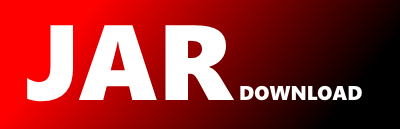
gherkin.GherkinLine Maven / Gradle / Ivy
The newest version!
package gherkin;
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
import static gherkin.StringUtils.ltrim;
public class GherkinLine implements IGherkinLine {
private final String lineText;
private final String trimmedLineText;
public GherkinLine(String lineText) {
this.lineText = lineText;
this.trimmedLineText = ltrim(lineText);
}
@Override
public Integer indent() {
return lineText.length() - trimmedLineText.length();
}
@Override
public void detach() {
}
@Override
public String getLineText(int indentToRemove) {
if (indentToRemove < 0 || indentToRemove > indent())
return trimmedLineText;
return lineText.substring(indentToRemove);
}
@Override
public boolean isEmpty() {
return trimmedLineText.length() == 0;
}
@Override
public boolean startsWith(String prefix) {
return trimmedLineText.startsWith(prefix);
}
@Override
public String getRestTrimmed(int length) {
return trimmedLineText.substring(length).trim();
}
@Override
public List getTags() {
return getSpans("\\s+");
}
@Override
public boolean startsWithTitleKeyword(String text) {
int textLength = text.length();
return trimmedLineText.length() > textLength &&
trimmedLineText.startsWith(text) &&
trimmedLineText.substring(textLength, textLength + GherkinLanguageConstants.TITLE_KEYWORD_SEPARATOR.length())
.equals(GherkinLanguageConstants.TITLE_KEYWORD_SEPARATOR);
// TODO aslak: extract startsWithFrom method for clarity
}
@Override
public List getTableCells() {
List lineSpans = new ArrayList();
StringBuilder cell = new StringBuilder();
boolean beforeFirst = true;
int startCol = 0;
for (int col = 0; col < trimmedLineText.length(); col++) {
char c = trimmedLineText.charAt(col);
if (c == '|') {
if (beforeFirst) {
// Skip the first empty span
beforeFirst = false;
} else {
int contentStart = 0;
while (contentStart < cell.length() && Character.isWhitespace(cell.charAt(contentStart))) {
contentStart++;
}
if (contentStart == cell.length()) {
contentStart = 0;
}
lineSpans.add(new GherkinLineSpan(indent() + startCol + contentStart + 2, cell.toString().trim()));
startCol = col;
}
cell = new StringBuilder();
} else if (c == '\\') {
col++;
c = trimmedLineText.charAt(col);
if (c == 'n') {
cell.append('\n');
} else {
cell.append(c);
}
} else {
cell.append(c);
}
}
return lineSpans;
}
private List getSpans(String delimiter) {
List lineSpans = new ArrayList();
Scanner scanner = new Scanner(trimmedLineText).useDelimiter(delimiter);
while (scanner.hasNext()) {
String cell = scanner.next();
int column = scanner.match().start() + indent() + 1;
lineSpans.add(new GherkinLineSpan(column, cell));
}
return lineSpans;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy