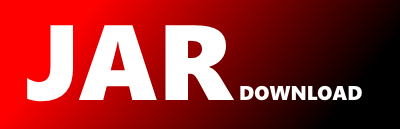
io.crossbar.autobahn.wamp.messages.Invocation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of autobahn-java Show documentation
Show all versions of autobahn-java Show documentation
WebSocket & WAMP for Java8+
The newest version!
///////////////////////////////////////////////////////////////////////////////
//
// AutobahnJava - http://crossbar.io/autobahn
//
// Copyright (c) Crossbar.io Technologies GmbH and contributors
//
// Licensed under the MIT License.
// http://www.opensource.org/licenses/mit-license.php
//
///////////////////////////////////////////////////////////////////////////////
package io.crossbar.autobahn.wamp.messages;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import io.crossbar.autobahn.wamp.exceptions.ProtocolError;
import io.crossbar.autobahn.wamp.interfaces.IMessage;
import io.crossbar.autobahn.wamp.utils.MessageUtil;
public class Invocation implements IMessage {
public static final int MESSAGE_TYPE = 68;
public final long request;
public final long registration;
public final Map details;
public final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy