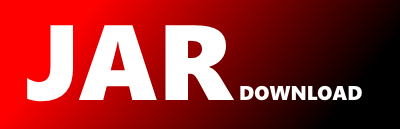
io.cloudshiftdev.awscdk.services.apigatewayv2.WebSocketIntegrationProps.kt Maven / Gradle / Ivy
@file:Suppress("RedundantVisibilityModifier","RedundantUnitReturnType","RemoveRedundantQualifierName","unused","UnusedImport","ClassName","REDUNDANT_PROJECTION","DEPRECATION")
package io.cloudshiftdev.awscdk.services.apigatewayv2
import io.cloudshiftdev.awscdk.Duration
import io.cloudshiftdev.awscdk.common.CdkDslMarker
import io.cloudshiftdev.awscdk.common.CdkObject
import io.cloudshiftdev.awscdk.common.CdkObjectWrappers
import io.cloudshiftdev.awscdk.services.iam.IRole
import kotlin.String
import kotlin.Unit
import kotlin.collections.Map
/**
* The integration properties.
*
* Example:
*
* ```
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import io.cloudshiftdev.awscdk.*;
* import io.cloudshiftdev.awscdk.services.apigatewayv2.*;
* import io.cloudshiftdev.awscdk.services.iam.*;
* Role role;
* WebSocketApi webSocketApi;
* WebSocketIntegrationProps webSocketIntegrationProps = WebSocketIntegrationProps.builder()
* .integrationType(WebSocketIntegrationType.AWS_PROXY)
* .integrationUri("integrationUri")
* .webSocketApi(webSocketApi)
* // the properties below are optional
* .contentHandling(ContentHandling.CONVERT_TO_BINARY)
* .credentialsRole(role)
* .integrationMethod("integrationMethod")
* .passthroughBehavior(PassthroughBehavior.WHEN_NO_MATCH)
* .requestParameters(Map.of(
* "requestParametersKey", "requestParameters"))
* .requestTemplates(Map.of(
* "requestTemplatesKey", "requestTemplates"))
* .templateSelectionExpression("templateSelectionExpression")
* .timeout(Duration.minutes(30))
* .build();
* ```
*/
public interface WebSocketIntegrationProps {
/**
* Specifies how to handle response payload content type conversions.
*
* Default: - The response payload will be passed through from the integration response to
* the route response or method response without modification.
*/
public fun contentHandling(): ContentHandling? =
unwrap(this).getContentHandling()?.let(ContentHandling::wrap)
/**
* Specifies the IAM role required for the integration.
*
* Default: - No IAM role required.
*/
public fun credentialsRole(): IRole? = unwrap(this).getCredentialsRole()?.let(IRole::wrap)
/**
* Specifies the integration's HTTP method type.
*
* Default: - No HTTP method required.
*/
public fun integrationMethod(): String? = unwrap(this).getIntegrationMethod()
/**
* Integration type.
*/
public fun integrationType(): WebSocketIntegrationType
/**
* Integration URI.
*/
public fun integrationUri(): String
/**
* Specifies the pass-through behavior for incoming requests based on the Content-Type header in
* the request, and the available mapping templates specified as the requestTemplates property on the
* Integration resource.
*
* There are three valid values: WHEN_NO_MATCH, WHEN_NO_TEMPLATES, and
* NEVER.
*
* Default: - No passthrough behavior required.
*/
public fun passthroughBehavior(): PassthroughBehavior? =
unwrap(this).getPassthroughBehavior()?.let(PassthroughBehavior::wrap)
/**
* The request parameters that API Gateway sends with the backend request.
*
* Specify request parameters as key-value pairs (string-to-string
* mappings), with a destination as the key and a source as the value.
*
* Default: - No request parameters required.
*/
public fun requestParameters(): Map = unwrap(this).getRequestParameters() ?:
emptyMap()
/**
* A map of Apache Velocity templates that are applied on the request payload.
*
* ```
* { "application/json": "{ \"statusCode\": 200 }" }
* ```
*
* Default: - No request templates required.
*/
public fun requestTemplates(): Map = unwrap(this).getRequestTemplates() ?:
emptyMap()
/**
* The template selection expression for the integration.
*
* Default: - No template selection expression required.
*/
public fun templateSelectionExpression(): String? = unwrap(this).getTemplateSelectionExpression()
/**
* The maximum amount of time an integration will run before it returns without a response.
*
* Must be between 50 milliseconds and 29 seconds.
*
* Default: Duration.seconds(29)
*/
public fun timeout(): Duration? = unwrap(this).getTimeout()?.let(Duration::wrap)
/**
* The WebSocket API to which this integration should be bound.
*/
public fun webSocketApi(): IWebSocketApi
/**
* A builder for [WebSocketIntegrationProps]
*/
@CdkDslMarker
public interface Builder {
/**
* @param contentHandling Specifies how to handle response payload content type conversions.
*/
public fun contentHandling(contentHandling: ContentHandling)
/**
* @param credentialsRole Specifies the IAM role required for the integration.
*/
public fun credentialsRole(credentialsRole: IRole)
/**
* @param integrationMethod Specifies the integration's HTTP method type.
*/
public fun integrationMethod(integrationMethod: String)
/**
* @param integrationType Integration type.
*/
public fun integrationType(integrationType: WebSocketIntegrationType)
/**
* @param integrationUri Integration URI.
*/
public fun integrationUri(integrationUri: String)
/**
* @param passthroughBehavior Specifies the pass-through behavior for incoming requests based on
* the Content-Type header in the request, and the available mapping templates specified as the
* requestTemplates property on the Integration resource.
* There are three valid values: WHEN_NO_MATCH, WHEN_NO_TEMPLATES, and
* NEVER.
*/
public fun passthroughBehavior(passthroughBehavior: PassthroughBehavior)
/**
* @param requestParameters The request parameters that API Gateway sends with the backend
* request.
* Specify request parameters as key-value pairs (string-to-string
* mappings), with a destination as the key and a source as the value.
*/
public fun requestParameters(requestParameters: Map)
/**
* @param requestTemplates A map of Apache Velocity templates that are applied on the request
* payload.
* ```
* { "application/json": "{ \"statusCode\": 200 }" }
* ```
*/
public fun requestTemplates(requestTemplates: Map)
/**
* @param templateSelectionExpression The template selection expression for the integration.
*/
public fun templateSelectionExpression(templateSelectionExpression: String)
/**
* @param timeout The maximum amount of time an integration will run before it returns without a
* response.
* Must be between 50 milliseconds and 29 seconds.
*/
public fun timeout(timeout: Duration)
/**
* @param webSocketApi The WebSocket API to which this integration should be bound.
*/
public fun webSocketApi(webSocketApi: IWebSocketApi)
}
private class BuilderImpl : Builder {
private val cdkBuilder:
software.amazon.awscdk.services.apigatewayv2.WebSocketIntegrationProps.Builder =
software.amazon.awscdk.services.apigatewayv2.WebSocketIntegrationProps.builder()
/**
* @param contentHandling Specifies how to handle response payload content type conversions.
*/
override fun contentHandling(contentHandling: ContentHandling) {
cdkBuilder.contentHandling(contentHandling.let(ContentHandling.Companion::unwrap))
}
/**
* @param credentialsRole Specifies the IAM role required for the integration.
*/
override fun credentialsRole(credentialsRole: IRole) {
cdkBuilder.credentialsRole(credentialsRole.let(IRole.Companion::unwrap))
}
/**
* @param integrationMethod Specifies the integration's HTTP method type.
*/
override fun integrationMethod(integrationMethod: String) {
cdkBuilder.integrationMethod(integrationMethod)
}
/**
* @param integrationType Integration type.
*/
override fun integrationType(integrationType: WebSocketIntegrationType) {
cdkBuilder.integrationType(integrationType.let(WebSocketIntegrationType.Companion::unwrap))
}
/**
* @param integrationUri Integration URI.
*/
override fun integrationUri(integrationUri: String) {
cdkBuilder.integrationUri(integrationUri)
}
/**
* @param passthroughBehavior Specifies the pass-through behavior for incoming requests based on
* the Content-Type header in the request, and the available mapping templates specified as the
* requestTemplates property on the Integration resource.
* There are three valid values: WHEN_NO_MATCH, WHEN_NO_TEMPLATES, and
* NEVER.
*/
override fun passthroughBehavior(passthroughBehavior: PassthroughBehavior) {
cdkBuilder.passthroughBehavior(passthroughBehavior.let(PassthroughBehavior.Companion::unwrap))
}
/**
* @param requestParameters The request parameters that API Gateway sends with the backend
* request.
* Specify request parameters as key-value pairs (string-to-string
* mappings), with a destination as the key and a source as the value.
*/
override fun requestParameters(requestParameters: Map) {
cdkBuilder.requestParameters(requestParameters)
}
/**
* @param requestTemplates A map of Apache Velocity templates that are applied on the request
* payload.
* ```
* { "application/json": "{ \"statusCode\": 200 }" }
* ```
*/
override fun requestTemplates(requestTemplates: Map) {
cdkBuilder.requestTemplates(requestTemplates)
}
/**
* @param templateSelectionExpression The template selection expression for the integration.
*/
override fun templateSelectionExpression(templateSelectionExpression: String) {
cdkBuilder.templateSelectionExpression(templateSelectionExpression)
}
/**
* @param timeout The maximum amount of time an integration will run before it returns without a
* response.
* Must be between 50 milliseconds and 29 seconds.
*/
override fun timeout(timeout: Duration) {
cdkBuilder.timeout(timeout.let(Duration.Companion::unwrap))
}
/**
* @param webSocketApi The WebSocket API to which this integration should be bound.
*/
override fun webSocketApi(webSocketApi: IWebSocketApi) {
cdkBuilder.webSocketApi(webSocketApi.let(IWebSocketApi.Companion::unwrap))
}
public fun build(): software.amazon.awscdk.services.apigatewayv2.WebSocketIntegrationProps =
cdkBuilder.build()
}
private class Wrapper(
cdkObject: software.amazon.awscdk.services.apigatewayv2.WebSocketIntegrationProps,
) : CdkObject(cdkObject),
WebSocketIntegrationProps {
/**
* Specifies how to handle response payload content type conversions.
*
* Default: - The response payload will be passed through from the integration response to
* the route response or method response without modification.
*/
override fun contentHandling(): ContentHandling? =
unwrap(this).getContentHandling()?.let(ContentHandling::wrap)
/**
* Specifies the IAM role required for the integration.
*
* Default: - No IAM role required.
*/
override fun credentialsRole(): IRole? = unwrap(this).getCredentialsRole()?.let(IRole::wrap)
/**
* Specifies the integration's HTTP method type.
*
* Default: - No HTTP method required.
*/
override fun integrationMethod(): String? = unwrap(this).getIntegrationMethod()
/**
* Integration type.
*/
override fun integrationType(): WebSocketIntegrationType =
unwrap(this).getIntegrationType().let(WebSocketIntegrationType::wrap)
/**
* Integration URI.
*/
override fun integrationUri(): String = unwrap(this).getIntegrationUri()
/**
* Specifies the pass-through behavior for incoming requests based on the Content-Type header in
* the request, and the available mapping templates specified as the requestTemplates property on
* the Integration resource.
*
* There are three valid values: WHEN_NO_MATCH, WHEN_NO_TEMPLATES, and
* NEVER.
*
* Default: - No passthrough behavior required.
*/
override fun passthroughBehavior(): PassthroughBehavior? =
unwrap(this).getPassthroughBehavior()?.let(PassthroughBehavior::wrap)
/**
* The request parameters that API Gateway sends with the backend request.
*
* Specify request parameters as key-value pairs (string-to-string
* mappings), with a destination as the key and a source as the value.
*
* Default: - No request parameters required.
*/
override fun requestParameters(): Map = unwrap(this).getRequestParameters() ?:
emptyMap()
/**
* A map of Apache Velocity templates that are applied on the request payload.
*
* ```
* { "application/json": "{ \"statusCode\": 200 }" }
* ```
*
* Default: - No request templates required.
*/
override fun requestTemplates(): Map = unwrap(this).getRequestTemplates() ?:
emptyMap()
/**
* The template selection expression for the integration.
*
* Default: - No template selection expression required.
*/
override fun templateSelectionExpression(): String? =
unwrap(this).getTemplateSelectionExpression()
/**
* The maximum amount of time an integration will run before it returns without a response.
*
* Must be between 50 milliseconds and 29 seconds.
*
* Default: Duration.seconds(29)
*/
override fun timeout(): Duration? = unwrap(this).getTimeout()?.let(Duration::wrap)
/**
* The WebSocket API to which this integration should be bound.
*/
override fun webSocketApi(): IWebSocketApi =
unwrap(this).getWebSocketApi().let(IWebSocketApi::wrap)
}
public companion object {
public operator fun invoke(block: Builder.() -> Unit = {}): WebSocketIntegrationProps {
val builderImpl = BuilderImpl()
return Wrapper(builderImpl.apply(block).build())
}
internal
fun wrap(cdkObject: software.amazon.awscdk.services.apigatewayv2.WebSocketIntegrationProps):
WebSocketIntegrationProps = CdkObjectWrappers.wrap(cdkObject) as? WebSocketIntegrationProps
?: Wrapper(cdkObject)
internal fun unwrap(wrapped: WebSocketIntegrationProps):
software.amazon.awscdk.services.apigatewayv2.WebSocketIntegrationProps = (wrapped as
CdkObject).cdkObject as
software.amazon.awscdk.services.apigatewayv2.WebSocketIntegrationProps
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy