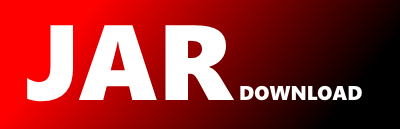
io.codemodder.remediation.xxe.SAXParserAtNewSPFixStrategy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codemodder-base Show documentation
Show all versions of codemodder-base Show documentation
Base framework for writing codemods in Java
package io.codemodder.remediation.xxe;
import static io.codemodder.remediation.xxe.XMLFixBuilder.addFeatureDisablingStatements;
import com.github.javaparser.ast.CompilationUnit;
import com.github.javaparser.ast.Node;
import com.github.javaparser.ast.expr.Expression;
import com.github.javaparser.ast.expr.MethodCallExpr;
import com.github.javaparser.ast.stmt.Statement;
import io.codemodder.ast.ASTs;
import io.codemodder.remediation.MatchAndFixStrategy;
import io.codemodder.remediation.SuccessOrReason;
import java.util.List;
import java.util.Optional;
/**
* Fix strategy for XXE vulnerabilities anchored to the SAXParser newSaxParser() calls. Finds the
* parser's declaration and add statements disabling external entities and features.
*/
final class SAXParserAtNewSPFixStrategy extends MatchAndFixStrategy {
/**
* Matches SaxParser y = (x.)newSaxParser(), where the node is the newSaxParser call.
*
* @param node
* @return
*/
public boolean match(final Node node) {
return ASTs.isInitializedToType(node, "newSAXParser", List.of("SAXParser")).isPresent();
}
@Override
public SuccessOrReason fix(final CompilationUnit cu, final Node node) {
var maybeCall =
Optional.of(node).map(n -> n instanceof MethodCallExpr ? (MethodCallExpr) n : null);
if (maybeCall.isEmpty()) {
return SuccessOrReason.reason("Not a method call.");
}
MethodCallExpr newSaxParserCall = maybeCall.get();
// the scope must be the SAXParserFactory
Optional newSaxParserCallScope = newSaxParserCall.getScope();
if (newSaxParserCallScope.isEmpty()) {
SuccessOrReason.reason("No scope found");
}
Expression scope = newSaxParserCallScope.get();
if (!scope.isNameExpr()) {
SuccessOrReason.reason("Scope is not a name");
}
Optional statement = scope.findAncestor(Statement.class);
if (statement.isEmpty()) {
return SuccessOrReason.reason("No statement found");
}
return addFeatureDisablingStatements(scope.asNameExpr(), statement.get(), true);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy