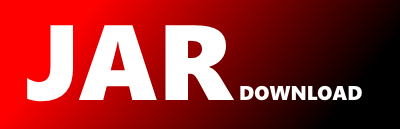
io.contek.zeus.client.IUserClient Maven / Gradle / Ivy
package io.contek.zeus.client;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableSet;
import io.contek.zeus.AssetSymbol;
import io.contek.zeus.MarketSymbol;
import io.contek.zeus.OrderKey;
import io.contek.zeus.client.errors.GatewayClientException;
import io.contek.zeus.common.*;
import io.contek.zeus.exchange.*;
import javax.annotation.Nullable;
import javax.annotation.concurrent.ThreadSafe;
import java.time.Instant;
import java.util.List;
import java.util.Map;
import java.util.Set;
import static com.google.common.collect.Iterables.getOnlyElement;
@ThreadSafe
public interface IUserClient {
void cancelOrdersForMarket(MarketSymbol marketSymbol) throws GatewayClientException;
default CancelOrderActionItem cancelOrder(OrderKey orderKey) throws GatewayClientException {
return getOnlyElement(cancelOrders(ImmutableSet.of(orderKey)));
}
ImmutableList cancelOrders(Set orderKeys)
throws GatewayClientException;
ImmutableList getAllMarginBalances() throws GatewayClientException;
ImmutableList GetPositionsForMargin(AssetSymbol marginAssetSymbol)
throws GatewayClientException;
AccountLeverageConfig getAccountLeverageConfig() throws GatewayClientException;
ImmutableList getFillHistory(MarketSymbol marketSymbol, Instant start, Instant end)
throws GatewayClientException;
default ImmutableList getOrderFills(OrderKey orderKey) throws GatewayClientException {
return getOrderFills(ImmutableSet.of(orderKey));
}
ImmutableList getOrderFills(Set orderKeys) throws GatewayClientException;
ImmutableList getOpenOrdersForMargin(AssetSymbol marginAssetSymbol)
throws GatewayClientException;
ImmutableList getOpenOrdersForMarket(MarketSymbol marketSymbol)
throws GatewayClientException;
@Nullable
default OrderSnapshot getOrder(OrderKey orderKey) throws GatewayClientException {
return getOnlyElement(getOrders(ImmutableSet.of(orderKey)), null);
}
ImmutableList getOrders(Set orderKeys) throws GatewayClientException;
@Nullable
default PositionSnapshot getPosition(MarketSymbol marketSymbol) throws GatewayClientException {
return getOnlyElement(getPositions(marketSymbol), null);
}
default ImmutableList getPositions(MarketSymbol marketSymbol)
throws GatewayClientException {
return getPositions(ImmutableSet.of(marketSymbol));
}
ImmutableList getPositions(Set marketSymbols)
throws GatewayClientException;
@Nullable
default WalletSnapshot getWallet(AssetSymbol assetSymbol) {
return getOnlyElement(getWallets(ImmutableSet.of(assetSymbol)), null);
}
ImmutableList getWallets(Set assetSymbols)
throws GatewayClientException;
default PlaceOrderActionItem placeOrder(OrderSpec orderSpec, PlaceOrderMetadata metadata) {
return placeOrder(
PlaceOrderInstruction.newBuilder().setOrderSpec(orderSpec).setMetadata(metadata).build());
}
default PlaceOrderActionItem placeOrder(PlaceOrderInstruction instruction)
throws GatewayClientException {
return getOnlyElement(placeOrders(ImmutableList.of(instruction)));
}
ImmutableList placeOrders(List instructions)
throws GatewayClientException;
void updateSettings(Map properties) throws GatewayClientException;
LeverageConfig updatePositionLeverageConfig(MarketSymbol marketSymbol, LeverageConfig config)
throws GatewayClientException;
ICloseableIterator subscribeOrderEventsForMarket(MarketSymbol marketSymbol)
throws GatewayClientException;
ICloseableIterator subscribePositionEventsForMarket(MarketSymbol marketSymbol)
throws GatewayClientException;
ICloseableIterator subscribePositionEventsForMargin(AssetSymbol marginAssetSymbol)
throws GatewayClientException;
ICloseableIterator subscribeFillEventsForMarket(MarketSymbol marketSymbol)
throws GatewayClientException;
ICloseableIterator subscribeFillEventsForMargin(AssetSymbol marginAssetSymbol)
throws GatewayClientException;
ICloseableIterator subscribeOrderEventsForMargin(AssetSymbol marginAssetSymbol)
throws GatewayClientException;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy