_in_ComponentInstance_inst = ComponentInstanceImpl.EMPTY_COMPONENTINSTANCE;
R2976_originates_from_ComponentInstance_inst = ComponentInstanceImpl.EMPTY_COMPONENTINSTANCE;
}
public static PendingEvent create( Sql context ) throws XtumlException {
PendingEvent newPendingEvent = new PendingEventImpl( context );
if ( context.addInstance( newPendingEvent ) ) {
newPendingEvent.getRunContext().addChange(new InstanceCreatedDelta(newPendingEvent, KEY_LETTERS));
return newPendingEvent;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static PendingEvent create( Sql context, UniqueId m_Event_ID, boolean m_isExecuting, boolean m_isCreation, UniqueId ref_SMevt_ID, UniqueId ref_Target_Inst_ID, UniqueId ref_nextEvent_ID, UniqueId ref_Sent_By_Inst_ID, UniqueId ref_next_self_Event_ID, UniqueId m_Sent_By_CIE_ID, UniqueId m_CIE_ID, UniqueId ref_Execution_Engine_ID, UniqueId ref_Originating_Execution_Engine_ID, String m_Label ) throws XtumlException {
return create(context, UniqueId.random(), m_Event_ID, m_isExecuting, m_isCreation, ref_SMevt_ID, ref_Target_Inst_ID, ref_nextEvent_ID, ref_Sent_By_Inst_ID, ref_next_self_Event_ID, m_Sent_By_CIE_ID, m_CIE_ID, ref_Execution_Engine_ID, ref_Originating_Execution_Engine_ID, m_Label);
}
public static PendingEvent create( Sql context, UniqueId instanceId, UniqueId m_Event_ID, boolean m_isExecuting, boolean m_isCreation, UniqueId ref_SMevt_ID, UniqueId ref_Target_Inst_ID, UniqueId ref_nextEvent_ID, UniqueId ref_Sent_By_Inst_ID, UniqueId ref_next_self_Event_ID, UniqueId m_Sent_By_CIE_ID, UniqueId m_CIE_ID, UniqueId ref_Execution_Engine_ID, UniqueId ref_Originating_Execution_Engine_ID, String m_Label ) throws XtumlException {
PendingEvent newPendingEvent = new PendingEventImpl( context, instanceId, m_Event_ID, m_isExecuting, m_isCreation, ref_SMevt_ID, ref_Target_Inst_ID, ref_nextEvent_ID, ref_Sent_By_Inst_ID, ref_next_self_Event_ID, m_Sent_By_CIE_ID, m_CIE_ID, ref_Execution_Engine_ID, ref_Originating_Execution_Engine_ID, m_Label );
if ( context.addInstance( newPendingEvent ) ) {
return newPendingEvent;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId m_Event_ID;
@Override
public UniqueId getEvent_ID() throws XtumlException {
checkLiving();
return m_Event_ID;
}
@Override
public void setEvent_ID(UniqueId m_Event_ID) throws XtumlException {
checkLiving();
if (m_Event_ID.inequality( this.m_Event_ID)) {
final UniqueId oldValue = this.m_Event_ID;
this.m_Event_ID = m_Event_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Event_ID", oldValue, this.m_Event_ID));
if ( !R2940_delivered_by_Timer().isEmpty() ) R2940_delivered_by_Timer().setEvent_ID( m_Event_ID );
if ( !R2939_will_be_processed_after_PendingEvent().isEmpty() ) R2939_will_be_processed_after_PendingEvent().setNext_self_Event_ID( m_Event_ID );
if ( !R2908_will_be_processed_before_PendingEvent().isEmpty() ) R2908_will_be_processed_before_PendingEvent().setNextEvent_ID( m_Event_ID );
if ( !R2944_EventQueueEntry().isEmpty() ) R2944_EventQueueEntry().setEvent_ID( m_Event_ID );
if ( !R2946_SelfQueueEntry().isEmpty() ) R2946_SelfQueueEntry().setEvent_ID( m_Event_ID );
if ( !R2933_DataItemValue().isEmpty() ) R2933_DataItemValue().setEvent_ID( m_Event_ID );
}
}
private boolean m_isExecuting;
@Override
public void setIsExecuting(boolean m_isExecuting) throws XtumlException {
checkLiving();
if (m_isExecuting != this.m_isExecuting) {
final boolean oldValue = this.m_isExecuting;
this.m_isExecuting = m_isExecuting;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_isExecuting", oldValue, this.m_isExecuting));
}
}
@Override
public boolean getIsExecuting() throws XtumlException {
checkLiving();
return m_isExecuting;
}
private boolean m_isCreation;
@Override
public boolean getIsCreation() throws XtumlException {
checkLiving();
return m_isCreation;
}
@Override
public void setIsCreation(boolean m_isCreation) throws XtumlException {
checkLiving();
if (m_isCreation != this.m_isCreation) {
final boolean oldValue = this.m_isCreation;
this.m_isCreation = m_isCreation;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_isCreation", oldValue, this.m_isCreation));
}
}
private UniqueId ref_SMevt_ID;
@Override
public UniqueId getSMevt_ID() throws XtumlException {
checkLiving();
return ref_SMevt_ID;
}
@Override
public void setSMevt_ID(UniqueId ref_SMevt_ID) throws XtumlException {
checkLiving();
if (ref_SMevt_ID.inequality( this.ref_SMevt_ID)) {
final UniqueId oldValue = this.ref_SMevt_ID;
this.ref_SMevt_ID = ref_SMevt_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_SMevt_ID", oldValue, this.ref_SMevt_ID));
}
}
private UniqueId ref_Target_Inst_ID;
@Override
public void setTarget_Inst_ID(UniqueId ref_Target_Inst_ID) throws XtumlException {
checkLiving();
if (ref_Target_Inst_ID.inequality( this.ref_Target_Inst_ID)) {
final UniqueId oldValue = this.ref_Target_Inst_ID;
this.ref_Target_Inst_ID = ref_Target_Inst_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Target_Inst_ID", oldValue, this.ref_Target_Inst_ID));
}
}
@Override
public UniqueId getTarget_Inst_ID() throws XtumlException {
checkLiving();
return ref_Target_Inst_ID;
}
private UniqueId ref_nextEvent_ID;
@Override
public void setNextEvent_ID(UniqueId ref_nextEvent_ID) throws XtumlException {
checkLiving();
if (ref_nextEvent_ID.inequality( this.ref_nextEvent_ID)) {
final UniqueId oldValue = this.ref_nextEvent_ID;
this.ref_nextEvent_ID = ref_nextEvent_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_nextEvent_ID", oldValue, this.ref_nextEvent_ID));
}
}
@Override
public UniqueId getNextEvent_ID() throws XtumlException {
checkLiving();
return ref_nextEvent_ID;
}
private UniqueId ref_Sent_By_Inst_ID;
@Override
public UniqueId getSent_By_Inst_ID() throws XtumlException {
checkLiving();
return ref_Sent_By_Inst_ID;
}
@Override
public void setSent_By_Inst_ID(UniqueId ref_Sent_By_Inst_ID) throws XtumlException {
checkLiving();
if (ref_Sent_By_Inst_ID.inequality( this.ref_Sent_By_Inst_ID)) {
final UniqueId oldValue = this.ref_Sent_By_Inst_ID;
this.ref_Sent_By_Inst_ID = ref_Sent_By_Inst_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Sent_By_Inst_ID", oldValue, this.ref_Sent_By_Inst_ID));
}
}
private UniqueId ref_next_self_Event_ID;
@Override
public void setNext_self_Event_ID(UniqueId ref_next_self_Event_ID) throws XtumlException {
checkLiving();
if (ref_next_self_Event_ID.inequality( this.ref_next_self_Event_ID)) {
final UniqueId oldValue = this.ref_next_self_Event_ID;
this.ref_next_self_Event_ID = ref_next_self_Event_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_next_self_Event_ID", oldValue, this.ref_next_self_Event_ID));
}
}
@Override
public UniqueId getNext_self_Event_ID() throws XtumlException {
checkLiving();
return ref_next_self_Event_ID;
}
private UniqueId m_Sent_By_CIE_ID;
@Override
public UniqueId getSent_By_CIE_ID() throws XtumlException {
checkLiving();
return m_Sent_By_CIE_ID;
}
@Override
public void setSent_By_CIE_ID(UniqueId m_Sent_By_CIE_ID) throws XtumlException {
checkLiving();
if (m_Sent_By_CIE_ID.inequality( this.m_Sent_By_CIE_ID)) {
final UniqueId oldValue = this.m_Sent_By_CIE_ID;
this.m_Sent_By_CIE_ID = m_Sent_By_CIE_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Sent_By_CIE_ID", oldValue, this.m_Sent_By_CIE_ID));
}
}
private UniqueId m_CIE_ID;
@Override
public UniqueId getCIE_ID() throws XtumlException {
checkLiving();
return m_CIE_ID;
}
@Override
public void setCIE_ID(UniqueId m_CIE_ID) throws XtumlException {
checkLiving();
if (m_CIE_ID.inequality( this.m_CIE_ID)) {
final UniqueId oldValue = this.m_CIE_ID;
this.m_CIE_ID = m_CIE_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_CIE_ID", oldValue, this.m_CIE_ID));
}
}
private UniqueId ref_Execution_Engine_ID;
@Override
public UniqueId getExecution_Engine_ID() throws XtumlException {
checkLiving();
return ref_Execution_Engine_ID;
}
@Override
public void setExecution_Engine_ID(UniqueId ref_Execution_Engine_ID) throws XtumlException {
checkLiving();
if (ref_Execution_Engine_ID.inequality( this.ref_Execution_Engine_ID)) {
final UniqueId oldValue = this.ref_Execution_Engine_ID;
this.ref_Execution_Engine_ID = ref_Execution_Engine_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Execution_Engine_ID", oldValue, this.ref_Execution_Engine_ID));
}
}
private UniqueId ref_Originating_Execution_Engine_ID;
@Override
public void setOriginating_Execution_Engine_ID(UniqueId ref_Originating_Execution_Engine_ID) throws XtumlException {
checkLiving();
if (ref_Originating_Execution_Engine_ID.inequality( this.ref_Originating_Execution_Engine_ID)) {
final UniqueId oldValue = this.ref_Originating_Execution_Engine_ID;
this.ref_Originating_Execution_Engine_ID = ref_Originating_Execution_Engine_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Originating_Execution_Engine_ID", oldValue, this.ref_Originating_Execution_Engine_ID));
}
}
@Override
public UniqueId getOriginating_Execution_Engine_ID() throws XtumlException {
checkLiving();
return ref_Originating_Execution_Engine_ID;
}
private String m_Label;
@Override
public void setLabel(String m_Label) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Label, this.m_Label)) {
final String oldValue = this.m_Label;
this.m_Label = m_Label;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Label", oldValue, this.m_Label));
}
}
@Override
public String getLabel() throws XtumlException {
checkLiving();
return m_Label;
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getEvent_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private StateMachineEvent R2906_is_instance_of_StateMachineEvent_inst;
@Override
public void setR2906_is_instance_of_StateMachineEvent( StateMachineEvent inst ) {
R2906_is_instance_of_StateMachineEvent_inst = inst;
}
@Override
public StateMachineEvent R2906_is_instance_of_StateMachineEvent() throws XtumlException {
return R2906_is_instance_of_StateMachineEvent_inst;
}
private I_INS R2907_is_pending_for_I_INS_inst;
@Override
public void setR2907_is_pending_for_I_INS( I_INS inst ) {
R2907_is_pending_for_I_INS_inst = inst;
}
@Override
public I_INS R2907_is_pending_for_I_INS() throws XtumlException {
return R2907_is_pending_for_I_INS_inst;
}
private PendingEvent R2908_will_be_processed_after_PendingEvent_inst;
@Override
public void setR2908_will_be_processed_after_PendingEvent( PendingEvent inst ) {
R2908_will_be_processed_after_PendingEvent_inst = inst;
}
@Override
public PendingEvent R2908_will_be_processed_after_PendingEvent() throws XtumlException {
return R2908_will_be_processed_after_PendingEvent_inst;
}
private PendingEvent R2908_will_be_processed_before_PendingEvent_inst;
@Override
public void setR2908_will_be_processed_before_PendingEvent( PendingEvent inst ) {
R2908_will_be_processed_before_PendingEvent_inst = inst;
}
@Override
public PendingEvent R2908_will_be_processed_before_PendingEvent() throws XtumlException {
return R2908_will_be_processed_before_PendingEvent_inst;
}
private DataItemValueSet R2933_DataItemValue_set;
@Override
public void addR2933_DataItemValue( DataItemValue inst ) {
R2933_DataItemValue_set.add(inst);
}
@Override
public void removeR2933_DataItemValue( DataItemValue inst ) {
R2933_DataItemValue_set.remove(inst);
}
@Override
public DataItemValueSet R2933_DataItemValue() throws XtumlException {
return R2933_DataItemValue_set;
}
private I_INS R2935_targets_I_INS_inst;
@Override
public void setR2935_targets_I_INS( I_INS inst ) {
R2935_targets_I_INS_inst = inst;
}
@Override
public I_INS R2935_targets_I_INS() throws XtumlException {
return R2935_targets_I_INS_inst;
}
private I_INS R2937_was_sent_from_I_INS_inst;
@Override
public void setR2937_was_sent_from_I_INS( I_INS inst ) {
R2937_was_sent_from_I_INS_inst = inst;
}
@Override
public I_INS R2937_was_sent_from_I_INS() throws XtumlException {
return R2937_was_sent_from_I_INS_inst;
}
private PendingEvent R2939_will_be_processed_after_PendingEvent_inst;
@Override
public void setR2939_will_be_processed_after_PendingEvent( PendingEvent inst ) {
R2939_will_be_processed_after_PendingEvent_inst = inst;
}
@Override
public PendingEvent R2939_will_be_processed_after_PendingEvent() throws XtumlException {
return R2939_will_be_processed_after_PendingEvent_inst;
}
private PendingEvent R2939_will_be_processed_before_PendingEvent_inst;
@Override
public void setR2939_will_be_processed_before_PendingEvent( PendingEvent inst ) {
R2939_will_be_processed_before_PendingEvent_inst = inst;
}
@Override
public PendingEvent R2939_will_be_processed_before_PendingEvent() throws XtumlException {
return R2939_will_be_processed_before_PendingEvent_inst;
}
private Timer R2940_delivered_by_Timer_inst;
@Override
public void setR2940_delivered_by_Timer( Timer inst ) {
R2940_delivered_by_Timer_inst = inst;
}
@Override
public Timer R2940_delivered_by_Timer() throws XtumlException {
return R2940_delivered_by_Timer_inst;
}
private EventQueueEntry R2944_EventQueueEntry_inst;
@Override
public void setR2944_EventQueueEntry( EventQueueEntry inst ) {
R2944_EventQueueEntry_inst = inst;
}
@Override
public EventQueueEntry R2944_EventQueueEntry() throws XtumlException {
return R2944_EventQueueEntry_inst;
}
private SelfQueueEntry R2946_SelfQueueEntry_inst;
@Override
public void setR2946_SelfQueueEntry( SelfQueueEntry inst ) {
R2946_SelfQueueEntry_inst = inst;
}
@Override
public SelfQueueEntry R2946_SelfQueueEntry() throws XtumlException {
return R2946_SelfQueueEntry_inst;
}
private ComponentInstance R2964_is_pending_in_ComponentInstance_inst;
@Override
public void setR2964_is_pending_in_ComponentInstance( ComponentInstance inst ) {
R2964_is_pending_in_ComponentInstance_inst = inst;
}
@Override
public ComponentInstance R2964_is_pending_in_ComponentInstance() throws XtumlException {
return R2964_is_pending_in_ComponentInstance_inst;
}
private ComponentInstance R2976_originates_from_ComponentInstance_inst;
@Override
public void setR2976_originates_from_ComponentInstance( ComponentInstance inst ) {
R2976_originates_from_ComponentInstance_inst = inst;
}
@Override
public ComponentInstance R2976_originates_from_ComponentInstance() throws XtumlException {
return R2976_originates_from_ComponentInstance_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public PendingEvent self() {
return this;
}
@Override
public PendingEvent oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_PENDINGEVENT;
}
}
class EmptyPendingEvent extends ModelInstance implements PendingEvent {
// attributes
public UniqueId getEvent_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setEvent_ID( UniqueId m_Event_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setIsExecuting( boolean m_isExecuting ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public boolean getIsExecuting() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public boolean getIsCreation() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setIsCreation( boolean m_isCreation ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getSMevt_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setSMevt_ID( UniqueId ref_SMevt_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setTarget_Inst_ID( UniqueId ref_Target_Inst_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getTarget_Inst_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setNextEvent_ID( UniqueId ref_nextEvent_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getNextEvent_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public UniqueId getSent_By_Inst_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setSent_By_Inst_ID( UniqueId ref_Sent_By_Inst_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setNext_self_Event_ID( UniqueId ref_next_self_Event_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getNext_self_Event_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public UniqueId getSent_By_CIE_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setSent_By_CIE_ID( UniqueId m_Sent_By_CIE_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getCIE_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setCIE_ID( UniqueId m_CIE_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getExecution_Engine_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setExecution_Engine_ID( UniqueId ref_Execution_Engine_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setOriginating_Execution_Engine_ID( UniqueId ref_Originating_Execution_Engine_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getOriginating_Execution_Engine_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setLabel( String m_Label ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getLabel() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
// operations
// selections
@Override
public StateMachineEvent R2906_is_instance_of_StateMachineEvent() {
return StateMachineEventImpl.EMPTY_STATEMACHINEEVENT;
}
@Override
public I_INS R2907_is_pending_for_I_INS() {
return I_INSImpl.EMPTY_I_INS;
}
@Override
public PendingEvent R2908_will_be_processed_after_PendingEvent() {
return PendingEventImpl.EMPTY_PENDINGEVENT;
}
@Override
public PendingEvent R2908_will_be_processed_before_PendingEvent() {
return PendingEventImpl.EMPTY_PENDINGEVENT;
}
@Override
public DataItemValueSet R2933_DataItemValue() {
return (new DataItemValueSetImpl());
}
@Override
public I_INS R2935_targets_I_INS() {
return I_INSImpl.EMPTY_I_INS;
}
@Override
public I_INS R2937_was_sent_from_I_INS() {
return I_INSImpl.EMPTY_I_INS;
}
@Override
public PendingEvent R2939_will_be_processed_after_PendingEvent() {
return PendingEventImpl.EMPTY_PENDINGEVENT;
}
@Override
public PendingEvent R2939_will_be_processed_before_PendingEvent() {
return PendingEventImpl.EMPTY_PENDINGEVENT;
}
@Override
public Timer R2940_delivered_by_Timer() {
return TimerImpl.EMPTY_TIMER;
}
@Override
public EventQueueEntry R2944_EventQueueEntry() {
return EventQueueEntryImpl.EMPTY_EVENTQUEUEENTRY;
}
@Override
public SelfQueueEntry R2946_SelfQueueEntry() {
return SelfQueueEntryImpl.EMPTY_SELFQUEUEENTRY;
}
@Override
public ComponentInstance R2964_is_pending_in_ComponentInstance() {
return ComponentInstanceImpl.EMPTY_COMPONENTINSTANCE;
}
@Override
public ComponentInstance R2976_originates_from_ComponentInstance() {
return ComponentInstanceImpl.EMPTY_COMPONENTINSTANCE;
}
@Override
public String getKeyLetters() {
return PendingEventImpl.KEY_LETTERS;
}
@Override
public PendingEvent self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public PendingEvent oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return PendingEventImpl.EMPTY_PENDINGEVENT;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy