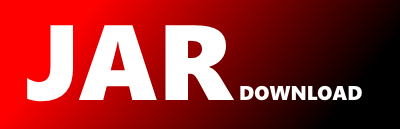
io.ciera.tool.sql.ooaofooa.instance.impl.StackFrameSetImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.instance.impl;
import io.ciera.runtime.summit.classes.InstanceSet;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.sql.ooaofooa.instance.BlockInStackFrameSet;
import io.ciera.tool.sql.ooaofooa.instance.I_INSSet;
import io.ciera.tool.sql.ooaofooa.instance.IntercomponentQueueEntrySet;
import io.ciera.tool.sql.ooaofooa.instance.StackFrame;
import io.ciera.tool.sql.ooaofooa.instance.StackFrameSet;
import io.ciera.tool.sql.ooaofooa.instance.StackSet;
import io.ciera.tool.sql.ooaofooa.instance.ValueInStackFrameSet;
import io.ciera.tool.sql.ooaofooa.instance.impl.BlockInStackFrameSetImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.I_INSSetImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.IntercomponentQueueEntrySetImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.StackFrameSetImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.StackSetImpl;
import io.ciera.tool.sql.ooaofooa.instance.impl.ValueInStackFrameSetImpl;
import java.util.Arrays;
import java.util.Comparator;
import java.util.List;
public class StackFrameSetImpl extends InstanceSet implements StackFrameSet {
public StackFrameSetImpl() {
}
public StackFrameSetImpl(Comparator super StackFrame> comp) {
super(comp);
}
// attributes
@Override
public void setChild_Stack_Frame_ID( UniqueId ref_Child_Stack_Frame_ID ) throws XtumlException {
for ( StackFrame stackframe : this ) stackframe.setChild_Stack_Frame_ID( ref_Child_Stack_Frame_ID );
}
@Override
public void setStack_ID( UniqueId ref_Stack_ID ) throws XtumlException {
for ( StackFrame stackframe : this ) stackframe.setStack_ID( ref_Stack_ID );
}
@Override
public void setBlocking_Stack_Frame_ID( UniqueId ref_Blocking_Stack_Frame_ID ) throws XtumlException {
for ( StackFrame stackframe : this ) stackframe.setBlocking_Stack_Frame_ID( ref_Blocking_Stack_Frame_ID );
}
@Override
public void setStack_Frame_ID( UniqueId m_Stack_Frame_ID ) throws XtumlException {
for ( StackFrame stackframe : this ) stackframe.setStack_Frame_ID( m_Stack_Frame_ID );
}
@Override
public void setReadyForInterrupt( boolean m_readyForInterrupt ) throws XtumlException {
for ( StackFrame stackframe : this ) stackframe.setReadyForInterrupt( m_readyForInterrupt );
}
@Override
public void setCreated_For_Wired_Bridge( boolean m_Created_For_Wired_Bridge ) throws XtumlException {
for ( StackFrame stackframe : this ) stackframe.setCreated_For_Wired_Bridge( m_Created_For_Wired_Bridge );
}
@Override
public void setInst_ID( UniqueId ref_Inst_ID ) throws XtumlException {
for ( StackFrame stackframe : this ) stackframe.setInst_ID( ref_Inst_ID );
}
@Override
public void setBridge_Caller_Stack_Frame_ID( UniqueId m_Bridge_Caller_Stack_Frame_ID ) throws XtumlException {
for ( StackFrame stackframe : this ) stackframe.setBridge_Caller_Stack_Frame_ID( m_Bridge_Caller_Stack_Frame_ID );
}
@Override
public void setValue_Q_Stack_ID( UniqueId ref_Value_Q_Stack_ID ) throws XtumlException {
for ( StackFrame stackframe : this ) stackframe.setValue_Q_Stack_ID( ref_Value_Q_Stack_ID );
}
@Override
public void setTop_Stack_Frame_Stack_ID( UniqueId ref_Top_Stack_Frame_Stack_ID ) throws XtumlException {
for ( StackFrame stackframe : this ) stackframe.setTop_Stack_Frame_Stack_ID( ref_Top_Stack_Frame_Stack_ID );
}
// selections
@Override
public BlockInStackFrameSet R2923_supplies_context_for_BlockInStackFrame() throws XtumlException {
BlockInStackFrameSet blockinstackframeset = new BlockInStackFrameSetImpl();
for ( StackFrame stackframe : this ) blockinstackframeset.addAll( stackframe.R2923_supplies_context_for_BlockInStackFrame() );
return blockinstackframeset;
}
@Override
public StackFrameSet R2928_next_context_StackFrame() throws XtumlException {
StackFrameSet stackframeset = new StackFrameSetImpl();
for ( StackFrame stackframe : this ) stackframeset.add( stackframe.R2928_next_context_StackFrame() );
return stackframeset;
}
@Override
public StackFrameSet R2928_previous_context_StackFrame() throws XtumlException {
StackFrameSet stackframeset = new StackFrameSetImpl();
for ( StackFrame stackframe : this ) stackframeset.add( stackframe.R2928_previous_context_StackFrame() );
return stackframeset;
}
@Override
public StackSet R2929_is_processed_by_Stack() throws XtumlException {
StackSet stackset = new StackSetImpl();
for ( StackFrame stackframe : this ) stackset.add( stackframe.R2929_is_processed_by_Stack() );
return stackset;
}
@Override
public StackSet R2943_processed_by_Stack() throws XtumlException {
StackSet stackset = new StackSetImpl();
for ( StackFrame stackframe : this ) stackset.add( stackframe.R2943_processed_by_Stack() );
return stackset;
}
@Override
public ValueInStackFrameSet R2951_ValueInStackFrame() throws XtumlException {
ValueInStackFrameSet valueinstackframeset = new ValueInStackFrameSetImpl();
for ( StackFrame stackframe : this ) valueinstackframeset.addAll( stackframe.R2951_ValueInStackFrame() );
return valueinstackframeset;
}
@Override
public I_INSSet R2954_has_context_I_INS() throws XtumlException {
I_INSSet i_insset = new I_INSSetImpl();
for ( StackFrame stackframe : this ) i_insset.add( stackframe.R2954_has_context_I_INS() );
return i_insset;
}
@Override
public StackFrameSet R2965_blocked_by_StackFrame() throws XtumlException {
StackFrameSet stackframeset = new StackFrameSetImpl();
for ( StackFrame stackframe : this ) stackframeset.add( stackframe.R2965_blocked_by_StackFrame() );
return stackframeset;
}
@Override
public StackFrameSet R2965_blocks_StackFrame() throws XtumlException {
StackFrameSet stackframeset = new StackFrameSetImpl();
for ( StackFrame stackframe : this ) stackframeset.add( stackframe.R2965_blocks_StackFrame() );
return stackframeset;
}
@Override
public IntercomponentQueueEntrySet R2966_is_enqueued_with_IntercomponentQueueEntry() throws XtumlException {
IntercomponentQueueEntrySet intercomponentqueueentryset = new IntercomponentQueueEntrySetImpl();
for ( StackFrame stackframe : this ) intercomponentqueueentryset.add( stackframe.R2966_is_enqueued_with_IntercomponentQueueEntry() );
return intercomponentqueueentryset;
}
@Override
public StackSet R2967_holds_return_value_for_Stack() throws XtumlException {
StackSet stackset = new StackSetImpl();
for ( StackFrame stackframe : this ) stackset.add( stackframe.R2967_holds_return_value_for_Stack() );
return stackset;
}
@Override
public StackFrame nullElement() {
return StackFrameImpl.EMPTY_STACKFRAME;
}
@Override
public StackFrameSet emptySet() {
return new StackFrameSetImpl();
}
@Override
public StackFrameSet emptySet(Comparator super StackFrame> comp) {
return new StackFrameSetImpl(comp);
}
@Override
public List elements() {
return Arrays.asList(toArray(new StackFrame[0]));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy