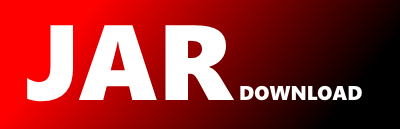
io.ciera.tool.sql.ooaofooa.value.impl.BridgeValueImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.value.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.domain.Bridge;
import io.ciera.tool.sql.ooaofooa.domain.impl.BridgeImpl;
import io.ciera.tool.sql.ooaofooa.value.BridgeValue;
import io.ciera.tool.sql.ooaofooa.value.V_PAR;
import io.ciera.tool.sql.ooaofooa.value.V_PARSet;
import io.ciera.tool.sql.ooaofooa.value.Value;
import io.ciera.tool.sql.ooaofooa.value.impl.V_PARSetImpl;
import io.ciera.tool.sql.ooaofooa.value.impl.ValueImpl;
public class BridgeValueImpl extends ModelInstance implements BridgeValue {
public static final String KEY_LETTERS = "V_BRV";
public static final BridgeValue EMPTY_BRIDGEVALUE = new EmptyBridgeValue();
private Sql context;
// constructors
private BridgeValueImpl( Sql context ) {
this.context = context;
ref_Value_ID = UniqueId.random();
ref_Brg_ID = UniqueId.random();
m_ParmListOK = false;
m_externalEntityKeyLettersLineNumber = 0;
m_externalEntityKeyLettersColumn = 0;
R801_is_a_Value_inst = ValueImpl.EMPTY_VALUE;
R810_has_V_PAR_set = new V_PARSetImpl();
R828_Bridge_inst = BridgeImpl.EMPTY_BRIDGE;
}
private BridgeValueImpl( Sql context, UniqueId instanceId, UniqueId ref_Value_ID, UniqueId ref_Brg_ID, boolean m_ParmListOK, int m_externalEntityKeyLettersLineNumber, int m_externalEntityKeyLettersColumn ) {
super(instanceId);
this.context = context;
this.ref_Value_ID = ref_Value_ID;
this.ref_Brg_ID = ref_Brg_ID;
this.m_ParmListOK = m_ParmListOK;
this.m_externalEntityKeyLettersLineNumber = m_externalEntityKeyLettersLineNumber;
this.m_externalEntityKeyLettersColumn = m_externalEntityKeyLettersColumn;
R801_is_a_Value_inst = ValueImpl.EMPTY_VALUE;
R810_has_V_PAR_set = new V_PARSetImpl();
R828_Bridge_inst = BridgeImpl.EMPTY_BRIDGE;
}
public static BridgeValue create( Sql context ) throws XtumlException {
BridgeValue newBridgeValue = new BridgeValueImpl( context );
if ( context.addInstance( newBridgeValue ) ) {
newBridgeValue.getRunContext().addChange(new InstanceCreatedDelta(newBridgeValue, KEY_LETTERS));
return newBridgeValue;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static BridgeValue create( Sql context, UniqueId ref_Value_ID, UniqueId ref_Brg_ID, boolean m_ParmListOK, int m_externalEntityKeyLettersLineNumber, int m_externalEntityKeyLettersColumn ) throws XtumlException {
return create(context, UniqueId.random(), ref_Value_ID, ref_Brg_ID, m_ParmListOK, m_externalEntityKeyLettersLineNumber, m_externalEntityKeyLettersColumn);
}
public static BridgeValue create( Sql context, UniqueId instanceId, UniqueId ref_Value_ID, UniqueId ref_Brg_ID, boolean m_ParmListOK, int m_externalEntityKeyLettersLineNumber, int m_externalEntityKeyLettersColumn ) throws XtumlException {
BridgeValue newBridgeValue = new BridgeValueImpl( context, instanceId, ref_Value_ID, ref_Brg_ID, m_ParmListOK, m_externalEntityKeyLettersLineNumber, m_externalEntityKeyLettersColumn );
if ( context.addInstance( newBridgeValue ) ) {
return newBridgeValue;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId ref_Value_ID;
@Override
public void setValue_ID(UniqueId ref_Value_ID) throws XtumlException {
checkLiving();
if (ref_Value_ID.inequality( this.ref_Value_ID)) {
final UniqueId oldValue = this.ref_Value_ID;
this.ref_Value_ID = ref_Value_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Value_ID", oldValue, this.ref_Value_ID));
if ( !R810_has_V_PAR().isEmpty() ) R810_has_V_PAR().setInvocation_Value_ID( ref_Value_ID );
}
}
@Override
public UniqueId getValue_ID() throws XtumlException {
checkLiving();
return ref_Value_ID;
}
private UniqueId ref_Brg_ID;
@Override
public void setBrg_ID(UniqueId ref_Brg_ID) throws XtumlException {
checkLiving();
if (ref_Brg_ID.inequality( this.ref_Brg_ID)) {
final UniqueId oldValue = this.ref_Brg_ID;
this.ref_Brg_ID = ref_Brg_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Brg_ID", oldValue, this.ref_Brg_ID));
}
}
@Override
public UniqueId getBrg_ID() throws XtumlException {
checkLiving();
return ref_Brg_ID;
}
private boolean m_ParmListOK;
@Override
public void setParmListOK(boolean m_ParmListOK) throws XtumlException {
checkLiving();
if (m_ParmListOK != this.m_ParmListOK) {
final boolean oldValue = this.m_ParmListOK;
this.m_ParmListOK = m_ParmListOK;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_ParmListOK", oldValue, this.m_ParmListOK));
}
}
@Override
public boolean getParmListOK() throws XtumlException {
checkLiving();
return m_ParmListOK;
}
private int m_externalEntityKeyLettersLineNumber;
@Override
public int getExternalEntityKeyLettersLineNumber() throws XtumlException {
checkLiving();
return m_externalEntityKeyLettersLineNumber;
}
@Override
public void setExternalEntityKeyLettersLineNumber(int m_externalEntityKeyLettersLineNumber) throws XtumlException {
checkLiving();
if (m_externalEntityKeyLettersLineNumber != this.m_externalEntityKeyLettersLineNumber) {
final int oldValue = this.m_externalEntityKeyLettersLineNumber;
this.m_externalEntityKeyLettersLineNumber = m_externalEntityKeyLettersLineNumber;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_externalEntityKeyLettersLineNumber", oldValue, this.m_externalEntityKeyLettersLineNumber));
}
}
private int m_externalEntityKeyLettersColumn;
@Override
public int getExternalEntityKeyLettersColumn() throws XtumlException {
checkLiving();
return m_externalEntityKeyLettersColumn;
}
@Override
public void setExternalEntityKeyLettersColumn(int m_externalEntityKeyLettersColumn) throws XtumlException {
checkLiving();
if (m_externalEntityKeyLettersColumn != this.m_externalEntityKeyLettersColumn) {
final int oldValue = this.m_externalEntityKeyLettersColumn;
this.m_externalEntityKeyLettersColumn = m_externalEntityKeyLettersColumn;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_externalEntityKeyLettersColumn", oldValue, this.m_externalEntityKeyLettersColumn));
}
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getValue_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private Value R801_is_a_Value_inst;
@Override
public void setR801_is_a_Value( Value inst ) {
R801_is_a_Value_inst = inst;
}
@Override
public Value R801_is_a_Value() throws XtumlException {
return R801_is_a_Value_inst;
}
private V_PARSet R810_has_V_PAR_set;
@Override
public void addR810_has_V_PAR( V_PAR inst ) {
R810_has_V_PAR_set.add(inst);
}
@Override
public void removeR810_has_V_PAR( V_PAR inst ) {
R810_has_V_PAR_set.remove(inst);
}
@Override
public V_PARSet R810_has_V_PAR() throws XtumlException {
return R810_has_V_PAR_set;
}
private Bridge R828_Bridge_inst;
@Override
public void setR828_Bridge( Bridge inst ) {
R828_Bridge_inst = inst;
}
@Override
public Bridge R828_Bridge() throws XtumlException {
return R828_Bridge_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public BridgeValue self() {
return this;
}
@Override
public BridgeValue oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_BRIDGEVALUE;
}
}
class EmptyBridgeValue extends ModelInstance implements BridgeValue {
// attributes
public void setValue_ID( UniqueId ref_Value_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getValue_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setBrg_ID( UniqueId ref_Brg_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getBrg_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setParmListOK( boolean m_ParmListOK ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public boolean getParmListOK() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public int getExternalEntityKeyLettersLineNumber() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setExternalEntityKeyLettersLineNumber( int m_externalEntityKeyLettersLineNumber ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getExternalEntityKeyLettersColumn() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setExternalEntityKeyLettersColumn( int m_externalEntityKeyLettersColumn ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
// operations
// selections
@Override
public Value R801_is_a_Value() {
return ValueImpl.EMPTY_VALUE;
}
@Override
public V_PARSet R810_has_V_PAR() {
return (new V_PARSetImpl());
}
@Override
public Bridge R828_Bridge() {
return BridgeImpl.EMPTY_BRIDGE;
}
@Override
public String getKeyLetters() {
return BridgeValueImpl.KEY_LETTERS;
}
@Override
public BridgeValue self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public BridgeValue oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return BridgeValueImpl.EMPTY_BRIDGEVALUE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy