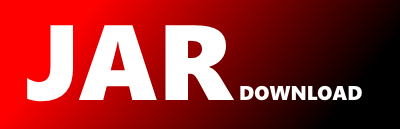
io.ciera.tool.sql.ooaofooa.subsystem.impl.O_ATTRImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.subsystem.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.architecture.classes.Attribute;
import io.ciera.tool.sql.architecture.classes.impl.AttributeImpl;
import io.ciera.tool.sql.ooaofooa.domain.DataType;
import io.ciera.tool.sql.ooaofooa.domain.Dimensions;
import io.ciera.tool.sql.ooaofooa.domain.DimensionsSet;
import io.ciera.tool.sql.ooaofooa.domain.impl.DataTypeImpl;
import io.ciera.tool.sql.ooaofooa.domain.impl.DimensionsSetImpl;
import io.ciera.tool.sql.ooaofooa.instance.AttributeValue;
import io.ciera.tool.sql.ooaofooa.instance.AttributeValueSet;
import io.ciera.tool.sql.ooaofooa.instance.impl.AttributeValueSetImpl;
import io.ciera.tool.sql.ooaofooa.interaction.InstanceAttributeValue;
import io.ciera.tool.sql.ooaofooa.interaction.InstanceAttributeValueSet;
import io.ciera.tool.sql.ooaofooa.interaction.impl.InstanceAttributeValueSetImpl;
import io.ciera.tool.sql.ooaofooa.subsystem.BaseAttribute;
import io.ciera.tool.sql.ooaofooa.subsystem.ClassIdentifierAttribute;
import io.ciera.tool.sql.ooaofooa.subsystem.ClassIdentifierAttributeSet;
import io.ciera.tool.sql.ooaofooa.subsystem.ModelClass;
import io.ciera.tool.sql.ooaofooa.subsystem.O_ATTR;
import io.ciera.tool.sql.ooaofooa.subsystem.ReferentialAttribute;
import io.ciera.tool.sql.ooaofooa.subsystem.impl.BaseAttributeImpl;
import io.ciera.tool.sql.ooaofooa.subsystem.impl.ClassIdentifierAttributeSetImpl;
import io.ciera.tool.sql.ooaofooa.subsystem.impl.ModelClassImpl;
import io.ciera.tool.sql.ooaofooa.subsystem.impl.O_ATTRImpl;
import io.ciera.tool.sql.ooaofooa.subsystem.impl.ReferentialAttributeImpl;
import io.ciera.tool.sql.ooaofooa.value.AttributeValueReference;
import io.ciera.tool.sql.ooaofooa.value.AttributeValueReferenceSet;
import io.ciera.tool.sql.ooaofooa.value.SelectedReference;
import io.ciera.tool.sql.ooaofooa.value.SelectedReferenceSet;
import io.ciera.tool.sql.ooaofooa.value.impl.AttributeValueReferenceSetImpl;
import io.ciera.tool.sql.ooaofooa.value.impl.SelectedReferenceSetImpl;
public class O_ATTRImpl extends ModelInstance implements O_ATTR {
public static final String KEY_LETTERS = "O_ATTR";
public static final O_ATTR EMPTY_O_ATTR = new EmptyO_ATTR();
private Sql context;
// constructors
private O_ATTRImpl( Sql context ) {
this.context = context;
m_Attr_ID = UniqueId.random();
ref_Obj_ID = UniqueId.random();
ref_PAttr_ID = UniqueId.random();
m_Name = "";
m_Descrip = "";
m_Prefix = "";
m_Root_Nam = "";
m_Pfx_Mode = 0;
ref_DT_ID = UniqueId.random();
m_Dimensions = "";
m_DefaultValue = "";
R102_abstracts_characteristics_of_ModelClass_inst = ModelClassImpl.EMPTY_MODELCLASS;
R103_precedes_O_ATTR_inst = O_ATTRImpl.EMPTY_O_ATTR;
R103_succeeds_O_ATTR_inst = O_ATTRImpl.EMPTY_O_ATTR;
R105_is_part_of__ClassIdentifierAttribute_set = new ClassIdentifierAttributeSetImpl();
R106_is_a_BaseAttribute_inst = BaseAttributeImpl.EMPTY_BASEATTRIBUTE;
R106_is_a_ReferentialAttribute_inst = ReferentialAttributeImpl.EMPTY_REFERENTIALATTRIBUTE;
R114_defines_type_of_DataType_inst = DataTypeImpl.EMPTY_DATATYPE;
R120_may_have_Dimensions_set = new DimensionsSetImpl();
R2910_has_instances_AttributeValue_set = new AttributeValueSetImpl();
R414_Attribute_inst = AttributeImpl.EMPTY_ATTRIBUTE;
R806_AttributeValueReference_set = new AttributeValueReferenceSetImpl();
R812_SelectedReference_set = new SelectedReferenceSetImpl();
R938_defines_InstanceAttributeValue_set = new InstanceAttributeValueSetImpl();
}
private O_ATTRImpl( Sql context, UniqueId instanceId, UniqueId m_Attr_ID, UniqueId ref_Obj_ID, UniqueId ref_PAttr_ID, String m_Name, String m_Descrip, String m_Prefix, String m_Root_Nam, int m_Pfx_Mode, UniqueId ref_DT_ID, String m_Dimensions, String m_DefaultValue ) {
super(instanceId);
this.context = context;
this.m_Attr_ID = m_Attr_ID;
this.ref_Obj_ID = ref_Obj_ID;
this.ref_PAttr_ID = ref_PAttr_ID;
this.m_Name = m_Name;
this.m_Descrip = m_Descrip;
this.m_Prefix = m_Prefix;
this.m_Root_Nam = m_Root_Nam;
this.m_Pfx_Mode = m_Pfx_Mode;
this.ref_DT_ID = ref_DT_ID;
this.m_Dimensions = m_Dimensions;
this.m_DefaultValue = m_DefaultValue;
R102_abstracts_characteristics_of_ModelClass_inst = ModelClassImpl.EMPTY_MODELCLASS;
R103_precedes_O_ATTR_inst = O_ATTRImpl.EMPTY_O_ATTR;
R103_succeeds_O_ATTR_inst = O_ATTRImpl.EMPTY_O_ATTR;
R105_is_part_of__ClassIdentifierAttribute_set = new ClassIdentifierAttributeSetImpl();
R106_is_a_BaseAttribute_inst = BaseAttributeImpl.EMPTY_BASEATTRIBUTE;
R106_is_a_ReferentialAttribute_inst = ReferentialAttributeImpl.EMPTY_REFERENTIALATTRIBUTE;
R114_defines_type_of_DataType_inst = DataTypeImpl.EMPTY_DATATYPE;
R120_may_have_Dimensions_set = new DimensionsSetImpl();
R2910_has_instances_AttributeValue_set = new AttributeValueSetImpl();
R414_Attribute_inst = AttributeImpl.EMPTY_ATTRIBUTE;
R806_AttributeValueReference_set = new AttributeValueReferenceSetImpl();
R812_SelectedReference_set = new SelectedReferenceSetImpl();
R938_defines_InstanceAttributeValue_set = new InstanceAttributeValueSetImpl();
}
public static O_ATTR create( Sql context ) throws XtumlException {
O_ATTR newO_ATTR = new O_ATTRImpl( context );
if ( context.addInstance( newO_ATTR ) ) {
newO_ATTR.getRunContext().addChange(new InstanceCreatedDelta(newO_ATTR, KEY_LETTERS));
return newO_ATTR;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static O_ATTR create( Sql context, UniqueId m_Attr_ID, UniqueId ref_Obj_ID, UniqueId ref_PAttr_ID, String m_Name, String m_Descrip, String m_Prefix, String m_Root_Nam, int m_Pfx_Mode, UniqueId ref_DT_ID, String m_Dimensions, String m_DefaultValue ) throws XtumlException {
return create(context, UniqueId.random(), m_Attr_ID, ref_Obj_ID, ref_PAttr_ID, m_Name, m_Descrip, m_Prefix, m_Root_Nam, m_Pfx_Mode, ref_DT_ID, m_Dimensions, m_DefaultValue);
}
public static O_ATTR create( Sql context, UniqueId instanceId, UniqueId m_Attr_ID, UniqueId ref_Obj_ID, UniqueId ref_PAttr_ID, String m_Name, String m_Descrip, String m_Prefix, String m_Root_Nam, int m_Pfx_Mode, UniqueId ref_DT_ID, String m_Dimensions, String m_DefaultValue ) throws XtumlException {
O_ATTR newO_ATTR = new O_ATTRImpl( context, instanceId, m_Attr_ID, ref_Obj_ID, ref_PAttr_ID, m_Name, m_Descrip, m_Prefix, m_Root_Nam, m_Pfx_Mode, ref_DT_ID, m_Dimensions, m_DefaultValue );
if ( context.addInstance( newO_ATTR ) ) {
return newO_ATTR;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId m_Attr_ID;
@Override
public UniqueId getAttr_ID() throws XtumlException {
checkLiving();
return m_Attr_ID;
}
@Override
public void setAttr_ID(UniqueId m_Attr_ID) throws XtumlException {
checkLiving();
if (m_Attr_ID.inequality( this.m_Attr_ID)) {
final UniqueId oldValue = this.m_Attr_ID;
this.m_Attr_ID = m_Attr_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Attr_ID", oldValue, this.m_Attr_ID));
if ( !R2910_has_instances_AttributeValue().isEmpty() ) R2910_has_instances_AttributeValue().setAttr_ID( m_Attr_ID );
if ( !R938_defines_InstanceAttributeValue().isEmpty() ) R938_defines_InstanceAttributeValue().setAttr_ID( m_Attr_ID );
if ( !R120_may_have_Dimensions().isEmpty() ) R120_may_have_Dimensions().setAttr_ID( m_Attr_ID );
if ( !R106_is_a_BaseAttribute().isEmpty() ) R106_is_a_BaseAttribute().setAttr_ID( m_Attr_ID );
if ( !R103_precedes_O_ATTR().isEmpty() ) R103_precedes_O_ATTR().setPAttr_ID( m_Attr_ID );
if ( !R812_SelectedReference().isEmpty() ) R812_SelectedReference().setAttr_ID( m_Attr_ID );
if ( !R806_AttributeValueReference().isEmpty() ) R806_AttributeValueReference().setAttr_ID( m_Attr_ID );
if ( !R106_is_a_ReferentialAttribute().isEmpty() ) R106_is_a_ReferentialAttribute().setAttr_ID( m_Attr_ID );
if ( !R105_is_part_of__ClassIdentifierAttribute().isEmpty() ) R105_is_part_of__ClassIdentifierAttribute().setAttr_ID( m_Attr_ID );
}
}
private UniqueId ref_Obj_ID;
@Override
public UniqueId getObj_ID() throws XtumlException {
checkLiving();
return ref_Obj_ID;
}
@Override
public void setObj_ID(UniqueId ref_Obj_ID) throws XtumlException {
checkLiving();
if (ref_Obj_ID.inequality( this.ref_Obj_ID)) {
final UniqueId oldValue = this.ref_Obj_ID;
this.ref_Obj_ID = ref_Obj_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Obj_ID", oldValue, this.ref_Obj_ID));
if ( !R120_may_have_Dimensions().isEmpty() ) R120_may_have_Dimensions().setObj_ID( ref_Obj_ID );
if ( !R938_defines_InstanceAttributeValue().isEmpty() ) R938_defines_InstanceAttributeValue().setObj_ID( ref_Obj_ID );
if ( !R106_is_a_BaseAttribute().isEmpty() ) R106_is_a_BaseAttribute().setObj_ID( ref_Obj_ID );
if ( !R105_is_part_of__ClassIdentifierAttribute().isEmpty() ) R105_is_part_of__ClassIdentifierAttribute().setObj_ID( ref_Obj_ID );
if ( !R2910_has_instances_AttributeValue().isEmpty() ) R2910_has_instances_AttributeValue().setObj_ID( ref_Obj_ID );
if ( !R806_AttributeValueReference().isEmpty() ) R806_AttributeValueReference().setObj_ID( ref_Obj_ID );
if ( !R812_SelectedReference().isEmpty() ) R812_SelectedReference().setObj_ID( ref_Obj_ID );
if ( !R106_is_a_ReferentialAttribute().isEmpty() ) R106_is_a_ReferentialAttribute().setObj_ID( ref_Obj_ID );
if ( !R103_precedes_O_ATTR().isEmpty() ) R103_precedes_O_ATTR().setObj_ID( ref_Obj_ID );
}
}
private UniqueId ref_PAttr_ID;
@Override
public void setPAttr_ID(UniqueId ref_PAttr_ID) throws XtumlException {
checkLiving();
if (ref_PAttr_ID.inequality( this.ref_PAttr_ID)) {
final UniqueId oldValue = this.ref_PAttr_ID;
this.ref_PAttr_ID = ref_PAttr_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_PAttr_ID", oldValue, this.ref_PAttr_ID));
}
}
@Override
public UniqueId getPAttr_ID() throws XtumlException {
checkLiving();
return ref_PAttr_ID;
}
private String m_Name;
@Override
public void setName(String m_Name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Name, this.m_Name)) {
final String oldValue = this.m_Name;
this.m_Name = m_Name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Name", oldValue, this.m_Name));
}
}
@Override
public String getName() throws XtumlException {
checkLiving();
return m_Name;
}
private String m_Descrip;
@Override
public String getDescrip() throws XtumlException {
checkLiving();
return m_Descrip;
}
@Override
public void setDescrip(String m_Descrip) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Descrip, this.m_Descrip)) {
final String oldValue = this.m_Descrip;
this.m_Descrip = m_Descrip;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Descrip", oldValue, this.m_Descrip));
}
}
private String m_Prefix;
@Override
public void setPrefix(String m_Prefix) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Prefix, this.m_Prefix)) {
final String oldValue = this.m_Prefix;
this.m_Prefix = m_Prefix;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Prefix", oldValue, this.m_Prefix));
}
}
@Override
public String getPrefix() throws XtumlException {
checkLiving();
return m_Prefix;
}
private String m_Root_Nam;
@Override
public String getRoot_Nam() throws XtumlException {
checkLiving();
return m_Root_Nam;
}
@Override
public void setRoot_Nam(String m_Root_Nam) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Root_Nam, this.m_Root_Nam)) {
final String oldValue = this.m_Root_Nam;
this.m_Root_Nam = m_Root_Nam;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Root_Nam", oldValue, this.m_Root_Nam));
}
}
private int m_Pfx_Mode;
@Override
public void setPfx_Mode(int m_Pfx_Mode) throws XtumlException {
checkLiving();
if (m_Pfx_Mode != this.m_Pfx_Mode) {
final int oldValue = this.m_Pfx_Mode;
this.m_Pfx_Mode = m_Pfx_Mode;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Pfx_Mode", oldValue, this.m_Pfx_Mode));
}
}
@Override
public int getPfx_Mode() throws XtumlException {
checkLiving();
return m_Pfx_Mode;
}
private UniqueId ref_DT_ID;
@Override
public void setDT_ID(UniqueId ref_DT_ID) throws XtumlException {
checkLiving();
if (ref_DT_ID.inequality( this.ref_DT_ID)) {
final UniqueId oldValue = this.ref_DT_ID;
this.ref_DT_ID = ref_DT_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_DT_ID", oldValue, this.ref_DT_ID));
}
}
@Override
public UniqueId getDT_ID() throws XtumlException {
checkLiving();
return ref_DT_ID;
}
private String m_Dimensions;
@Override
public void setDimensions(String m_Dimensions) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Dimensions, this.m_Dimensions)) {
final String oldValue = this.m_Dimensions;
this.m_Dimensions = m_Dimensions;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Dimensions", oldValue, this.m_Dimensions));
}
}
@Override
public String getDimensions() throws XtumlException {
checkLiving();
return m_Dimensions;
}
private String m_DefaultValue;
@Override
public String getDefaultValue() throws XtumlException {
checkLiving();
return m_DefaultValue;
}
@Override
public void setDefaultValue(String m_DefaultValue) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_DefaultValue, this.m_DefaultValue)) {
final String oldValue = this.m_DefaultValue;
this.m_DefaultValue = m_DefaultValue;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_DefaultValue", oldValue, this.m_DefaultValue));
}
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getAttr_ID(), getObj_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private ModelClass R102_abstracts_characteristics_of_ModelClass_inst;
@Override
public void setR102_abstracts_characteristics_of_ModelClass( ModelClass inst ) {
R102_abstracts_characteristics_of_ModelClass_inst = inst;
}
@Override
public ModelClass R102_abstracts_characteristics_of_ModelClass() throws XtumlException {
return R102_abstracts_characteristics_of_ModelClass_inst;
}
private O_ATTR R103_precedes_O_ATTR_inst;
@Override
public void setR103_precedes_O_ATTR( O_ATTR inst ) {
R103_precedes_O_ATTR_inst = inst;
}
@Override
public O_ATTR R103_precedes_O_ATTR() throws XtumlException {
return R103_precedes_O_ATTR_inst;
}
private O_ATTR R103_succeeds_O_ATTR_inst;
@Override
public void setR103_succeeds_O_ATTR( O_ATTR inst ) {
R103_succeeds_O_ATTR_inst = inst;
}
@Override
public O_ATTR R103_succeeds_O_ATTR() throws XtumlException {
return R103_succeeds_O_ATTR_inst;
}
private ClassIdentifierAttributeSet R105_is_part_of__ClassIdentifierAttribute_set;
@Override
public void addR105_is_part_of__ClassIdentifierAttribute( ClassIdentifierAttribute inst ) {
R105_is_part_of__ClassIdentifierAttribute_set.add(inst);
}
@Override
public void removeR105_is_part_of__ClassIdentifierAttribute( ClassIdentifierAttribute inst ) {
R105_is_part_of__ClassIdentifierAttribute_set.remove(inst);
}
@Override
public ClassIdentifierAttributeSet R105_is_part_of__ClassIdentifierAttribute() throws XtumlException {
return R105_is_part_of__ClassIdentifierAttribute_set;
}
private BaseAttribute R106_is_a_BaseAttribute_inst;
@Override
public void setR106_is_a_BaseAttribute( BaseAttribute inst ) {
R106_is_a_BaseAttribute_inst = inst;
}
@Override
public BaseAttribute R106_is_a_BaseAttribute() throws XtumlException {
return R106_is_a_BaseAttribute_inst;
}
private ReferentialAttribute R106_is_a_ReferentialAttribute_inst;
@Override
public void setR106_is_a_ReferentialAttribute( ReferentialAttribute inst ) {
R106_is_a_ReferentialAttribute_inst = inst;
}
@Override
public ReferentialAttribute R106_is_a_ReferentialAttribute() throws XtumlException {
return R106_is_a_ReferentialAttribute_inst;
}
private DataType R114_defines_type_of_DataType_inst;
@Override
public void setR114_defines_type_of_DataType( DataType inst ) {
R114_defines_type_of_DataType_inst = inst;
}
@Override
public DataType R114_defines_type_of_DataType() throws XtumlException {
return R114_defines_type_of_DataType_inst;
}
private DimensionsSet R120_may_have_Dimensions_set;
@Override
public void addR120_may_have_Dimensions( Dimensions inst ) {
R120_may_have_Dimensions_set.add(inst);
}
@Override
public void removeR120_may_have_Dimensions( Dimensions inst ) {
R120_may_have_Dimensions_set.remove(inst);
}
@Override
public DimensionsSet R120_may_have_Dimensions() throws XtumlException {
return R120_may_have_Dimensions_set;
}
private AttributeValueSet R2910_has_instances_AttributeValue_set;
@Override
public void addR2910_has_instances_AttributeValue( AttributeValue inst ) {
R2910_has_instances_AttributeValue_set.add(inst);
}
@Override
public void removeR2910_has_instances_AttributeValue( AttributeValue inst ) {
R2910_has_instances_AttributeValue_set.remove(inst);
}
@Override
public AttributeValueSet R2910_has_instances_AttributeValue() throws XtumlException {
return R2910_has_instances_AttributeValue_set;
}
private Attribute R414_Attribute_inst;
@Override
public void setR414_Attribute( Attribute inst ) {
R414_Attribute_inst = inst;
}
@Override
public Attribute R414_Attribute() throws XtumlException {
return R414_Attribute_inst;
}
private AttributeValueReferenceSet R806_AttributeValueReference_set;
@Override
public void addR806_AttributeValueReference( AttributeValueReference inst ) {
R806_AttributeValueReference_set.add(inst);
}
@Override
public void removeR806_AttributeValueReference( AttributeValueReference inst ) {
R806_AttributeValueReference_set.remove(inst);
}
@Override
public AttributeValueReferenceSet R806_AttributeValueReference() throws XtumlException {
return R806_AttributeValueReference_set;
}
private SelectedReferenceSet R812_SelectedReference_set;
@Override
public void addR812_SelectedReference( SelectedReference inst ) {
R812_SelectedReference_set.add(inst);
}
@Override
public void removeR812_SelectedReference( SelectedReference inst ) {
R812_SelectedReference_set.remove(inst);
}
@Override
public SelectedReferenceSet R812_SelectedReference() throws XtumlException {
return R812_SelectedReference_set;
}
private InstanceAttributeValueSet R938_defines_InstanceAttributeValue_set;
@Override
public void addR938_defines_InstanceAttributeValue( InstanceAttributeValue inst ) {
R938_defines_InstanceAttributeValue_set.add(inst);
}
@Override
public void removeR938_defines_InstanceAttributeValue( InstanceAttributeValue inst ) {
R938_defines_InstanceAttributeValue_set.remove(inst);
}
@Override
public InstanceAttributeValueSet R938_defines_InstanceAttributeValue() throws XtumlException {
return R938_defines_InstanceAttributeValue_set;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public O_ATTR self() {
return this;
}
@Override
public O_ATTR oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_O_ATTR;
}
}
class EmptyO_ATTR extends ModelInstance implements O_ATTR {
// attributes
public UniqueId getAttr_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setAttr_ID( UniqueId m_Attr_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getObj_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setObj_ID( UniqueId ref_Obj_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setPAttr_ID( UniqueId ref_PAttr_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getPAttr_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setName( String m_Name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getName() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getDescrip() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setDescrip( String m_Descrip ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setPrefix( String m_Prefix ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getPrefix() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getRoot_Nam() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setRoot_Nam( String m_Root_Nam ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setPfx_Mode( int m_Pfx_Mode ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getPfx_Mode() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setDT_ID( UniqueId ref_DT_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getDT_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setDimensions( String m_Dimensions ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getDimensions() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getDefaultValue() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setDefaultValue( String m_DefaultValue ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
// operations
// selections
@Override
public ModelClass R102_abstracts_characteristics_of_ModelClass() {
return ModelClassImpl.EMPTY_MODELCLASS;
}
@Override
public O_ATTR R103_precedes_O_ATTR() {
return O_ATTRImpl.EMPTY_O_ATTR;
}
@Override
public O_ATTR R103_succeeds_O_ATTR() {
return O_ATTRImpl.EMPTY_O_ATTR;
}
@Override
public ClassIdentifierAttributeSet R105_is_part_of__ClassIdentifierAttribute() {
return (new ClassIdentifierAttributeSetImpl());
}
@Override
public BaseAttribute R106_is_a_BaseAttribute() {
return BaseAttributeImpl.EMPTY_BASEATTRIBUTE;
}
@Override
public ReferentialAttribute R106_is_a_ReferentialAttribute() {
return ReferentialAttributeImpl.EMPTY_REFERENTIALATTRIBUTE;
}
@Override
public DataType R114_defines_type_of_DataType() {
return DataTypeImpl.EMPTY_DATATYPE;
}
@Override
public DimensionsSet R120_may_have_Dimensions() {
return (new DimensionsSetImpl());
}
@Override
public AttributeValueSet R2910_has_instances_AttributeValue() {
return (new AttributeValueSetImpl());
}
@Override
public Attribute R414_Attribute() {
return AttributeImpl.EMPTY_ATTRIBUTE;
}
@Override
public AttributeValueReferenceSet R806_AttributeValueReference() {
return (new AttributeValueReferenceSetImpl());
}
@Override
public SelectedReferenceSet R812_SelectedReference() {
return (new SelectedReferenceSetImpl());
}
@Override
public InstanceAttributeValueSet R938_defines_InstanceAttributeValue() {
return (new InstanceAttributeValueSetImpl());
}
@Override
public String getKeyLetters() {
return O_ATTRImpl.KEY_LETTERS;
}
@Override
public O_ATTR self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public O_ATTR oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return O_ATTRImpl.EMPTY_O_ATTR;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy