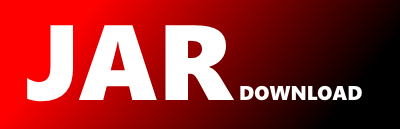
io.cloudstate.javasupport.crdt.PNCounterMap Maven / Gradle / Ivy
package io.cloudstate.javasupport.crdt;
import java.util.*;
/**
* Convenience wrapper class for {@link ORMap} that uses {@link PNCounter}'s for values.
*
* This offers a few extra methods for interacting with the map.
*
* @param The type for keys.
*/
public final class PNCounterMap extends AbstractORMapWrapper
implements Map {
public PNCounterMap(ORMap ormap) {
super(ormap);
}
/**
* Get the value for the given key.
*
* This differs from {@link Map#get(Object)} in that it returns a primitive long
,
* and thus avoids an allocation.
*
* @param key The key to get the value for.
* @return The current value of the counter at that key, or zero if no counter exists for that
* key.
*/
public long getValue(Object key) {
PNCounter counter = ormap.get(key);
if (counter != null) {
return counter.getValue();
} else {
return 0;
}
}
/**
* Increment the counter at the given key by the given amount.
*
*
The counter will be created if it is not already in the map.
*
* @param key The key of the counter.
* @param by The amount to increment by.
* @return The new value of the counter.
*/
public long increment(Object key, long by) {
return getOrUpdate(key).increment(by);
}
/**
* Decrement the counter at the given key by the given amount.
*
*
The counter will be created if it is not already in the map.
*
* @param key The key of the counter.
* @param by The amount to decrement by.
* @return The new value of the counter.
*/
public long decrement(Object key, long by) {
return getOrUpdate(key).decrement(by);
}
/** Not supported on PNCounter, use increment/decrement instead. */
@Override
public Long put(K key, Long value) {
throw new UnsupportedOperationException(
"Put is not supported on PNCounterMap, use increment or decrement instead");
}
@Override
Long getCrdtValue(PNCounter pnCounter) {
return pnCounter.getValue();
}
@Override
void setCrdtValue(PNCounter pnCounter, Long value) {
throw new UnsupportedOperationException(
"Using value mutating methods on PNCounterMap is not supported, use increment or decrement instead");
}
@Override
PNCounter getOrUpdateCrdt(K key, Long value) {
return ormap.getOrCreate(key, CrdtFactory::newPNCounter);
}
private PNCounter getOrUpdate(Object key) {
return ormap.getOrCreate((K) key, CrdtFactory::newPNCounter);
}
}