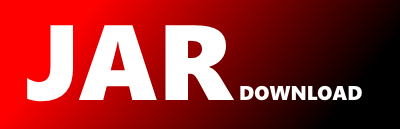
io.ciera.tool.sql.ooaofooa.message.impl.MessageArgumentImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.message.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.message.BridgeArgument;
import io.ciera.tool.sql.ooaofooa.message.EventArgument;
import io.ciera.tool.sql.ooaofooa.message.ExecutablePropertyArgument;
import io.ciera.tool.sql.ooaofooa.message.FunctionArgument;
import io.ciera.tool.sql.ooaofooa.message.InformalArgument;
import io.ciera.tool.sql.ooaofooa.message.MSG_M;
import io.ciera.tool.sql.ooaofooa.message.MessageArgument;
import io.ciera.tool.sql.ooaofooa.message.OperationArgument;
import io.ciera.tool.sql.ooaofooa.message.impl.BridgeArgumentImpl;
import io.ciera.tool.sql.ooaofooa.message.impl.EventArgumentImpl;
import io.ciera.tool.sql.ooaofooa.message.impl.ExecutablePropertyArgumentImpl;
import io.ciera.tool.sql.ooaofooa.message.impl.FunctionArgumentImpl;
import io.ciera.tool.sql.ooaofooa.message.impl.InformalArgumentImpl;
import io.ciera.tool.sql.ooaofooa.message.impl.MSG_MImpl;
import io.ciera.tool.sql.ooaofooa.message.impl.OperationArgumentImpl;
public class MessageArgumentImpl extends ModelInstance implements MessageArgument {
public static final String KEY_LETTERS = "MSG_A";
public static final MessageArgument EMPTY_MESSAGEARGUMENT = new EmptyMessageArgument();
private Sql context;
// constructors
private MessageArgumentImpl( Sql context ) {
this.context = context;
m_Arg_ID = UniqueId.random();
ref_Informal_Msg_ID = UniqueId.random();
ref_Formal_Msg_ID = UniqueId.random();
m_Label = "";
m_Value = "";
m_InformalName = "";
m_Descrip = "";
m_isFormal = false;
R1000_belongs_to_informal_MSG_M_inst = MSG_MImpl.EMPTY_MSG_M;
R1001_belongs_to_formal_MSG_M_inst = MSG_MImpl.EMPTY_MSG_M;
R1013_is_a_BridgeArgument_inst = BridgeArgumentImpl.EMPTY_BRIDGEARGUMENT;
R1013_is_a_EventArgument_inst = EventArgumentImpl.EMPTY_EVENTARGUMENT;
R1013_is_a_ExecutablePropertyArgument_inst = ExecutablePropertyArgumentImpl.EMPTY_EXECUTABLEPROPERTYARGUMENT;
R1013_is_a_FunctionArgument_inst = FunctionArgumentImpl.EMPTY_FUNCTIONARGUMENT;
R1013_is_a_InformalArgument_inst = InformalArgumentImpl.EMPTY_INFORMALARGUMENT;
R1013_is_a_OperationArgument_inst = OperationArgumentImpl.EMPTY_OPERATIONARGUMENT;
}
private MessageArgumentImpl( Sql context, UniqueId instanceId, UniqueId m_Arg_ID, UniqueId ref_Informal_Msg_ID, UniqueId ref_Formal_Msg_ID, String m_Label, String m_Value, String m_InformalName, String m_Descrip, boolean m_isFormal ) {
super(instanceId);
this.context = context;
this.m_Arg_ID = m_Arg_ID;
this.ref_Informal_Msg_ID = ref_Informal_Msg_ID;
this.ref_Formal_Msg_ID = ref_Formal_Msg_ID;
this.m_Label = m_Label;
this.m_Value = m_Value;
this.m_InformalName = m_InformalName;
this.m_Descrip = m_Descrip;
this.m_isFormal = m_isFormal;
R1000_belongs_to_informal_MSG_M_inst = MSG_MImpl.EMPTY_MSG_M;
R1001_belongs_to_formal_MSG_M_inst = MSG_MImpl.EMPTY_MSG_M;
R1013_is_a_BridgeArgument_inst = BridgeArgumentImpl.EMPTY_BRIDGEARGUMENT;
R1013_is_a_EventArgument_inst = EventArgumentImpl.EMPTY_EVENTARGUMENT;
R1013_is_a_ExecutablePropertyArgument_inst = ExecutablePropertyArgumentImpl.EMPTY_EXECUTABLEPROPERTYARGUMENT;
R1013_is_a_FunctionArgument_inst = FunctionArgumentImpl.EMPTY_FUNCTIONARGUMENT;
R1013_is_a_InformalArgument_inst = InformalArgumentImpl.EMPTY_INFORMALARGUMENT;
R1013_is_a_OperationArgument_inst = OperationArgumentImpl.EMPTY_OPERATIONARGUMENT;
}
public static MessageArgument create( Sql context ) throws XtumlException {
MessageArgument newMessageArgument = new MessageArgumentImpl( context );
if ( context.addInstance( newMessageArgument ) ) {
newMessageArgument.getRunContext().addChange(new InstanceCreatedDelta(newMessageArgument, KEY_LETTERS));
return newMessageArgument;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static MessageArgument create( Sql context, UniqueId m_Arg_ID, UniqueId ref_Informal_Msg_ID, UniqueId ref_Formal_Msg_ID, String m_Label, String m_Value, String m_InformalName, String m_Descrip, boolean m_isFormal ) throws XtumlException {
return create(context, UniqueId.random(), m_Arg_ID, ref_Informal_Msg_ID, ref_Formal_Msg_ID, m_Label, m_Value, m_InformalName, m_Descrip, m_isFormal);
}
public static MessageArgument create( Sql context, UniqueId instanceId, UniqueId m_Arg_ID, UniqueId ref_Informal_Msg_ID, UniqueId ref_Formal_Msg_ID, String m_Label, String m_Value, String m_InformalName, String m_Descrip, boolean m_isFormal ) throws XtumlException {
MessageArgument newMessageArgument = new MessageArgumentImpl( context, instanceId, m_Arg_ID, ref_Informal_Msg_ID, ref_Formal_Msg_ID, m_Label, m_Value, m_InformalName, m_Descrip, m_isFormal );
if ( context.addInstance( newMessageArgument ) ) {
return newMessageArgument;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId m_Arg_ID;
@Override
public UniqueId getArg_ID() throws XtumlException {
checkLiving();
return m_Arg_ID;
}
@Override
public void setArg_ID(UniqueId m_Arg_ID) throws XtumlException {
checkLiving();
if (m_Arg_ID.inequality( this.m_Arg_ID)) {
final UniqueId oldValue = this.m_Arg_ID;
this.m_Arg_ID = m_Arg_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Arg_ID", oldValue, this.m_Arg_ID));
if ( !R1013_is_a_EventArgument().isEmpty() ) R1013_is_a_EventArgument().setArg_ID( m_Arg_ID );
if ( !R1013_is_a_FunctionArgument().isEmpty() ) R1013_is_a_FunctionArgument().setArg_ID( m_Arg_ID );
if ( !R1013_is_a_InformalArgument().isEmpty() ) R1013_is_a_InformalArgument().setArg_ID( m_Arg_ID );
if ( !R1013_is_a_BridgeArgument().isEmpty() ) R1013_is_a_BridgeArgument().setArg_ID( m_Arg_ID );
if ( !R1013_is_a_ExecutablePropertyArgument().isEmpty() ) R1013_is_a_ExecutablePropertyArgument().setArg_ID( m_Arg_ID );
if ( !R1013_is_a_OperationArgument().isEmpty() ) R1013_is_a_OperationArgument().setArg_ID( m_Arg_ID );
}
}
private UniqueId ref_Informal_Msg_ID;
@Override
public void setInformal_Msg_ID(UniqueId ref_Informal_Msg_ID) throws XtumlException {
checkLiving();
if (ref_Informal_Msg_ID.inequality( this.ref_Informal_Msg_ID)) {
final UniqueId oldValue = this.ref_Informal_Msg_ID;
this.ref_Informal_Msg_ID = ref_Informal_Msg_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Informal_Msg_ID", oldValue, this.ref_Informal_Msg_ID));
}
}
@Override
public UniqueId getInformal_Msg_ID() throws XtumlException {
checkLiving();
return ref_Informal_Msg_ID;
}
private UniqueId ref_Formal_Msg_ID;
@Override
public void setFormal_Msg_ID(UniqueId ref_Formal_Msg_ID) throws XtumlException {
checkLiving();
if (ref_Formal_Msg_ID.inequality( this.ref_Formal_Msg_ID)) {
final UniqueId oldValue = this.ref_Formal_Msg_ID;
this.ref_Formal_Msg_ID = ref_Formal_Msg_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Formal_Msg_ID", oldValue, this.ref_Formal_Msg_ID));
}
}
@Override
public UniqueId getFormal_Msg_ID() throws XtumlException {
checkLiving();
return ref_Formal_Msg_ID;
}
private String m_Label;
@Override
public String getLabel() throws XtumlException {
checkLiving();
return m_Label;
}
@Override
public void setLabel(String m_Label) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Label, this.m_Label)) {
final String oldValue = this.m_Label;
this.m_Label = m_Label;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Label", oldValue, this.m_Label));
}
}
private String m_Value;
@Override
public String getValue() throws XtumlException {
checkLiving();
return m_Value;
}
@Override
public void setValue(String m_Value) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Value, this.m_Value)) {
final String oldValue = this.m_Value;
this.m_Value = m_Value;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Value", oldValue, this.m_Value));
}
}
private String m_InformalName;
@Override
public String getInformalName() throws XtumlException {
checkLiving();
return m_InformalName;
}
@Override
public void setInformalName(String m_InformalName) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_InformalName, this.m_InformalName)) {
final String oldValue = this.m_InformalName;
this.m_InformalName = m_InformalName;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_InformalName", oldValue, this.m_InformalName));
}
}
private String m_Descrip;
@Override
public void setDescrip(String m_Descrip) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Descrip, this.m_Descrip)) {
final String oldValue = this.m_Descrip;
this.m_Descrip = m_Descrip;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Descrip", oldValue, this.m_Descrip));
}
}
@Override
public String getDescrip() throws XtumlException {
checkLiving();
return m_Descrip;
}
private boolean m_isFormal;
@Override
public void setIsFormal(boolean m_isFormal) throws XtumlException {
checkLiving();
if (m_isFormal != this.m_isFormal) {
final boolean oldValue = this.m_isFormal;
this.m_isFormal = m_isFormal;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_isFormal", oldValue, this.m_isFormal));
}
}
@Override
public boolean getIsFormal() throws XtumlException {
checkLiving();
return m_isFormal;
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getArg_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private MSG_M R1000_belongs_to_informal_MSG_M_inst;
@Override
public void setR1000_belongs_to_informal_MSG_M( MSG_M inst ) {
R1000_belongs_to_informal_MSG_M_inst = inst;
}
@Override
public MSG_M R1000_belongs_to_informal_MSG_M() throws XtumlException {
return R1000_belongs_to_informal_MSG_M_inst;
}
private MSG_M R1001_belongs_to_formal_MSG_M_inst;
@Override
public void setR1001_belongs_to_formal_MSG_M( MSG_M inst ) {
R1001_belongs_to_formal_MSG_M_inst = inst;
}
@Override
public MSG_M R1001_belongs_to_formal_MSG_M() throws XtumlException {
return R1001_belongs_to_formal_MSG_M_inst;
}
private BridgeArgument R1013_is_a_BridgeArgument_inst;
@Override
public void setR1013_is_a_BridgeArgument( BridgeArgument inst ) {
R1013_is_a_BridgeArgument_inst = inst;
}
@Override
public BridgeArgument R1013_is_a_BridgeArgument() throws XtumlException {
return R1013_is_a_BridgeArgument_inst;
}
private EventArgument R1013_is_a_EventArgument_inst;
@Override
public void setR1013_is_a_EventArgument( EventArgument inst ) {
R1013_is_a_EventArgument_inst = inst;
}
@Override
public EventArgument R1013_is_a_EventArgument() throws XtumlException {
return R1013_is_a_EventArgument_inst;
}
private ExecutablePropertyArgument R1013_is_a_ExecutablePropertyArgument_inst;
@Override
public void setR1013_is_a_ExecutablePropertyArgument( ExecutablePropertyArgument inst ) {
R1013_is_a_ExecutablePropertyArgument_inst = inst;
}
@Override
public ExecutablePropertyArgument R1013_is_a_ExecutablePropertyArgument() throws XtumlException {
return R1013_is_a_ExecutablePropertyArgument_inst;
}
private FunctionArgument R1013_is_a_FunctionArgument_inst;
@Override
public void setR1013_is_a_FunctionArgument( FunctionArgument inst ) {
R1013_is_a_FunctionArgument_inst = inst;
}
@Override
public FunctionArgument R1013_is_a_FunctionArgument() throws XtumlException {
return R1013_is_a_FunctionArgument_inst;
}
private InformalArgument R1013_is_a_InformalArgument_inst;
@Override
public void setR1013_is_a_InformalArgument( InformalArgument inst ) {
R1013_is_a_InformalArgument_inst = inst;
}
@Override
public InformalArgument R1013_is_a_InformalArgument() throws XtumlException {
return R1013_is_a_InformalArgument_inst;
}
private OperationArgument R1013_is_a_OperationArgument_inst;
@Override
public void setR1013_is_a_OperationArgument( OperationArgument inst ) {
R1013_is_a_OperationArgument_inst = inst;
}
@Override
public OperationArgument R1013_is_a_OperationArgument() throws XtumlException {
return R1013_is_a_OperationArgument_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public MessageArgument self() {
return this;
}
@Override
public MessageArgument oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_MESSAGEARGUMENT;
}
}
class EmptyMessageArgument extends ModelInstance implements MessageArgument {
// attributes
public UniqueId getArg_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setArg_ID( UniqueId m_Arg_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setInformal_Msg_ID( UniqueId ref_Informal_Msg_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getInformal_Msg_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setFormal_Msg_ID( UniqueId ref_Formal_Msg_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getFormal_Msg_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getLabel() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setLabel( String m_Label ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getValue() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setValue( String m_Value ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getInformalName() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setInformalName( String m_InformalName ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setDescrip( String m_Descrip ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getDescrip() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setIsFormal( boolean m_isFormal ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public boolean getIsFormal() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
// operations
// selections
@Override
public MSG_M R1000_belongs_to_informal_MSG_M() {
return MSG_MImpl.EMPTY_MSG_M;
}
@Override
public MSG_M R1001_belongs_to_formal_MSG_M() {
return MSG_MImpl.EMPTY_MSG_M;
}
@Override
public BridgeArgument R1013_is_a_BridgeArgument() {
return BridgeArgumentImpl.EMPTY_BRIDGEARGUMENT;
}
@Override
public EventArgument R1013_is_a_EventArgument() {
return EventArgumentImpl.EMPTY_EVENTARGUMENT;
}
@Override
public ExecutablePropertyArgument R1013_is_a_ExecutablePropertyArgument() {
return ExecutablePropertyArgumentImpl.EMPTY_EXECUTABLEPROPERTYARGUMENT;
}
@Override
public FunctionArgument R1013_is_a_FunctionArgument() {
return FunctionArgumentImpl.EMPTY_FUNCTIONARGUMENT;
}
@Override
public InformalArgument R1013_is_a_InformalArgument() {
return InformalArgumentImpl.EMPTY_INFORMALARGUMENT;
}
@Override
public OperationArgument R1013_is_a_OperationArgument() {
return OperationArgumentImpl.EMPTY_OPERATIONARGUMENT;
}
@Override
public String getKeyLetters() {
return MessageArgumentImpl.KEY_LETTERS;
}
@Override
public MessageArgument self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public MessageArgument oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return MessageArgumentImpl.EMPTY_MESSAGEARGUMENT;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy