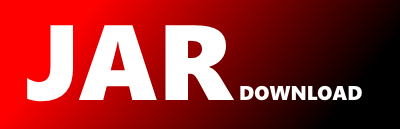
cloudstate.entity.proto Maven / Gradle / Ivy
// Copyright 2019 Lightbend Inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
// gRPC interface for Event Sourced Entity user functions.
syntax = "proto3";
package cloudstate;
// Any is used so that domain events defined according to the functions business domain can be embedded inside
// the protocol.
import "google/protobuf/any.proto";
import "google/protobuf/empty.proto";
import "google/protobuf/descriptor.proto";
option java_package = "io.cloudstate.protocol";
// A reply to the sender.
message Reply {
// The reply payload
google.protobuf.Any payload = 1;
}
// Forwards handling of this request to another entity.
message Forward {
// The name of the service to forward to.
string service_name = 1;
// The name of the command.
string command_name = 2;
// The payload.
google.protobuf.Any payload = 3;
}
// An action for the client
message ClientAction {
oneof action {
// Send a reply
Reply reply = 1;
// Forward to another entity
Forward forward = 2;
// Send a failure to the client
Failure failure = 3;
}
}
// A side effect to be done after this command is handled.
message SideEffect {
// The name of the service to perform the side effect on.
string service_name = 1;
// The name of the command.
string command_name = 2;
// The payload of the command.
google.protobuf.Any payload = 3;
// Whether this side effect should be performed synchronously, ie, before the reply is eventually
// sent, or not.
bool synchronous = 4;
}
// A command. For each command received, a reply must be sent with a matching command id.
message Command {
// The ID of the entity.
string entity_id = 1;
// A command id.
int64 id = 2;
// Command name
string name = 3;
// The command payload.
google.protobuf.Any payload = 4;
// Whether the command is streamed or not
bool streamed = 5;
}
message StreamCancelled {
// The ID of the entity
string entity_id = 1;
// The command id
int64 id = 2;
}
// A failure reply. If this is returned, it will be translated into a gRPC unknown
// error with the corresponding description if supplied.
message Failure {
// The id of the command being replied to. Must match the input command.
int64 command_id = 1;
// A description of the error.
string description = 2;
}
message EntitySpec {
// This should be the Descriptors.FileDescriptorSet in proto serialized from as generated by:
// protoc --include_imports \
// --proto_path= \
// --descriptor_set_out=user-function.desc \
//
bytes proto = 1;
// The entities being served.
repeated Entity entities = 2;
// Optional information about the service.
ServiceInfo service_info = 3;
}
// Information about the service that proxy is proxying to.
// All of the information in here is optional. It may be useful for debug purposes.
message ServiceInfo {
// The name of the service, eg, "shopping-cart".
string service_name = 1;
// The version of the service.
string service_version = 2;
// A description of the runtime for the service. Can be anything, but examples might be:
// - node v10.15.2
// - OpenJDK Runtime Environment 1.8.0_192-b12
string service_runtime = 3;
// If using a support library, the name of that library, eg "cloudstate"
string support_library_name = 4;
// The version of the support library being used.
string support_library_version = 5;
}
message Entity {
// The type of entity. By convention, this should be a fully qualified entity protocol grpc
// service name, for example, cloudstate.eventsourced.EventSourced.
string entity_type = 1;
// The name of the service to load from the protobuf file.
string service_name = 2;
// The ID to namespace state by. How this is used depends on the type of entity, for example,
// event sourced entities will prefix this to the persistence id.
string persistence_id = 3;
}
message UserFunctionError {
string message = 1;
}
message ProxyInfo {
int32 protocol_major_version = 1;
int32 protocol_minor_version = 2;
string proxy_name = 3;
string proxy_version = 4;
repeated string supported_entity_types = 5;
}
// Entity discovery service.
service EntityDiscovery {
// Discover what entities the user function wishes to serve.
rpc discover(ProxyInfo) returns (EntitySpec) {}
// Report an error back to the user function. This will only be invoked to tell the user function
// that it has done something wrong, eg, violated the protocol, tried to use an entity type that
// isn't supported, or attempted to forward to an entity that doesn't exist, etc. These messages
// should be logged clearly for debugging purposes.
rpc reportError(UserFunctionError) returns (google.protobuf.Empty) {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy