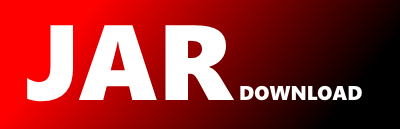
io.cloudshiftdev.awscdk.SynthesizeStackArtifactOptions.kt Maven / Gradle / Ivy
@file:Suppress("RedundantVisibilityModifier","RedundantUnitReturnType","RemoveRedundantQualifierName","unused","UnusedImport","ClassName","REDUNDANT_PROJECTION","DEPRECATION")
package io.cloudshiftdev.awscdk
import io.cloudshiftdev.awscdk.cloud_assembly_schema.BootstrapRole
import io.cloudshiftdev.awscdk.common.CdkDslMarker
import io.cloudshiftdev.awscdk.common.CdkObject
import io.cloudshiftdev.awscdk.common.CdkObjectWrappers
import kotlin.Any
import kotlin.Number
import kotlin.String
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Stack artifact options.
*
* A subset of `cxschema.AwsCloudFormationStackProperties` of optional settings that need to be
* configurable by synthesizers, plus `additionalDependencies`.
*
* Example:
*
* ```
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import io.cloudshiftdev.awscdk.*;
* Object assumeRoleAdditionalOptions;
* SynthesizeStackArtifactOptions synthesizeStackArtifactOptions =
* SynthesizeStackArtifactOptions.builder()
* .additionalDependencies(List.of("additionalDependencies"))
* .assumeRoleAdditionalOptions(Map.of(
* "assumeRoleAdditionalOptionsKey", assumeRoleAdditionalOptions))
* .assumeRoleArn("assumeRoleArn")
* .assumeRoleExternalId("assumeRoleExternalId")
* .bootstrapStackVersionSsmParameter("bootstrapStackVersionSsmParameter")
* .cloudFormationExecutionRoleArn("cloudFormationExecutionRoleArn")
* .lookupRole(BootstrapRole.builder()
* .arn("arn")
* // the properties below are optional
* .assumeRoleAdditionalOptions(Map.of(
* "assumeRoleAdditionalOptionsKey", assumeRoleAdditionalOptions))
* .assumeRoleExternalId("assumeRoleExternalId")
* .bootstrapStackVersionSsmParameter("bootstrapStackVersionSsmParameter")
* .requiresBootstrapStackVersion(123)
* .build())
* .parameters(Map.of(
* "parametersKey", "parameters"))
* .requiresBootstrapStackVersion(123)
* .stackTemplateAssetObjectUrl("stackTemplateAssetObjectUrl")
* .build();
* ```
*/
public interface SynthesizeStackArtifactOptions {
/**
* Identifiers of additional dependencies.
*
* Default: - No additional dependencies
*/
public fun additionalDependencies(): List = unwrap(this).getAdditionalDependencies() ?:
emptyList()
/**
* Additional options to pass to STS when assuming the role for cloudformation deployments.
*
* * `RoleArn` should not be used. Use the dedicated `assumeRoleArn` property instead.
* * `ExternalId` should not be used. Use the dedicated `assumeRoleExternalId` instead.
* * `TransitiveTagKeys` defaults to use all keys (if any) specified in `Tags`. E.g, all tags are
* transitive by default.
*
* Default: - No additional options.
*
* [Documentation](https://docs.aws.amazon.com/AWSJavaScriptSDK/latest/AWS/STS.html#assumeRole-property)
*/
public fun assumeRoleAdditionalOptions(): Map =
unwrap(this).getAssumeRoleAdditionalOptions() ?: emptyMap()
/**
* The role that needs to be assumed to deploy the stack.
*
* Default: - No role is assumed (current credentials are used)
*/
public fun assumeRoleArn(): String? = unwrap(this).getAssumeRoleArn()
/**
* The externalID to use with the assumeRoleArn.
*
* Default: - No externalID is used
*/
public fun assumeRoleExternalId(): String? = unwrap(this).getAssumeRoleExternalId()
/**
* SSM parameter where the bootstrap stack version number can be found.
*
* Only used if `requiresBootstrapStackVersion` is set.
*
* * If this value is not set, the bootstrap stack name must be known at
* deployment time so the stack version can be looked up from the stack
* outputs.
* * If this value is set, the bootstrap stack can have any name because
* we won't need to look it up.
*
* Default: - Bootstrap stack version number looked up
*/
public fun bootstrapStackVersionSsmParameter(): String? =
unwrap(this).getBootstrapStackVersionSsmParameter()
/**
* The role that is passed to CloudFormation to execute the change set.
*
* Default: - No role is passed (currently assumed role/credentials are used)
*/
public fun cloudFormationExecutionRoleArn(): String? =
unwrap(this).getCloudFormationExecutionRoleArn()
/**
* The role to use to look up values from the target AWS account.
*
* Default: - None
*/
public fun lookupRole(): BootstrapRole? = unwrap(this).getLookupRole()?.let(BootstrapRole::wrap)
/**
* Values for CloudFormation stack parameters that should be passed when the stack is deployed.
*
* Default: - No parameters
*/
public fun parameters(): Map = unwrap(this).getParameters() ?: emptyMap()
/**
* Version of bootstrap stack required to deploy this stack.
*
* Default: - No bootstrap stack required
*/
public fun requiresBootstrapStackVersion(): Number? =
unwrap(this).getRequiresBootstrapStackVersion()
/**
* If the stack template has already been included in the asset manifest, its asset URL.
*
* Default: - Not uploaded yet, upload just before deploying
*/
public fun stackTemplateAssetObjectUrl(): String? = unwrap(this).getStackTemplateAssetObjectUrl()
/**
* A builder for [SynthesizeStackArtifactOptions]
*/
@CdkDslMarker
public interface Builder {
/**
* @param additionalDependencies Identifiers of additional dependencies.
*/
public fun additionalDependencies(additionalDependencies: List)
/**
* @param additionalDependencies Identifiers of additional dependencies.
*/
public fun additionalDependencies(vararg additionalDependencies: String)
/**
* @param assumeRoleAdditionalOptions Additional options to pass to STS when assuming the role
* for cloudformation deployments.
* * `RoleArn` should not be used. Use the dedicated `assumeRoleArn` property instead.
* * `ExternalId` should not be used. Use the dedicated `assumeRoleExternalId` instead.
* * `TransitiveTagKeys` defaults to use all keys (if any) specified in `Tags`. E.g, all tags
* are transitive by default.
*/
public fun assumeRoleAdditionalOptions(assumeRoleAdditionalOptions: Map)
/**
* @param assumeRoleArn The role that needs to be assumed to deploy the stack.
*/
public fun assumeRoleArn(assumeRoleArn: String)
/**
* @param assumeRoleExternalId The externalID to use with the assumeRoleArn.
*/
public fun assumeRoleExternalId(assumeRoleExternalId: String)
/**
* @param bootstrapStackVersionSsmParameter SSM parameter where the bootstrap stack version
* number can be found.
* Only used if `requiresBootstrapStackVersion` is set.
*
* * If this value is not set, the bootstrap stack name must be known at
* deployment time so the stack version can be looked up from the stack
* outputs.
* * If this value is set, the bootstrap stack can have any name because
* we won't need to look it up.
*/
public fun bootstrapStackVersionSsmParameter(bootstrapStackVersionSsmParameter: String)
/**
* @param cloudFormationExecutionRoleArn The role that is passed to CloudFormation to execute
* the change set.
*/
public fun cloudFormationExecutionRoleArn(cloudFormationExecutionRoleArn: String)
/**
* @param lookupRole The role to use to look up values from the target AWS account.
*/
public fun lookupRole(lookupRole: BootstrapRole)
/**
* @param lookupRole The role to use to look up values from the target AWS account.
*/
@kotlin.Suppress("INAPPLICABLE_JVM_NAME")
@JvmName("1931a134460bf2dc8dbbd5562b372cf8502a457973718015da4df8928d7c72dd")
public fun lookupRole(lookupRole: BootstrapRole.Builder.() -> Unit)
/**
* @param parameters Values for CloudFormation stack parameters that should be passed when the
* stack is deployed.
*/
public fun parameters(parameters: Map)
/**
* @param requiresBootstrapStackVersion Version of bootstrap stack required to deploy this
* stack.
*/
public fun requiresBootstrapStackVersion(requiresBootstrapStackVersion: Number)
/**
* @param stackTemplateAssetObjectUrl If the stack template has already been included in the
* asset manifest, its asset URL.
*/
public fun stackTemplateAssetObjectUrl(stackTemplateAssetObjectUrl: String)
}
private class BuilderImpl : Builder {
private val cdkBuilder: software.amazon.awscdk.SynthesizeStackArtifactOptions.Builder =
software.amazon.awscdk.SynthesizeStackArtifactOptions.builder()
/**
* @param additionalDependencies Identifiers of additional dependencies.
*/
override fun additionalDependencies(additionalDependencies: List) {
cdkBuilder.additionalDependencies(additionalDependencies)
}
/**
* @param additionalDependencies Identifiers of additional dependencies.
*/
override fun additionalDependencies(vararg additionalDependencies: String): Unit =
additionalDependencies(additionalDependencies.toList())
/**
* @param assumeRoleAdditionalOptions Additional options to pass to STS when assuming the role
* for cloudformation deployments.
* * `RoleArn` should not be used. Use the dedicated `assumeRoleArn` property instead.
* * `ExternalId` should not be used. Use the dedicated `assumeRoleExternalId` instead.
* * `TransitiveTagKeys` defaults to use all keys (if any) specified in `Tags`. E.g, all tags
* are transitive by default.
*/
override fun assumeRoleAdditionalOptions(assumeRoleAdditionalOptions: Map) {
cdkBuilder.assumeRoleAdditionalOptions(assumeRoleAdditionalOptions.mapValues{CdkObjectWrappers.unwrap(it.value)})
}
/**
* @param assumeRoleArn The role that needs to be assumed to deploy the stack.
*/
override fun assumeRoleArn(assumeRoleArn: String) {
cdkBuilder.assumeRoleArn(assumeRoleArn)
}
/**
* @param assumeRoleExternalId The externalID to use with the assumeRoleArn.
*/
override fun assumeRoleExternalId(assumeRoleExternalId: String) {
cdkBuilder.assumeRoleExternalId(assumeRoleExternalId)
}
/**
* @param bootstrapStackVersionSsmParameter SSM parameter where the bootstrap stack version
* number can be found.
* Only used if `requiresBootstrapStackVersion` is set.
*
* * If this value is not set, the bootstrap stack name must be known at
* deployment time so the stack version can be looked up from the stack
* outputs.
* * If this value is set, the bootstrap stack can have any name because
* we won't need to look it up.
*/
override fun bootstrapStackVersionSsmParameter(bootstrapStackVersionSsmParameter: String) {
cdkBuilder.bootstrapStackVersionSsmParameter(bootstrapStackVersionSsmParameter)
}
/**
* @param cloudFormationExecutionRoleArn The role that is passed to CloudFormation to execute
* the change set.
*/
override fun cloudFormationExecutionRoleArn(cloudFormationExecutionRoleArn: String) {
cdkBuilder.cloudFormationExecutionRoleArn(cloudFormationExecutionRoleArn)
}
/**
* @param lookupRole The role to use to look up values from the target AWS account.
*/
override fun lookupRole(lookupRole: BootstrapRole) {
cdkBuilder.lookupRole(lookupRole.let(BootstrapRole.Companion::unwrap))
}
/**
* @param lookupRole The role to use to look up values from the target AWS account.
*/
@kotlin.Suppress("INAPPLICABLE_JVM_NAME")
@JvmName("1931a134460bf2dc8dbbd5562b372cf8502a457973718015da4df8928d7c72dd")
override fun lookupRole(lookupRole: BootstrapRole.Builder.() -> Unit): Unit =
lookupRole(BootstrapRole(lookupRole))
/**
* @param parameters Values for CloudFormation stack parameters that should be passed when the
* stack is deployed.
*/
override fun parameters(parameters: Map) {
cdkBuilder.parameters(parameters)
}
/**
* @param requiresBootstrapStackVersion Version of bootstrap stack required to deploy this
* stack.
*/
override fun requiresBootstrapStackVersion(requiresBootstrapStackVersion: Number) {
cdkBuilder.requiresBootstrapStackVersion(requiresBootstrapStackVersion)
}
/**
* @param stackTemplateAssetObjectUrl If the stack template has already been included in the
* asset manifest, its asset URL.
*/
override fun stackTemplateAssetObjectUrl(stackTemplateAssetObjectUrl: String) {
cdkBuilder.stackTemplateAssetObjectUrl(stackTemplateAssetObjectUrl)
}
public fun build(): software.amazon.awscdk.SynthesizeStackArtifactOptions = cdkBuilder.build()
}
private class Wrapper(
cdkObject: software.amazon.awscdk.SynthesizeStackArtifactOptions,
) : CdkObject(cdkObject),
SynthesizeStackArtifactOptions {
/**
* Identifiers of additional dependencies.
*
* Default: - No additional dependencies
*/
override fun additionalDependencies(): List = unwrap(this).getAdditionalDependencies()
?: emptyList()
/**
* Additional options to pass to STS when assuming the role for cloudformation deployments.
*
* * `RoleArn` should not be used. Use the dedicated `assumeRoleArn` property instead.
* * `ExternalId` should not be used. Use the dedicated `assumeRoleExternalId` instead.
* * `TransitiveTagKeys` defaults to use all keys (if any) specified in `Tags`. E.g, all tags
* are transitive by default.
*
* Default: - No additional options.
*
* [Documentation](https://docs.aws.amazon.com/AWSJavaScriptSDK/latest/AWS/STS.html#assumeRole-property)
*/
override fun assumeRoleAdditionalOptions(): Map =
unwrap(this).getAssumeRoleAdditionalOptions() ?: emptyMap()
/**
* The role that needs to be assumed to deploy the stack.
*
* Default: - No role is assumed (current credentials are used)
*/
override fun assumeRoleArn(): String? = unwrap(this).getAssumeRoleArn()
/**
* The externalID to use with the assumeRoleArn.
*
* Default: - No externalID is used
*/
override fun assumeRoleExternalId(): String? = unwrap(this).getAssumeRoleExternalId()
/**
* SSM parameter where the bootstrap stack version number can be found.
*
* Only used if `requiresBootstrapStackVersion` is set.
*
* * If this value is not set, the bootstrap stack name must be known at
* deployment time so the stack version can be looked up from the stack
* outputs.
* * If this value is set, the bootstrap stack can have any name because
* we won't need to look it up.
*
* Default: - Bootstrap stack version number looked up
*/
override fun bootstrapStackVersionSsmParameter(): String? =
unwrap(this).getBootstrapStackVersionSsmParameter()
/**
* The role that is passed to CloudFormation to execute the change set.
*
* Default: - No role is passed (currently assumed role/credentials are used)
*/
override fun cloudFormationExecutionRoleArn(): String? =
unwrap(this).getCloudFormationExecutionRoleArn()
/**
* The role to use to look up values from the target AWS account.
*
* Default: - None
*/
override fun lookupRole(): BootstrapRole? =
unwrap(this).getLookupRole()?.let(BootstrapRole::wrap)
/**
* Values for CloudFormation stack parameters that should be passed when the stack is deployed.
*
* Default: - No parameters
*/
override fun parameters(): Map = unwrap(this).getParameters() ?: emptyMap()
/**
* Version of bootstrap stack required to deploy this stack.
*
* Default: - No bootstrap stack required
*/
override fun requiresBootstrapStackVersion(): Number? =
unwrap(this).getRequiresBootstrapStackVersion()
/**
* If the stack template has already been included in the asset manifest, its asset URL.
*
* Default: - Not uploaded yet, upload just before deploying
*/
override fun stackTemplateAssetObjectUrl(): String? =
unwrap(this).getStackTemplateAssetObjectUrl()
}
public companion object {
public operator fun invoke(block: Builder.() -> Unit = {}): SynthesizeStackArtifactOptions {
val builderImpl = BuilderImpl()
return Wrapper(builderImpl.apply(block).build())
}
internal fun wrap(cdkObject: software.amazon.awscdk.SynthesizeStackArtifactOptions):
SynthesizeStackArtifactOptions = CdkObjectWrappers.wrap(cdkObject) as?
SynthesizeStackArtifactOptions ?: Wrapper(cdkObject)
internal fun unwrap(wrapped: SynthesizeStackArtifactOptions):
software.amazon.awscdk.SynthesizeStackArtifactOptions = (wrapped as CdkObject).cdkObject as
software.amazon.awscdk.SynthesizeStackArtifactOptions
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy