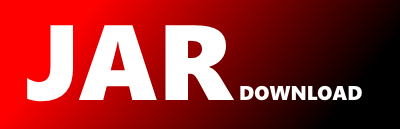
io.ciera.tool.sql.ooaofooa.instance.impl.TimerImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.instance.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.instance.PendingEvent;
import io.ciera.tool.sql.ooaofooa.instance.Timer;
import io.ciera.tool.sql.ooaofooa.instance.impl.PendingEventImpl;
import ooaofooa.datatypes.Long;
public class TimerImpl extends ModelInstance implements Timer {
public static final String KEY_LETTERS = "I_TIM";
public static final Timer EMPTY_TIMER = new EmptyTimer();
private Sql context;
// constructors
private TimerImpl( Sql context ) {
this.context = context;
m_Timer_ID = UniqueId.random();
m_delay = 0;
m_running = false;
m_recurring = false;
ref_Event_ID = UniqueId.random();
m_Label = "";
m_expiration = new Long();
R2940_provides_delayed_delivery_of_PendingEvent_inst = PendingEventImpl.EMPTY_PENDINGEVENT;
}
private TimerImpl( Sql context, UniqueId instanceId, UniqueId m_Timer_ID, int m_delay, boolean m_running, boolean m_recurring, UniqueId ref_Event_ID, String m_Label, Long m_expiration ) {
super(instanceId);
this.context = context;
this.m_Timer_ID = m_Timer_ID;
this.m_delay = m_delay;
this.m_running = m_running;
this.m_recurring = m_recurring;
this.ref_Event_ID = ref_Event_ID;
this.m_Label = m_Label;
this.m_expiration = m_expiration;
R2940_provides_delayed_delivery_of_PendingEvent_inst = PendingEventImpl.EMPTY_PENDINGEVENT;
}
public static Timer create( Sql context ) throws XtumlException {
Timer newTimer = new TimerImpl( context );
if ( context.addInstance( newTimer ) ) {
newTimer.getRunContext().addChange(new InstanceCreatedDelta(newTimer, KEY_LETTERS));
return newTimer;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static Timer create( Sql context, UniqueId m_Timer_ID, int m_delay, boolean m_running, boolean m_recurring, UniqueId ref_Event_ID, String m_Label, Long m_expiration ) throws XtumlException {
return create(context, UniqueId.random(), m_Timer_ID, m_delay, m_running, m_recurring, ref_Event_ID, m_Label, m_expiration);
}
public static Timer create( Sql context, UniqueId instanceId, UniqueId m_Timer_ID, int m_delay, boolean m_running, boolean m_recurring, UniqueId ref_Event_ID, String m_Label, Long m_expiration ) throws XtumlException {
Timer newTimer = new TimerImpl( context, instanceId, m_Timer_ID, m_delay, m_running, m_recurring, ref_Event_ID, m_Label, m_expiration );
if ( context.addInstance( newTimer ) ) {
return newTimer;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId m_Timer_ID;
@Override
public UniqueId getTimer_ID() throws XtumlException {
checkLiving();
return m_Timer_ID;
}
@Override
public void setTimer_ID(UniqueId m_Timer_ID) throws XtumlException {
checkLiving();
if (m_Timer_ID.inequality( this.m_Timer_ID)) {
final UniqueId oldValue = this.m_Timer_ID;
this.m_Timer_ID = m_Timer_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Timer_ID", oldValue, this.m_Timer_ID));
}
}
private int m_delay;
@Override
public void setDelay(int m_delay) throws XtumlException {
checkLiving();
if (m_delay != this.m_delay) {
final int oldValue = this.m_delay;
this.m_delay = m_delay;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_delay", oldValue, this.m_delay));
}
}
@Override
public int getDelay() throws XtumlException {
checkLiving();
return m_delay;
}
private boolean m_running;
@Override
public void setRunning(boolean m_running) throws XtumlException {
checkLiving();
if (m_running != this.m_running) {
final boolean oldValue = this.m_running;
this.m_running = m_running;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_running", oldValue, this.m_running));
}
}
@Override
public boolean getRunning() throws XtumlException {
checkLiving();
return m_running;
}
private boolean m_recurring;
@Override
public boolean getRecurring() throws XtumlException {
checkLiving();
return m_recurring;
}
@Override
public void setRecurring(boolean m_recurring) throws XtumlException {
checkLiving();
if (m_recurring != this.m_recurring) {
final boolean oldValue = this.m_recurring;
this.m_recurring = m_recurring;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_recurring", oldValue, this.m_recurring));
}
}
private UniqueId ref_Event_ID;
@Override
public UniqueId getEvent_ID() throws XtumlException {
checkLiving();
return ref_Event_ID;
}
@Override
public void setEvent_ID(UniqueId ref_Event_ID) throws XtumlException {
checkLiving();
if (ref_Event_ID.inequality( this.ref_Event_ID)) {
final UniqueId oldValue = this.ref_Event_ID;
this.ref_Event_ID = ref_Event_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Event_ID", oldValue, this.ref_Event_ID));
}
}
private String m_Label;
@Override
public void setLabel(String m_Label) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Label, this.m_Label)) {
final String oldValue = this.m_Label;
this.m_Label = m_Label;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Label", oldValue, this.m_Label));
}
}
@Override
public String getLabel() throws XtumlException {
checkLiving();
return m_Label;
}
private Long m_expiration;
@Override
public Long getExpiration() throws XtumlException {
checkLiving();
return m_expiration;
}
@Override
public void setExpiration(Long m_expiration) throws XtumlException {
checkLiving();
if (m_expiration.inequality( this.m_expiration)) {
final Long oldValue = this.m_expiration;
this.m_expiration = m_expiration;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_expiration", oldValue, this.m_expiration));
}
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getTimer_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private PendingEvent R2940_provides_delayed_delivery_of_PendingEvent_inst;
@Override
public void setR2940_provides_delayed_delivery_of_PendingEvent( PendingEvent inst ) {
R2940_provides_delayed_delivery_of_PendingEvent_inst = inst;
}
@Override
public PendingEvent R2940_provides_delayed_delivery_of_PendingEvent() throws XtumlException {
return R2940_provides_delayed_delivery_of_PendingEvent_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public Timer self() {
return this;
}
@Override
public Timer oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_TIMER;
}
}
class EmptyTimer extends ModelInstance implements Timer {
// attributes
public UniqueId getTimer_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setTimer_ID( UniqueId m_Timer_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setDelay( int m_delay ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getDelay() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setRunning( boolean m_running ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public boolean getRunning() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public boolean getRecurring() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setRecurring( boolean m_recurring ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getEvent_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setEvent_ID( UniqueId ref_Event_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setLabel( String m_Label ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getLabel() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public Long getExpiration() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setExpiration( Long m_expiration ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
// operations
// selections
@Override
public PendingEvent R2940_provides_delayed_delivery_of_PendingEvent() {
return PendingEventImpl.EMPTY_PENDINGEVENT;
}
@Override
public String getKeyLetters() {
return TimerImpl.KEY_LETTERS;
}
@Override
public Timer self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public Timer oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return TimerImpl.EMPTY_TIMER;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy