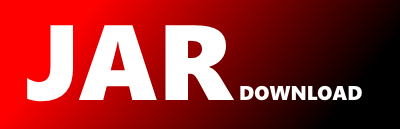
io.cloudslang.samples.controlactions.BranchActions Maven / Gradle / Ivy
/*******************************************************************************
* (c) Copyright 2014 Hewlett-Packard Development Company, L.P.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Apache License v2.0 which accompany this distribution.
*
* The Apache License is available at
* http://www.apache.org/licenses/LICENSE-2.0
*
*******************************************************************************/
package io.cloudslang.samples.controlactions;
import io.cloudslang.score.api.EndBranchDataContainer;
import io.cloudslang.score.lang.ExecutionRuntimeServices;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* User: wahnonm
* Date: 14/08/14
* Time: 10:36
*/
public class BranchActions {
public static final String STEP_POSITION = "stepPosition";
public static final String EXECUTION_PLAN_ID = "executionPlanId";
public static final String BRANCH_RESULTS = "branchResults";
public static final String BRANCH_CONTEXTS = "branchContexts";
public static final String PARALLEL_EXECUTION_PLAN_IDS = "parallelExecutionPlanIds";
@SuppressWarnings("unused")
public void split(ExecutionRuntimeServices executionRuntimeServices, Long stepPosition, String executionPlanId){
executionRuntimeServices.addBranch(stepPosition, executionPlanId, new HashMap());
}
@SuppressWarnings("unused")
public void splitWithContext(ExecutionRuntimeServices executionRuntimeServices,
Map executionContext,
String flowUuid,
Map context,
List inputKeysFromParentContext){
Map initialContext = new HashMap<>();
initialContext.putAll(context);
if (inputKeysFromParentContext != null) {
for (String inputKey : inputKeysFromParentContext) {
initialContext.put(inputKey, executionContext.get(inputKey));
}
}
executionRuntimeServices.addBranch(0L, flowUuid, initialContext);
}
@SuppressWarnings("unused")
public void join(ExecutionRuntimeServices executionRuntimeServices,Map executionContext){
List branches = executionRuntimeServices.getFinishedChildBranchesData();
for (EndBranchDataContainer branch : branches) {
Map branchContext = branch.getContexts();
executionContext.putAll(branchContext);
}
}
@SuppressWarnings("unused")
public void joinBranches(ExecutionRuntimeServices executionRuntimeServices, Map executionContext){
List branches = executionRuntimeServices.getFinishedChildBranchesData();
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy