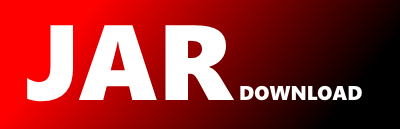
io.contek.zeus.client.ZeusClient Maven / Gradle / Ivy
package io.contek.zeus.client;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Maps;
import io.contek.zeus.AccountId;
import io.contek.zeus.ExchangeId;
import javax.annotation.concurrent.NotThreadSafe;
import javax.annotation.concurrent.ThreadSafe;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.NoSuchElementException;
@ThreadSafe
public final class ZeusClient {
private final ImmutableMap pools;
public ZeusClient(Collection pools) {
this.pools = Maps.uniqueIndex(pools, Client::getExchangeId);
}
public static Builder newBuilder() {
return new Builder();
}
public IMarketClient market(ExchangeId exchangeId) {
return client(exchangeId).market();
}
public IUserClient user(AccountId accountId) {
return client(accountId.getExchangeId()).user(accountId.getAccountName());
}
private Client client(ExchangeId exchangeId) {
Client client = pools.get(exchangeId);
if (client == null) {
throw new NoSuchElementException("No client found for " + exchangeId);
}
return client;
}
@NotThreadSafe
public static final class Builder {
public List clients = new ArrayList<>();
public Builder addPool(Client client) {
this.clients.add(client);
return this;
}
public Builder addPools(Collection clients) {
this.clients.addAll(clients);
return this;
}
public Builder setClients(List clients) {
this.clients = clients;
return this;
}
public ZeusClient build() {
return new ZeusClient(clients);
}
private Builder() {}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy