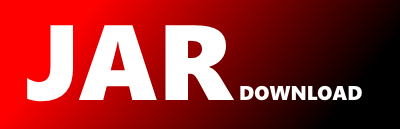
io.cloudshiftdev.awscdk.services.cognito.OAuthSettings.kt Maven / Gradle / Ivy
@file:Suppress("RedundantVisibilityModifier","RedundantUnitReturnType","RemoveRedundantQualifierName","unused","UnusedImport","ClassName","REDUNDANT_PROJECTION","DEPRECATION")
package io.cloudshiftdev.awscdk.services.cognito
import io.cloudshiftdev.awscdk.common.CdkDslMarker
import io.cloudshiftdev.awscdk.common.CdkObject
import io.cloudshiftdev.awscdk.common.CdkObjectWrappers
import kotlin.String
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* OAuth settings to configure the interaction between the app and this client.
*
* Example:
*
* ```
* UserPool pool = new UserPool(this, "Pool");
* ResourceServerScope readOnlyScope =
* ResourceServerScope.Builder.create().scopeName("read").scopeDescription("Read-only access").build();
* ResourceServerScope fullAccessScope =
* ResourceServerScope.Builder.create().scopeName("*").scopeDescription("Full access").build();
* UserPoolResourceServer userServer = pool.addResourceServer("ResourceServer",
* UserPoolResourceServerOptions.builder()
* .identifier("users")
* .scopes(List.of(readOnlyScope, fullAccessScope))
* .build());
* UserPoolClient readOnlyClient = pool.addClient("read-only-client",
* UserPoolClientOptions.builder()
* // ...
* .oAuth(OAuthSettings.builder()
* // ...
* .scopes(List.of(OAuthScope.resourceServer(userServer, readOnlyScope)))
* .build())
* .build());
* UserPoolClient fullAccessClient = pool.addClient("full-access-client",
* UserPoolClientOptions.builder()
* // ...
* .oAuth(OAuthSettings.builder()
* // ...
* .scopes(List.of(OAuthScope.resourceServer(userServer, fullAccessScope)))
* .build())
* .build());
* ```
*/
public interface OAuthSettings {
/**
* List of allowed redirect URLs for the identity providers.
*
* Default: - ['https://example.com'] if either authorizationCodeGrant or implicitCodeGrant flows
* are enabled, no callback URLs otherwise.
*/
public fun callbackUrls(): List = unwrap(this).getCallbackUrls() ?: emptyList()
/**
* The default redirect URI. Must be in the `callbackUrls` list.
*
* A redirect URI must:
*
* * Be an absolute URI
* * Be registered with the authorization server.
* * Not include a fragment component.
*
* Default: - no default redirect URI
*
* @see https://tools.ietf.org/html/rfc6749#section-3.1.2
* Amazon Cognito requires HTTPS over HTTP except for http://localhost for testing purposes only.
* App callback URLs such as myapp://example are also supported.
*/
public fun defaultRedirectUri(): String? = unwrap(this).getDefaultRedirectUri()
/**
* OAuth flows that are allowed with this client.
*
* Default: {authorizationCodeGrant:true,implicitCodeGrant:true}
*
* [Documentation]( - the 'Allowed OAuth Flows' section at
* https://docs.aws.amazon.com/cognito/latest/developerguide/cognito-user-pools-app-idp-settings.html)
*/
public fun flows(): OAuthFlows? = unwrap(this).getFlows()?.let(OAuthFlows::wrap)
/**
* List of allowed logout URLs for the identity providers.
*
* Default: - no logout URLs
*/
public fun logoutUrls(): List = unwrap(this).getLogoutUrls() ?: emptyList()
/**
* OAuth scopes that are allowed with this client.
*
* Default:
* [OAuthScope.PHONE,OAuthScope.EMAIL,OAuthScope.OPENID,OAuthScope.PROFILE,OAuthScope.COGNITO_ADMIN]
*
* [Documentation](https://docs.aws.amazon.com/cognito/latest/developerguide/cognito-user-pools-app-idp-settings.html)
*/
public fun scopes(): List = unwrap(this).getScopes()?.map(OAuthScope::wrap) ?:
emptyList()
/**
* A builder for [OAuthSettings]
*/
@CdkDslMarker
public interface Builder {
/**
* @param callbackUrls List of allowed redirect URLs for the identity providers.
*/
public fun callbackUrls(callbackUrls: List)
/**
* @param callbackUrls List of allowed redirect URLs for the identity providers.
*/
public fun callbackUrls(vararg callbackUrls: String)
/**
* @param defaultRedirectUri The default redirect URI. Must be in the `callbackUrls` list.
* A redirect URI must:
*
* * Be an absolute URI
* * Be registered with the authorization server.
* * Not include a fragment component.
*/
public fun defaultRedirectUri(defaultRedirectUri: String)
/**
* @param flows OAuth flows that are allowed with this client.
*/
public fun flows(flows: OAuthFlows)
/**
* @param flows OAuth flows that are allowed with this client.
*/
@kotlin.Suppress("INAPPLICABLE_JVM_NAME")
@JvmName("cb93ec242576c1797aed90a7fd0d4beb2c24332026aa722bda799a62aa9f77fd")
public fun flows(flows: OAuthFlows.Builder.() -> Unit)
/**
* @param logoutUrls List of allowed logout URLs for the identity providers.
*/
public fun logoutUrls(logoutUrls: List)
/**
* @param logoutUrls List of allowed logout URLs for the identity providers.
*/
public fun logoutUrls(vararg logoutUrls: String)
/**
* @param scopes OAuth scopes that are allowed with this client.
*/
public fun scopes(scopes: List)
/**
* @param scopes OAuth scopes that are allowed with this client.
*/
public fun scopes(vararg scopes: OAuthScope)
}
private class BuilderImpl : Builder {
private val cdkBuilder: software.amazon.awscdk.services.cognito.OAuthSettings.Builder =
software.amazon.awscdk.services.cognito.OAuthSettings.builder()
/**
* @param callbackUrls List of allowed redirect URLs for the identity providers.
*/
override fun callbackUrls(callbackUrls: List) {
cdkBuilder.callbackUrls(callbackUrls)
}
/**
* @param callbackUrls List of allowed redirect URLs for the identity providers.
*/
override fun callbackUrls(vararg callbackUrls: String): Unit =
callbackUrls(callbackUrls.toList())
/**
* @param defaultRedirectUri The default redirect URI. Must be in the `callbackUrls` list.
* A redirect URI must:
*
* * Be an absolute URI
* * Be registered with the authorization server.
* * Not include a fragment component.
*/
override fun defaultRedirectUri(defaultRedirectUri: String) {
cdkBuilder.defaultRedirectUri(defaultRedirectUri)
}
/**
* @param flows OAuth flows that are allowed with this client.
*/
override fun flows(flows: OAuthFlows) {
cdkBuilder.flows(flows.let(OAuthFlows.Companion::unwrap))
}
/**
* @param flows OAuth flows that are allowed with this client.
*/
@kotlin.Suppress("INAPPLICABLE_JVM_NAME")
@JvmName("cb93ec242576c1797aed90a7fd0d4beb2c24332026aa722bda799a62aa9f77fd")
override fun flows(flows: OAuthFlows.Builder.() -> Unit): Unit = flows(OAuthFlows(flows))
/**
* @param logoutUrls List of allowed logout URLs for the identity providers.
*/
override fun logoutUrls(logoutUrls: List) {
cdkBuilder.logoutUrls(logoutUrls)
}
/**
* @param logoutUrls List of allowed logout URLs for the identity providers.
*/
override fun logoutUrls(vararg logoutUrls: String): Unit = logoutUrls(logoutUrls.toList())
/**
* @param scopes OAuth scopes that are allowed with this client.
*/
override fun scopes(scopes: List) {
cdkBuilder.scopes(scopes.map(OAuthScope.Companion::unwrap))
}
/**
* @param scopes OAuth scopes that are allowed with this client.
*/
override fun scopes(vararg scopes: OAuthScope): Unit = scopes(scopes.toList())
public fun build(): software.amazon.awscdk.services.cognito.OAuthSettings = cdkBuilder.build()
}
private class Wrapper(
cdkObject: software.amazon.awscdk.services.cognito.OAuthSettings,
) : CdkObject(cdkObject),
OAuthSettings {
/**
* List of allowed redirect URLs for the identity providers.
*
* Default: - ['https://example.com'] if either authorizationCodeGrant or implicitCodeGrant
* flows are enabled, no callback URLs otherwise.
*/
override fun callbackUrls(): List = unwrap(this).getCallbackUrls() ?: emptyList()
/**
* The default redirect URI. Must be in the `callbackUrls` list.
*
* A redirect URI must:
*
* * Be an absolute URI
* * Be registered with the authorization server.
* * Not include a fragment component.
*
* Default: - no default redirect URI
*
* @see https://tools.ietf.org/html/rfc6749#section-3.1.2
* Amazon Cognito requires HTTPS over HTTP except for http://localhost for testing purposes
* only.
* App callback URLs such as myapp://example are also supported.
*/
override fun defaultRedirectUri(): String? = unwrap(this).getDefaultRedirectUri()
/**
* OAuth flows that are allowed with this client.
*
* Default: {authorizationCodeGrant:true,implicitCodeGrant:true}
*
* [Documentation]( - the 'Allowed OAuth Flows' section at
* https://docs.aws.amazon.com/cognito/latest/developerguide/cognito-user-pools-app-idp-settings.html)
*/
override fun flows(): OAuthFlows? = unwrap(this).getFlows()?.let(OAuthFlows::wrap)
/**
* List of allowed logout URLs for the identity providers.
*
* Default: - no logout URLs
*/
override fun logoutUrls(): List = unwrap(this).getLogoutUrls() ?: emptyList()
/**
* OAuth scopes that are allowed with this client.
*
* Default:
* [OAuthScope.PHONE,OAuthScope.EMAIL,OAuthScope.OPENID,OAuthScope.PROFILE,OAuthScope.COGNITO_ADMIN]
*
* [Documentation](https://docs.aws.amazon.com/cognito/latest/developerguide/cognito-user-pools-app-idp-settings.html)
*/
override fun scopes(): List = unwrap(this).getScopes()?.map(OAuthScope::wrap) ?:
emptyList()
}
public companion object {
public operator fun invoke(block: Builder.() -> Unit = {}): OAuthSettings {
val builderImpl = BuilderImpl()
return Wrapper(builderImpl.apply(block).build())
}
internal fun wrap(cdkObject: software.amazon.awscdk.services.cognito.OAuthSettings):
OAuthSettings = CdkObjectWrappers.wrap(cdkObject) as? OAuthSettings ?: Wrapper(cdkObject)
internal fun unwrap(wrapped: OAuthSettings):
software.amazon.awscdk.services.cognito.OAuthSettings = (wrapped as CdkObject).cdkObject as
software.amazon.awscdk.services.cognito.OAuthSettings
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy