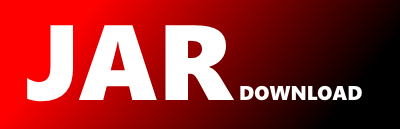
io.ciera.tool.sql.ooaofooa.statemachine.impl.SM_SMImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.statemachine.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.statemachine.Action;
import io.ciera.tool.sql.ooaofooa.statemachine.ActionSet;
import io.ciera.tool.sql.ooaofooa.statemachine.ClassStateMachine;
import io.ciera.tool.sql.ooaofooa.statemachine.InstanceStateMachine;
import io.ciera.tool.sql.ooaofooa.statemachine.MealyStateMachine;
import io.ciera.tool.sql.ooaofooa.statemachine.MooreStateMachine;
import io.ciera.tool.sql.ooaofooa.statemachine.SM_SM;
import io.ciera.tool.sql.ooaofooa.statemachine.StateMachineEvent;
import io.ciera.tool.sql.ooaofooa.statemachine.StateMachineEventDataItem;
import io.ciera.tool.sql.ooaofooa.statemachine.StateMachineEventDataItemSet;
import io.ciera.tool.sql.ooaofooa.statemachine.StateMachineEventSet;
import io.ciera.tool.sql.ooaofooa.statemachine.StateMachineState;
import io.ciera.tool.sql.ooaofooa.statemachine.StateMachineStateSet;
import io.ciera.tool.sql.ooaofooa.statemachine.Transition;
import io.ciera.tool.sql.ooaofooa.statemachine.TransitionSet;
import io.ciera.tool.sql.ooaofooa.statemachine.impl.ActionSetImpl;
import io.ciera.tool.sql.ooaofooa.statemachine.impl.ClassStateMachineImpl;
import io.ciera.tool.sql.ooaofooa.statemachine.impl.InstanceStateMachineImpl;
import io.ciera.tool.sql.ooaofooa.statemachine.impl.MealyStateMachineImpl;
import io.ciera.tool.sql.ooaofooa.statemachine.impl.MooreStateMachineImpl;
import io.ciera.tool.sql.ooaofooa.statemachine.impl.StateMachineEventDataItemSetImpl;
import io.ciera.tool.sql.ooaofooa.statemachine.impl.StateMachineEventSetImpl;
import io.ciera.tool.sql.ooaofooa.statemachine.impl.StateMachineStateSetImpl;
import io.ciera.tool.sql.ooaofooa.statemachine.impl.TransitionSetImpl;
public class SM_SMImpl extends ModelInstance implements SM_SM {
public static final String KEY_LETTERS = "SM_SM";
public static final SM_SM EMPTY_SM_SM = new EmptySM_SM();
private Sql context;
// constructors
private SM_SMImpl( Sql context ) {
this.context = context;
m_SM_ID = UniqueId.random();
m_Descrip = "";
m_Config_ID = 0;
R501_is_decomposed_into_StateMachineState_set = new StateMachineStateSetImpl();
R502_can_be_communicated_to_via_StateMachineEvent_set = new StateMachineEventSetImpl();
R505_contains_Transition_set = new TransitionSetImpl();
R510_is_a_MealyStateMachine_inst = MealyStateMachineImpl.EMPTY_MEALYSTATEMACHINE;
R510_is_a_MooreStateMachine_inst = MooreStateMachineImpl.EMPTY_MOORESTATEMACHINE;
R515_contains_Action_set = new ActionSetImpl();
R516_can_asynchronously_communicate_via_StateMachineEventDataItem_set = new StateMachineEventDataItemSetImpl();
R517_is_a_ClassStateMachine_inst = ClassStateMachineImpl.EMPTY_CLASSSTATEMACHINE;
R517_is_a_InstanceStateMachine_inst = InstanceStateMachineImpl.EMPTY_INSTANCESTATEMACHINE;
}
private SM_SMImpl( Sql context, UniqueId instanceId, UniqueId m_SM_ID, String m_Descrip, int m_Config_ID ) {
super(instanceId);
this.context = context;
this.m_SM_ID = m_SM_ID;
this.m_Descrip = m_Descrip;
this.m_Config_ID = m_Config_ID;
R501_is_decomposed_into_StateMachineState_set = new StateMachineStateSetImpl();
R502_can_be_communicated_to_via_StateMachineEvent_set = new StateMachineEventSetImpl();
R505_contains_Transition_set = new TransitionSetImpl();
R510_is_a_MealyStateMachine_inst = MealyStateMachineImpl.EMPTY_MEALYSTATEMACHINE;
R510_is_a_MooreStateMachine_inst = MooreStateMachineImpl.EMPTY_MOORESTATEMACHINE;
R515_contains_Action_set = new ActionSetImpl();
R516_can_asynchronously_communicate_via_StateMachineEventDataItem_set = new StateMachineEventDataItemSetImpl();
R517_is_a_ClassStateMachine_inst = ClassStateMachineImpl.EMPTY_CLASSSTATEMACHINE;
R517_is_a_InstanceStateMachine_inst = InstanceStateMachineImpl.EMPTY_INSTANCESTATEMACHINE;
}
public static SM_SM create( Sql context ) throws XtumlException {
SM_SM newSM_SM = new SM_SMImpl( context );
if ( context.addInstance( newSM_SM ) ) {
newSM_SM.getRunContext().addChange(new InstanceCreatedDelta(newSM_SM, KEY_LETTERS));
return newSM_SM;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static SM_SM create( Sql context, UniqueId m_SM_ID, String m_Descrip, int m_Config_ID ) throws XtumlException {
return create(context, UniqueId.random(), m_SM_ID, m_Descrip, m_Config_ID);
}
public static SM_SM create( Sql context, UniqueId instanceId, UniqueId m_SM_ID, String m_Descrip, int m_Config_ID ) throws XtumlException {
SM_SM newSM_SM = new SM_SMImpl( context, instanceId, m_SM_ID, m_Descrip, m_Config_ID );
if ( context.addInstance( newSM_SM ) ) {
return newSM_SM;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId m_SM_ID;
@Override
public void setSM_ID(UniqueId m_SM_ID) throws XtumlException {
checkLiving();
if (m_SM_ID.inequality( this.m_SM_ID)) {
final UniqueId oldValue = this.m_SM_ID;
this.m_SM_ID = m_SM_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_SM_ID", oldValue, this.m_SM_ID));
if ( !R517_is_a_ClassStateMachine().isEmpty() ) R517_is_a_ClassStateMachine().setSM_ID( m_SM_ID );
if ( !R510_is_a_MooreStateMachine().isEmpty() ) R510_is_a_MooreStateMachine().setSM_ID( m_SM_ID );
if ( !R516_can_asynchronously_communicate_via_StateMachineEventDataItem().isEmpty() ) R516_can_asynchronously_communicate_via_StateMachineEventDataItem().setSM_ID( m_SM_ID );
if ( !R510_is_a_MealyStateMachine().isEmpty() ) R510_is_a_MealyStateMachine().setSM_ID( m_SM_ID );
if ( !R502_can_be_communicated_to_via_StateMachineEvent().isEmpty() ) R502_can_be_communicated_to_via_StateMachineEvent().setSM_ID( m_SM_ID );
if ( !R515_contains_Action().isEmpty() ) R515_contains_Action().setSM_ID( m_SM_ID );
if ( !R505_contains_Transition().isEmpty() ) R505_contains_Transition().setSM_ID( m_SM_ID );
if ( !R517_is_a_InstanceStateMachine().isEmpty() ) R517_is_a_InstanceStateMachine().setSM_ID( m_SM_ID );
if ( !R501_is_decomposed_into_StateMachineState().isEmpty() ) R501_is_decomposed_into_StateMachineState().setSM_ID( m_SM_ID );
}
}
@Override
public UniqueId getSM_ID() throws XtumlException {
checkLiving();
return m_SM_ID;
}
private String m_Descrip;
@Override
public void setDescrip(String m_Descrip) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Descrip, this.m_Descrip)) {
final String oldValue = this.m_Descrip;
this.m_Descrip = m_Descrip;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Descrip", oldValue, this.m_Descrip));
}
}
@Override
public String getDescrip() throws XtumlException {
checkLiving();
return m_Descrip;
}
private int m_Config_ID;
@Override
public void setConfig_ID(int m_Config_ID) throws XtumlException {
checkLiving();
if (m_Config_ID != this.m_Config_ID) {
final int oldValue = this.m_Config_ID;
this.m_Config_ID = m_Config_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Config_ID", oldValue, this.m_Config_ID));
}
}
@Override
public int getConfig_ID() throws XtumlException {
checkLiving();
return m_Config_ID;
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getSM_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private StateMachineStateSet R501_is_decomposed_into_StateMachineState_set;
@Override
public void addR501_is_decomposed_into_StateMachineState( StateMachineState inst ) {
R501_is_decomposed_into_StateMachineState_set.add(inst);
}
@Override
public void removeR501_is_decomposed_into_StateMachineState( StateMachineState inst ) {
R501_is_decomposed_into_StateMachineState_set.remove(inst);
}
@Override
public StateMachineStateSet R501_is_decomposed_into_StateMachineState() throws XtumlException {
return R501_is_decomposed_into_StateMachineState_set;
}
private StateMachineEventSet R502_can_be_communicated_to_via_StateMachineEvent_set;
@Override
public void addR502_can_be_communicated_to_via_StateMachineEvent( StateMachineEvent inst ) {
R502_can_be_communicated_to_via_StateMachineEvent_set.add(inst);
}
@Override
public void removeR502_can_be_communicated_to_via_StateMachineEvent( StateMachineEvent inst ) {
R502_can_be_communicated_to_via_StateMachineEvent_set.remove(inst);
}
@Override
public StateMachineEventSet R502_can_be_communicated_to_via_StateMachineEvent() throws XtumlException {
return R502_can_be_communicated_to_via_StateMachineEvent_set;
}
private TransitionSet R505_contains_Transition_set;
@Override
public void addR505_contains_Transition( Transition inst ) {
R505_contains_Transition_set.add(inst);
}
@Override
public void removeR505_contains_Transition( Transition inst ) {
R505_contains_Transition_set.remove(inst);
}
@Override
public TransitionSet R505_contains_Transition() throws XtumlException {
return R505_contains_Transition_set;
}
private MealyStateMachine R510_is_a_MealyStateMachine_inst;
@Override
public void setR510_is_a_MealyStateMachine( MealyStateMachine inst ) {
R510_is_a_MealyStateMachine_inst = inst;
}
@Override
public MealyStateMachine R510_is_a_MealyStateMachine() throws XtumlException {
return R510_is_a_MealyStateMachine_inst;
}
private MooreStateMachine R510_is_a_MooreStateMachine_inst;
@Override
public void setR510_is_a_MooreStateMachine( MooreStateMachine inst ) {
R510_is_a_MooreStateMachine_inst = inst;
}
@Override
public MooreStateMachine R510_is_a_MooreStateMachine() throws XtumlException {
return R510_is_a_MooreStateMachine_inst;
}
private ActionSet R515_contains_Action_set;
@Override
public void addR515_contains_Action( Action inst ) {
R515_contains_Action_set.add(inst);
}
@Override
public void removeR515_contains_Action( Action inst ) {
R515_contains_Action_set.remove(inst);
}
@Override
public ActionSet R515_contains_Action() throws XtumlException {
return R515_contains_Action_set;
}
private StateMachineEventDataItemSet R516_can_asynchronously_communicate_via_StateMachineEventDataItem_set;
@Override
public void addR516_can_asynchronously_communicate_via_StateMachineEventDataItem( StateMachineEventDataItem inst ) {
R516_can_asynchronously_communicate_via_StateMachineEventDataItem_set.add(inst);
}
@Override
public void removeR516_can_asynchronously_communicate_via_StateMachineEventDataItem( StateMachineEventDataItem inst ) {
R516_can_asynchronously_communicate_via_StateMachineEventDataItem_set.remove(inst);
}
@Override
public StateMachineEventDataItemSet R516_can_asynchronously_communicate_via_StateMachineEventDataItem() throws XtumlException {
return R516_can_asynchronously_communicate_via_StateMachineEventDataItem_set;
}
private ClassStateMachine R517_is_a_ClassStateMachine_inst;
@Override
public void setR517_is_a_ClassStateMachine( ClassStateMachine inst ) {
R517_is_a_ClassStateMachine_inst = inst;
}
@Override
public ClassStateMachine R517_is_a_ClassStateMachine() throws XtumlException {
return R517_is_a_ClassStateMachine_inst;
}
private InstanceStateMachine R517_is_a_InstanceStateMachine_inst;
@Override
public void setR517_is_a_InstanceStateMachine( InstanceStateMachine inst ) {
R517_is_a_InstanceStateMachine_inst = inst;
}
@Override
public InstanceStateMachine R517_is_a_InstanceStateMachine() throws XtumlException {
return R517_is_a_InstanceStateMachine_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public SM_SM self() {
return this;
}
@Override
public SM_SM oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_SM_SM;
}
}
class EmptySM_SM extends ModelInstance implements SM_SM {
// attributes
public void setSM_ID( UniqueId m_SM_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getSM_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setDescrip( String m_Descrip ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getDescrip() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setConfig_ID( int m_Config_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getConfig_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
// operations
// selections
@Override
public StateMachineStateSet R501_is_decomposed_into_StateMachineState() {
return (new StateMachineStateSetImpl());
}
@Override
public StateMachineEventSet R502_can_be_communicated_to_via_StateMachineEvent() {
return (new StateMachineEventSetImpl());
}
@Override
public TransitionSet R505_contains_Transition() {
return (new TransitionSetImpl());
}
@Override
public MealyStateMachine R510_is_a_MealyStateMachine() {
return MealyStateMachineImpl.EMPTY_MEALYSTATEMACHINE;
}
@Override
public MooreStateMachine R510_is_a_MooreStateMachine() {
return MooreStateMachineImpl.EMPTY_MOORESTATEMACHINE;
}
@Override
public ActionSet R515_contains_Action() {
return (new ActionSetImpl());
}
@Override
public StateMachineEventDataItemSet R516_can_asynchronously_communicate_via_StateMachineEventDataItem() {
return (new StateMachineEventDataItemSetImpl());
}
@Override
public ClassStateMachine R517_is_a_ClassStateMachine() {
return ClassStateMachineImpl.EMPTY_CLASSSTATEMACHINE;
}
@Override
public InstanceStateMachine R517_is_a_InstanceStateMachine() {
return InstanceStateMachineImpl.EMPTY_INSTANCESTATEMACHINE;
}
@Override
public String getKeyLetters() {
return SM_SMImpl.KEY_LETTERS;
}
@Override
public SM_SM self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public SM_SM oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return SM_SMImpl.EMPTY_SM_SM;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy