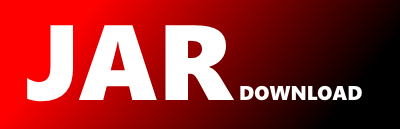
io.ciera.tool.sql.ooaofooa.message.impl.SynchronousMessageImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.message.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.message.BridgeMessage;
import io.ciera.tool.sql.ooaofooa.message.FunctionMessage;
import io.ciera.tool.sql.ooaofooa.message.InformalSynchronousMessage;
import io.ciera.tool.sql.ooaofooa.message.InterfaceOperationMessage;
import io.ciera.tool.sql.ooaofooa.message.MSG_M;
import io.ciera.tool.sql.ooaofooa.message.OperationMessage;
import io.ciera.tool.sql.ooaofooa.message.SynchronousMessage;
import io.ciera.tool.sql.ooaofooa.message.impl.BridgeMessageImpl;
import io.ciera.tool.sql.ooaofooa.message.impl.FunctionMessageImpl;
import io.ciera.tool.sql.ooaofooa.message.impl.InformalSynchronousMessageImpl;
import io.ciera.tool.sql.ooaofooa.message.impl.InterfaceOperationMessageImpl;
import io.ciera.tool.sql.ooaofooa.message.impl.MSG_MImpl;
import io.ciera.tool.sql.ooaofooa.message.impl.OperationMessageImpl;
public class SynchronousMessageImpl extends ModelInstance implements SynchronousMessage {
public static final String KEY_LETTERS = "MSG_SM";
public static final SynchronousMessage EMPTY_SYNCHRONOUSMESSAGE = new EmptySynchronousMessage();
private Sql context;
// constructors
private SynchronousMessageImpl( Sql context ) {
this.context = context;
ref_Msg_ID = UniqueId.random();
m_InformalName = "";
m_Descrip = "";
m_GuardCondition = "";
m_ResultTarget = "";
m_ReturnValue = "";
m_isFormal = false;
m_Label = "";
m_SequenceNumb = "";
R1018_is_a_MSG_M_inst = MSG_MImpl.EMPTY_MSG_M;
R1020_is_a_BridgeMessage_inst = BridgeMessageImpl.EMPTY_BRIDGEMESSAGE;
R1020_is_a_FunctionMessage_inst = FunctionMessageImpl.EMPTY_FUNCTIONMESSAGE;
R1020_is_a_InformalSynchronousMessage_inst = InformalSynchronousMessageImpl.EMPTY_INFORMALSYNCHRONOUSMESSAGE;
R1020_is_a_InterfaceOperationMessage_inst = InterfaceOperationMessageImpl.EMPTY_INTERFACEOPERATIONMESSAGE;
R1020_is_a_OperationMessage_inst = OperationMessageImpl.EMPTY_OPERATIONMESSAGE;
}
private SynchronousMessageImpl( Sql context, UniqueId instanceId, UniqueId ref_Msg_ID, String m_InformalName, String m_Descrip, String m_GuardCondition, String m_ResultTarget, String m_ReturnValue, boolean m_isFormal, String m_Label, String m_SequenceNumb ) {
super(instanceId);
this.context = context;
this.ref_Msg_ID = ref_Msg_ID;
this.m_InformalName = m_InformalName;
this.m_Descrip = m_Descrip;
this.m_GuardCondition = m_GuardCondition;
this.m_ResultTarget = m_ResultTarget;
this.m_ReturnValue = m_ReturnValue;
this.m_isFormal = m_isFormal;
this.m_Label = m_Label;
this.m_SequenceNumb = m_SequenceNumb;
R1018_is_a_MSG_M_inst = MSG_MImpl.EMPTY_MSG_M;
R1020_is_a_BridgeMessage_inst = BridgeMessageImpl.EMPTY_BRIDGEMESSAGE;
R1020_is_a_FunctionMessage_inst = FunctionMessageImpl.EMPTY_FUNCTIONMESSAGE;
R1020_is_a_InformalSynchronousMessage_inst = InformalSynchronousMessageImpl.EMPTY_INFORMALSYNCHRONOUSMESSAGE;
R1020_is_a_InterfaceOperationMessage_inst = InterfaceOperationMessageImpl.EMPTY_INTERFACEOPERATIONMESSAGE;
R1020_is_a_OperationMessage_inst = OperationMessageImpl.EMPTY_OPERATIONMESSAGE;
}
public static SynchronousMessage create( Sql context ) throws XtumlException {
SynchronousMessage newSynchronousMessage = new SynchronousMessageImpl( context );
if ( context.addInstance( newSynchronousMessage ) ) {
newSynchronousMessage.getRunContext().addChange(new InstanceCreatedDelta(newSynchronousMessage, KEY_LETTERS));
return newSynchronousMessage;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static SynchronousMessage create( Sql context, UniqueId ref_Msg_ID, String m_InformalName, String m_Descrip, String m_GuardCondition, String m_ResultTarget, String m_ReturnValue, boolean m_isFormal, String m_Label, String m_SequenceNumb ) throws XtumlException {
return create(context, UniqueId.random(), ref_Msg_ID, m_InformalName, m_Descrip, m_GuardCondition, m_ResultTarget, m_ReturnValue, m_isFormal, m_Label, m_SequenceNumb);
}
public static SynchronousMessage create( Sql context, UniqueId instanceId, UniqueId ref_Msg_ID, String m_InformalName, String m_Descrip, String m_GuardCondition, String m_ResultTarget, String m_ReturnValue, boolean m_isFormal, String m_Label, String m_SequenceNumb ) throws XtumlException {
SynchronousMessage newSynchronousMessage = new SynchronousMessageImpl( context, instanceId, ref_Msg_ID, m_InformalName, m_Descrip, m_GuardCondition, m_ResultTarget, m_ReturnValue, m_isFormal, m_Label, m_SequenceNumb );
if ( context.addInstance( newSynchronousMessage ) ) {
return newSynchronousMessage;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId ref_Msg_ID;
@Override
public UniqueId getMsg_ID() throws XtumlException {
checkLiving();
return ref_Msg_ID;
}
@Override
public void setMsg_ID(UniqueId ref_Msg_ID) throws XtumlException {
checkLiving();
if (ref_Msg_ID.inequality( this.ref_Msg_ID)) {
final UniqueId oldValue = this.ref_Msg_ID;
this.ref_Msg_ID = ref_Msg_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Msg_ID", oldValue, this.ref_Msg_ID));
if ( !R1020_is_a_FunctionMessage().isEmpty() ) R1020_is_a_FunctionMessage().setMsg_ID( ref_Msg_ID );
if ( !R1020_is_a_InterfaceOperationMessage().isEmpty() ) R1020_is_a_InterfaceOperationMessage().setMsg_ID( ref_Msg_ID );
if ( !R1020_is_a_InformalSynchronousMessage().isEmpty() ) R1020_is_a_InformalSynchronousMessage().setMsg_ID( ref_Msg_ID );
if ( !R1020_is_a_OperationMessage().isEmpty() ) R1020_is_a_OperationMessage().setMsg_ID( ref_Msg_ID );
if ( !R1020_is_a_BridgeMessage().isEmpty() ) R1020_is_a_BridgeMessage().setMsg_ID( ref_Msg_ID );
}
}
private String m_InformalName;
@Override
public String getInformalName() throws XtumlException {
checkLiving();
return m_InformalName;
}
@Override
public void setInformalName(String m_InformalName) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_InformalName, this.m_InformalName)) {
final String oldValue = this.m_InformalName;
this.m_InformalName = m_InformalName;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_InformalName", oldValue, this.m_InformalName));
}
}
private String m_Descrip;
@Override
public String getDescrip() throws XtumlException {
checkLiving();
return m_Descrip;
}
@Override
public void setDescrip(String m_Descrip) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Descrip, this.m_Descrip)) {
final String oldValue = this.m_Descrip;
this.m_Descrip = m_Descrip;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Descrip", oldValue, this.m_Descrip));
}
}
private String m_GuardCondition;
@Override
public void setGuardCondition(String m_GuardCondition) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_GuardCondition, this.m_GuardCondition)) {
final String oldValue = this.m_GuardCondition;
this.m_GuardCondition = m_GuardCondition;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_GuardCondition", oldValue, this.m_GuardCondition));
}
}
@Override
public String getGuardCondition() throws XtumlException {
checkLiving();
return m_GuardCondition;
}
private String m_ResultTarget;
@Override
public String getResultTarget() throws XtumlException {
checkLiving();
return m_ResultTarget;
}
@Override
public void setResultTarget(String m_ResultTarget) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_ResultTarget, this.m_ResultTarget)) {
final String oldValue = this.m_ResultTarget;
this.m_ResultTarget = m_ResultTarget;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_ResultTarget", oldValue, this.m_ResultTarget));
}
}
private String m_ReturnValue;
@Override
public String getReturnValue() throws XtumlException {
checkLiving();
return m_ReturnValue;
}
@Override
public void setReturnValue(String m_ReturnValue) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_ReturnValue, this.m_ReturnValue)) {
final String oldValue = this.m_ReturnValue;
this.m_ReturnValue = m_ReturnValue;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_ReturnValue", oldValue, this.m_ReturnValue));
}
}
private boolean m_isFormal;
@Override
public void setIsFormal(boolean m_isFormal) throws XtumlException {
checkLiving();
if (m_isFormal != this.m_isFormal) {
final boolean oldValue = this.m_isFormal;
this.m_isFormal = m_isFormal;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_isFormal", oldValue, this.m_isFormal));
}
}
@Override
public boolean getIsFormal() throws XtumlException {
checkLiving();
return m_isFormal;
}
private String m_Label;
@Override
public String getLabel() throws XtumlException {
checkLiving();
return m_Label;
}
@Override
public void setLabel(String m_Label) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Label, this.m_Label)) {
final String oldValue = this.m_Label;
this.m_Label = m_Label;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Label", oldValue, this.m_Label));
}
}
private String m_SequenceNumb;
@Override
public void setSequenceNumb(String m_SequenceNumb) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_SequenceNumb, this.m_SequenceNumb)) {
final String oldValue = this.m_SequenceNumb;
this.m_SequenceNumb = m_SequenceNumb;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_SequenceNumb", oldValue, this.m_SequenceNumb));
}
}
@Override
public String getSequenceNumb() throws XtumlException {
checkLiving();
return m_SequenceNumb;
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getMsg_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private MSG_M R1018_is_a_MSG_M_inst;
@Override
public void setR1018_is_a_MSG_M( MSG_M inst ) {
R1018_is_a_MSG_M_inst = inst;
}
@Override
public MSG_M R1018_is_a_MSG_M() throws XtumlException {
return R1018_is_a_MSG_M_inst;
}
private BridgeMessage R1020_is_a_BridgeMessage_inst;
@Override
public void setR1020_is_a_BridgeMessage( BridgeMessage inst ) {
R1020_is_a_BridgeMessage_inst = inst;
}
@Override
public BridgeMessage R1020_is_a_BridgeMessage() throws XtumlException {
return R1020_is_a_BridgeMessage_inst;
}
private FunctionMessage R1020_is_a_FunctionMessage_inst;
@Override
public void setR1020_is_a_FunctionMessage( FunctionMessage inst ) {
R1020_is_a_FunctionMessage_inst = inst;
}
@Override
public FunctionMessage R1020_is_a_FunctionMessage() throws XtumlException {
return R1020_is_a_FunctionMessage_inst;
}
private InformalSynchronousMessage R1020_is_a_InformalSynchronousMessage_inst;
@Override
public void setR1020_is_a_InformalSynchronousMessage( InformalSynchronousMessage inst ) {
R1020_is_a_InformalSynchronousMessage_inst = inst;
}
@Override
public InformalSynchronousMessage R1020_is_a_InformalSynchronousMessage() throws XtumlException {
return R1020_is_a_InformalSynchronousMessage_inst;
}
private InterfaceOperationMessage R1020_is_a_InterfaceOperationMessage_inst;
@Override
public void setR1020_is_a_InterfaceOperationMessage( InterfaceOperationMessage inst ) {
R1020_is_a_InterfaceOperationMessage_inst = inst;
}
@Override
public InterfaceOperationMessage R1020_is_a_InterfaceOperationMessage() throws XtumlException {
return R1020_is_a_InterfaceOperationMessage_inst;
}
private OperationMessage R1020_is_a_OperationMessage_inst;
@Override
public void setR1020_is_a_OperationMessage( OperationMessage inst ) {
R1020_is_a_OperationMessage_inst = inst;
}
@Override
public OperationMessage R1020_is_a_OperationMessage() throws XtumlException {
return R1020_is_a_OperationMessage_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public SynchronousMessage self() {
return this;
}
@Override
public SynchronousMessage oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_SYNCHRONOUSMESSAGE;
}
}
class EmptySynchronousMessage extends ModelInstance implements SynchronousMessage {
// attributes
public UniqueId getMsg_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setMsg_ID( UniqueId ref_Msg_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getInformalName() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setInformalName( String m_InformalName ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getDescrip() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setDescrip( String m_Descrip ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setGuardCondition( String m_GuardCondition ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getGuardCondition() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getResultTarget() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setResultTarget( String m_ResultTarget ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getReturnValue() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setReturnValue( String m_ReturnValue ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setIsFormal( boolean m_isFormal ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public boolean getIsFormal() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getLabel() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setLabel( String m_Label ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setSequenceNumb( String m_SequenceNumb ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getSequenceNumb() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
// operations
// selections
@Override
public MSG_M R1018_is_a_MSG_M() {
return MSG_MImpl.EMPTY_MSG_M;
}
@Override
public BridgeMessage R1020_is_a_BridgeMessage() {
return BridgeMessageImpl.EMPTY_BRIDGEMESSAGE;
}
@Override
public FunctionMessage R1020_is_a_FunctionMessage() {
return FunctionMessageImpl.EMPTY_FUNCTIONMESSAGE;
}
@Override
public InformalSynchronousMessage R1020_is_a_InformalSynchronousMessage() {
return InformalSynchronousMessageImpl.EMPTY_INFORMALSYNCHRONOUSMESSAGE;
}
@Override
public InterfaceOperationMessage R1020_is_a_InterfaceOperationMessage() {
return InterfaceOperationMessageImpl.EMPTY_INTERFACEOPERATIONMESSAGE;
}
@Override
public OperationMessage R1020_is_a_OperationMessage() {
return OperationMessageImpl.EMPTY_OPERATIONMESSAGE;
}
@Override
public String getKeyLetters() {
return SynchronousMessageImpl.KEY_LETTERS;
}
@Override
public SynchronousMessage self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public SynchronousMessage oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return SynchronousMessageImpl.EMPTY_SYNCHRONOUSMESSAGE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy