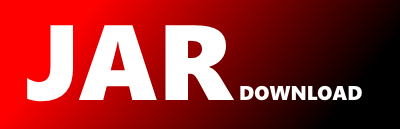
io.crnk.jpa.internal.JpaResourceFieldInformationProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of crnk-jpa Show documentation
Show all versions of crnk-jpa Show documentation
JSON API framework for Java
package io.crnk.jpa.internal;
import javax.persistence.Column;
import javax.persistence.ElementCollection;
import javax.persistence.EmbeddedId;
import javax.persistence.FetchType;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.Lob;
import javax.persistence.ManyToMany;
import javax.persistence.ManyToOne;
import javax.persistence.OneToMany;
import javax.persistence.OneToOne;
import javax.persistence.Version;
import io.crnk.core.engine.information.bean.BeanAttributeInformation;
import io.crnk.core.engine.information.resource.ResourceFieldInformationProviderBase;
import io.crnk.core.engine.information.resource.ResourceFieldType;
import io.crnk.core.engine.internal.utils.StringUtils;
import io.crnk.core.resource.annotations.SerializeType;
import io.crnk.core.utils.Optional;
public class JpaResourceFieldInformationProvider extends ResourceFieldInformationProviderBase {
@Override
public Optional isSortable(BeanAttributeInformation attributeDesc) {
Optional lob = attributeDesc.getAnnotation(Lob.class);
if (lob.isPresent()) {
return Optional.of(false);
}
return Optional.empty();
}
@Override
public Optional isFilterable(BeanAttributeInformation attributeDesc) {
Optional lob = attributeDesc.getAnnotation(Lob.class);
if (lob.isPresent()) {
return Optional.of(false);
}
return Optional.empty();
}
@Override
public Optional isPostable(BeanAttributeInformation attributeDesc) {
Optional column = attributeDesc.getAnnotation(Column.class);
Optional version = attributeDesc.getAnnotation(Version.class);
if (!version.isPresent() && column.isPresent()) {
return Optional.of(column.get().insertable());
}
Optional generatedValue = attributeDesc.getAnnotation(GeneratedValue.class);
if (generatedValue.isPresent()) {
return Optional.of(false);
}
return Optional.empty();
}
@Override
public Optional isPatchable(BeanAttributeInformation attributeDesc) {
Optional column = attributeDesc.getAnnotation(Column.class);
Optional version = attributeDesc.getAnnotation(Version.class);
if (!version.isPresent() && column.isPresent()) {
return Optional.of(column.get().updatable());
}
Optional generatedValue = attributeDesc.getAnnotation(GeneratedValue.class);
if (generatedValue.isPresent()) {
return Optional.of(false);
}
return Optional.empty();
}
@Override
public Optional getFieldType(BeanAttributeInformation attributeDesc) {
Optional oneToOne = attributeDesc.getAnnotation(OneToOne.class);
Optional oneToMany = attributeDesc.getAnnotation(OneToMany.class);
Optional manyToOne = attributeDesc.getAnnotation(ManyToOne.class);
Optional manyToMany = attributeDesc.getAnnotation(ManyToMany.class);
if (oneToOne.isPresent() || oneToMany.isPresent() || manyToOne.isPresent() || manyToMany.isPresent()) {
return Optional.of(ResourceFieldType.RELATIONSHIP);
}
Optional id = attributeDesc.getAnnotation(Id.class);
Optional embeddedId = attributeDesc.getAnnotation(EmbeddedId.class);
if (id.isPresent() || embeddedId.isPresent()) {
return Optional.of(ResourceFieldType.ID);
}
return Optional.empty();
}
@Override
public Optional getOppositeName(BeanAttributeInformation attributeDesc) {
Optional oneToMany = attributeDesc.getAnnotation(OneToMany.class);
if (oneToMany.isPresent()) {
return Optional.ofNullable(StringUtils.emptyToNull(oneToMany.get().mappedBy()));
}
Optional manyToMany = attributeDesc.getAnnotation(ManyToMany.class);
if (manyToMany.isPresent()) {
return Optional.ofNullable(StringUtils.emptyToNull(manyToMany.get().mappedBy()));
}
return Optional.empty();
}
@Override
public Optional getSerializeType(BeanAttributeInformation attributeDesc) {
Optional oneToMany = attributeDesc.getAnnotation(OneToMany.class);
if (oneToMany.isPresent()) {
return toSerializeType(oneToMany.get().fetch());
}
Optional manyToOne = attributeDesc.getAnnotation(ManyToOne.class);
if (manyToOne.isPresent()) {
return toSerializeType(manyToOne.get().fetch());
}
Optional manyToMany = attributeDesc.getAnnotation(ManyToMany.class);
if (manyToMany.isPresent()) {
return toSerializeType(manyToMany.get().fetch());
}
Optional elementCollection = attributeDesc.getAnnotation(ElementCollection.class);
if (elementCollection.isPresent()) {
return toSerializeType(elementCollection.get().fetch());
}
return Optional.empty();
}
private Optional toSerializeType(FetchType fetch) {
return Optional.of(fetch == FetchType.EAGER ? SerializeType.ONLY_ID : SerializeType.LAZY);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy