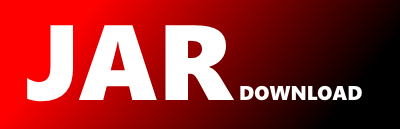
xbr.network.SimpleBuyer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of autobahn-java Show documentation
Show all versions of autobahn-java Show documentation
WebSocket & WAMP for Java8+
The newest version!
///////////////////////////////////////////////////////////////////////////////
//
// AutobahnJava - http://crossbar.io/autobahn
//
// Copyright (c) Crossbar.io Technologies GmbH and contributors
//
// Licensed under the MIT License.
// http://www.opensource.org/licenses/mit-license.php
//
///////////////////////////////////////////////////////////////////////////////
package xbr.network;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.dataformat.cbor.CBORFactory;
import org.libsodium.jni.SodiumConstants;
import org.web3j.crypto.Credentials;
import org.web3j.crypto.ECKeyPair;
import org.web3j.utils.Numeric;
import java.math.BigInteger;
import java.security.SecureRandom;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.atomic.AtomicReference;
import io.crossbar.autobahn.utils.ABLogger;
import io.crossbar.autobahn.utils.IABLogger;
import io.crossbar.autobahn.wamp.Session;
import io.crossbar.autobahn.wamp.exceptions.ApplicationError;
import xbr.network.crypto.SealedBox;
import xbr.network.crypto.SecretBox;
import xbr.network.pojo.Quote;
import xbr.network.pojo.Receipt;
import static org.libsodium.jni.NaCl.sodium;
public class SimpleBuyer {
private static final IABLogger LOGGER = ABLogger.getLogger(SimpleBuyer.class.getName());
private final byte[] mEthPrivateKey;
private final byte[] mEthPublicKey;
private final byte[] mEthAddr;
private final byte[] mMarketMakerAddr;
private final BigInteger mMaxPrice;
private final ECKeyPair mECKey;
private HashMap mKeys;
private Session mSession;
private boolean mRunning;
private int mSeq;
private BigInteger mRemainingBalance;
private HashMap mChannel;
private Map mMakerConfig;
private final byte[] mPrivateKey;
private final byte[] mPublicKey;
public SimpleBuyer(String marketMakerAddr, String buyerKey, BigInteger maxPrice) {
mECKey = ECKeyPair.create(Numeric.hexStringToByteArray(buyerKey));
mMarketMakerAddr = Numeric.hexStringToByteArray(marketMakerAddr);
mEthPrivateKey = mECKey.getPrivateKey().toByteArray();
mEthPublicKey = mECKey.getPublicKey().toByteArray();
mEthAddr = Numeric.hexStringToByteArray(Credentials.create(mECKey).getAddress());
mPrivateKey = new byte[SodiumConstants.SECRETKEY_BYTES];
mPublicKey = new byte[SodiumConstants.PUBLICKEY_BYTES];
sodium().crypto_box_keypair(mPublicKey, mPrivateKey);
mMaxPrice = maxPrice;
mKeys = new HashMap<>();
}
private boolean isConnected() {
return mSession != null && mSession.isConnected();
}
public CompletableFuture start(Session session, String consumerID) {
CompletableFuture future = new CompletableFuture<>();
if (mRunning) {
future.completeExceptionally(new IllegalStateException("Already running..."));
return future;
}
mSession = session;
mRunning = true;
mSession.call(
"xbr.marketmaker.get_config",
Map.class
).thenCompose(makerConfig -> {
mMakerConfig = makerConfig;
return mSession.call(
"xbr.marketmaker.get_active_payment_channel",
new TypeReference>() {},
mEthAddr);
}).thenCompose(channel -> {
mChannel = channel;
return balance();
}).thenAccept(balance -> {
future.complete((BigInteger) balance.get("remaining"));
}).exceptionally(throwable -> {
future.completeExceptionally(throwable);
return null;
});
return future;
}
public CompletableFuture stop() {
CompletableFuture future = new CompletableFuture<>();
if (!mRunning) {
future.completeExceptionally(new IllegalStateException("Already stopped..."));
return future;
}
mRunning = false;
return CompletableFuture.completedFuture(false);
}
public CompletableFuture> balance() {
CompletableFuture> future = new CompletableFuture<>();
if (!isConnected()) {
future.completeExceptionally(new IllegalStateException("Session not connected"));
return future;
}
mSession.call(
"xbr.marketmaker.get_payment_channel_balance",
new TypeReference>() {},
mChannel.get("channel_oid")
).thenAccept(paymentBalance -> {
mRemainingBalance = new BigInteger((byte[]) paymentBalance.get("remaining"));
HashMap res = new HashMap<>();
res.put("amount", paymentBalance.get("amount"));
res.put("remaining", mRemainingBalance);
res.put("inflight", paymentBalance.get("inflight"));
mSeq = (int) paymentBalance.get("seq");
future.complete(res);
}).exceptionally(throwable -> {
future.completeExceptionally(throwable);
return null;
});
return future;
}
public CompletableFuture> openChannel(byte[] buyerAddr,
BigInteger amount) {
CompletableFuture> future = new CompletableFuture<>();
if (!isConnected()) {
future.completeExceptionally(new IllegalStateException("Session not connected"));
return future;
}
mSession.call(
"xbr.marketmaker.open_payment_channel",
new TypeReference>() {},
buyerAddr,
mEthAddr,
amount,
new SecureRandom(new byte[64])
).thenAccept(paymentChannel -> {
BigInteger remaining = new BigInteger((byte[]) paymentChannel.get("remaining"));
HashMap res = new HashMap<>();
res.put("amount", paymentChannel.get("amount"));
res.put("remaining", remaining);
res.put("inflight", paymentChannel.get("inflight"));
future.complete(res);
}).exceptionally(throwable -> {
future.completeExceptionally(throwable);
return null;
});
return future;
}
public void closeChannel() {
}
private CompletableFuture
© 2015 - 2025 Weber Informatics LLC | Privacy Policy