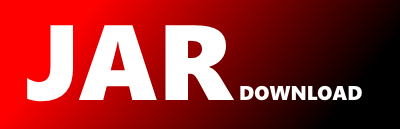
io.cortical.retina.model.Sample Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of retina-api-java-sdk Show documentation
Show all versions of retina-api-java-sdk Show documentation
cortical.io's java client - a simple java http client which simplifies communication between any java application and the Retina server using the Retina's REST API.
package io.cortical.retina.model;
import java.util.ArrayList;
import java.util.List;
/**
* A classification sample containing lists of positive {@link Text}
* examples (mandatory), and potentially negative examples (optional).
*
* This container's fields are named such that the resulting json
* fields match the required field naming of the classify endpoint.
*/
public class Sample {
private List positiveExamples;
private List negativeExamples;
public Sample() {
positiveExamples = new ArrayList<>();
negativeExamples = new ArrayList<>();
}
/**
* Sets the specified list as the list of positive
* examples.
* @param pos
*/
public void setPositiveExamples(List pos) {
this.positiveExamples = pos;
}
/**
* Returns the list of positive examples.
* @return
*/
public List getPositiveExamples() {
return positiveExamples;
}
/**
* Sets the specified list as the list of negative
* examples.
* @param neg
*/
public void setNegativeExamples(List neg) {
this.negativeExamples = neg;
}
/**
* Returns the list of negative examples.
* @return
*/
public List getNegativeExamples() {
return negativeExamples;
}
/**
* Adds a single positive {@link Text} to this {@code Sample}'s
* list of positive examples.
* @param text
*/
public void addPositiveExample(Text text) {
positiveExamples.add(text);
}
/**
* Adds a single negative {@link Text} to this {@code Sample}'s
* list of negative examples.
* @param text
*/
public void addNegativeExample(Text text) {
negativeExamples.add(text);
}
/**
* Adds a single positive String to this {@code Sample}'s
* list of positive examples.
* @param text
*/
public void addPositiveExample(String text) {
positiveExamples.add(new Text(text));
}
/**
* Adds a single negative String to this {@code Sample}'s
* list of negative examples.
* @param text
*/
public void addNegativeExample(String text) {
negativeExamples.add(new Text(text));
}
/**
* Adds the specified list to this {@code Sample}'s list
* of positive examples.
* @param positiveTexts
*/
public void addAllPositive(List positiveTexts) {
if(positiveTexts == null) return;
positiveExamples.addAll(positiveTexts);
}
/**
* Adds the specified list to this {@code Sample}'s list
* of negative examples.
* @param negativeTexts
*/
public void addAllNegative(List negativeTexts) {
if(negativeTexts == null) return;
negativeExamples.addAll(negativeTexts);
}
/**
* Adds the specified list to this {@code Sample}'s list
* of positive examples.
* @param positiveTexts
*/
public void addAllPositive(String... positiveTexts) {
if(positiveTexts == null) return;
for(String s : positiveTexts) {
positiveExamples.add(new Text(s));
}
}
/**
* Adds the specified list to this {@code Sample}'s list
* of negative examples.
* @param negativeTexts
*/
public void addAllNegative(String... negativeTexts) {
if(negativeTexts == null) return;
for(String s : negativeTexts) {
negativeExamples.add(new Text(s));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy