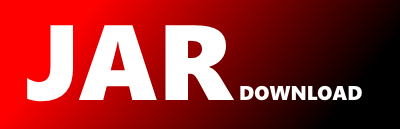
io.cloudshiftdev.awscdk.services.stepfunctions.tasks.SageMakerCreateModelProps.kt Maven / Gradle / Ivy
@file:Suppress("RedundantVisibilityModifier","RedundantUnitReturnType","RemoveRedundantQualifierName","unused","UnusedImport","ClassName","REDUNDANT_PROJECTION","DEPRECATION")
package io.cloudshiftdev.awscdk.services.stepfunctions.tasks
import io.cloudshiftdev.awscdk.Duration
import io.cloudshiftdev.awscdk.common.CdkDslMarker
import io.cloudshiftdev.awscdk.common.CdkObject
import io.cloudshiftdev.awscdk.common.CdkObjectWrappers
import io.cloudshiftdev.awscdk.services.ec2.IVpc
import io.cloudshiftdev.awscdk.services.ec2.SubnetSelection
import io.cloudshiftdev.awscdk.services.iam.IRole
import io.cloudshiftdev.awscdk.services.stepfunctions.Credentials
import io.cloudshiftdev.awscdk.services.stepfunctions.IntegrationPattern
import io.cloudshiftdev.awscdk.services.stepfunctions.TaskInput
import io.cloudshiftdev.awscdk.services.stepfunctions.TaskStateBaseProps
import io.cloudshiftdev.awscdk.services.stepfunctions.Timeout
import kotlin.Any
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Properties for creating an Amazon SageMaker model.
*
* Example:
*
* ```
* SageMakerCreateModel.Builder.create(this, "Sagemaker")
* .modelName("MyModel")
* .primaryContainer(ContainerDefinition.Builder.create()
* .image(DockerImage.fromJsonExpression(JsonPath.stringAt("$.Model.imageName")))
* .mode(Mode.SINGLE_MODEL)
* .modelS3Location(S3Location.fromJsonExpression("$.TrainingJob.ModelArtifacts.S3ModelArtifacts"))
* .build())
* .build();
* ```
*
* [Documentation](https://docs.aws.amazon.com/step-functions/latest/dg/connect-sagemaker.html)
*/
public interface SageMakerCreateModelProps : TaskStateBaseProps {
/**
* Specifies the containers in the inference pipeline.
*
* Default: - None
*/
public fun containers(): List =
unwrap(this).getContainers()?.map(IContainerDefinition::wrap) ?: emptyList()
/**
* Isolates the model container.
*
* No inbound or outbound network calls can be made to or from the model container.
*
* Default: false
*/
public fun enableNetworkIsolation(): Boolean? = unwrap(this).getEnableNetworkIsolation()
/**
* The name of the new model.
*/
public fun modelName(): String
/**
* The definition of the primary docker image containing inference code, associated artifacts, and
* custom environment map that the inference code uses when the model is deployed for predictions.
*/
public fun primaryContainer(): IContainerDefinition
/**
* An execution role that you can pass in a CreateModel API request.
*
* Default: - a role will be created.
*/
public fun role(): IRole? = unwrap(this).getRole()?.let(IRole::wrap)
/**
* The subnets of the VPC to which the hosted model is connected (Note this parameter is only used
* when VPC is provided).
*
* Default: - Private Subnets are selected
*/
public fun subnetSelection(): SubnetSelection? =
unwrap(this).getSubnetSelection()?.let(SubnetSelection::wrap)
/**
* Tags to be applied to the model.
*
* Default: - No tags
*/
public fun tags(): TaskInput? = unwrap(this).getTags()?.let(TaskInput::wrap)
/**
* The VPC that is accessible by the hosted model.
*
* Default: - None
*/
public fun vpc(): IVpc? = unwrap(this).getVpc()?.let(IVpc::wrap)
/**
* A builder for [SageMakerCreateModelProps]
*/
@CdkDslMarker
public interface Builder {
/**
* @param comment An optional description for this state.
*/
public fun comment(comment: String)
/**
* @param containers Specifies the containers in the inference pipeline.
*/
public fun containers(containers: List)
/**
* @param containers Specifies the containers in the inference pipeline.
*/
public fun containers(vararg containers: IContainerDefinition)
/**
* @param credentials Credentials for an IAM Role that the State Machine assumes for executing
* the task.
* This enables cross-account resource invocations.
*/
public fun credentials(credentials: Credentials)
/**
* @param credentials Credentials for an IAM Role that the State Machine assumes for executing
* the task.
* This enables cross-account resource invocations.
*/
@kotlin.Suppress("INAPPLICABLE_JVM_NAME")
@JvmName("daee7ed6dc5cb17c876e351d8fd2a736c1169ad0a2f0470cdbd832749f89cb4f")
public fun credentials(credentials: Credentials.Builder.() -> Unit)
/**
* @param enableNetworkIsolation Isolates the model container.
* No inbound or outbound network calls can be made to or from the model container.
*/
public fun enableNetworkIsolation(enableNetworkIsolation: Boolean)
/**
* @param heartbeat Timeout for the heartbeat.
* @deprecated use `heartbeatTimeout`
*/
@Deprecated(message = "deprecated in CDK")
public fun heartbeat(heartbeat: Duration)
/**
* @param heartbeatTimeout Timeout for the heartbeat.
* [disable-awslint:duration-prop-type] is needed because all props interface in
* aws-stepfunctions-tasks extend this interface
*/
public fun heartbeatTimeout(heartbeatTimeout: Timeout)
/**
* @param inputPath JSONPath expression to select part of the state to be the input to this
* state.
* May also be the special value JsonPath.DISCARD, which will cause the effective
* input to be the empty object {}.
*/
public fun inputPath(inputPath: String)
/**
* @param integrationPattern AWS Step Functions integrates with services directly in the Amazon
* States Language.
* You can control these AWS services using service integration patterns.
*
* Depending on the AWS Service, the Service Integration Pattern availability will vary.
*/
public fun integrationPattern(integrationPattern: IntegrationPattern)
/**
* @param modelName The name of the new model.
*/
public fun modelName(modelName: String)
/**
* @param outputPath JSONPath expression to select select a portion of the state output to pass
* to the next state.
* May also be the special value JsonPath.DISCARD, which will cause the effective
* output to be the empty object {}.
*/
public fun outputPath(outputPath: String)
/**
* @param primaryContainer The definition of the primary docker image containing inference code,
* associated artifacts, and custom environment map that the inference code uses when the model is
* deployed for predictions.
*/
public fun primaryContainer(primaryContainer: IContainerDefinition)
/**
* @param resultPath JSONPath expression to indicate where to inject the state's output.
* May also be the special value JsonPath.DISCARD, which will cause the state's
* input to become its output.
*/
public fun resultPath(resultPath: String)
/**
* @param resultSelector The JSON that will replace the state's raw result and become the
* effective result before ResultPath is applied.
* You can use ResultSelector to create a payload with values that are static
* or selected from the state's raw result.
*/
public fun resultSelector(resultSelector: Map)
/**
* @param role An execution role that you can pass in a CreateModel API request.
*/
public fun role(role: IRole)
/**
* @param stateName Optional name for this state.
*/
public fun stateName(stateName: String)
/**
* @param subnetSelection The subnets of the VPC to which the hosted model is connected (Note
* this parameter is only used when VPC is provided).
*/
public fun subnetSelection(subnetSelection: SubnetSelection)
/**
* @param subnetSelection The subnets of the VPC to which the hosted model is connected (Note
* this parameter is only used when VPC is provided).
*/
@kotlin.Suppress("INAPPLICABLE_JVM_NAME")
@JvmName("1222fd18edff5e49cf637348eb0533b90c9677cf03896b91a9a3f6d5e7c67d70")
public fun subnetSelection(subnetSelection: SubnetSelection.Builder.() -> Unit)
/**
* @param tags Tags to be applied to the model.
*/
public fun tags(tags: TaskInput)
/**
* @param taskTimeout Timeout for the task.
* [disable-awslint:duration-prop-type] is needed because all props interface in
* aws-stepfunctions-tasks extend this interface
*/
public fun taskTimeout(taskTimeout: Timeout)
/**
* @param timeout Timeout for the task.
* @deprecated use `taskTimeout`
*/
@Deprecated(message = "deprecated in CDK")
public fun timeout(timeout: Duration)
/**
* @param vpc The VPC that is accessible by the hosted model.
*/
public fun vpc(vpc: IVpc)
}
private class BuilderImpl : Builder {
private val cdkBuilder:
software.amazon.awscdk.services.stepfunctions.tasks.SageMakerCreateModelProps.Builder =
software.amazon.awscdk.services.stepfunctions.tasks.SageMakerCreateModelProps.builder()
/**
* @param comment An optional description for this state.
*/
override fun comment(comment: String) {
cdkBuilder.comment(comment)
}
/**
* @param containers Specifies the containers in the inference pipeline.
*/
override fun containers(containers: List) {
cdkBuilder.containers(containers.map(IContainerDefinition.Companion::unwrap))
}
/**
* @param containers Specifies the containers in the inference pipeline.
*/
override fun containers(vararg containers: IContainerDefinition): Unit =
containers(containers.toList())
/**
* @param credentials Credentials for an IAM Role that the State Machine assumes for executing
* the task.
* This enables cross-account resource invocations.
*/
override fun credentials(credentials: Credentials) {
cdkBuilder.credentials(credentials.let(Credentials.Companion::unwrap))
}
/**
* @param credentials Credentials for an IAM Role that the State Machine assumes for executing
* the task.
* This enables cross-account resource invocations.
*/
@kotlin.Suppress("INAPPLICABLE_JVM_NAME")
@JvmName("daee7ed6dc5cb17c876e351d8fd2a736c1169ad0a2f0470cdbd832749f89cb4f")
override fun credentials(credentials: Credentials.Builder.() -> Unit): Unit =
credentials(Credentials(credentials))
/**
* @param enableNetworkIsolation Isolates the model container.
* No inbound or outbound network calls can be made to or from the model container.
*/
override fun enableNetworkIsolation(enableNetworkIsolation: Boolean) {
cdkBuilder.enableNetworkIsolation(enableNetworkIsolation)
}
/**
* @param heartbeat Timeout for the heartbeat.
* @deprecated use `heartbeatTimeout`
*/
@Deprecated(message = "deprecated in CDK")
override fun heartbeat(heartbeat: Duration) {
cdkBuilder.heartbeat(heartbeat.let(Duration.Companion::unwrap))
}
/**
* @param heartbeatTimeout Timeout for the heartbeat.
* [disable-awslint:duration-prop-type] is needed because all props interface in
* aws-stepfunctions-tasks extend this interface
*/
override fun heartbeatTimeout(heartbeatTimeout: Timeout) {
cdkBuilder.heartbeatTimeout(heartbeatTimeout.let(Timeout.Companion::unwrap))
}
/**
* @param inputPath JSONPath expression to select part of the state to be the input to this
* state.
* May also be the special value JsonPath.DISCARD, which will cause the effective
* input to be the empty object {}.
*/
override fun inputPath(inputPath: String) {
cdkBuilder.inputPath(inputPath)
}
/**
* @param integrationPattern AWS Step Functions integrates with services directly in the Amazon
* States Language.
* You can control these AWS services using service integration patterns.
*
* Depending on the AWS Service, the Service Integration Pattern availability will vary.
*/
override fun integrationPattern(integrationPattern: IntegrationPattern) {
cdkBuilder.integrationPattern(integrationPattern.let(IntegrationPattern.Companion::unwrap))
}
/**
* @param modelName The name of the new model.
*/
override fun modelName(modelName: String) {
cdkBuilder.modelName(modelName)
}
/**
* @param outputPath JSONPath expression to select select a portion of the state output to pass
* to the next state.
* May also be the special value JsonPath.DISCARD, which will cause the effective
* output to be the empty object {}.
*/
override fun outputPath(outputPath: String) {
cdkBuilder.outputPath(outputPath)
}
/**
* @param primaryContainer The definition of the primary docker image containing inference code,
* associated artifacts, and custom environment map that the inference code uses when the model is
* deployed for predictions.
*/
override fun primaryContainer(primaryContainer: IContainerDefinition) {
cdkBuilder.primaryContainer(primaryContainer.let(IContainerDefinition.Companion::unwrap))
}
/**
* @param resultPath JSONPath expression to indicate where to inject the state's output.
* May also be the special value JsonPath.DISCARD, which will cause the state's
* input to become its output.
*/
override fun resultPath(resultPath: String) {
cdkBuilder.resultPath(resultPath)
}
/**
* @param resultSelector The JSON that will replace the state's raw result and become the
* effective result before ResultPath is applied.
* You can use ResultSelector to create a payload with values that are static
* or selected from the state's raw result.
*/
override fun resultSelector(resultSelector: Map) {
cdkBuilder.resultSelector(resultSelector.mapValues{CdkObjectWrappers.unwrap(it.value)})
}
/**
* @param role An execution role that you can pass in a CreateModel API request.
*/
override fun role(role: IRole) {
cdkBuilder.role(role.let(IRole.Companion::unwrap))
}
/**
* @param stateName Optional name for this state.
*/
override fun stateName(stateName: String) {
cdkBuilder.stateName(stateName)
}
/**
* @param subnetSelection The subnets of the VPC to which the hosted model is connected (Note
* this parameter is only used when VPC is provided).
*/
override fun subnetSelection(subnetSelection: SubnetSelection) {
cdkBuilder.subnetSelection(subnetSelection.let(SubnetSelection.Companion::unwrap))
}
/**
* @param subnetSelection The subnets of the VPC to which the hosted model is connected (Note
* this parameter is only used when VPC is provided).
*/
@kotlin.Suppress("INAPPLICABLE_JVM_NAME")
@JvmName("1222fd18edff5e49cf637348eb0533b90c9677cf03896b91a9a3f6d5e7c67d70")
override fun subnetSelection(subnetSelection: SubnetSelection.Builder.() -> Unit): Unit =
subnetSelection(SubnetSelection(subnetSelection))
/**
* @param tags Tags to be applied to the model.
*/
override fun tags(tags: TaskInput) {
cdkBuilder.tags(tags.let(TaskInput.Companion::unwrap))
}
/**
* @param taskTimeout Timeout for the task.
* [disable-awslint:duration-prop-type] is needed because all props interface in
* aws-stepfunctions-tasks extend this interface
*/
override fun taskTimeout(taskTimeout: Timeout) {
cdkBuilder.taskTimeout(taskTimeout.let(Timeout.Companion::unwrap))
}
/**
* @param timeout Timeout for the task.
* @deprecated use `taskTimeout`
*/
@Deprecated(message = "deprecated in CDK")
override fun timeout(timeout: Duration) {
cdkBuilder.timeout(timeout.let(Duration.Companion::unwrap))
}
/**
* @param vpc The VPC that is accessible by the hosted model.
*/
override fun vpc(vpc: IVpc) {
cdkBuilder.vpc(vpc.let(IVpc.Companion::unwrap))
}
public fun build():
software.amazon.awscdk.services.stepfunctions.tasks.SageMakerCreateModelProps =
cdkBuilder.build()
}
private class Wrapper(
cdkObject: software.amazon.awscdk.services.stepfunctions.tasks.SageMakerCreateModelProps,
) : CdkObject(cdkObject),
SageMakerCreateModelProps {
/**
* An optional description for this state.
*
* Default: - No comment
*/
override fun comment(): String? = unwrap(this).getComment()
/**
* Specifies the containers in the inference pipeline.
*
* Default: - None
*/
override fun containers(): List =
unwrap(this).getContainers()?.map(IContainerDefinition::wrap) ?: emptyList()
/**
* Credentials for an IAM Role that the State Machine assumes for executing the task.
*
* This enables cross-account resource invocations.
*
* Default: - None (Task is executed using the State Machine's execution role)
*
* [Documentation](https://docs.aws.amazon.com/step-functions/latest/dg/concepts-access-cross-acct-resources.html)
*/
override fun credentials(): Credentials? = unwrap(this).getCredentials()?.let(Credentials::wrap)
/**
* Isolates the model container.
*
* No inbound or outbound network calls can be made to or from the model container.
*
* Default: false
*/
override fun enableNetworkIsolation(): Boolean? = unwrap(this).getEnableNetworkIsolation()
/**
* (deprecated) Timeout for the heartbeat.
*
* Default: - None
*
* @deprecated use `heartbeatTimeout`
*/
@Deprecated(message = "deprecated in CDK")
override fun heartbeat(): Duration? = unwrap(this).getHeartbeat()?.let(Duration::wrap)
/**
* Timeout for the heartbeat.
*
* [disable-awslint:duration-prop-type] is needed because all props interface in
* aws-stepfunctions-tasks extend this interface
*
* Default: - None
*/
override fun heartbeatTimeout(): Timeout? =
unwrap(this).getHeartbeatTimeout()?.let(Timeout::wrap)
/**
* JSONPath expression to select part of the state to be the input to this state.
*
* May also be the special value JsonPath.DISCARD, which will cause the effective
* input to be the empty object {}.
*
* Default: - The entire task input (JSON path '$')
*/
override fun inputPath(): String? = unwrap(this).getInputPath()
/**
* AWS Step Functions integrates with services directly in the Amazon States Language.
*
* You can control these AWS services using service integration patterns.
*
* Depending on the AWS Service, the Service Integration Pattern availability will vary.
*
* Default: - `IntegrationPattern.REQUEST_RESPONSE` for most tasks.
* `IntegrationPattern.RUN_JOB` for the following exceptions:
* `BatchSubmitJob`, `EmrAddStep`, `EmrCreateCluster`, `EmrTerminationCluster`, and
* `EmrContainersStartJobRun`.
*
* [Documentation](https://docs.aws.amazon.com/step-functions/latest/dg/connect-supported-services.html)
*/
override fun integrationPattern(): IntegrationPattern? =
unwrap(this).getIntegrationPattern()?.let(IntegrationPattern::wrap)
/**
* The name of the new model.
*/
override fun modelName(): String = unwrap(this).getModelName()
/**
* JSONPath expression to select select a portion of the state output to pass to the next state.
*
* May also be the special value JsonPath.DISCARD, which will cause the effective
* output to be the empty object {}.
*
* Default: - The entire JSON node determined by the state input, the task result,
* and resultPath is passed to the next state (JSON path '$')
*/
override fun outputPath(): String? = unwrap(this).getOutputPath()
/**
* The definition of the primary docker image containing inference code, associated artifacts,
* and custom environment map that the inference code uses when the model is deployed for
* predictions.
*/
override fun primaryContainer(): IContainerDefinition =
unwrap(this).getPrimaryContainer().let(IContainerDefinition::wrap)
/**
* JSONPath expression to indicate where to inject the state's output.
*
* May also be the special value JsonPath.DISCARD, which will cause the state's
* input to become its output.
*
* Default: - Replaces the entire input with the result (JSON path '$')
*/
override fun resultPath(): String? = unwrap(this).getResultPath()
/**
* The JSON that will replace the state's raw result and become the effective result before
* ResultPath is applied.
*
* You can use ResultSelector to create a payload with values that are static
* or selected from the state's raw result.
*
* Default: - None
*
* [Documentation](https://docs.aws.amazon.com/step-functions/latest/dg/input-output-inputpath-params.html#input-output-resultselector)
*/
override fun resultSelector(): Map = unwrap(this).getResultSelector() ?: emptyMap()
/**
* An execution role that you can pass in a CreateModel API request.
*
* Default: - a role will be created.
*/
override fun role(): IRole? = unwrap(this).getRole()?.let(IRole::wrap)
/**
* Optional name for this state.
*
* Default: - The construct ID will be used as state name
*/
override fun stateName(): String? = unwrap(this).getStateName()
/**
* The subnets of the VPC to which the hosted model is connected (Note this parameter is only
* used when VPC is provided).
*
* Default: - Private Subnets are selected
*/
override fun subnetSelection(): SubnetSelection? =
unwrap(this).getSubnetSelection()?.let(SubnetSelection::wrap)
/**
* Tags to be applied to the model.
*
* Default: - No tags
*/
override fun tags(): TaskInput? = unwrap(this).getTags()?.let(TaskInput::wrap)
/**
* Timeout for the task.
*
* [disable-awslint:duration-prop-type] is needed because all props interface in
* aws-stepfunctions-tasks extend this interface
*
* Default: - None
*/
override fun taskTimeout(): Timeout? = unwrap(this).getTaskTimeout()?.let(Timeout::wrap)
/**
* (deprecated) Timeout for the task.
*
* Default: - None
*
* @deprecated use `taskTimeout`
*/
@Deprecated(message = "deprecated in CDK")
override fun timeout(): Duration? = unwrap(this).getTimeout()?.let(Duration::wrap)
/**
* The VPC that is accessible by the hosted model.
*
* Default: - None
*/
override fun vpc(): IVpc? = unwrap(this).getVpc()?.let(IVpc::wrap)
}
public companion object {
public operator fun invoke(block: Builder.() -> Unit = {}): SageMakerCreateModelProps {
val builderImpl = BuilderImpl()
return Wrapper(builderImpl.apply(block).build())
}
internal
fun wrap(cdkObject: software.amazon.awscdk.services.stepfunctions.tasks.SageMakerCreateModelProps):
SageMakerCreateModelProps = CdkObjectWrappers.wrap(cdkObject) as? SageMakerCreateModelProps
?: Wrapper(cdkObject)
internal fun unwrap(wrapped: SageMakerCreateModelProps):
software.amazon.awscdk.services.stepfunctions.tasks.SageMakerCreateModelProps = (wrapped as
CdkObject).cdkObject as
software.amazon.awscdk.services.stepfunctions.tasks.SageMakerCreateModelProps
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy