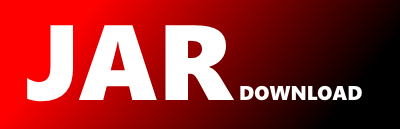
org.jclouds.net.domain.IpPermission Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jclouds-compute Show documentation
Show all versions of jclouds-compute Show documentation
jclouds components to access compute providers
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jclouds.net.domain;
import static com.google.common.base.Objects.equal;
import static com.google.common.base.Preconditions.checkArgument;
import static com.google.common.base.Preconditions.checkNotNull;
import static com.google.common.collect.Iterables.transform;
import static org.jclouds.util.Strings2.isCidrFormat;
import java.util.Set;
import com.google.common.annotations.Beta;
import com.google.common.base.Function;
import com.google.common.base.Objects;
import com.google.common.base.Objects.ToStringHelper;
import com.google.common.collect.ImmutableMultimap;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.Iterables;
import com.google.common.collect.LinkedHashMultimap;
import com.google.common.collect.Multimap;
import com.google.common.collect.Sets;
/**
* Ingress access to a destination protocol on particular ports by source, which could be an ip
* range (cidrblock), set of explicit security group ids in the current tenant, or security group
* names in another tenant.
*
* @author Adrian Cole
* @see IpPermissions
*/
@Beta
public class IpPermission implements Comparable {
public static Builder builder() {
return new Builder();
}
public static class Builder {
private IpProtocol ipProtocol;
private int fromPort;
private int toPort;
private Multimap tenantIdGroupNamePairs = LinkedHashMultimap.create();
private Set groupIds = Sets.newLinkedHashSet();
private Set cidrBlocks = Sets.newLinkedHashSet();
/**
*
* @see IpPermission#getIpProtocol()
*/
public Builder ipProtocol(IpProtocol ipProtocol) {
this.ipProtocol = ipProtocol;
return this;
}
/**
*
* @see IpPermission#getFromPort()
*/
public Builder fromPort(int fromPort) {
this.fromPort = fromPort;
return this;
}
/**
*
* @see IpPermission#getToPort()
*/
public Builder toPort(int toPort) {
this.toPort = toPort;
return this;
}
/**
* @see IpPermission#getTenantIdGroupNamePairs()
*/
public Builder tenantIdGroupNamePair(String tenantId, String groupName) {
this.tenantIdGroupNamePairs.put(tenantId, groupName);
return this;
}
/**
* @see IpPermission#getTenantIdGroupNamePairs()
*/
public Builder tenantIdGroupNamePairs(Multimap tenantIdGroupNamePairs) {
this.tenantIdGroupNamePairs.putAll(tenantIdGroupNamePairs);
return this;
}
/**
* @see IpPermission#getCidrBlocks()
*/
public Builder cidrBlock(String cidrBlock) {
checkArgument(isCidrFormat(cidrBlock), "cidrBlock %s is not a valid CIDR", cidrBlock);
this.cidrBlocks.add(cidrBlock);
return this;
}
/**
* @see IpPermission#getCidrBlocks()
*/
public Builder cidrBlocks(Iterable cidrBlocks) {
Iterables.addAll(this.cidrBlocks, transform(cidrBlocks,
new Function() {
@Override
public String apply(String input) {
checkArgument(isCidrFormat(input),
"input %s is not a valid CIDR",
input);
return input;
}
}));
return this;
}
/**
* @see IpPermission#getGroupIds()
*/
public Builder groupId(String groupId) {
this.groupIds.add(groupId);
return this;
}
/**
* @see IpPermission#getGroupIds()
*/
public Builder groupIds(Iterable groupIds) {
Iterables.addAll(this.groupIds, groupIds);
return this;
}
public IpPermission build() {
return new IpPermission(ipProtocol, fromPort, toPort, tenantIdGroupNamePairs, groupIds, cidrBlocks);
}
}
private final int fromPort;
private final int toPort;
private final Multimap tenantIdGroupNamePairs;
private final Set groupIds;
private final IpProtocol ipProtocol;
private final Set cidrBlocks;
public IpPermission(IpProtocol ipProtocol, int fromPort, int toPort,
Multimap tenantIdGroupNamePairs, Iterable groupIds, Iterable cidrBlocks) {
this.fromPort = fromPort;
this.toPort = toPort;
this.tenantIdGroupNamePairs = ImmutableMultimap.copyOf(checkNotNull(tenantIdGroupNamePairs,
"tenantIdGroupNamePairs"));
this.ipProtocol = checkNotNull(ipProtocol, "ipProtocol");
this.groupIds = ImmutableSet.copyOf(checkNotNull(groupIds, "groupIds"));
this.cidrBlocks = ImmutableSet.copyOf(checkNotNull(cidrBlocks, "cidrBlocks"));
}
/**
* {@inheritDoc}
*/
public int compareTo(IpPermission o) {
return (this == o) ? 0 : getIpProtocol().compareTo(o.getIpProtocol());
}
/**
* destination IP protocol
*/
public IpProtocol getIpProtocol() {
return ipProtocol;
}
/**
* Start of destination port range for the TCP and UDP protocols, or an ICMP type number. An ICMP
* type number of -1 indicates a wildcard (i.e., any ICMP type number).
*/
public int getFromPort() {
return fromPort;
}
/**
* End of destination port range for the TCP and UDP protocols, or an ICMP code. An ICMP code of
* -1 indicates a wildcard (i.e., any ICMP code).
*/
public int getToPort() {
return toPort;
}
/**
* source of traffic allowed is on basis of another group in a tenant, as opposed to by cidr
*/
public Multimap getTenantIdGroupNamePairs() {
return tenantIdGroupNamePairs;
}
/**
* source of traffic allowed is on basis of another groupid in the same tenant
*/
public Set getGroupIds() {
return groupIds;
}
/**
* source of traffic is a cidrRange
*/
public Set getCidrBlocks() {
return cidrBlocks;
}
@Override
public boolean equals(Object o) {
if (this == o)
return true;
// allow subtypes
if (o == null || !(o instanceof IpPermission))
return false;
IpPermission that = IpPermission.class.cast(o);
return equal(this.ipProtocol, that.ipProtocol) && equal(this.fromPort, that.fromPort)
&& equal(this.toPort, that.toPort) && equal(this.tenantIdGroupNamePairs, that.tenantIdGroupNamePairs)
&& equal(this.groupIds, that.groupIds) && equal(this.cidrBlocks, that.cidrBlocks);
}
@Override
public int hashCode() {
return Objects.hashCode(ipProtocol, fromPort, toPort, tenantIdGroupNamePairs, groupIds, cidrBlocks);
}
@Override
public String toString() {
return string().toString();
}
protected ToStringHelper string() {
return Objects.toStringHelper("").add("ipProtocol", ipProtocol).add("fromPort", fromPort).add("toPort", toPort)
.add("tenantIdGroupNamePairs", tenantIdGroupNamePairs).add("groupIds", groupIds).add("cidrBlocks",
cidrBlocks);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy