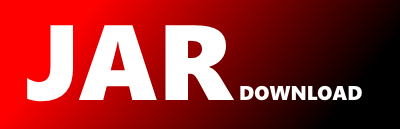
io.ciera.tool.sql.ooaofooa.instance.impl.SelfQueueEntryImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.instance.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.instance.ComponentInstance;
import io.ciera.tool.sql.ooaofooa.instance.PendingEvent;
import io.ciera.tool.sql.ooaofooa.instance.SelfQueueEntry;
import io.ciera.tool.sql.ooaofooa.instance.impl.ComponentInstanceImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.PendingEventImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.SelfQueueEntryImpl;
public class SelfQueueEntryImpl extends ModelInstance implements SelfQueueEntry {
public static final String KEY_LETTERS = "I_SQE";
public static final SelfQueueEntry EMPTY_SELFQUEUEENTRY = new EmptySelfQueueEntry();
private Sql context;
// constructors
private SelfQueueEntryImpl( Sql context ) {
this.context = context;
m_Self_Queue_Entry_ID = UniqueId.random();
ref_Execution_Engine_ID = UniqueId.random();
ref_Event_ID = UniqueId.random();
ref_Next_Self_Queue_Entry_ID = UniqueId.random();
R2946_ComponentInstance_inst = ComponentInstanceImpl.EMPTY_COMPONENTINSTANCE;
R2946_PendingEvent_inst = PendingEventImpl.EMPTY_PENDINGEVENT;
R2947_follows_SelfQueueEntry_inst = SelfQueueEntryImpl.EMPTY_SELFQUEUEENTRY;
R2947_precedes_SelfQueueEntry_inst = SelfQueueEntryImpl.EMPTY_SELFQUEUEENTRY;
}
private SelfQueueEntryImpl( Sql context, UniqueId instanceId, UniqueId m_Self_Queue_Entry_ID, UniqueId ref_Execution_Engine_ID, UniqueId ref_Event_ID, UniqueId ref_Next_Self_Queue_Entry_ID ) {
super(instanceId);
this.context = context;
this.m_Self_Queue_Entry_ID = m_Self_Queue_Entry_ID;
this.ref_Execution_Engine_ID = ref_Execution_Engine_ID;
this.ref_Event_ID = ref_Event_ID;
this.ref_Next_Self_Queue_Entry_ID = ref_Next_Self_Queue_Entry_ID;
R2946_ComponentInstance_inst = ComponentInstanceImpl.EMPTY_COMPONENTINSTANCE;
R2946_PendingEvent_inst = PendingEventImpl.EMPTY_PENDINGEVENT;
R2947_follows_SelfQueueEntry_inst = SelfQueueEntryImpl.EMPTY_SELFQUEUEENTRY;
R2947_precedes_SelfQueueEntry_inst = SelfQueueEntryImpl.EMPTY_SELFQUEUEENTRY;
}
public static SelfQueueEntry create( Sql context ) throws XtumlException {
SelfQueueEntry newSelfQueueEntry = new SelfQueueEntryImpl( context );
if ( context.addInstance( newSelfQueueEntry ) ) {
newSelfQueueEntry.getRunContext().addChange(new InstanceCreatedDelta(newSelfQueueEntry, KEY_LETTERS));
return newSelfQueueEntry;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static SelfQueueEntry create( Sql context, UniqueId m_Self_Queue_Entry_ID, UniqueId ref_Execution_Engine_ID, UniqueId ref_Event_ID, UniqueId ref_Next_Self_Queue_Entry_ID ) throws XtumlException {
return create(context, UniqueId.random(), m_Self_Queue_Entry_ID, ref_Execution_Engine_ID, ref_Event_ID, ref_Next_Self_Queue_Entry_ID);
}
public static SelfQueueEntry create( Sql context, UniqueId instanceId, UniqueId m_Self_Queue_Entry_ID, UniqueId ref_Execution_Engine_ID, UniqueId ref_Event_ID, UniqueId ref_Next_Self_Queue_Entry_ID ) throws XtumlException {
SelfQueueEntry newSelfQueueEntry = new SelfQueueEntryImpl( context, instanceId, m_Self_Queue_Entry_ID, ref_Execution_Engine_ID, ref_Event_ID, ref_Next_Self_Queue_Entry_ID );
if ( context.addInstance( newSelfQueueEntry ) ) {
return newSelfQueueEntry;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId m_Self_Queue_Entry_ID;
@Override
public UniqueId getSelf_Queue_Entry_ID() throws XtumlException {
checkLiving();
return m_Self_Queue_Entry_ID;
}
@Override
public void setSelf_Queue_Entry_ID(UniqueId m_Self_Queue_Entry_ID) throws XtumlException {
checkLiving();
if (m_Self_Queue_Entry_ID.inequality( this.m_Self_Queue_Entry_ID)) {
final UniqueId oldValue = this.m_Self_Queue_Entry_ID;
this.m_Self_Queue_Entry_ID = m_Self_Queue_Entry_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Self_Queue_Entry_ID", oldValue, this.m_Self_Queue_Entry_ID));
if ( !R2947_precedes_SelfQueueEntry().isEmpty() ) R2947_precedes_SelfQueueEntry().setNext_Self_Queue_Entry_ID( m_Self_Queue_Entry_ID );
}
}
private UniqueId ref_Execution_Engine_ID;
@Override
public void setExecution_Engine_ID(UniqueId ref_Execution_Engine_ID) throws XtumlException {
checkLiving();
if (ref_Execution_Engine_ID.inequality( this.ref_Execution_Engine_ID)) {
final UniqueId oldValue = this.ref_Execution_Engine_ID;
this.ref_Execution_Engine_ID = ref_Execution_Engine_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Execution_Engine_ID", oldValue, this.ref_Execution_Engine_ID));
}
}
@Override
public UniqueId getExecution_Engine_ID() throws XtumlException {
checkLiving();
return ref_Execution_Engine_ID;
}
private UniqueId ref_Event_ID;
@Override
public void setEvent_ID(UniqueId ref_Event_ID) throws XtumlException {
checkLiving();
if (ref_Event_ID.inequality( this.ref_Event_ID)) {
final UniqueId oldValue = this.ref_Event_ID;
this.ref_Event_ID = ref_Event_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Event_ID", oldValue, this.ref_Event_ID));
}
}
@Override
public UniqueId getEvent_ID() throws XtumlException {
checkLiving();
return ref_Event_ID;
}
private UniqueId ref_Next_Self_Queue_Entry_ID;
@Override
public UniqueId getNext_Self_Queue_Entry_ID() throws XtumlException {
checkLiving();
return ref_Next_Self_Queue_Entry_ID;
}
@Override
public void setNext_Self_Queue_Entry_ID(UniqueId ref_Next_Self_Queue_Entry_ID) throws XtumlException {
checkLiving();
if (ref_Next_Self_Queue_Entry_ID.inequality( this.ref_Next_Self_Queue_Entry_ID)) {
final UniqueId oldValue = this.ref_Next_Self_Queue_Entry_ID;
this.ref_Next_Self_Queue_Entry_ID = ref_Next_Self_Queue_Entry_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Next_Self_Queue_Entry_ID", oldValue, this.ref_Next_Self_Queue_Entry_ID));
}
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getSelf_Queue_Entry_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private ComponentInstance R2946_ComponentInstance_inst;
@Override
public void setR2946_ComponentInstance( ComponentInstance inst ) {
R2946_ComponentInstance_inst = inst;
}
@Override
public ComponentInstance R2946_ComponentInstance() throws XtumlException {
return R2946_ComponentInstance_inst;
}
private PendingEvent R2946_PendingEvent_inst;
@Override
public void setR2946_PendingEvent( PendingEvent inst ) {
R2946_PendingEvent_inst = inst;
}
@Override
public PendingEvent R2946_PendingEvent() throws XtumlException {
return R2946_PendingEvent_inst;
}
private SelfQueueEntry R2947_follows_SelfQueueEntry_inst;
@Override
public void setR2947_follows_SelfQueueEntry( SelfQueueEntry inst ) {
R2947_follows_SelfQueueEntry_inst = inst;
}
@Override
public SelfQueueEntry R2947_follows_SelfQueueEntry() throws XtumlException {
return R2947_follows_SelfQueueEntry_inst;
}
private SelfQueueEntry R2947_precedes_SelfQueueEntry_inst;
@Override
public void setR2947_precedes_SelfQueueEntry( SelfQueueEntry inst ) {
R2947_precedes_SelfQueueEntry_inst = inst;
}
@Override
public SelfQueueEntry R2947_precedes_SelfQueueEntry() throws XtumlException {
return R2947_precedes_SelfQueueEntry_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public SelfQueueEntry self() {
return this;
}
@Override
public SelfQueueEntry oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_SELFQUEUEENTRY;
}
}
class EmptySelfQueueEntry extends ModelInstance implements SelfQueueEntry {
// attributes
public UniqueId getSelf_Queue_Entry_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setSelf_Queue_Entry_ID( UniqueId m_Self_Queue_Entry_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setExecution_Engine_ID( UniqueId ref_Execution_Engine_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getExecution_Engine_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setEvent_ID( UniqueId ref_Event_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getEvent_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public UniqueId getNext_Self_Queue_Entry_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setNext_Self_Queue_Entry_ID( UniqueId ref_Next_Self_Queue_Entry_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
// operations
// selections
@Override
public ComponentInstance R2946_ComponentInstance() {
return ComponentInstanceImpl.EMPTY_COMPONENTINSTANCE;
}
@Override
public PendingEvent R2946_PendingEvent() {
return PendingEventImpl.EMPTY_PENDINGEVENT;
}
@Override
public SelfQueueEntry R2947_follows_SelfQueueEntry() {
return SelfQueueEntryImpl.EMPTY_SELFQUEUEENTRY;
}
@Override
public SelfQueueEntry R2947_precedes_SelfQueueEntry() {
return SelfQueueEntryImpl.EMPTY_SELFQUEUEENTRY;
}
@Override
public String getKeyLetters() {
return SelfQueueEntryImpl.KEY_LETTERS;
}
@Override
public SelfQueueEntry self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public SelfQueueEntry oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return SelfQueueEntryImpl.EMPTY_SELFQUEUEENTRY;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy