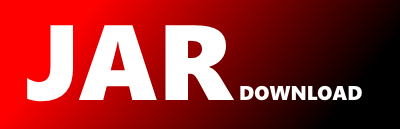
io.ciera.tool.sql.ooaofooa.interaction.impl.LifespanImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.interaction.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.interaction.ActorParticipant;
import io.ciera.tool.sql.ooaofooa.interaction.InteractionParticipant;
import io.ciera.tool.sql.ooaofooa.interaction.Lifespan;
import io.ciera.tool.sql.ooaofooa.interaction.TimingMark;
import io.ciera.tool.sql.ooaofooa.interaction.TimingMarkSet;
import io.ciera.tool.sql.ooaofooa.interaction.impl.ActorParticipantImpl;
import io.ciera.tool.sql.ooaofooa.interaction.impl.InteractionParticipantImpl;
import io.ciera.tool.sql.ooaofooa.interaction.impl.TimingMarkSetImpl;
public class LifespanImpl extends ModelInstance implements Lifespan {
public static final String KEY_LETTERS = "SQ_LS";
public static final Lifespan EMPTY_LIFESPAN = new EmptyLifespan();
private Sql context;
// constructors
private LifespanImpl( Sql context ) {
this.context = context;
ref_Part_ID = UniqueId.random();
ref_Source_Part_ID = UniqueId.random();
m_Descrip = "";
m_Destroyed = false;
R930_is_a_InteractionParticipant_inst = InteractionParticipantImpl.EMPTY_INTERACTIONPARTICIPANT;
R931_has_a_point_in_time_referenced_by_TimingMark_set = new TimingMarkSetImpl();
R940_extends_from_InteractionParticipant_inst = InteractionParticipantImpl.EMPTY_INTERACTIONPARTICIPANT;
R949_defines_bounds_of_existence_ActorParticipant_inst = ActorParticipantImpl.EMPTY_ACTORPARTICIPANT;
}
private LifespanImpl( Sql context, UniqueId instanceId, UniqueId ref_Part_ID, UniqueId ref_Source_Part_ID, String m_Descrip, boolean m_Destroyed ) {
super(instanceId);
this.context = context;
this.ref_Part_ID = ref_Part_ID;
this.ref_Source_Part_ID = ref_Source_Part_ID;
this.m_Descrip = m_Descrip;
this.m_Destroyed = m_Destroyed;
R930_is_a_InteractionParticipant_inst = InteractionParticipantImpl.EMPTY_INTERACTIONPARTICIPANT;
R931_has_a_point_in_time_referenced_by_TimingMark_set = new TimingMarkSetImpl();
R940_extends_from_InteractionParticipant_inst = InteractionParticipantImpl.EMPTY_INTERACTIONPARTICIPANT;
R949_defines_bounds_of_existence_ActorParticipant_inst = ActorParticipantImpl.EMPTY_ACTORPARTICIPANT;
}
public static Lifespan create( Sql context ) throws XtumlException {
Lifespan newLifespan = new LifespanImpl( context );
if ( context.addInstance( newLifespan ) ) {
newLifespan.getRunContext().addChange(new InstanceCreatedDelta(newLifespan, KEY_LETTERS));
return newLifespan;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static Lifespan create( Sql context, UniqueId ref_Part_ID, UniqueId ref_Source_Part_ID, String m_Descrip, boolean m_Destroyed ) throws XtumlException {
return create(context, UniqueId.random(), ref_Part_ID, ref_Source_Part_ID, m_Descrip, m_Destroyed);
}
public static Lifespan create( Sql context, UniqueId instanceId, UniqueId ref_Part_ID, UniqueId ref_Source_Part_ID, String m_Descrip, boolean m_Destroyed ) throws XtumlException {
Lifespan newLifespan = new LifespanImpl( context, instanceId, ref_Part_ID, ref_Source_Part_ID, m_Descrip, m_Destroyed );
if ( context.addInstance( newLifespan ) ) {
return newLifespan;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId ref_Part_ID;
@Override
public void setPart_ID(UniqueId ref_Part_ID) throws XtumlException {
checkLiving();
if (ref_Part_ID.inequality( this.ref_Part_ID)) {
final UniqueId oldValue = this.ref_Part_ID;
this.ref_Part_ID = ref_Part_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Part_ID", oldValue, this.ref_Part_ID));
if ( !R931_has_a_point_in_time_referenced_by_TimingMark().isEmpty() ) R931_has_a_point_in_time_referenced_by_TimingMark().setPart_ID( ref_Part_ID );
if ( !R949_defines_bounds_of_existence_ActorParticipant().isEmpty() ) R949_defines_bounds_of_existence_ActorParticipant().setLS_Part_ID( ref_Part_ID );
}
}
@Override
public UniqueId getPart_ID() throws XtumlException {
checkLiving();
return ref_Part_ID;
}
private UniqueId ref_Source_Part_ID;
@Override
public void setSource_Part_ID(UniqueId ref_Source_Part_ID) throws XtumlException {
checkLiving();
if (ref_Source_Part_ID.inequality( this.ref_Source_Part_ID)) {
final UniqueId oldValue = this.ref_Source_Part_ID;
this.ref_Source_Part_ID = ref_Source_Part_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Source_Part_ID", oldValue, this.ref_Source_Part_ID));
}
}
@Override
public UniqueId getSource_Part_ID() throws XtumlException {
checkLiving();
return ref_Source_Part_ID;
}
private String m_Descrip;
@Override
public String getDescrip() throws XtumlException {
checkLiving();
return m_Descrip;
}
@Override
public void setDescrip(String m_Descrip) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Descrip, this.m_Descrip)) {
final String oldValue = this.m_Descrip;
this.m_Descrip = m_Descrip;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Descrip", oldValue, this.m_Descrip));
}
}
private boolean m_Destroyed;
@Override
public boolean getDestroyed() throws XtumlException {
checkLiving();
return m_Destroyed;
}
@Override
public void setDestroyed(boolean m_Destroyed) throws XtumlException {
checkLiving();
if (m_Destroyed != this.m_Destroyed) {
final boolean oldValue = this.m_Destroyed;
this.m_Destroyed = m_Destroyed;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Destroyed", oldValue, this.m_Destroyed));
}
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getPart_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private InteractionParticipant R930_is_a_InteractionParticipant_inst;
@Override
public void setR930_is_a_InteractionParticipant( InteractionParticipant inst ) {
R930_is_a_InteractionParticipant_inst = inst;
}
@Override
public InteractionParticipant R930_is_a_InteractionParticipant() throws XtumlException {
return R930_is_a_InteractionParticipant_inst;
}
private TimingMarkSet R931_has_a_point_in_time_referenced_by_TimingMark_set;
@Override
public void addR931_has_a_point_in_time_referenced_by_TimingMark( TimingMark inst ) {
R931_has_a_point_in_time_referenced_by_TimingMark_set.add(inst);
}
@Override
public void removeR931_has_a_point_in_time_referenced_by_TimingMark( TimingMark inst ) {
R931_has_a_point_in_time_referenced_by_TimingMark_set.remove(inst);
}
@Override
public TimingMarkSet R931_has_a_point_in_time_referenced_by_TimingMark() throws XtumlException {
return R931_has_a_point_in_time_referenced_by_TimingMark_set;
}
private InteractionParticipant R940_extends_from_InteractionParticipant_inst;
@Override
public void setR940_extends_from_InteractionParticipant( InteractionParticipant inst ) {
R940_extends_from_InteractionParticipant_inst = inst;
}
@Override
public InteractionParticipant R940_extends_from_InteractionParticipant() throws XtumlException {
return R940_extends_from_InteractionParticipant_inst;
}
private ActorParticipant R949_defines_bounds_of_existence_ActorParticipant_inst;
@Override
public void setR949_defines_bounds_of_existence_ActorParticipant( ActorParticipant inst ) {
R949_defines_bounds_of_existence_ActorParticipant_inst = inst;
}
@Override
public ActorParticipant R949_defines_bounds_of_existence_ActorParticipant() throws XtumlException {
return R949_defines_bounds_of_existence_ActorParticipant_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public Lifespan self() {
return this;
}
@Override
public Lifespan oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_LIFESPAN;
}
}
class EmptyLifespan extends ModelInstance implements Lifespan {
// attributes
public void setPart_ID( UniqueId ref_Part_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getPart_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setSource_Part_ID( UniqueId ref_Source_Part_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getSource_Part_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getDescrip() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setDescrip( String m_Descrip ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public boolean getDestroyed() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setDestroyed( boolean m_Destroyed ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
// operations
// selections
@Override
public InteractionParticipant R930_is_a_InteractionParticipant() {
return InteractionParticipantImpl.EMPTY_INTERACTIONPARTICIPANT;
}
@Override
public TimingMarkSet R931_has_a_point_in_time_referenced_by_TimingMark() {
return (new TimingMarkSetImpl());
}
@Override
public InteractionParticipant R940_extends_from_InteractionParticipant() {
return InteractionParticipantImpl.EMPTY_INTERACTIONPARTICIPANT;
}
@Override
public ActorParticipant R949_defines_bounds_of_existence_ActorParticipant() {
return ActorParticipantImpl.EMPTY_ACTORPARTICIPANT;
}
@Override
public String getKeyLetters() {
return LifespanImpl.KEY_LETTERS;
}
@Override
public Lifespan self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public Lifespan oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return LifespanImpl.EMPTY_LIFESPAN;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy