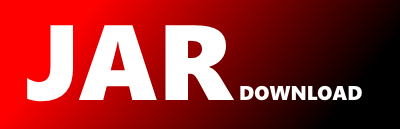
com.cobweb.io.service.ReadService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cobweb Show documentation
Show all versions of cobweb Show documentation
A Social Network for Internet of Things
The newest version!
package com.cobweb.io.service;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.Locale;
import com.cobweb.io.meta.Device;
import com.cobweb.io.meta.DeviceType;
import com.cobweb.io.meta.FriendRequest;
import com.cobweb.io.meta.LoggedUser;
import com.cobweb.io.meta.Payload;
import com.cobweb.io.meta.Sensor;
import com.cobweb.io.meta.SensorType;
import com.cobweb.io.meta.User;
import com.orientechnologies.orient.core.record.impl.ODocument;
import com.orientechnologies.orient.core.sql.query.OSQLSynchQuery;
import com.tinkerpop.blueprints.Vertex;
/**
* The Class ReadService.
*
* @author Yasith Lokuge
*/
public class ReadService implements AbstractService{
/** The invalid. */
private final String INVALID = "Invalid user name or password";
/**
* Read.
*
* @param loggedUser the logged user
* @param data the data
* @return the string
*/
public String read(LoggedUser loggedUser,String data){
String email= loggedUser.getEmail();
String password = loggedUser.getPassword();
String info;
List result = graph.getRawGraph().query(new OSQLSynchQuery
© 2015 - 2024 Weber Informatics LLC | Privacy Policy