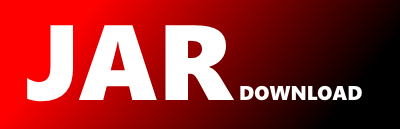
io.ciera.tool.sql.ooaofooa.instance.impl.StackFrameImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.instance.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.instance.BlockInStackFrame;
import io.ciera.tool.sql.ooaofooa.instance.BlockInStackFrameSet;
import io.ciera.tool.sql.ooaofooa.instance.I_INS;
import io.ciera.tool.sql.ooaofooa.instance.IntercomponentQueueEntry;
import io.ciera.tool.sql.ooaofooa.instance.Stack;
import io.ciera.tool.sql.ooaofooa.instance.StackFrame;
import io.ciera.tool.sql.ooaofooa.instance.ValueInStackFrame;
import io.ciera.tool.sql.ooaofooa.instance.ValueInStackFrameSet;
import io.ciera.tool.sql.ooaofooa.instance.impl.BlockInStackFrameSetImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.I_INSImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.IntercomponentQueueEntryImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.StackFrameImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.StackImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.ValueInStackFrameSetImpl;
public class StackFrameImpl extends ModelInstance implements StackFrame {
public static final String KEY_LETTERS = "I_STF";
public static final StackFrame EMPTY_STACKFRAME = new EmptyStackFrame();
private Sql context;
// constructors
private StackFrameImpl( Sql context ) {
this.context = context;
m_Stack_Frame_ID = UniqueId.random();
m_Created_For_Wired_Bridge = false;
m_readyForInterrupt = false;
m_Bridge_Caller_Stack_Frame_ID = UniqueId.random();
ref_Child_Stack_Frame_ID = UniqueId.random();
ref_Top_Stack_Frame_Stack_ID = UniqueId.random();
ref_Stack_ID = UniqueId.random();
ref_Inst_ID = UniqueId.random();
ref_Value_Q_Stack_ID = UniqueId.random();
ref_Blocking_Stack_Frame_ID = UniqueId.random();
R2923_supplies_context_for_BlockInStackFrame_set = new BlockInStackFrameSetImpl();
R2928_next_context_StackFrame_inst = StackFrameImpl.EMPTY_STACKFRAME;
R2928_previous_context_StackFrame_inst = StackFrameImpl.EMPTY_STACKFRAME;
R2929_is_processed_by_Stack_inst = StackImpl.EMPTY_STACK;
R2943_processed_by_Stack_inst = StackImpl.EMPTY_STACK;
R2951_ValueInStackFrame_set = new ValueInStackFrameSetImpl();
R2954_has_context_I_INS_inst = I_INSImpl.EMPTY_I_INS;
R2965_blocked_by_StackFrame_inst = StackFrameImpl.EMPTY_STACKFRAME;
R2965_blocks_StackFrame_inst = StackFrameImpl.EMPTY_STACKFRAME;
R2966_is_enqueued_with_IntercomponentQueueEntry_inst = IntercomponentQueueEntryImpl.EMPTY_INTERCOMPONENTQUEUEENTRY;
R2967_holds_return_value_for_Stack_inst = StackImpl.EMPTY_STACK;
}
private StackFrameImpl( Sql context, UniqueId instanceId, UniqueId m_Stack_Frame_ID, boolean m_Created_For_Wired_Bridge, boolean m_readyForInterrupt, UniqueId m_Bridge_Caller_Stack_Frame_ID, UniqueId ref_Child_Stack_Frame_ID, UniqueId ref_Top_Stack_Frame_Stack_ID, UniqueId ref_Stack_ID, UniqueId ref_Inst_ID, UniqueId ref_Value_Q_Stack_ID, UniqueId ref_Blocking_Stack_Frame_ID ) {
super(instanceId);
this.context = context;
this.m_Stack_Frame_ID = m_Stack_Frame_ID;
this.m_Created_For_Wired_Bridge = m_Created_For_Wired_Bridge;
this.m_readyForInterrupt = m_readyForInterrupt;
this.m_Bridge_Caller_Stack_Frame_ID = m_Bridge_Caller_Stack_Frame_ID;
this.ref_Child_Stack_Frame_ID = ref_Child_Stack_Frame_ID;
this.ref_Top_Stack_Frame_Stack_ID = ref_Top_Stack_Frame_Stack_ID;
this.ref_Stack_ID = ref_Stack_ID;
this.ref_Inst_ID = ref_Inst_ID;
this.ref_Value_Q_Stack_ID = ref_Value_Q_Stack_ID;
this.ref_Blocking_Stack_Frame_ID = ref_Blocking_Stack_Frame_ID;
R2923_supplies_context_for_BlockInStackFrame_set = new BlockInStackFrameSetImpl();
R2928_next_context_StackFrame_inst = StackFrameImpl.EMPTY_STACKFRAME;
R2928_previous_context_StackFrame_inst = StackFrameImpl.EMPTY_STACKFRAME;
R2929_is_processed_by_Stack_inst = StackImpl.EMPTY_STACK;
R2943_processed_by_Stack_inst = StackImpl.EMPTY_STACK;
R2951_ValueInStackFrame_set = new ValueInStackFrameSetImpl();
R2954_has_context_I_INS_inst = I_INSImpl.EMPTY_I_INS;
R2965_blocked_by_StackFrame_inst = StackFrameImpl.EMPTY_STACKFRAME;
R2965_blocks_StackFrame_inst = StackFrameImpl.EMPTY_STACKFRAME;
R2966_is_enqueued_with_IntercomponentQueueEntry_inst = IntercomponentQueueEntryImpl.EMPTY_INTERCOMPONENTQUEUEENTRY;
R2967_holds_return_value_for_Stack_inst = StackImpl.EMPTY_STACK;
}
public static StackFrame create( Sql context ) throws XtumlException {
StackFrame newStackFrame = new StackFrameImpl( context );
if ( context.addInstance( newStackFrame ) ) {
newStackFrame.getRunContext().addChange(new InstanceCreatedDelta(newStackFrame, KEY_LETTERS));
return newStackFrame;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static StackFrame create( Sql context, UniqueId m_Stack_Frame_ID, boolean m_Created_For_Wired_Bridge, boolean m_readyForInterrupt, UniqueId m_Bridge_Caller_Stack_Frame_ID, UniqueId ref_Child_Stack_Frame_ID, UniqueId ref_Top_Stack_Frame_Stack_ID, UniqueId ref_Stack_ID, UniqueId ref_Inst_ID, UniqueId ref_Value_Q_Stack_ID, UniqueId ref_Blocking_Stack_Frame_ID ) throws XtumlException {
return create(context, UniqueId.random(), m_Stack_Frame_ID, m_Created_For_Wired_Bridge, m_readyForInterrupt, m_Bridge_Caller_Stack_Frame_ID, ref_Child_Stack_Frame_ID, ref_Top_Stack_Frame_Stack_ID, ref_Stack_ID, ref_Inst_ID, ref_Value_Q_Stack_ID, ref_Blocking_Stack_Frame_ID);
}
public static StackFrame create( Sql context, UniqueId instanceId, UniqueId m_Stack_Frame_ID, boolean m_Created_For_Wired_Bridge, boolean m_readyForInterrupt, UniqueId m_Bridge_Caller_Stack_Frame_ID, UniqueId ref_Child_Stack_Frame_ID, UniqueId ref_Top_Stack_Frame_Stack_ID, UniqueId ref_Stack_ID, UniqueId ref_Inst_ID, UniqueId ref_Value_Q_Stack_ID, UniqueId ref_Blocking_Stack_Frame_ID ) throws XtumlException {
StackFrame newStackFrame = new StackFrameImpl( context, instanceId, m_Stack_Frame_ID, m_Created_For_Wired_Bridge, m_readyForInterrupt, m_Bridge_Caller_Stack_Frame_ID, ref_Child_Stack_Frame_ID, ref_Top_Stack_Frame_Stack_ID, ref_Stack_ID, ref_Inst_ID, ref_Value_Q_Stack_ID, ref_Blocking_Stack_Frame_ID );
if ( context.addInstance( newStackFrame ) ) {
return newStackFrame;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId m_Stack_Frame_ID;
@Override
public UniqueId getStack_Frame_ID() throws XtumlException {
checkLiving();
return m_Stack_Frame_ID;
}
@Override
public void setStack_Frame_ID(UniqueId m_Stack_Frame_ID) throws XtumlException {
checkLiving();
if (m_Stack_Frame_ID.inequality( this.m_Stack_Frame_ID)) {
final UniqueId oldValue = this.m_Stack_Frame_ID;
this.m_Stack_Frame_ID = m_Stack_Frame_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Stack_Frame_ID", oldValue, this.m_Stack_Frame_ID));
if ( !R2928_previous_context_StackFrame().isEmpty() ) R2928_previous_context_StackFrame().setChild_Stack_Frame_ID( m_Stack_Frame_ID );
if ( !R2965_blocks_StackFrame().isEmpty() ) R2965_blocks_StackFrame().setBlocking_Stack_Frame_ID( m_Stack_Frame_ID );
if ( !R2951_ValueInStackFrame().isEmpty() ) R2951_ValueInStackFrame().setStack_Frame_ID( m_Stack_Frame_ID );
if ( !R2923_supplies_context_for_BlockInStackFrame().isEmpty() ) R2923_supplies_context_for_BlockInStackFrame().setStack_Frame_ID( m_Stack_Frame_ID );
if ( !R2966_is_enqueued_with_IntercomponentQueueEntry().isEmpty() ) R2966_is_enqueued_with_IntercomponentQueueEntry().setStack_Frame_ID( m_Stack_Frame_ID );
}
}
private boolean m_Created_For_Wired_Bridge;
@Override
public void setCreated_For_Wired_Bridge(boolean m_Created_For_Wired_Bridge) throws XtumlException {
checkLiving();
if (m_Created_For_Wired_Bridge != this.m_Created_For_Wired_Bridge) {
final boolean oldValue = this.m_Created_For_Wired_Bridge;
this.m_Created_For_Wired_Bridge = m_Created_For_Wired_Bridge;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Created_For_Wired_Bridge", oldValue, this.m_Created_For_Wired_Bridge));
}
}
@Override
public boolean getCreated_For_Wired_Bridge() throws XtumlException {
checkLiving();
return m_Created_For_Wired_Bridge;
}
private boolean m_readyForInterrupt;
@Override
public void setReadyForInterrupt(boolean m_readyForInterrupt) throws XtumlException {
checkLiving();
if (m_readyForInterrupt != this.m_readyForInterrupt) {
final boolean oldValue = this.m_readyForInterrupt;
this.m_readyForInterrupt = m_readyForInterrupt;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_readyForInterrupt", oldValue, this.m_readyForInterrupt));
}
}
@Override
public boolean getReadyForInterrupt() throws XtumlException {
checkLiving();
return m_readyForInterrupt;
}
private UniqueId m_Bridge_Caller_Stack_Frame_ID;
@Override
public UniqueId getBridge_Caller_Stack_Frame_ID() throws XtumlException {
checkLiving();
return m_Bridge_Caller_Stack_Frame_ID;
}
@Override
public void setBridge_Caller_Stack_Frame_ID(UniqueId m_Bridge_Caller_Stack_Frame_ID) throws XtumlException {
checkLiving();
if (m_Bridge_Caller_Stack_Frame_ID.inequality( this.m_Bridge_Caller_Stack_Frame_ID)) {
final UniqueId oldValue = this.m_Bridge_Caller_Stack_Frame_ID;
this.m_Bridge_Caller_Stack_Frame_ID = m_Bridge_Caller_Stack_Frame_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Bridge_Caller_Stack_Frame_ID", oldValue, this.m_Bridge_Caller_Stack_Frame_ID));
}
}
private UniqueId ref_Child_Stack_Frame_ID;
@Override
public void setChild_Stack_Frame_ID(UniqueId ref_Child_Stack_Frame_ID) throws XtumlException {
checkLiving();
if (ref_Child_Stack_Frame_ID.inequality( this.ref_Child_Stack_Frame_ID)) {
final UniqueId oldValue = this.ref_Child_Stack_Frame_ID;
this.ref_Child_Stack_Frame_ID = ref_Child_Stack_Frame_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Child_Stack_Frame_ID", oldValue, this.ref_Child_Stack_Frame_ID));
}
}
@Override
public UniqueId getChild_Stack_Frame_ID() throws XtumlException {
checkLiving();
return ref_Child_Stack_Frame_ID;
}
private UniqueId ref_Top_Stack_Frame_Stack_ID;
@Override
public UniqueId getTop_Stack_Frame_Stack_ID() throws XtumlException {
checkLiving();
return ref_Top_Stack_Frame_Stack_ID;
}
@Override
public void setTop_Stack_Frame_Stack_ID(UniqueId ref_Top_Stack_Frame_Stack_ID) throws XtumlException {
checkLiving();
if (ref_Top_Stack_Frame_Stack_ID.inequality( this.ref_Top_Stack_Frame_Stack_ID)) {
final UniqueId oldValue = this.ref_Top_Stack_Frame_Stack_ID;
this.ref_Top_Stack_Frame_Stack_ID = ref_Top_Stack_Frame_Stack_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Top_Stack_Frame_Stack_ID", oldValue, this.ref_Top_Stack_Frame_Stack_ID));
}
}
private UniqueId ref_Stack_ID;
@Override
public void setStack_ID(UniqueId ref_Stack_ID) throws XtumlException {
checkLiving();
if (ref_Stack_ID.inequality( this.ref_Stack_ID)) {
final UniqueId oldValue = this.ref_Stack_ID;
this.ref_Stack_ID = ref_Stack_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Stack_ID", oldValue, this.ref_Stack_ID));
}
}
@Override
public UniqueId getStack_ID() throws XtumlException {
checkLiving();
return ref_Stack_ID;
}
private UniqueId ref_Inst_ID;
@Override
public UniqueId getInst_ID() throws XtumlException {
checkLiving();
return ref_Inst_ID;
}
@Override
public void setInst_ID(UniqueId ref_Inst_ID) throws XtumlException {
checkLiving();
if (ref_Inst_ID.inequality( this.ref_Inst_ID)) {
final UniqueId oldValue = this.ref_Inst_ID;
this.ref_Inst_ID = ref_Inst_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Inst_ID", oldValue, this.ref_Inst_ID));
}
}
private UniqueId ref_Value_Q_Stack_ID;
@Override
public UniqueId getValue_Q_Stack_ID() throws XtumlException {
checkLiving();
return ref_Value_Q_Stack_ID;
}
@Override
public void setValue_Q_Stack_ID(UniqueId ref_Value_Q_Stack_ID) throws XtumlException {
checkLiving();
if (ref_Value_Q_Stack_ID.inequality( this.ref_Value_Q_Stack_ID)) {
final UniqueId oldValue = this.ref_Value_Q_Stack_ID;
this.ref_Value_Q_Stack_ID = ref_Value_Q_Stack_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Value_Q_Stack_ID", oldValue, this.ref_Value_Q_Stack_ID));
}
}
private UniqueId ref_Blocking_Stack_Frame_ID;
@Override
public UniqueId getBlocking_Stack_Frame_ID() throws XtumlException {
checkLiving();
return ref_Blocking_Stack_Frame_ID;
}
@Override
public void setBlocking_Stack_Frame_ID(UniqueId ref_Blocking_Stack_Frame_ID) throws XtumlException {
checkLiving();
if (ref_Blocking_Stack_Frame_ID.inequality( this.ref_Blocking_Stack_Frame_ID)) {
final UniqueId oldValue = this.ref_Blocking_Stack_Frame_ID;
this.ref_Blocking_Stack_Frame_ID = ref_Blocking_Stack_Frame_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Blocking_Stack_Frame_ID", oldValue, this.ref_Blocking_Stack_Frame_ID));
}
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getStack_Frame_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private BlockInStackFrameSet R2923_supplies_context_for_BlockInStackFrame_set;
@Override
public void addR2923_supplies_context_for_BlockInStackFrame( BlockInStackFrame inst ) {
R2923_supplies_context_for_BlockInStackFrame_set.add(inst);
}
@Override
public void removeR2923_supplies_context_for_BlockInStackFrame( BlockInStackFrame inst ) {
R2923_supplies_context_for_BlockInStackFrame_set.remove(inst);
}
@Override
public BlockInStackFrameSet R2923_supplies_context_for_BlockInStackFrame() throws XtumlException {
return R2923_supplies_context_for_BlockInStackFrame_set;
}
private StackFrame R2928_next_context_StackFrame_inst;
@Override
public void setR2928_next_context_StackFrame( StackFrame inst ) {
R2928_next_context_StackFrame_inst = inst;
}
@Override
public StackFrame R2928_next_context_StackFrame() throws XtumlException {
return R2928_next_context_StackFrame_inst;
}
private StackFrame R2928_previous_context_StackFrame_inst;
@Override
public void setR2928_previous_context_StackFrame( StackFrame inst ) {
R2928_previous_context_StackFrame_inst = inst;
}
@Override
public StackFrame R2928_previous_context_StackFrame() throws XtumlException {
return R2928_previous_context_StackFrame_inst;
}
private Stack R2929_is_processed_by_Stack_inst;
@Override
public void setR2929_is_processed_by_Stack( Stack inst ) {
R2929_is_processed_by_Stack_inst = inst;
}
@Override
public Stack R2929_is_processed_by_Stack() throws XtumlException {
return R2929_is_processed_by_Stack_inst;
}
private Stack R2943_processed_by_Stack_inst;
@Override
public void setR2943_processed_by_Stack( Stack inst ) {
R2943_processed_by_Stack_inst = inst;
}
@Override
public Stack R2943_processed_by_Stack() throws XtumlException {
return R2943_processed_by_Stack_inst;
}
private ValueInStackFrameSet R2951_ValueInStackFrame_set;
@Override
public void addR2951_ValueInStackFrame( ValueInStackFrame inst ) {
R2951_ValueInStackFrame_set.add(inst);
}
@Override
public void removeR2951_ValueInStackFrame( ValueInStackFrame inst ) {
R2951_ValueInStackFrame_set.remove(inst);
}
@Override
public ValueInStackFrameSet R2951_ValueInStackFrame() throws XtumlException {
return R2951_ValueInStackFrame_set;
}
private I_INS R2954_has_context_I_INS_inst;
@Override
public void setR2954_has_context_I_INS( I_INS inst ) {
R2954_has_context_I_INS_inst = inst;
}
@Override
public I_INS R2954_has_context_I_INS() throws XtumlException {
return R2954_has_context_I_INS_inst;
}
private StackFrame R2965_blocked_by_StackFrame_inst;
@Override
public void setR2965_blocked_by_StackFrame( StackFrame inst ) {
R2965_blocked_by_StackFrame_inst = inst;
}
@Override
public StackFrame R2965_blocked_by_StackFrame() throws XtumlException {
return R2965_blocked_by_StackFrame_inst;
}
private StackFrame R2965_blocks_StackFrame_inst;
@Override
public void setR2965_blocks_StackFrame( StackFrame inst ) {
R2965_blocks_StackFrame_inst = inst;
}
@Override
public StackFrame R2965_blocks_StackFrame() throws XtumlException {
return R2965_blocks_StackFrame_inst;
}
private IntercomponentQueueEntry R2966_is_enqueued_with_IntercomponentQueueEntry_inst;
@Override
public void setR2966_is_enqueued_with_IntercomponentQueueEntry( IntercomponentQueueEntry inst ) {
R2966_is_enqueued_with_IntercomponentQueueEntry_inst = inst;
}
@Override
public IntercomponentQueueEntry R2966_is_enqueued_with_IntercomponentQueueEntry() throws XtumlException {
return R2966_is_enqueued_with_IntercomponentQueueEntry_inst;
}
private Stack R2967_holds_return_value_for_Stack_inst;
@Override
public void setR2967_holds_return_value_for_Stack( Stack inst ) {
R2967_holds_return_value_for_Stack_inst = inst;
}
@Override
public Stack R2967_holds_return_value_for_Stack() throws XtumlException {
return R2967_holds_return_value_for_Stack_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public StackFrame self() {
return this;
}
@Override
public StackFrame oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_STACKFRAME;
}
}
class EmptyStackFrame extends ModelInstance implements StackFrame {
// attributes
public UniqueId getStack_Frame_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setStack_Frame_ID( UniqueId m_Stack_Frame_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setCreated_For_Wired_Bridge( boolean m_Created_For_Wired_Bridge ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public boolean getCreated_For_Wired_Bridge() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setReadyForInterrupt( boolean m_readyForInterrupt ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public boolean getReadyForInterrupt() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public UniqueId getBridge_Caller_Stack_Frame_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setBridge_Caller_Stack_Frame_ID( UniqueId m_Bridge_Caller_Stack_Frame_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setChild_Stack_Frame_ID( UniqueId ref_Child_Stack_Frame_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getChild_Stack_Frame_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public UniqueId getTop_Stack_Frame_Stack_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setTop_Stack_Frame_Stack_ID( UniqueId ref_Top_Stack_Frame_Stack_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setStack_ID( UniqueId ref_Stack_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getStack_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public UniqueId getInst_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setInst_ID( UniqueId ref_Inst_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getValue_Q_Stack_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setValue_Q_Stack_ID( UniqueId ref_Value_Q_Stack_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getBlocking_Stack_Frame_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setBlocking_Stack_Frame_ID( UniqueId ref_Blocking_Stack_Frame_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
// operations
// selections
@Override
public BlockInStackFrameSet R2923_supplies_context_for_BlockInStackFrame() {
return (new BlockInStackFrameSetImpl());
}
@Override
public StackFrame R2928_next_context_StackFrame() {
return StackFrameImpl.EMPTY_STACKFRAME;
}
@Override
public StackFrame R2928_previous_context_StackFrame() {
return StackFrameImpl.EMPTY_STACKFRAME;
}
@Override
public Stack R2929_is_processed_by_Stack() {
return StackImpl.EMPTY_STACK;
}
@Override
public Stack R2943_processed_by_Stack() {
return StackImpl.EMPTY_STACK;
}
@Override
public ValueInStackFrameSet R2951_ValueInStackFrame() {
return (new ValueInStackFrameSetImpl());
}
@Override
public I_INS R2954_has_context_I_INS() {
return I_INSImpl.EMPTY_I_INS;
}
@Override
public StackFrame R2965_blocked_by_StackFrame() {
return StackFrameImpl.EMPTY_STACKFRAME;
}
@Override
public StackFrame R2965_blocks_StackFrame() {
return StackFrameImpl.EMPTY_STACKFRAME;
}
@Override
public IntercomponentQueueEntry R2966_is_enqueued_with_IntercomponentQueueEntry() {
return IntercomponentQueueEntryImpl.EMPTY_INTERCOMPONENTQUEUEENTRY;
}
@Override
public Stack R2967_holds_return_value_for_Stack() {
return StackImpl.EMPTY_STACK;
}
@Override
public String getKeyLetters() {
return StackFrameImpl.KEY_LETTERS;
}
@Override
public StackFrame self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public StackFrame oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return StackFrameImpl.EMPTY_STACKFRAME;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy