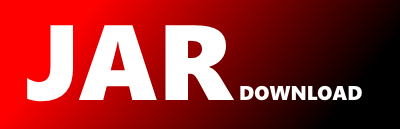
io.contek.tinker.rearm.RearmBiMapStore Maven / Gradle / Ivy
The newest version!
package io.contek.tinker.rearm;
import com.google.common.collect.ImmutableBiMap;
import java.nio.file.Path;
import java.util.NoSuchElementException;
import java.util.function.Supplier;
import javax.annotation.Nullable;
import javax.annotation.concurrent.ThreadSafe;
@ThreadSafe
public abstract class RearmBiMapStore extends RearmStore> {
protected RearmBiMapStore(Path configPath, IParser parser) {
super(configPath, parser);
}
public final Value get(Key key) throws NoSuchElementException {
return getOrThrow(key, NoSuchElementException::new);
}
public final Value getOrThrow(Key key, Supplier t) throws E {
Value value = getNullable(key);
if (value == null) {
throw t.get();
}
return value;
}
@Nullable
public final Value getNullable(Key key) {
ImmutableBiMap map = getMap();
return map.get(key);
}
public final ImmutableBiMap getMap() {
ImmutableBiMap item = getParsedConfig();
return item == null ? ImmutableBiMap.of() : item;
}
/** Parser to read and parse {@link ImmutableBiMap} from a file. */
@ThreadSafe
public interface IParser extends RearmStore.IParser> {}
/** Listener which gets called when {@link RearmBiMapStore} has update. */
@ThreadSafe
public interface IListener extends RearmStore.IListener> {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy