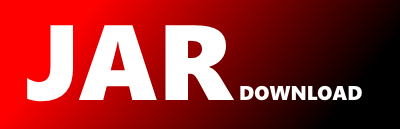
io.contek.tinker.rearm.RearmTableStore Maven / Gradle / Ivy
The newest version!
package io.contek.tinker.rearm;
import com.google.common.collect.ImmutableTable;
import com.google.common.collect.Table;
import java.nio.file.Path;
import java.util.NoSuchElementException;
import java.util.function.Supplier;
import javax.annotation.Nullable;
import javax.annotation.concurrent.ThreadSafe;
@ThreadSafe
public abstract class RearmTableStore
extends RearmStore> {
protected RearmTableStore(Path configPath, IParser parser) {
super(configPath, parser);
}
public final Value get(RowKey rowKey, ColumnKey columnKey) throws NoSuchElementException {
return getOrThrow(rowKey, columnKey, NoSuchElementException::new);
}
public final Value getOrThrow(
RowKey rowKey, ColumnKey columnKey, Supplier t) throws E {
Value value = getNullable(rowKey, columnKey);
if (value == null) {
throw t.get();
}
return value;
}
@Nullable
public final Value getNullable(RowKey rowKey, ColumnKey columnKey) {
Table table = getTable();
return table.get(rowKey, columnKey);
}
public final ImmutableTable getTable() {
ImmutableTable item = getParsedConfig();
return item == null ? ImmutableTable.of() : item;
}
/** Parser to read and parse {@link ImmutableTable} from a file. */
@ThreadSafe
public interface IParser
extends RearmStore.IParser> {}
/** Listener which gets called when {@link ImmutableTable} has update. */
@ThreadSafe
public interface IListener
extends RearmStore.IListener> {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy