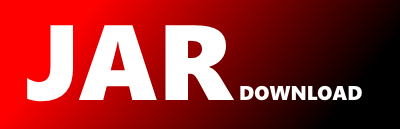
io.cloudshiftdev.awscdk.services.securitylake.CfnDataLakeProps.kt Maven / Gradle / Ivy
@file:Suppress("RedundantVisibilityModifier","RedundantUnitReturnType","RemoveRedundantQualifierName","unused","UnusedImport","ClassName","REDUNDANT_PROJECTION","DEPRECATION")
package io.cloudshiftdev.awscdk.services.securitylake
import io.cloudshiftdev.awscdk.CfnTag
import io.cloudshiftdev.awscdk.IResolvable
import io.cloudshiftdev.awscdk.common.CdkDslMarker
import io.cloudshiftdev.awscdk.common.CdkObject
import io.cloudshiftdev.awscdk.common.CdkObjectWrappers
import kotlin.Any
import kotlin.String
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Properties for defining a `CfnDataLake`.
*
* Example:
*
* ```
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import io.cloudshiftdev.awscdk.services.securitylake.*;
* CfnDataLakeProps cfnDataLakeProps = CfnDataLakeProps.builder()
* .encryptionConfiguration(EncryptionConfigurationProperty.builder()
* .kmsKeyId("kmsKeyId")
* .build())
* .lifecycleConfiguration(LifecycleConfigurationProperty.builder()
* .expiration(ExpirationProperty.builder()
* .days(123)
* .build())
* .transitions(List.of(TransitionsProperty.builder()
* .days(123)
* .storageClass("storageClass")
* .build()))
* .build())
* .metaStoreManagerRoleArn("metaStoreManagerRoleArn")
* .replicationConfiguration(ReplicationConfigurationProperty.builder()
* .regions(List.of("regions"))
* .roleArn("roleArn")
* .build())
* .tags(List.of(CfnTag.builder()
* .key("key")
* .value("value")
* .build()))
* .build();
* ```
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-securitylake-datalake.html)
*/
public interface CfnDataLakeProps {
/**
* Provides encryption details of the Amazon Security Lake object.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-securitylake-datalake.html#cfn-securitylake-datalake-encryptionconfiguration)
*/
public fun encryptionConfiguration(): Any? = unwrap(this).getEncryptionConfiguration()
/**
* You can customize Security Lake to store data in your preferred AWS Regions for your preferred
* amount of time.
*
* Lifecycle management can help you comply with different compliance requirements. For more
* details, see [Lifecycle
* management](https://docs.aws.amazon.com//security-lake/latest/userguide/lifecycle-management.html)
* in the Amazon Security Lake User Guide.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-securitylake-datalake.html#cfn-securitylake-datalake-lifecycleconfiguration)
*/
public fun lifecycleConfiguration(): Any? = unwrap(this).getLifecycleConfiguration()
/**
* The Amazon Resource Name (ARN) used to create and update the AWS Glue table.
*
* This table contains partitions generated by the ingestion and normalization of AWS log sources
* and custom sources.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-securitylake-datalake.html#cfn-securitylake-datalake-metastoremanagerrolearn)
*/
public fun metaStoreManagerRoleArn(): String? = unwrap(this).getMetaStoreManagerRoleArn()
/**
* Provides replication details of Amazon Security Lake object.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-securitylake-datalake.html#cfn-securitylake-datalake-replicationconfiguration)
*/
public fun replicationConfiguration(): Any? = unwrap(this).getReplicationConfiguration()
/**
* An array of objects, one for each tag to associate with the data lake configuration.
*
* For each tag, you must specify both a tag key and a tag value. A tag value cannot be null, but
* it can be an empty string.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-securitylake-datalake.html#cfn-securitylake-datalake-tags)
*/
public fun tags(): List = unwrap(this).getTags()?.map(CfnTag::wrap) ?: emptyList()
/**
* A builder for [CfnDataLakeProps]
*/
@CdkDslMarker
public interface Builder {
/**
* @param encryptionConfiguration Provides encryption details of the Amazon Security Lake
* object.
*/
public fun encryptionConfiguration(encryptionConfiguration: IResolvable)
/**
* @param encryptionConfiguration Provides encryption details of the Amazon Security Lake
* object.
*/
public
fun encryptionConfiguration(encryptionConfiguration: CfnDataLake.EncryptionConfigurationProperty)
/**
* @param encryptionConfiguration Provides encryption details of the Amazon Security Lake
* object.
*/
@kotlin.Suppress("INAPPLICABLE_JVM_NAME")
@JvmName("a2442f58b3b01717546f85581c27351d34307c1fc218aa20cdbbfa5aed72aef0")
public
fun encryptionConfiguration(encryptionConfiguration: CfnDataLake.EncryptionConfigurationProperty.Builder.() -> Unit)
/**
* @param lifecycleConfiguration You can customize Security Lake to store data in your preferred
* AWS Regions for your preferred amount of time.
* Lifecycle management can help you comply with different compliance requirements. For more
* details, see [Lifecycle
* management](https://docs.aws.amazon.com//security-lake/latest/userguide/lifecycle-management.html)
* in the Amazon Security Lake User Guide.
*/
public fun lifecycleConfiguration(lifecycleConfiguration: IResolvable)
/**
* @param lifecycleConfiguration You can customize Security Lake to store data in your preferred
* AWS Regions for your preferred amount of time.
* Lifecycle management can help you comply with different compliance requirements. For more
* details, see [Lifecycle
* management](https://docs.aws.amazon.com//security-lake/latest/userguide/lifecycle-management.html)
* in the Amazon Security Lake User Guide.
*/
public
fun lifecycleConfiguration(lifecycleConfiguration: CfnDataLake.LifecycleConfigurationProperty)
/**
* @param lifecycleConfiguration You can customize Security Lake to store data in your preferred
* AWS Regions for your preferred amount of time.
* Lifecycle management can help you comply with different compliance requirements. For more
* details, see [Lifecycle
* management](https://docs.aws.amazon.com//security-lake/latest/userguide/lifecycle-management.html)
* in the Amazon Security Lake User Guide.
*/
@kotlin.Suppress("INAPPLICABLE_JVM_NAME")
@JvmName("39020733219eabebae180a595797416552420ba0991e920504c36fda6c86b2b6")
public
fun lifecycleConfiguration(lifecycleConfiguration: CfnDataLake.LifecycleConfigurationProperty.Builder.() -> Unit)
/**
* @param metaStoreManagerRoleArn The Amazon Resource Name (ARN) used to create and update the
* AWS Glue table.
* This table contains partitions generated by the ingestion and normalization of AWS log
* sources and custom sources.
*/
public fun metaStoreManagerRoleArn(metaStoreManagerRoleArn: String)
/**
* @param replicationConfiguration Provides replication details of Amazon Security Lake object.
*/
public fun replicationConfiguration(replicationConfiguration: IResolvable)
/**
* @param replicationConfiguration Provides replication details of Amazon Security Lake object.
*/
public
fun replicationConfiguration(replicationConfiguration: CfnDataLake.ReplicationConfigurationProperty)
/**
* @param replicationConfiguration Provides replication details of Amazon Security Lake object.
*/
@kotlin.Suppress("INAPPLICABLE_JVM_NAME")
@JvmName("c59cae038283dae77140399f42e6a487456dd1ca71bdb37389c3ca252a7bce5e")
public
fun replicationConfiguration(replicationConfiguration: CfnDataLake.ReplicationConfigurationProperty.Builder.() -> Unit)
/**
* @param tags An array of objects, one for each tag to associate with the data lake
* configuration.
* For each tag, you must specify both a tag key and a tag value. A tag value cannot be null,
* but it can be an empty string.
*/
public fun tags(tags: List)
/**
* @param tags An array of objects, one for each tag to associate with the data lake
* configuration.
* For each tag, you must specify both a tag key and a tag value. A tag value cannot be null,
* but it can be an empty string.
*/
public fun tags(vararg tags: CfnTag)
}
private class BuilderImpl : Builder {
private val cdkBuilder: software.amazon.awscdk.services.securitylake.CfnDataLakeProps.Builder =
software.amazon.awscdk.services.securitylake.CfnDataLakeProps.builder()
/**
* @param encryptionConfiguration Provides encryption details of the Amazon Security Lake
* object.
*/
override fun encryptionConfiguration(encryptionConfiguration: IResolvable) {
cdkBuilder.encryptionConfiguration(encryptionConfiguration.let(IResolvable.Companion::unwrap))
}
/**
* @param encryptionConfiguration Provides encryption details of the Amazon Security Lake
* object.
*/
override
fun encryptionConfiguration(encryptionConfiguration: CfnDataLake.EncryptionConfigurationProperty) {
cdkBuilder.encryptionConfiguration(encryptionConfiguration.let(CfnDataLake.EncryptionConfigurationProperty.Companion::unwrap))
}
/**
* @param encryptionConfiguration Provides encryption details of the Amazon Security Lake
* object.
*/
@kotlin.Suppress("INAPPLICABLE_JVM_NAME")
@JvmName("a2442f58b3b01717546f85581c27351d34307c1fc218aa20cdbbfa5aed72aef0")
override
fun encryptionConfiguration(encryptionConfiguration: CfnDataLake.EncryptionConfigurationProperty.Builder.() -> Unit):
Unit =
encryptionConfiguration(CfnDataLake.EncryptionConfigurationProperty(encryptionConfiguration))
/**
* @param lifecycleConfiguration You can customize Security Lake to store data in your preferred
* AWS Regions for your preferred amount of time.
* Lifecycle management can help you comply with different compliance requirements. For more
* details, see [Lifecycle
* management](https://docs.aws.amazon.com//security-lake/latest/userguide/lifecycle-management.html)
* in the Amazon Security Lake User Guide.
*/
override fun lifecycleConfiguration(lifecycleConfiguration: IResolvable) {
cdkBuilder.lifecycleConfiguration(lifecycleConfiguration.let(IResolvable.Companion::unwrap))
}
/**
* @param lifecycleConfiguration You can customize Security Lake to store data in your preferred
* AWS Regions for your preferred amount of time.
* Lifecycle management can help you comply with different compliance requirements. For more
* details, see [Lifecycle
* management](https://docs.aws.amazon.com//security-lake/latest/userguide/lifecycle-management.html)
* in the Amazon Security Lake User Guide.
*/
override
fun lifecycleConfiguration(lifecycleConfiguration: CfnDataLake.LifecycleConfigurationProperty) {
cdkBuilder.lifecycleConfiguration(lifecycleConfiguration.let(CfnDataLake.LifecycleConfigurationProperty.Companion::unwrap))
}
/**
* @param lifecycleConfiguration You can customize Security Lake to store data in your preferred
* AWS Regions for your preferred amount of time.
* Lifecycle management can help you comply with different compliance requirements. For more
* details, see [Lifecycle
* management](https://docs.aws.amazon.com//security-lake/latest/userguide/lifecycle-management.html)
* in the Amazon Security Lake User Guide.
*/
@kotlin.Suppress("INAPPLICABLE_JVM_NAME")
@JvmName("39020733219eabebae180a595797416552420ba0991e920504c36fda6c86b2b6")
override
fun lifecycleConfiguration(lifecycleConfiguration: CfnDataLake.LifecycleConfigurationProperty.Builder.() -> Unit):
Unit =
lifecycleConfiguration(CfnDataLake.LifecycleConfigurationProperty(lifecycleConfiguration))
/**
* @param metaStoreManagerRoleArn The Amazon Resource Name (ARN) used to create and update the
* AWS Glue table.
* This table contains partitions generated by the ingestion and normalization of AWS log
* sources and custom sources.
*/
override fun metaStoreManagerRoleArn(metaStoreManagerRoleArn: String) {
cdkBuilder.metaStoreManagerRoleArn(metaStoreManagerRoleArn)
}
/**
* @param replicationConfiguration Provides replication details of Amazon Security Lake object.
*/
override fun replicationConfiguration(replicationConfiguration: IResolvable) {
cdkBuilder.replicationConfiguration(replicationConfiguration.let(IResolvable.Companion::unwrap))
}
/**
* @param replicationConfiguration Provides replication details of Amazon Security Lake object.
*/
override
fun replicationConfiguration(replicationConfiguration: CfnDataLake.ReplicationConfigurationProperty) {
cdkBuilder.replicationConfiguration(replicationConfiguration.let(CfnDataLake.ReplicationConfigurationProperty.Companion::unwrap))
}
/**
* @param replicationConfiguration Provides replication details of Amazon Security Lake object.
*/
@kotlin.Suppress("INAPPLICABLE_JVM_NAME")
@JvmName("c59cae038283dae77140399f42e6a487456dd1ca71bdb37389c3ca252a7bce5e")
override
fun replicationConfiguration(replicationConfiguration: CfnDataLake.ReplicationConfigurationProperty.Builder.() -> Unit):
Unit =
replicationConfiguration(CfnDataLake.ReplicationConfigurationProperty(replicationConfiguration))
/**
* @param tags An array of objects, one for each tag to associate with the data lake
* configuration.
* For each tag, you must specify both a tag key and a tag value. A tag value cannot be null,
* but it can be an empty string.
*/
override fun tags(tags: List) {
cdkBuilder.tags(tags.map(CfnTag.Companion::unwrap))
}
/**
* @param tags An array of objects, one for each tag to associate with the data lake
* configuration.
* For each tag, you must specify both a tag key and a tag value. A tag value cannot be null,
* but it can be an empty string.
*/
override fun tags(vararg tags: CfnTag): Unit = tags(tags.toList())
public fun build(): software.amazon.awscdk.services.securitylake.CfnDataLakeProps =
cdkBuilder.build()
}
private class Wrapper(
cdkObject: software.amazon.awscdk.services.securitylake.CfnDataLakeProps,
) : CdkObject(cdkObject),
CfnDataLakeProps {
/**
* Provides encryption details of the Amazon Security Lake object.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-securitylake-datalake.html#cfn-securitylake-datalake-encryptionconfiguration)
*/
override fun encryptionConfiguration(): Any? = unwrap(this).getEncryptionConfiguration()
/**
* You can customize Security Lake to store data in your preferred AWS Regions for your
* preferred amount of time.
*
* Lifecycle management can help you comply with different compliance requirements. For more
* details, see [Lifecycle
* management](https://docs.aws.amazon.com//security-lake/latest/userguide/lifecycle-management.html)
* in the Amazon Security Lake User Guide.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-securitylake-datalake.html#cfn-securitylake-datalake-lifecycleconfiguration)
*/
override fun lifecycleConfiguration(): Any? = unwrap(this).getLifecycleConfiguration()
/**
* The Amazon Resource Name (ARN) used to create and update the AWS Glue table.
*
* This table contains partitions generated by the ingestion and normalization of AWS log
* sources and custom sources.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-securitylake-datalake.html#cfn-securitylake-datalake-metastoremanagerrolearn)
*/
override fun metaStoreManagerRoleArn(): String? = unwrap(this).getMetaStoreManagerRoleArn()
/**
* Provides replication details of Amazon Security Lake object.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-securitylake-datalake.html#cfn-securitylake-datalake-replicationconfiguration)
*/
override fun replicationConfiguration(): Any? = unwrap(this).getReplicationConfiguration()
/**
* An array of objects, one for each tag to associate with the data lake configuration.
*
* For each tag, you must specify both a tag key and a tag value. A tag value cannot be null,
* but it can be an empty string.
*
* [Documentation](http://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-securitylake-datalake.html#cfn-securitylake-datalake-tags)
*/
override fun tags(): List = unwrap(this).getTags()?.map(CfnTag::wrap) ?: emptyList()
}
public companion object {
public operator fun invoke(block: Builder.() -> Unit = {}): CfnDataLakeProps {
val builderImpl = BuilderImpl()
return Wrapper(builderImpl.apply(block).build())
}
internal fun wrap(cdkObject: software.amazon.awscdk.services.securitylake.CfnDataLakeProps):
CfnDataLakeProps = CdkObjectWrappers.wrap(cdkObject) as? CfnDataLakeProps ?:
Wrapper(cdkObject)
internal fun unwrap(wrapped: CfnDataLakeProps):
software.amazon.awscdk.services.securitylake.CfnDataLakeProps = (wrapped as
CdkObject).cdkObject as software.amazon.awscdk.services.securitylake.CfnDataLakeProps
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy