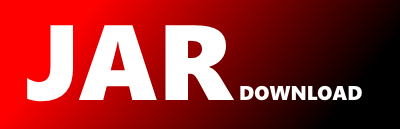
io.contek.zeus.server.errors.ExchangeOrderNotUpdatedException Maven / Gradle / Ivy
package io.contek.zeus.server.errors;
import io.contek.zeus.OrderKey;
import io.contek.zeus.common.OrderStatus;
import javax.annotation.Nullable;
import javax.annotation.concurrent.NotThreadSafe;
import static io.contek.zeus.common.OrderStatus.OPEN;
import static java.lang.String.format;
@NotThreadSafe
public final class ExchangeOrderNotUpdatedException extends ExchangeTemporaryErrorException {
private final OrderKey orderKey;
private final OrderStatus expectedStatus;
private final OrderStatus actualStatus;
public ExchangeOrderNotUpdatedException(
OrderKey orderKey, OrderStatus expectedStatus, @Nullable OrderStatus actualStatus) {
super(
format(
"Order [%s] expected status: %s, actual status: %s",
orderKey, expectedStatus, actualStatus));
this.orderKey = orderKey;
this.expectedStatus = expectedStatus;
this.actualStatus = actualStatus;
}
public static ExchangeOrderNotUpdatedException notFound(OrderKey orderKey) {
return notFound(orderKey, OPEN);
}
public static ExchangeOrderNotUpdatedException notFound(
OrderKey orderKey, OrderStatus expectedStatus) {
return new ExchangeOrderNotUpdatedException(orderKey, expectedStatus, null);
}
public OrderKey getOrderKey() {
return orderKey;
}
public OrderStatus getExpectedStatus() {
return expectedStatus;
}
@Nullable
public OrderStatus getActualStatus() {
return actualStatus;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy