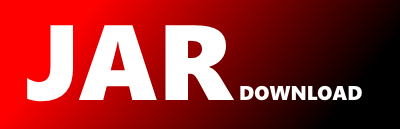
io.ciera.tool.sql.ooaofooa.interaction.impl.InteractionParticipantSetImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.interaction.impl;
import io.ciera.runtime.summit.classes.InstanceSet;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.sql.ooaofooa.communication.CommunicationLinkSet;
import io.ciera.tool.sql.ooaofooa.communication.impl.CommunicationLinkSetImpl;
import io.ciera.tool.sql.ooaofooa.interaction.ActorParticipantSet;
import io.ciera.tool.sql.ooaofooa.interaction.ClassInstanceParticipantSet;
import io.ciera.tool.sql.ooaofooa.interaction.ClassParticipantSet;
import io.ciera.tool.sql.ooaofooa.interaction.ComponentParticipantSet;
import io.ciera.tool.sql.ooaofooa.interaction.ExternalEntityParticipantSet;
import io.ciera.tool.sql.ooaofooa.interaction.InteractionParticipant;
import io.ciera.tool.sql.ooaofooa.interaction.InteractionParticipantSet;
import io.ciera.tool.sql.ooaofooa.interaction.LifespanSet;
import io.ciera.tool.sql.ooaofooa.interaction.PackageParticipantSet;
import io.ciera.tool.sql.ooaofooa.interaction.UseCaseParticipantSet;
import io.ciera.tool.sql.ooaofooa.interaction.impl.ActorParticipantSetImpl;
import io.ciera.tool.sql.ooaofooa.interaction.impl.ClassInstanceParticipantSetImpl;
import io.ciera.tool.sql.ooaofooa.interaction.impl.ClassParticipantSetImpl;
import io.ciera.tool.sql.ooaofooa.interaction.impl.ComponentParticipantSetImpl;
import io.ciera.tool.sql.ooaofooa.interaction.impl.ExternalEntityParticipantSetImpl;
import io.ciera.tool.sql.ooaofooa.interaction.impl.LifespanSetImpl;
import io.ciera.tool.sql.ooaofooa.interaction.impl.PackageParticipantSetImpl;
import io.ciera.tool.sql.ooaofooa.interaction.impl.UseCaseParticipantSetImpl;
import io.ciera.tool.sql.ooaofooa.message.MSG_MSet;
import io.ciera.tool.sql.ooaofooa.message.impl.MSG_MSetImpl;
import io.ciera.tool.sql.ooaofooa.packageableelement.PackageableElementSet;
import io.ciera.tool.sql.ooaofooa.packageableelement.impl.PackageableElementSetImpl;
import io.ciera.tool.sql.ooaofooa.usecase.UseCaseAssociationSet;
import io.ciera.tool.sql.ooaofooa.usecase.impl.UseCaseAssociationSetImpl;
import java.util.Arrays;
import java.util.Comparator;
import java.util.List;
public class InteractionParticipantSetImpl extends InstanceSet implements InteractionParticipantSet {
public InteractionParticipantSetImpl() {
}
public InteractionParticipantSetImpl(Comparator super InteractionParticipant> comp) {
super(comp);
}
// attributes
@Override
public void setSequence_Package_IDdeprecated( UniqueId m_Sequence_Package_IDdeprecated ) throws XtumlException {
for ( InteractionParticipant interactionparticipant : this ) interactionparticipant.setSequence_Package_IDdeprecated( m_Sequence_Package_IDdeprecated );
}
@Override
public void setPart_ID( UniqueId ref_Part_ID ) throws XtumlException {
for ( InteractionParticipant interactionparticipant : this ) interactionparticipant.setPart_ID( ref_Part_ID );
}
// selections
@Override
public MSG_MSet R1007_receives_MSG_M() throws XtumlException {
MSG_MSet msg_mset = new MSG_MSetImpl();
for ( InteractionParticipant interactionparticipant : this ) msg_mset.addAll( interactionparticipant.R1007_receives_MSG_M() );
return msg_mset;
}
@Override
public MSG_MSet R1008_sends_MSG_M() throws XtumlException {
MSG_MSet msg_mset = new MSG_MSetImpl();
for ( InteractionParticipant interactionparticipant : this ) msg_mset.addAll( interactionparticipant.R1008_sends_MSG_M() );
return msg_mset;
}
@Override
public CommunicationLinkSet R1133_is_start_point_CommunicationLink() throws XtumlException {
CommunicationLinkSet communicationlinkset = new CommunicationLinkSetImpl();
for ( InteractionParticipant interactionparticipant : this ) communicationlinkset.addAll( interactionparticipant.R1133_is_start_point_CommunicationLink() );
return communicationlinkset;
}
@Override
public CommunicationLinkSet R1134_is_destination_CommunicationLink() throws XtumlException {
CommunicationLinkSet communicationlinkset = new CommunicationLinkSetImpl();
for ( InteractionParticipant interactionparticipant : this ) communicationlinkset.addAll( interactionparticipant.R1134_is_destination_CommunicationLink() );
return communicationlinkset;
}
@Override
public UseCaseAssociationSet R1206_is_source_UseCaseAssociation() throws XtumlException {
UseCaseAssociationSet usecaseassociationset = new UseCaseAssociationSetImpl();
for ( InteractionParticipant interactionparticipant : this ) usecaseassociationset.addAll( interactionparticipant.R1206_is_source_UseCaseAssociation() );
return usecaseassociationset;
}
@Override
public UseCaseAssociationSet R1207_is_destination_UseCaseAssociation() throws XtumlException {
UseCaseAssociationSet usecaseassociationset = new UseCaseAssociationSetImpl();
for ( InteractionParticipant interactionparticipant : this ) usecaseassociationset.addAll( interactionparticipant.R1207_is_destination_UseCaseAssociation() );
return usecaseassociationset;
}
@Override
public PackageableElementSet R8001_is_a_PackageableElement() throws XtumlException {
PackageableElementSet packageableelementset = new PackageableElementSetImpl();
for ( InteractionParticipant interactionparticipant : this ) packageableelementset.add( interactionparticipant.R8001_is_a_PackageableElement() );
return packageableelementset;
}
@Override
public ActorParticipantSet R930_is_a_ActorParticipant() throws XtumlException {
ActorParticipantSet actorparticipantset = new ActorParticipantSetImpl();
for ( InteractionParticipant interactionparticipant : this ) actorparticipantset.add( interactionparticipant.R930_is_a_ActorParticipant() );
return actorparticipantset;
}
@Override
public ClassInstanceParticipantSet R930_is_a_ClassInstanceParticipant() throws XtumlException {
ClassInstanceParticipantSet classinstanceparticipantset = new ClassInstanceParticipantSetImpl();
for ( InteractionParticipant interactionparticipant : this ) classinstanceparticipantset.add( interactionparticipant.R930_is_a_ClassInstanceParticipant() );
return classinstanceparticipantset;
}
@Override
public ClassParticipantSet R930_is_a_ClassParticipant() throws XtumlException {
ClassParticipantSet classparticipantset = new ClassParticipantSetImpl();
for ( InteractionParticipant interactionparticipant : this ) classparticipantset.add( interactionparticipant.R930_is_a_ClassParticipant() );
return classparticipantset;
}
@Override
public ComponentParticipantSet R930_is_a_ComponentParticipant() throws XtumlException {
ComponentParticipantSet componentparticipantset = new ComponentParticipantSetImpl();
for ( InteractionParticipant interactionparticipant : this ) componentparticipantset.add( interactionparticipant.R930_is_a_ComponentParticipant() );
return componentparticipantset;
}
@Override
public ExternalEntityParticipantSet R930_is_a_ExternalEntityParticipant() throws XtumlException {
ExternalEntityParticipantSet externalentityparticipantset = new ExternalEntityParticipantSetImpl();
for ( InteractionParticipant interactionparticipant : this ) externalentityparticipantset.add( interactionparticipant.R930_is_a_ExternalEntityParticipant() );
return externalentityparticipantset;
}
@Override
public LifespanSet R930_is_a_Lifespan() throws XtumlException {
LifespanSet lifespanset = new LifespanSetImpl();
for ( InteractionParticipant interactionparticipant : this ) lifespanset.add( interactionparticipant.R930_is_a_Lifespan() );
return lifespanset;
}
@Override
public PackageParticipantSet R930_is_a_PackageParticipant() throws XtumlException {
PackageParticipantSet packageparticipantset = new PackageParticipantSetImpl();
for ( InteractionParticipant interactionparticipant : this ) packageparticipantset.add( interactionparticipant.R930_is_a_PackageParticipant() );
return packageparticipantset;
}
@Override
public UseCaseParticipantSet R930_is_a_UseCaseParticipant() throws XtumlException {
UseCaseParticipantSet usecaseparticipantset = new UseCaseParticipantSetImpl();
for ( InteractionParticipant interactionparticipant : this ) usecaseparticipantset.add( interactionparticipant.R930_is_a_UseCaseParticipant() );
return usecaseparticipantset;
}
@Override
public LifespanSet R940_is_source_of_span_Lifespan() throws XtumlException {
LifespanSet lifespanset = new LifespanSetImpl();
for ( InteractionParticipant interactionparticipant : this ) lifespanset.add( interactionparticipant.R940_is_source_of_span_Lifespan() );
return lifespanset;
}
@Override
public InteractionParticipant nullElement() {
return InteractionParticipantImpl.EMPTY_INTERACTIONPARTICIPANT;
}
@Override
public InteractionParticipantSet emptySet() {
return new InteractionParticipantSetImpl();
}
@Override
public InteractionParticipantSet emptySet(Comparator super InteractionParticipant> comp) {
return new InteractionParticipantSetImpl(comp);
}
@Override
public List elements() {
return Arrays.asList(toArray(new InteractionParticipant[0]));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy