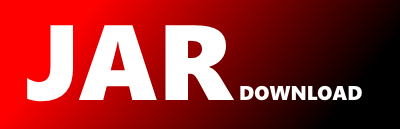
types.ImportType Maven / Gradle / Ivy
package types;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IXtumlType;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public enum ImportType implements IXtumlType {
UNINITIALIZED_ENUM( -1 ),
BOTH( 2 ),
IMPL( 0 ),
INT( 1 );
private final int value;
ImportType() {
value = -1;
}
ImportType( int value ) {
this.value = value;
}
public int getValue() {
return value;
}
@Override
public boolean equality( IXtumlType value ) throws XtumlException {
if (value instanceof ImportType) {
return null != value && this.value == ((ImportType)value).getValue();
}
else return false;
}
@Override
public String serialize() {
return Integer.toString(value);
}
public static ImportType deserialize(Object o) throws XtumlException {
if (o instanceof ImportType) {
return (ImportType)o;
}
else if (o instanceof Integer) {
return valueOf((int)o);
}
else if (o instanceof String) {
try {
return valueOf(Integer.parseInt((String)o));
}
catch (NumberFormatException e1) {
Matcher m = Pattern.compile("([A-Za-z][A-Za-z0-9_]*)::([A-Za-z][A-Za-z0-9_]*)").matcher((String)o);
if (m.matches() && "importtype".equals(m.group(1).toLowerCase())) {
try {
return Enum.valueOf(ImportType.class, m.group(2).toUpperCase());
} catch (IllegalArgumentException e2) {/* do nothing */}
}
}
}
throw new XtumlException("Cannot deserialize value: " + o != null ? o.toString() : "null");
}
public static ImportType valueOf(int value) {
switch( value ) {
case 2:
return BOTH;
case 0:
return IMPL;
case 1:
return INT;
default:
return UNINITIALIZED_ENUM;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy