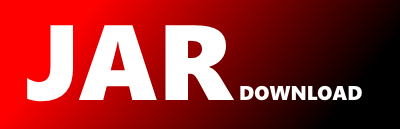
io.crossbar.autobahn.wamp.interfaces.ISession Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of autobahn-java Show documentation
Show all versions of autobahn-java Show documentation
WebSocket & WAMP for Java8+
The newest version!
///////////////////////////////////////////////////////////////////////////////
//
// AutobahnJava - http://crossbar.io/autobahn
//
// Copyright (c) Crossbar.io Technologies GmbH and contributors
//
// Licensed under the MIT License.
// http://www.opensource.org/licenses/mit-license.php
//
///////////////////////////////////////////////////////////////////////////////
package io.crossbar.autobahn.wamp.interfaces;
import com.fasterxml.jackson.core.type.TypeReference;
import java.util.List;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
import io.crossbar.autobahn.wamp.Session;
import io.crossbar.autobahn.wamp.types.CallOptions;
import io.crossbar.autobahn.wamp.types.CallResult;
import io.crossbar.autobahn.wamp.types.CloseDetails;
import io.crossbar.autobahn.wamp.types.EventDetails;
import io.crossbar.autobahn.wamp.types.InvocationDetails;
import io.crossbar.autobahn.wamp.types.Publication;
import io.crossbar.autobahn.wamp.types.PublishOptions;
import io.crossbar.autobahn.wamp.types.ReceptionResult;
import io.crossbar.autobahn.wamp.types.RegisterOptions;
import io.crossbar.autobahn.wamp.types.Registration;
import io.crossbar.autobahn.wamp.types.SessionDetails;
import io.crossbar.autobahn.wamp.types.SubscribeOptions;
import io.crossbar.autobahn.wamp.types.Subscription;
public interface ISession {
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(String topic, Runnable handler);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @param options options for the subscribe
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
Runnable handler,
SubscribeOptions options);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
Consumer> handler);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
Consumer> handler,
SubscribeOptions options);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @param resultType TypeReference encapsulating the class of the first
* parameter of the callback method
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
Consumer handler,
TypeReference resultType);
CompletableFuture subscribe(
String topic,
Consumer handler,
Class resultType);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @param resultType TypeReference encapsulating the class of the first
* parameter of the callback method
* @param options options for the subscribe
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
Consumer handler,
TypeReference resultType,
SubscribeOptions options);
CompletableFuture subscribe(
String topic,
Consumer handler,
Class resultType,
SubscribeOptions options);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
Function, CompletableFuture> handler);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @param options options for the subscribe
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
Function, CompletableFuture> handler,
SubscribeOptions options);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @param resultType TypeReference encapsulating the class of the first
* parameter of the callback method
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
Function> handler,
TypeReference resultType);
CompletableFuture subscribe(
String topic,
Function> handler,
Class resultType);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @param resultType TypeReference encapsulating the class of the first
* parameter of the callback method
* @param options options for the subscribe
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
Function> handler,
TypeReference resultType,
SubscribeOptions options);
CompletableFuture subscribe(
String topic,
Function> handler,
Class resultType,
SubscribeOptions options);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
BiConsumer, EventDetails> handler);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @param options options for the subscribe
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
BiConsumer, EventDetails> handler,
SubscribeOptions options);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @param resultType TypeReference encapsulating the class of the first
* parameter of the callback method
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
BiConsumer handler,
TypeReference resultType);
CompletableFuture subscribe(
String topic,
BiConsumer handler,
Class resultType);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @param resultType TypeReference encapsulating the class of the first
* parameter of the callback method
* @param options options for the subscribe
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
BiConsumer handler,
TypeReference resultType,
SubscribeOptions options);
CompletableFuture subscribe(
String topic,
BiConsumer handler,
Class resultType,
SubscribeOptions options);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
BiFunction, EventDetails, CompletableFuture> handler);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @param options options for the subscribe
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
BiFunction, EventDetails, CompletableFuture> handler,
SubscribeOptions options);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @param resultType TypeReference encapsulating the class of the first
* parameter of the callback method
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
BiFunction> handler,
TypeReference resultType);
CompletableFuture subscribe(
String topic,
BiFunction> handler,
Class resultType);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @param resultType TypeReference encapsulating the class of the first
* parameter of the callback method
* @param options options for the subscribe
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
BiFunction> handler,
TypeReference resultType,
SubscribeOptions options);
CompletableFuture subscribe(
String topic,
BiFunction> handler,
Class resultType,
SubscribeOptions options);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
TriConsumer, Map, EventDetails> handler);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @param options options for the subscribe
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
TriConsumer, Map, EventDetails> handler,
SubscribeOptions options);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
TriFunction, Map, EventDetails,
CompletableFuture> handler);
/**
* Subscribes to a WAMP topic.
* @param topic URI of the topic to subscribe
* @param handler callback method for results of publication to the topic
* @param options options for the subscribe
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Subscription}
*/
CompletableFuture subscribe(
String topic,
TriFunction, Map, EventDetails,
CompletableFuture> handler,
SubscribeOptions options);
CompletableFuture unsubscribe(Subscription subscription);
/**
* Publishes to a WAMP topic.
* @param topic URI of the topic
* @param args positional arguments for the topic
* @param kwargs keyword arguments for the topic
* @param options options for the publication
* @return a CompletableFuture that resolves to an instance of
* {@link io.crossbar.autobahn.wamp.types.Publication}
*/
CompletableFuture publish(
String topic,
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy