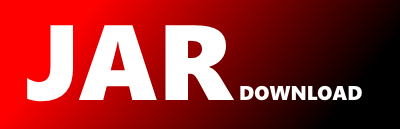
io.cloudshiftdev.awscdk.services.codepipeline.CfnWebhook.kt Maven / Gradle / Ivy
@file:Suppress("RedundantVisibilityModifier","RedundantUnitReturnType","RemoveRedundantQualifierName","unused","UnusedImport","ClassName","REDUNDANT_PROJECTION","DEPRECATION")
package io.cloudshiftdev.awscdk.services.codepipeline
import io.cloudshiftdev.awscdk.CfnResource
import io.cloudshiftdev.awscdk.IInspectable
import io.cloudshiftdev.awscdk.IResolvable
import io.cloudshiftdev.awscdk.TreeInspector
import io.cloudshiftdev.awscdk.common.CdkDslMarker
import io.cloudshiftdev.awscdk.common.CdkObject
import io.cloudshiftdev.awscdk.common.CdkObjectWrappers
import kotlin.Any
import kotlin.Boolean
import kotlin.Number
import kotlin.String
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
import io.cloudshiftdev.constructs.Construct as CloudshiftdevConstructsConstruct
import software.constructs.Construct as SoftwareConstructsConstruct
/**
* The `AWS::CodePipeline::Webhook` resource creates and registers your webhook.
*
* After the webhook is created and registered, it triggers your pipeline to start every time an
* external event occurs. For more information, see [Migrate polling pipelines to use event-based
* change
* detection](https://docs.aws.amazon.com/codepipeline/latest/userguide/update-change-detection.html)
* in the *AWS CodePipeline User Guide* .
*
* We strongly recommend that you use AWS Secrets Manager to store your credentials. If you use
* Secrets Manager, you must have already configured and stored your secret parameters in Secrets
* Manager. For more information, see [Using Dynamic References to Specify Template
* Values](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/dynamic-references.html#dynamic-references-secretsmanager)
* .
*
*
* When passing secret parameters, do not enter the value directly into the template. The value is
* rendered as plaintext and is therefore readable. For security reasons, do not use plaintext in your
* AWS CloudFormation template to store your credentials.
*
*
* Example:
*
* ```
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import io.cloudshiftdev.awscdk.services.codepipeline.*;
* CfnWebhook cfnWebhook = CfnWebhook.Builder.create(this, "MyCfnWebhook")
* .authentication("authentication")
* .authenticationConfiguration(WebhookAuthConfigurationProperty.builder()
* .allowedIpRange("allowedIpRange")
* .secretToken("secretToken")
* .build())
* .filters(List.of(WebhookFilterRuleProperty.builder()
* .jsonPath("jsonPath")
* // the properties below are optional
* .matchEquals("matchEquals")
* .build()))
* .targetAction("targetAction")
* .targetPipeline("targetPipeline")
* .targetPipelineVersion(123)
* // the properties below are optional
* .name("name")
* .registerWithThirdParty(false)
* .build();
* ```
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html)
*/
public open class CfnWebhook(
cdkObject: software.amazon.awscdk.services.codepipeline.CfnWebhook,
) : CfnResource(cdkObject),
IInspectable {
public constructor(
scope: CloudshiftdevConstructsConstruct,
id: String,
props: CfnWebhookProps,
) :
this(software.amazon.awscdk.services.codepipeline.CfnWebhook(scope.let(CloudshiftdevConstructsConstruct.Companion::unwrap),
id, props.let(CfnWebhookProps.Companion::unwrap))
)
public constructor(
scope: CloudshiftdevConstructsConstruct,
id: String,
props: CfnWebhookProps.Builder.() -> Unit,
) : this(scope, id, CfnWebhookProps(props)
)
/**
*
*/
public open fun attrId(): String = unwrap(this).getAttrId()
/**
* The webhook URL generated by AWS CodePipeline , such as
* `https://eu-central-1.webhooks.aws/trigger123456` .
*/
public open fun attrUrl(): String = unwrap(this).getAttrUrl()
/**
* Supported options are GITHUB_HMAC, IP, and UNAUTHENTICATED.
*/
public open fun authentication(): String = unwrap(this).getAuthentication()
/**
* Supported options are GITHUB_HMAC, IP, and UNAUTHENTICATED.
*/
public open fun authentication(`value`: String) {
unwrap(this).setAuthentication(`value`)
}
/**
* Properties that configure the authentication applied to incoming webhook trigger requests.
*/
public open fun authenticationConfiguration(): Any = unwrap(this).getAuthenticationConfiguration()
/**
* Properties that configure the authentication applied to incoming webhook trigger requests.
*/
public open fun authenticationConfiguration(`value`: IResolvable) {
unwrap(this).setAuthenticationConfiguration(`value`.let(IResolvable.Companion::unwrap))
}
/**
* Properties that configure the authentication applied to incoming webhook trigger requests.
*/
public open fun authenticationConfiguration(`value`: WebhookAuthConfigurationProperty) {
unwrap(this).setAuthenticationConfiguration(`value`.let(WebhookAuthConfigurationProperty.Companion::unwrap))
}
/**
* Properties that configure the authentication applied to incoming webhook trigger requests.
*/
@kotlin.Suppress("INAPPLICABLE_JVM_NAME")
@JvmName("cd78bec4077abfccb48c6e5cb9bcb8d8fc0be3233ef7bf516b5b45c66e202253")
public open
fun authenticationConfiguration(`value`: WebhookAuthConfigurationProperty.Builder.() -> Unit):
Unit = authenticationConfiguration(WebhookAuthConfigurationProperty(`value`))
/**
* A list of rules applied to the body/payload sent in the POST request to a webhook URL.
*/
public open fun filters(): Any = unwrap(this).getFilters()
/**
* A list of rules applied to the body/payload sent in the POST request to a webhook URL.
*/
public open fun filters(`value`: IResolvable) {
unwrap(this).setFilters(`value`.let(IResolvable.Companion::unwrap))
}
/**
* A list of rules applied to the body/payload sent in the POST request to a webhook URL.
*/
public open fun filters(`value`: List) {
unwrap(this).setFilters(`value`.map{CdkObjectWrappers.unwrap(it)})
}
/**
* A list of rules applied to the body/payload sent in the POST request to a webhook URL.
*/
public open fun filters(vararg `value`: Any): Unit = filters(`value`.toList())
/**
* Examines the CloudFormation resource and discloses attributes.
*
* @param inspector tree inspector to collect and process attributes.
*/
public override fun inspect(inspector: TreeInspector) {
unwrap(this).inspect(inspector.let(TreeInspector.Companion::unwrap))
}
/**
* The name of the webhook.
*/
public open fun name(): String? = unwrap(this).getName()
/**
* The name of the webhook.
*/
public open fun name(`value`: String) {
unwrap(this).setName(`value`)
}
/**
* Configures a connection between the webhook that was created and the external tool with events
* to be detected.
*/
public open fun registerWithThirdParty(): Any? = unwrap(this).getRegisterWithThirdParty()
/**
* Configures a connection between the webhook that was created and the external tool with events
* to be detected.
*/
public open fun registerWithThirdParty(`value`: Boolean) {
unwrap(this).setRegisterWithThirdParty(`value`)
}
/**
* Configures a connection between the webhook that was created and the external tool with events
* to be detected.
*/
public open fun registerWithThirdParty(`value`: IResolvable) {
unwrap(this).setRegisterWithThirdParty(`value`.let(IResolvable.Companion::unwrap))
}
/**
* The name of the action in a pipeline you want to connect to the webhook.
*/
public open fun targetAction(): String = unwrap(this).getTargetAction()
/**
* The name of the action in a pipeline you want to connect to the webhook.
*/
public open fun targetAction(`value`: String) {
unwrap(this).setTargetAction(`value`)
}
/**
* The name of the pipeline you want to connect to the webhook.
*/
public open fun targetPipeline(): String = unwrap(this).getTargetPipeline()
/**
* The name of the pipeline you want to connect to the webhook.
*/
public open fun targetPipeline(`value`: String) {
unwrap(this).setTargetPipeline(`value`)
}
/**
* The version number of the pipeline to be connected to the trigger request.
*/
public open fun targetPipelineVersion(): Number = unwrap(this).getTargetPipelineVersion()
/**
* The version number of the pipeline to be connected to the trigger request.
*/
public open fun targetPipelineVersion(`value`: Number) {
unwrap(this).setTargetPipelineVersion(`value`)
}
/**
* A fluent builder for [io.cloudshiftdev.awscdk.services.codepipeline.CfnWebhook].
*/
@CdkDslMarker
public interface Builder {
/**
* Supported options are GITHUB_HMAC, IP, and UNAUTHENTICATED.
*
*
* When creating CodePipeline webhooks, do not use your own credentials or reuse the same secret
* token across multiple webhooks. For optimal security, generate a unique secret token for each
* webhook you create. The secret token is an arbitrary string that you provide, which GitHub uses
* to compute and sign the webhook payloads sent to CodePipeline, for protecting the integrity and
* authenticity of the webhook payloads. Using your own credentials or reusing the same token
* across multiple webhooks can lead to security vulnerabilities. > If a secret token was
* provided, it will be redacted in the response.
*
*
* * For information about the authentication scheme implemented by GITHUB_HMAC, see [Securing
* your webhooks](https://docs.aws.amazon.com/https://developer.github.com/webhooks/securing/) on
* the GitHub Developer website.
* * IP rejects webhooks trigger requests unless they originate from an IP address in the IP
* range whitelisted in the authentication configuration.
* * UNAUTHENTICATED accepts all webhook trigger requests regardless of origin.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-authentication)
* @param authentication Supported options are GITHUB_HMAC, IP, and UNAUTHENTICATED.
*/
public fun authentication(authentication: String)
/**
* Properties that configure the authentication applied to incoming webhook trigger requests.
*
* The required properties depend on the authentication type. For GITHUB_HMAC, only the
* `SecretToken` property must be set. For IP, only the `AllowedIPRange` property must be set to a
* valid CIDR range. For UNAUTHENTICATED, no properties can be set.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-authenticationconfiguration)
* @param authenticationConfiguration Properties that configure the authentication applied to
* incoming webhook trigger requests.
*/
public fun authenticationConfiguration(authenticationConfiguration: IResolvable)
/**
* Properties that configure the authentication applied to incoming webhook trigger requests.
*
* The required properties depend on the authentication type. For GITHUB_HMAC, only the
* `SecretToken` property must be set. For IP, only the `AllowedIPRange` property must be set to a
* valid CIDR range. For UNAUTHENTICATED, no properties can be set.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-authenticationconfiguration)
* @param authenticationConfiguration Properties that configure the authentication applied to
* incoming webhook trigger requests.
*/
public
fun authenticationConfiguration(authenticationConfiguration: WebhookAuthConfigurationProperty)
/**
* Properties that configure the authentication applied to incoming webhook trigger requests.
*
* The required properties depend on the authentication type. For GITHUB_HMAC, only the
* `SecretToken` property must be set. For IP, only the `AllowedIPRange` property must be set to a
* valid CIDR range. For UNAUTHENTICATED, no properties can be set.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-authenticationconfiguration)
* @param authenticationConfiguration Properties that configure the authentication applied to
* incoming webhook trigger requests.
*/
@kotlin.Suppress("INAPPLICABLE_JVM_NAME")
@JvmName("0fab646e614c021815fbcc2e077fc03ead501f79f293f303855a27d9f2a24795")
public
fun authenticationConfiguration(authenticationConfiguration: WebhookAuthConfigurationProperty.Builder.() -> Unit)
/**
* A list of rules applied to the body/payload sent in the POST request to a webhook URL.
*
* All defined rules must pass for the request to be accepted and the pipeline started.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-filters)
* @param filters A list of rules applied to the body/payload sent in the POST request to a
* webhook URL.
*/
public fun filters(filters: IResolvable)
/**
* A list of rules applied to the body/payload sent in the POST request to a webhook URL.
*
* All defined rules must pass for the request to be accepted and the pipeline started.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-filters)
* @param filters A list of rules applied to the body/payload sent in the POST request to a
* webhook URL.
*/
public fun filters(filters: List)
/**
* A list of rules applied to the body/payload sent in the POST request to a webhook URL.
*
* All defined rules must pass for the request to be accepted and the pipeline started.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-filters)
* @param filters A list of rules applied to the body/payload sent in the POST request to a
* webhook URL.
*/
public fun filters(vararg filters: Any)
/**
* The name of the webhook.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-name)
* @param name The name of the webhook.
*/
public fun name(name: String)
/**
* Configures a connection between the webhook that was created and the external tool with
* events to be detected.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-registerwiththirdparty)
* @param registerWithThirdParty Configures a connection between the webhook that was created
* and the external tool with events to be detected.
*/
public fun registerWithThirdParty(registerWithThirdParty: Boolean)
/**
* Configures a connection between the webhook that was created and the external tool with
* events to be detected.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-registerwiththirdparty)
* @param registerWithThirdParty Configures a connection between the webhook that was created
* and the external tool with events to be detected.
*/
public fun registerWithThirdParty(registerWithThirdParty: IResolvable)
/**
* The name of the action in a pipeline you want to connect to the webhook.
*
* The action must be from the source (first) stage of the pipeline.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-targetaction)
* @param targetAction The name of the action in a pipeline you want to connect to the webhook.
*/
public fun targetAction(targetAction: String)
/**
* The name of the pipeline you want to connect to the webhook.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-targetpipeline)
* @param targetPipeline The name of the pipeline you want to connect to the webhook.
*/
public fun targetPipeline(targetPipeline: String)
/**
* The version number of the pipeline to be connected to the trigger request.
*
* Required: Yes
*
* Type: Integer
*
* Update requires: [No
* interruption](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/using-cfn-updating-stacks-update-behaviors.html#update-no-interrupt)
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-targetpipelineversion)
* @param targetPipelineVersion The version number of the pipeline to be connected to the
* trigger request.
*/
public fun targetPipelineVersion(targetPipelineVersion: Number)
}
private class BuilderImpl(
scope: SoftwareConstructsConstruct,
id: String,
) : Builder {
private val cdkBuilder: software.amazon.awscdk.services.codepipeline.CfnWebhook.Builder =
software.amazon.awscdk.services.codepipeline.CfnWebhook.Builder.create(scope, id)
/**
* Supported options are GITHUB_HMAC, IP, and UNAUTHENTICATED.
*
*
* When creating CodePipeline webhooks, do not use your own credentials or reuse the same secret
* token across multiple webhooks. For optimal security, generate a unique secret token for each
* webhook you create. The secret token is an arbitrary string that you provide, which GitHub uses
* to compute and sign the webhook payloads sent to CodePipeline, for protecting the integrity and
* authenticity of the webhook payloads. Using your own credentials or reusing the same token
* across multiple webhooks can lead to security vulnerabilities. > If a secret token was
* provided, it will be redacted in the response.
*
*
* * For information about the authentication scheme implemented by GITHUB_HMAC, see [Securing
* your webhooks](https://docs.aws.amazon.com/https://developer.github.com/webhooks/securing/) on
* the GitHub Developer website.
* * IP rejects webhooks trigger requests unless they originate from an IP address in the IP
* range whitelisted in the authentication configuration.
* * UNAUTHENTICATED accepts all webhook trigger requests regardless of origin.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-authentication)
* @param authentication Supported options are GITHUB_HMAC, IP, and UNAUTHENTICATED.
*/
override fun authentication(authentication: String) {
cdkBuilder.authentication(authentication)
}
/**
* Properties that configure the authentication applied to incoming webhook trigger requests.
*
* The required properties depend on the authentication type. For GITHUB_HMAC, only the
* `SecretToken` property must be set. For IP, only the `AllowedIPRange` property must be set to a
* valid CIDR range. For UNAUTHENTICATED, no properties can be set.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-authenticationconfiguration)
* @param authenticationConfiguration Properties that configure the authentication applied to
* incoming webhook trigger requests.
*/
override fun authenticationConfiguration(authenticationConfiguration: IResolvable) {
cdkBuilder.authenticationConfiguration(authenticationConfiguration.let(IResolvable.Companion::unwrap))
}
/**
* Properties that configure the authentication applied to incoming webhook trigger requests.
*
* The required properties depend on the authentication type. For GITHUB_HMAC, only the
* `SecretToken` property must be set. For IP, only the `AllowedIPRange` property must be set to a
* valid CIDR range. For UNAUTHENTICATED, no properties can be set.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-authenticationconfiguration)
* @param authenticationConfiguration Properties that configure the authentication applied to
* incoming webhook trigger requests.
*/
override
fun authenticationConfiguration(authenticationConfiguration: WebhookAuthConfigurationProperty) {
cdkBuilder.authenticationConfiguration(authenticationConfiguration.let(WebhookAuthConfigurationProperty.Companion::unwrap))
}
/**
* Properties that configure the authentication applied to incoming webhook trigger requests.
*
* The required properties depend on the authentication type. For GITHUB_HMAC, only the
* `SecretToken` property must be set. For IP, only the `AllowedIPRange` property must be set to a
* valid CIDR range. For UNAUTHENTICATED, no properties can be set.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-authenticationconfiguration)
* @param authenticationConfiguration Properties that configure the authentication applied to
* incoming webhook trigger requests.
*/
@kotlin.Suppress("INAPPLICABLE_JVM_NAME")
@JvmName("0fab646e614c021815fbcc2e077fc03ead501f79f293f303855a27d9f2a24795")
override
fun authenticationConfiguration(authenticationConfiguration: WebhookAuthConfigurationProperty.Builder.() -> Unit):
Unit =
authenticationConfiguration(WebhookAuthConfigurationProperty(authenticationConfiguration))
/**
* A list of rules applied to the body/payload sent in the POST request to a webhook URL.
*
* All defined rules must pass for the request to be accepted and the pipeline started.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-filters)
* @param filters A list of rules applied to the body/payload sent in the POST request to a
* webhook URL.
*/
override fun filters(filters: IResolvable) {
cdkBuilder.filters(filters.let(IResolvable.Companion::unwrap))
}
/**
* A list of rules applied to the body/payload sent in the POST request to a webhook URL.
*
* All defined rules must pass for the request to be accepted and the pipeline started.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-filters)
* @param filters A list of rules applied to the body/payload sent in the POST request to a
* webhook URL.
*/
override fun filters(filters: List) {
cdkBuilder.filters(filters.map{CdkObjectWrappers.unwrap(it)})
}
/**
* A list of rules applied to the body/payload sent in the POST request to a webhook URL.
*
* All defined rules must pass for the request to be accepted and the pipeline started.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-filters)
* @param filters A list of rules applied to the body/payload sent in the POST request to a
* webhook URL.
*/
override fun filters(vararg filters: Any): Unit = filters(filters.toList())
/**
* The name of the webhook.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-name)
* @param name The name of the webhook.
*/
override fun name(name: String) {
cdkBuilder.name(name)
}
/**
* Configures a connection between the webhook that was created and the external tool with
* events to be detected.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-registerwiththirdparty)
* @param registerWithThirdParty Configures a connection between the webhook that was created
* and the external tool with events to be detected.
*/
override fun registerWithThirdParty(registerWithThirdParty: Boolean) {
cdkBuilder.registerWithThirdParty(registerWithThirdParty)
}
/**
* Configures a connection between the webhook that was created and the external tool with
* events to be detected.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-registerwiththirdparty)
* @param registerWithThirdParty Configures a connection between the webhook that was created
* and the external tool with events to be detected.
*/
override fun registerWithThirdParty(registerWithThirdParty: IResolvable) {
cdkBuilder.registerWithThirdParty(registerWithThirdParty.let(IResolvable.Companion::unwrap))
}
/**
* The name of the action in a pipeline you want to connect to the webhook.
*
* The action must be from the source (first) stage of the pipeline.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-targetaction)
* @param targetAction The name of the action in a pipeline you want to connect to the webhook.
*/
override fun targetAction(targetAction: String) {
cdkBuilder.targetAction(targetAction)
}
/**
* The name of the pipeline you want to connect to the webhook.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-targetpipeline)
* @param targetPipeline The name of the pipeline you want to connect to the webhook.
*/
override fun targetPipeline(targetPipeline: String) {
cdkBuilder.targetPipeline(targetPipeline)
}
/**
* The version number of the pipeline to be connected to the trigger request.
*
* Required: Yes
*
* Type: Integer
*
* Update requires: [No
* interruption](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/using-cfn-updating-stacks-update-behaviors.html#update-no-interrupt)
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codepipeline-webhook.html#cfn-codepipeline-webhook-targetpipelineversion)
* @param targetPipelineVersion The version number of the pipeline to be connected to the
* trigger request.
*/
override fun targetPipelineVersion(targetPipelineVersion: Number) {
cdkBuilder.targetPipelineVersion(targetPipelineVersion)
}
public fun build(): software.amazon.awscdk.services.codepipeline.CfnWebhook = cdkBuilder.build()
}
public companion object {
public val CFN_RESOURCE_TYPE_NAME: String =
software.amazon.awscdk.services.codepipeline.CfnWebhook.CFN_RESOURCE_TYPE_NAME
public operator fun invoke(
scope: CloudshiftdevConstructsConstruct,
id: String,
block: Builder.() -> Unit = {},
): CfnWebhook {
val builderImpl = BuilderImpl(CloudshiftdevConstructsConstruct.unwrap(scope), id)
return CfnWebhook(builderImpl.apply(block).build())
}
internal fun wrap(cdkObject: software.amazon.awscdk.services.codepipeline.CfnWebhook):
CfnWebhook = CfnWebhook(cdkObject)
internal fun unwrap(wrapped: CfnWebhook):
software.amazon.awscdk.services.codepipeline.CfnWebhook = wrapped.cdkObject as
software.amazon.awscdk.services.codepipeline.CfnWebhook
}
/**
* The authentication applied to incoming webhook trigger requests.
*
* Example:
*
* ```
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import io.cloudshiftdev.awscdk.services.codepipeline.*;
* WebhookAuthConfigurationProperty webhookAuthConfigurationProperty =
* WebhookAuthConfigurationProperty.builder()
* .allowedIpRange("allowedIpRange")
* .secretToken("secretToken")
* .build();
* ```
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-codepipeline-webhook-webhookauthconfiguration.html)
*/
public interface WebhookAuthConfigurationProperty {
/**
* The property used to configure acceptance of webhooks in an IP address range.
*
* For IP, only the `AllowedIPRange` property must be set. This property must be set to a valid
* CIDR range.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-codepipeline-webhook-webhookauthconfiguration.html#cfn-codepipeline-webhook-webhookauthconfiguration-allowediprange)
*/
public fun allowedIpRange(): String? = unwrap(this).getAllowedIpRange()
/**
* The property used to configure GitHub authentication. For GITHUB_HMAC, only the `SecretToken`
* property must be set.
*
*
* When creating CodePipeline webhooks, do not use your own credentials or reuse the same secret
* token across multiple webhooks. For optimal security, generate a unique secret token for each
* webhook you create. The secret token is an arbitrary string that you provide, which GitHub uses
* to compute and sign the webhook payloads sent to CodePipeline, for protecting the integrity and
* authenticity of the webhook payloads. Using your own credentials or reusing the same token
* across multiple webhooks can lead to security vulnerabilities. > If a secret token was
* provided, it will be redacted in the response.
*
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-codepipeline-webhook-webhookauthconfiguration.html#cfn-codepipeline-webhook-webhookauthconfiguration-secrettoken)
*/
public fun secretToken(): String? = unwrap(this).getSecretToken()
/**
* A builder for [WebhookAuthConfigurationProperty]
*/
@CdkDslMarker
public interface Builder {
/**
* @param allowedIpRange The property used to configure acceptance of webhooks in an IP
* address range.
* For IP, only the `AllowedIPRange` property must be set. This property must be set to a
* valid CIDR range.
*/
public fun allowedIpRange(allowedIpRange: String)
/**
* @param secretToken The property used to configure GitHub authentication. For GITHUB_HMAC,
* only the `SecretToken` property must be set.
*
* When creating CodePipeline webhooks, do not use your own credentials or reuse the same
* secret token across multiple webhooks. For optimal security, generate a unique secret token
* for each webhook you create. The secret token is an arbitrary string that you provide, which
* GitHub uses to compute and sign the webhook payloads sent to CodePipeline, for protecting the
* integrity and authenticity of the webhook payloads. Using your own credentials or reusing the
* same token across multiple webhooks can lead to security vulnerabilities. > If a secret
* token was provided, it will be redacted in the response.
*/
public fun secretToken(secretToken: String)
}
private class BuilderImpl : Builder {
private val cdkBuilder:
software.amazon.awscdk.services.codepipeline.CfnWebhook.WebhookAuthConfigurationProperty.Builder
=
software.amazon.awscdk.services.codepipeline.CfnWebhook.WebhookAuthConfigurationProperty.builder()
/**
* @param allowedIpRange The property used to configure acceptance of webhooks in an IP
* address range.
* For IP, only the `AllowedIPRange` property must be set. This property must be set to a
* valid CIDR range.
*/
override fun allowedIpRange(allowedIpRange: String) {
cdkBuilder.allowedIpRange(allowedIpRange)
}
/**
* @param secretToken The property used to configure GitHub authentication. For GITHUB_HMAC,
* only the `SecretToken` property must be set.
*
* When creating CodePipeline webhooks, do not use your own credentials or reuse the same
* secret token across multiple webhooks. For optimal security, generate a unique secret token
* for each webhook you create. The secret token is an arbitrary string that you provide, which
* GitHub uses to compute and sign the webhook payloads sent to CodePipeline, for protecting the
* integrity and authenticity of the webhook payloads. Using your own credentials or reusing the
* same token across multiple webhooks can lead to security vulnerabilities. > If a secret
* token was provided, it will be redacted in the response.
*/
override fun secretToken(secretToken: String) {
cdkBuilder.secretToken(secretToken)
}
public fun build():
software.amazon.awscdk.services.codepipeline.CfnWebhook.WebhookAuthConfigurationProperty =
cdkBuilder.build()
}
private class Wrapper(
cdkObject: software.amazon.awscdk.services.codepipeline.CfnWebhook.WebhookAuthConfigurationProperty,
) : CdkObject(cdkObject),
WebhookAuthConfigurationProperty {
/**
* The property used to configure acceptance of webhooks in an IP address range.
*
* For IP, only the `AllowedIPRange` property must be set. This property must be set to a
* valid CIDR range.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-codepipeline-webhook-webhookauthconfiguration.html#cfn-codepipeline-webhook-webhookauthconfiguration-allowediprange)
*/
override fun allowedIpRange(): String? = unwrap(this).getAllowedIpRange()
/**
* The property used to configure GitHub authentication. For GITHUB_HMAC, only the
* `SecretToken` property must be set.
*
*
* When creating CodePipeline webhooks, do not use your own credentials or reuse the same
* secret token across multiple webhooks. For optimal security, generate a unique secret token
* for each webhook you create. The secret token is an arbitrary string that you provide, which
* GitHub uses to compute and sign the webhook payloads sent to CodePipeline, for protecting the
* integrity and authenticity of the webhook payloads. Using your own credentials or reusing the
* same token across multiple webhooks can lead to security vulnerabilities. > If a secret
* token was provided, it will be redacted in the response.
*
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-codepipeline-webhook-webhookauthconfiguration.html#cfn-codepipeline-webhook-webhookauthconfiguration-secrettoken)
*/
override fun secretToken(): String? = unwrap(this).getSecretToken()
}
public companion object {
public operator fun invoke(block: Builder.() -> Unit = {}): WebhookAuthConfigurationProperty {
val builderImpl = BuilderImpl()
return Wrapper(builderImpl.apply(block).build())
}
internal
fun wrap(cdkObject: software.amazon.awscdk.services.codepipeline.CfnWebhook.WebhookAuthConfigurationProperty):
WebhookAuthConfigurationProperty = CdkObjectWrappers.wrap(cdkObject) as?
WebhookAuthConfigurationProperty ?: Wrapper(cdkObject)
internal fun unwrap(wrapped: WebhookAuthConfigurationProperty):
software.amazon.awscdk.services.codepipeline.CfnWebhook.WebhookAuthConfigurationProperty =
(wrapped as CdkObject).cdkObject as
software.amazon.awscdk.services.codepipeline.CfnWebhook.WebhookAuthConfigurationProperty
}
}
/**
* The event criteria that specify when a webhook notification is sent to your URL.
*
* Example:
*
* ```
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import io.cloudshiftdev.awscdk.services.codepipeline.*;
* WebhookFilterRuleProperty webhookFilterRuleProperty = WebhookFilterRuleProperty.builder()
* .jsonPath("jsonPath")
* // the properties below are optional
* .matchEquals("matchEquals")
* .build();
* ```
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-codepipeline-webhook-webhookfilterrule.html)
*/
public interface WebhookFilterRuleProperty {
/**
* A JsonPath expression that is applied to the body/payload of the webhook.
*
* The value selected by the JsonPath expression must match the value specified in the
* `MatchEquals` field. Otherwise, the request is ignored. For more information, see [Java JsonPath
* implementation](https://docs.aws.amazon.com/https://github.com/json-path/JsonPath) in GitHub.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-codepipeline-webhook-webhookfilterrule.html#cfn-codepipeline-webhook-webhookfilterrule-jsonpath)
*/
public fun jsonPath(): String
/**
* The value selected by the `JsonPath` expression must match what is supplied in the
* `MatchEquals` field.
*
* Otherwise, the request is ignored. Properties from the target action configuration can be
* included as placeholders in this value by surrounding the action configuration key with curly
* brackets. For example, if the value supplied here is "refs/heads/{Branch}" and the target action
* has an action configuration property called "Branch" with a value of "main", the `MatchEquals`
* value is evaluated as "refs/heads/main". For a list of action configuration properties for
* built-in action types, see [Pipeline Structure Reference Action
* Requirements](https://docs.aws.amazon.com/codepipeline/latest/userguide/reference-pipeline-structure.html#action-requirements)
* .
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-codepipeline-webhook-webhookfilterrule.html#cfn-codepipeline-webhook-webhookfilterrule-matchequals)
*/
public fun matchEquals(): String? = unwrap(this).getMatchEquals()
/**
* A builder for [WebhookFilterRuleProperty]
*/
@CdkDslMarker
public interface Builder {
/**
* @param jsonPath A JsonPath expression that is applied to the body/payload of the webhook.
* The value selected by the JsonPath expression must match the value specified in the
* `MatchEquals` field. Otherwise, the request is ignored. For more information, see [Java
* JsonPath implementation](https://docs.aws.amazon.com/https://github.com/json-path/JsonPath) in
* GitHub.
*/
public fun jsonPath(jsonPath: String)
/**
* @param matchEquals The value selected by the `JsonPath` expression must match what is
* supplied in the `MatchEquals` field.
* Otherwise, the request is ignored. Properties from the target action configuration can be
* included as placeholders in this value by surrounding the action configuration key with curly
* brackets. For example, if the value supplied here is "refs/heads/{Branch}" and the target
* action has an action configuration property called "Branch" with a value of "main", the
* `MatchEquals` value is evaluated as "refs/heads/main". For a list of action configuration
* properties for built-in action types, see [Pipeline Structure Reference Action
* Requirements](https://docs.aws.amazon.com/codepipeline/latest/userguide/reference-pipeline-structure.html#action-requirements)
* .
*/
public fun matchEquals(matchEquals: String)
}
private class BuilderImpl : Builder {
private val cdkBuilder:
software.amazon.awscdk.services.codepipeline.CfnWebhook.WebhookFilterRuleProperty.Builder
=
software.amazon.awscdk.services.codepipeline.CfnWebhook.WebhookFilterRuleProperty.builder()
/**
* @param jsonPath A JsonPath expression that is applied to the body/payload of the webhook.
* The value selected by the JsonPath expression must match the value specified in the
* `MatchEquals` field. Otherwise, the request is ignored. For more information, see [Java
* JsonPath implementation](https://docs.aws.amazon.com/https://github.com/json-path/JsonPath) in
* GitHub.
*/
override fun jsonPath(jsonPath: String) {
cdkBuilder.jsonPath(jsonPath)
}
/**
* @param matchEquals The value selected by the `JsonPath` expression must match what is
* supplied in the `MatchEquals` field.
* Otherwise, the request is ignored. Properties from the target action configuration can be
* included as placeholders in this value by surrounding the action configuration key with curly
* brackets. For example, if the value supplied here is "refs/heads/{Branch}" and the target
* action has an action configuration property called "Branch" with a value of "main", the
* `MatchEquals` value is evaluated as "refs/heads/main". For a list of action configuration
* properties for built-in action types, see [Pipeline Structure Reference Action
* Requirements](https://docs.aws.amazon.com/codepipeline/latest/userguide/reference-pipeline-structure.html#action-requirements)
* .
*/
override fun matchEquals(matchEquals: String) {
cdkBuilder.matchEquals(matchEquals)
}
public fun build():
software.amazon.awscdk.services.codepipeline.CfnWebhook.WebhookFilterRuleProperty =
cdkBuilder.build()
}
private class Wrapper(
cdkObject: software.amazon.awscdk.services.codepipeline.CfnWebhook.WebhookFilterRuleProperty,
) : CdkObject(cdkObject),
WebhookFilterRuleProperty {
/**
* A JsonPath expression that is applied to the body/payload of the webhook.
*
* The value selected by the JsonPath expression must match the value specified in the
* `MatchEquals` field. Otherwise, the request is ignored. For more information, see [Java
* JsonPath implementation](https://docs.aws.amazon.com/https://github.com/json-path/JsonPath) in
* GitHub.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-codepipeline-webhook-webhookfilterrule.html#cfn-codepipeline-webhook-webhookfilterrule-jsonpath)
*/
override fun jsonPath(): String = unwrap(this).getJsonPath()
/**
* The value selected by the `JsonPath` expression must match what is supplied in the
* `MatchEquals` field.
*
* Otherwise, the request is ignored. Properties from the target action configuration can be
* included as placeholders in this value by surrounding the action configuration key with curly
* brackets. For example, if the value supplied here is "refs/heads/{Branch}" and the target
* action has an action configuration property called "Branch" with a value of "main", the
* `MatchEquals` value is evaluated as "refs/heads/main". For a list of action configuration
* properties for built-in action types, see [Pipeline Structure Reference Action
* Requirements](https://docs.aws.amazon.com/codepipeline/latest/userguide/reference-pipeline-structure.html#action-requirements)
* .
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-codepipeline-webhook-webhookfilterrule.html#cfn-codepipeline-webhook-webhookfilterrule-matchequals)
*/
override fun matchEquals(): String? = unwrap(this).getMatchEquals()
}
public companion object {
public operator fun invoke(block: Builder.() -> Unit = {}): WebhookFilterRuleProperty {
val builderImpl = BuilderImpl()
return Wrapper(builderImpl.apply(block).build())
}
internal
fun wrap(cdkObject: software.amazon.awscdk.services.codepipeline.CfnWebhook.WebhookFilterRuleProperty):
WebhookFilterRuleProperty = CdkObjectWrappers.wrap(cdkObject) as?
WebhookFilterRuleProperty ?: Wrapper(cdkObject)
internal fun unwrap(wrapped: WebhookFilterRuleProperty):
software.amazon.awscdk.services.codepipeline.CfnWebhook.WebhookFilterRuleProperty =
(wrapped as CdkObject).cdkObject as
software.amazon.awscdk.services.codepipeline.CfnWebhook.WebhookFilterRuleProperty
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy