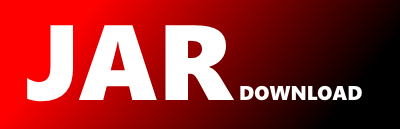
io.cucumber.cucumberexpressions.NumberParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cucumber-expressions Show documentation
Show all versions of cucumber-expressions Show documentation
Cucumber Expressions are simple patterns for matching Step Definitions with Gherkin steps
package io.cucumber.cucumberexpressions;
import java.math.BigDecimal;
import java.text.DecimalFormat;
import java.text.DecimalFormatSymbols;
import java.text.NumberFormat;
import java.text.ParseException;
import java.util.Locale;
final class NumberParser {
private final NumberFormat numberFormat;
NumberParser(Locale locale) {
numberFormat = DecimalFormat.getNumberInstance(locale);
if (numberFormat instanceof DecimalFormat) {
DecimalFormat decimalFormat = (DecimalFormat) numberFormat;
decimalFormat.setParseBigDecimal(true);
DecimalFormatSymbols symbols = KeyboardFriendlyDecimalFormatSymbols.getInstance(locale);
decimalFormat.setDecimalFormatSymbols(symbols);
}
}
double parseDouble(String s) {
return parse(s).doubleValue();
}
float parseFloat(String s) {
return parse(s).floatValue();
}
BigDecimal parseBigDecimal(String s) {
if (numberFormat instanceof DecimalFormat) {
return (BigDecimal) parse(s);
}
// Fall back to default big decimal format
// if the locale does not have a DecimalFormat
return new BigDecimal(s);
}
private Number parse(String s) {
try {
return numberFormat.parse(s);
} catch (ParseException e) {
throw new CucumberExpressionException("Failed to parse number", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy