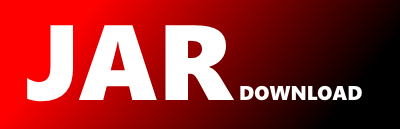
org.jclouds.compute.strategy.InitializeRunScriptOnNodeOrPlaceInBadMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-compute Show documentation
Show all versions of jclouds-compute Show documentation
jclouds components to access compute providers
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jclouds.compute.strategy;
import static com.google.common.base.Preconditions.checkNotNull;
import java.util.Map;
import java.util.concurrent.Callable;
import javax.inject.Inject;
import org.jclouds.compute.callables.RunScriptOnNode;
import org.jclouds.compute.domain.NodeMetadata;
import org.jclouds.compute.options.RunScriptOptions;
import org.jclouds.scriptbuilder.domain.Statement;
import com.google.common.base.Objects;
import com.google.inject.assistedinject.Assisted;
/**
*
* @author Adrian Cole
*/
public class InitializeRunScriptOnNodeOrPlaceInBadMap implements Callable {
public interface Factory {
Callable create(NodeMetadata node, Statement script, RunScriptOptions options,
Map badNodes);
}
private final RunScriptOnNode.Factory runScriptOnNodeFactory;
private final Statement script;
private final Map badNodes;
private final NodeMetadata node;
private final RunScriptOptions options;
@Inject
InitializeRunScriptOnNodeOrPlaceInBadMap(RunScriptOnNode.Factory runScriptOnNodeFactory,
@Assisted NodeMetadata node, @Assisted Statement script, @Assisted RunScriptOptions options,
@Assisted Map badNodes) {
this.runScriptOnNodeFactory = checkNotNull(runScriptOnNodeFactory, "runScriptOnNodeFactory");
this.script = checkNotNull(script, "script");
this.badNodes = checkNotNull(badNodes, "badNodes");
this.node = checkNotNull(node, "node");
this.options = checkNotNull(options, "options");
}
@Override
public RunScriptOnNode call() throws Exception {
try {
return runScriptOnNodeFactory.create(node, script, options).init();
} catch (Exception e) {
badNodes.put(node, e);
return null;
}
}
@Override
public String toString() {
return Objects.toStringHelper(this).add("node", node).add("options", options).toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy