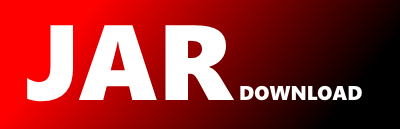
io.ciera.tool.sql.ooaofooa.relateandunrelate.impl.RelateUsingImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.relateandunrelate.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.association.Association;
import io.ciera.tool.sql.ooaofooa.association.impl.AssociationImpl;
import io.ciera.tool.sql.ooaofooa.body.ACT_SMT;
import io.ciera.tool.sql.ooaofooa.body.impl.ACT_SMTImpl;
import io.ciera.tool.sql.ooaofooa.relateandunrelate.RelateUsing;
import io.ciera.tool.sql.ooaofooa.value.V_VAR;
import io.ciera.tool.sql.ooaofooa.value.impl.V_VARImpl;
public class RelateUsingImpl extends ModelInstance implements RelateUsing {
public static final String KEY_LETTERS = "ACT_RU";
public static final RelateUsing EMPTY_RELATEUSING = new EmptyRelateUsing();
private Sql context;
// constructors
private RelateUsingImpl( Sql context ) {
this.context = context;
ref_Statement_ID = UniqueId.random();
ref_One_Side_Var_ID = UniqueId.random();
ref_Other_Side_Var_ID = UniqueId.random();
ref_Associative_Var_ID = UniqueId.random();
m_relationship_phrase = "";
ref_Rel_ID = UniqueId.random();
m_associationNumberLineNumber = 0;
m_associationNumberColumn = 0;
m_associationPhraseLineNumber = 0;
m_associationPhraseColumn = 0;
R603_is_a_ACT_SMT_inst = ACT_SMTImpl.EMPTY_ACT_SMT;
R617_one_V_VAR_inst = V_VARImpl.EMPTY_V_VAR;
R618_other_V_VAR_inst = V_VARImpl.EMPTY_V_VAR;
R619_using_V_VAR_inst = V_VARImpl.EMPTY_V_VAR;
R654_creates_Association_inst = AssociationImpl.EMPTY_ASSOCIATION;
}
private RelateUsingImpl( Sql context, UniqueId instanceId, UniqueId ref_Statement_ID, UniqueId ref_One_Side_Var_ID, UniqueId ref_Other_Side_Var_ID, UniqueId ref_Associative_Var_ID, String m_relationship_phrase, UniqueId ref_Rel_ID, int m_associationNumberLineNumber, int m_associationNumberColumn, int m_associationPhraseLineNumber, int m_associationPhraseColumn ) {
super(instanceId);
this.context = context;
this.ref_Statement_ID = ref_Statement_ID;
this.ref_One_Side_Var_ID = ref_One_Side_Var_ID;
this.ref_Other_Side_Var_ID = ref_Other_Side_Var_ID;
this.ref_Associative_Var_ID = ref_Associative_Var_ID;
this.m_relationship_phrase = m_relationship_phrase;
this.ref_Rel_ID = ref_Rel_ID;
this.m_associationNumberLineNumber = m_associationNumberLineNumber;
this.m_associationNumberColumn = m_associationNumberColumn;
this.m_associationPhraseLineNumber = m_associationPhraseLineNumber;
this.m_associationPhraseColumn = m_associationPhraseColumn;
R603_is_a_ACT_SMT_inst = ACT_SMTImpl.EMPTY_ACT_SMT;
R617_one_V_VAR_inst = V_VARImpl.EMPTY_V_VAR;
R618_other_V_VAR_inst = V_VARImpl.EMPTY_V_VAR;
R619_using_V_VAR_inst = V_VARImpl.EMPTY_V_VAR;
R654_creates_Association_inst = AssociationImpl.EMPTY_ASSOCIATION;
}
public static RelateUsing create( Sql context ) throws XtumlException {
RelateUsing newRelateUsing = new RelateUsingImpl( context );
if ( context.addInstance( newRelateUsing ) ) {
newRelateUsing.getRunContext().addChange(new InstanceCreatedDelta(newRelateUsing, KEY_LETTERS));
return newRelateUsing;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static RelateUsing create( Sql context, UniqueId ref_Statement_ID, UniqueId ref_One_Side_Var_ID, UniqueId ref_Other_Side_Var_ID, UniqueId ref_Associative_Var_ID, String m_relationship_phrase, UniqueId ref_Rel_ID, int m_associationNumberLineNumber, int m_associationNumberColumn, int m_associationPhraseLineNumber, int m_associationPhraseColumn ) throws XtumlException {
return create(context, UniqueId.random(), ref_Statement_ID, ref_One_Side_Var_ID, ref_Other_Side_Var_ID, ref_Associative_Var_ID, m_relationship_phrase, ref_Rel_ID, m_associationNumberLineNumber, m_associationNumberColumn, m_associationPhraseLineNumber, m_associationPhraseColumn);
}
public static RelateUsing create( Sql context, UniqueId instanceId, UniqueId ref_Statement_ID, UniqueId ref_One_Side_Var_ID, UniqueId ref_Other_Side_Var_ID, UniqueId ref_Associative_Var_ID, String m_relationship_phrase, UniqueId ref_Rel_ID, int m_associationNumberLineNumber, int m_associationNumberColumn, int m_associationPhraseLineNumber, int m_associationPhraseColumn ) throws XtumlException {
RelateUsing newRelateUsing = new RelateUsingImpl( context, instanceId, ref_Statement_ID, ref_One_Side_Var_ID, ref_Other_Side_Var_ID, ref_Associative_Var_ID, m_relationship_phrase, ref_Rel_ID, m_associationNumberLineNumber, m_associationNumberColumn, m_associationPhraseLineNumber, m_associationPhraseColumn );
if ( context.addInstance( newRelateUsing ) ) {
return newRelateUsing;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId ref_Statement_ID;
@Override
public void setStatement_ID(UniqueId ref_Statement_ID) throws XtumlException {
checkLiving();
if (ref_Statement_ID.inequality( this.ref_Statement_ID)) {
final UniqueId oldValue = this.ref_Statement_ID;
this.ref_Statement_ID = ref_Statement_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Statement_ID", oldValue, this.ref_Statement_ID));
}
}
@Override
public UniqueId getStatement_ID() throws XtumlException {
checkLiving();
return ref_Statement_ID;
}
private UniqueId ref_One_Side_Var_ID;
@Override
public UniqueId getOne_Side_Var_ID() throws XtumlException {
checkLiving();
return ref_One_Side_Var_ID;
}
@Override
public void setOne_Side_Var_ID(UniqueId ref_One_Side_Var_ID) throws XtumlException {
checkLiving();
if (ref_One_Side_Var_ID.inequality( this.ref_One_Side_Var_ID)) {
final UniqueId oldValue = this.ref_One_Side_Var_ID;
this.ref_One_Side_Var_ID = ref_One_Side_Var_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_One_Side_Var_ID", oldValue, this.ref_One_Side_Var_ID));
}
}
private UniqueId ref_Other_Side_Var_ID;
@Override
public UniqueId getOther_Side_Var_ID() throws XtumlException {
checkLiving();
return ref_Other_Side_Var_ID;
}
@Override
public void setOther_Side_Var_ID(UniqueId ref_Other_Side_Var_ID) throws XtumlException {
checkLiving();
if (ref_Other_Side_Var_ID.inequality( this.ref_Other_Side_Var_ID)) {
final UniqueId oldValue = this.ref_Other_Side_Var_ID;
this.ref_Other_Side_Var_ID = ref_Other_Side_Var_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Other_Side_Var_ID", oldValue, this.ref_Other_Side_Var_ID));
}
}
private UniqueId ref_Associative_Var_ID;
@Override
public void setAssociative_Var_ID(UniqueId ref_Associative_Var_ID) throws XtumlException {
checkLiving();
if (ref_Associative_Var_ID.inequality( this.ref_Associative_Var_ID)) {
final UniqueId oldValue = this.ref_Associative_Var_ID;
this.ref_Associative_Var_ID = ref_Associative_Var_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Associative_Var_ID", oldValue, this.ref_Associative_Var_ID));
}
}
@Override
public UniqueId getAssociative_Var_ID() throws XtumlException {
checkLiving();
return ref_Associative_Var_ID;
}
private String m_relationship_phrase;
@Override
public void setRelationship_phrase(String m_relationship_phrase) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_relationship_phrase, this.m_relationship_phrase)) {
final String oldValue = this.m_relationship_phrase;
this.m_relationship_phrase = m_relationship_phrase;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_relationship_phrase", oldValue, this.m_relationship_phrase));
}
}
@Override
public String getRelationship_phrase() throws XtumlException {
checkLiving();
return m_relationship_phrase;
}
private UniqueId ref_Rel_ID;
@Override
public void setRel_ID(UniqueId ref_Rel_ID) throws XtumlException {
checkLiving();
if (ref_Rel_ID.inequality( this.ref_Rel_ID)) {
final UniqueId oldValue = this.ref_Rel_ID;
this.ref_Rel_ID = ref_Rel_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Rel_ID", oldValue, this.ref_Rel_ID));
}
}
@Override
public UniqueId getRel_ID() throws XtumlException {
checkLiving();
return ref_Rel_ID;
}
private int m_associationNumberLineNumber;
@Override
public void setAssociationNumberLineNumber(int m_associationNumberLineNumber) throws XtumlException {
checkLiving();
if (m_associationNumberLineNumber != this.m_associationNumberLineNumber) {
final int oldValue = this.m_associationNumberLineNumber;
this.m_associationNumberLineNumber = m_associationNumberLineNumber;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_associationNumberLineNumber", oldValue, this.m_associationNumberLineNumber));
}
}
@Override
public int getAssociationNumberLineNumber() throws XtumlException {
checkLiving();
return m_associationNumberLineNumber;
}
private int m_associationNumberColumn;
@Override
public void setAssociationNumberColumn(int m_associationNumberColumn) throws XtumlException {
checkLiving();
if (m_associationNumberColumn != this.m_associationNumberColumn) {
final int oldValue = this.m_associationNumberColumn;
this.m_associationNumberColumn = m_associationNumberColumn;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_associationNumberColumn", oldValue, this.m_associationNumberColumn));
}
}
@Override
public int getAssociationNumberColumn() throws XtumlException {
checkLiving();
return m_associationNumberColumn;
}
private int m_associationPhraseLineNumber;
@Override
public void setAssociationPhraseLineNumber(int m_associationPhraseLineNumber) throws XtumlException {
checkLiving();
if (m_associationPhraseLineNumber != this.m_associationPhraseLineNumber) {
final int oldValue = this.m_associationPhraseLineNumber;
this.m_associationPhraseLineNumber = m_associationPhraseLineNumber;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_associationPhraseLineNumber", oldValue, this.m_associationPhraseLineNumber));
}
}
@Override
public int getAssociationPhraseLineNumber() throws XtumlException {
checkLiving();
return m_associationPhraseLineNumber;
}
private int m_associationPhraseColumn;
@Override
public void setAssociationPhraseColumn(int m_associationPhraseColumn) throws XtumlException {
checkLiving();
if (m_associationPhraseColumn != this.m_associationPhraseColumn) {
final int oldValue = this.m_associationPhraseColumn;
this.m_associationPhraseColumn = m_associationPhraseColumn;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_associationPhraseColumn", oldValue, this.m_associationPhraseColumn));
}
}
@Override
public int getAssociationPhraseColumn() throws XtumlException {
checkLiving();
return m_associationPhraseColumn;
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getStatement_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private ACT_SMT R603_is_a_ACT_SMT_inst;
@Override
public void setR603_is_a_ACT_SMT( ACT_SMT inst ) {
R603_is_a_ACT_SMT_inst = inst;
}
@Override
public ACT_SMT R603_is_a_ACT_SMT() throws XtumlException {
return R603_is_a_ACT_SMT_inst;
}
private V_VAR R617_one_V_VAR_inst;
@Override
public void setR617_one_V_VAR( V_VAR inst ) {
R617_one_V_VAR_inst = inst;
}
@Override
public V_VAR R617_one_V_VAR() throws XtumlException {
return R617_one_V_VAR_inst;
}
private V_VAR R618_other_V_VAR_inst;
@Override
public void setR618_other_V_VAR( V_VAR inst ) {
R618_other_V_VAR_inst = inst;
}
@Override
public V_VAR R618_other_V_VAR() throws XtumlException {
return R618_other_V_VAR_inst;
}
private V_VAR R619_using_V_VAR_inst;
@Override
public void setR619_using_V_VAR( V_VAR inst ) {
R619_using_V_VAR_inst = inst;
}
@Override
public V_VAR R619_using_V_VAR() throws XtumlException {
return R619_using_V_VAR_inst;
}
private Association R654_creates_Association_inst;
@Override
public void setR654_creates_Association( Association inst ) {
R654_creates_Association_inst = inst;
}
@Override
public Association R654_creates_Association() throws XtumlException {
return R654_creates_Association_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public RelateUsing self() {
return this;
}
@Override
public RelateUsing oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_RELATEUSING;
}
}
class EmptyRelateUsing extends ModelInstance implements RelateUsing {
// attributes
public void setStatement_ID( UniqueId ref_Statement_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getStatement_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public UniqueId getOne_Side_Var_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setOne_Side_Var_ID( UniqueId ref_One_Side_Var_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getOther_Side_Var_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setOther_Side_Var_ID( UniqueId ref_Other_Side_Var_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setAssociative_Var_ID( UniqueId ref_Associative_Var_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getAssociative_Var_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setRelationship_phrase( String m_relationship_phrase ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getRelationship_phrase() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setRel_ID( UniqueId ref_Rel_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getRel_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setAssociationNumberLineNumber( int m_associationNumberLineNumber ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getAssociationNumberLineNumber() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setAssociationNumberColumn( int m_associationNumberColumn ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getAssociationNumberColumn() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setAssociationPhraseLineNumber( int m_associationPhraseLineNumber ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getAssociationPhraseLineNumber() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setAssociationPhraseColumn( int m_associationPhraseColumn ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getAssociationPhraseColumn() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
// operations
// selections
@Override
public ACT_SMT R603_is_a_ACT_SMT() {
return ACT_SMTImpl.EMPTY_ACT_SMT;
}
@Override
public V_VAR R617_one_V_VAR() {
return V_VARImpl.EMPTY_V_VAR;
}
@Override
public V_VAR R618_other_V_VAR() {
return V_VARImpl.EMPTY_V_VAR;
}
@Override
public V_VAR R619_using_V_VAR() {
return V_VARImpl.EMPTY_V_VAR;
}
@Override
public Association R654_creates_Association() {
return AssociationImpl.EMPTY_ASSOCIATION;
}
@Override
public String getKeyLetters() {
return RelateUsingImpl.KEY_LETTERS;
}
@Override
public RelateUsing self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public RelateUsing oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return RelateUsingImpl.EMPTY_RELATEUSING;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy