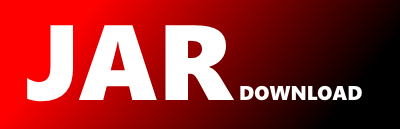
gherkin.Parser Maven / Gradle / Ivy
The newest version!
// This code was generated by Berp (http://https://github.com/gasparnagy/berp/).
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
package gherkin;
import java.io.Reader;
import java.io.StringReader;
import java.util.ArrayDeque;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
import java.util.Queue;
import static java.util.Arrays.asList;
public class Parser {
public enum TokenType {
None,
EOF,
Empty,
Comment,
TagLine,
FeatureLine,
BackgroundLine,
ScenarioLine,
ScenarioOutlineLine,
ExamplesLine,
StepLine,
DocStringSeparator,
TableRow,
Language,
Other,
;
}
public enum RuleType {
None,
_EOF, // #EOF
_Empty, // #Empty
_Comment, // #Comment
_TagLine, // #TagLine
_FeatureLine, // #FeatureLine
_BackgroundLine, // #BackgroundLine
_ScenarioLine, // #ScenarioLine
_ScenarioOutlineLine, // #ScenarioOutlineLine
_ExamplesLine, // #ExamplesLine
_StepLine, // #StepLine
_DocStringSeparator, // #DocStringSeparator
_TableRow, // #TableRow
_Language, // #Language
_Other, // #Other
Feature, // Feature! := Feature_Header Background? Scenario_Definition*
Feature_Header, // Feature_Header! := #Language? Tags? #FeatureLine Feature_Description
Background, // Background! := #BackgroundLine Background_Description Scenario_Step*
Scenario_Definition, // Scenario_Definition! := Tags? (Scenario | ScenarioOutline)
Scenario, // Scenario! := #ScenarioLine Scenario_Description Scenario_Step*
ScenarioOutline, // ScenarioOutline! := #ScenarioOutlineLine ScenarioOutline_Description ScenarioOutline_Step* Examples_Definition+
Examples_Definition, // Examples_Definition! [#Empty|#Comment|#TagLine->#ExamplesLine] := Tags? Examples
Examples, // Examples! := #ExamplesLine Examples_Description #TableRow #TableRow+
Scenario_Step, // Scenario_Step := Step
ScenarioOutline_Step, // ScenarioOutline_Step := Step
Step, // Step! := #StepLine Step_Arg?
Step_Arg, // Step_Arg := (DataTable | DocString)
DataTable, // DataTable! := #TableRow+
DocString, // DocString! := #DocStringSeparator #Other* #DocStringSeparator
Tags, // Tags! := #TagLine+
Feature_Description, // Feature_Description := Description_Helper
Background_Description, // Background_Description := Description_Helper
Scenario_Description, // Scenario_Description := Description_Helper
ScenarioOutline_Description, // ScenarioOutline_Description := Description_Helper
Examples_Description, // Examples_Description := Description_Helper
Description_Helper, // Description_Helper := #Empty* Description? #Comment*
Description, // Description! := #Other+
;
public static RuleType cast(TokenType tokenType) {
return RuleType.values()[tokenType.ordinal()];
}
}
private final Builder builder;
public boolean stopAtFirstError;
class ParserContext {
public final ITokenScanner tokenScanner;
public final ITokenMatcher tokenMatcher;
public final Queue tokenQueue;
public final List errors;
ParserContext(ITokenScanner tokenScanner, ITokenMatcher tokenMatcher, Queue tokenQueue, List errors) {
this.tokenScanner = tokenScanner;
this.tokenMatcher = tokenMatcher;
this.tokenQueue = tokenQueue;
this.errors = errors;
}
}
public Parser(Builder builder) {
this.builder = builder;
}
public T parse(String source) {
return parse(new StringReader(source));
}
public T parse(Reader source) {
return parse(new TokenScanner(source));
}
public T parse(ITokenScanner tokenScanner) {
return parse(tokenScanner, new TokenMatcher());
}
public T parse(String source, ITokenMatcher tokenMatcher) {
return parse(new StringReader(source), tokenMatcher);
}
public T parse(Reader source, ITokenMatcher tokenMatcher) {
return parse(new TokenScanner(source), tokenMatcher);
}
public T parse(ITokenScanner tokenScanner, ITokenMatcher tokenMatcher) {
builder.reset();
tokenMatcher.reset();
ParserContext context = new ParserContext(
tokenScanner,
tokenMatcher,
new LinkedList(),
new ArrayList()
);
startRule(context, RuleType.Feature);
int state = 0;
Token token;
do {
token = readToken(context);
state = matchToken(state, token, context);
} while (!token.isEOF());
endRule(context, RuleType.Feature);
if (context.errors.size() > 0) {
throw new ParserException.CompositeParserException(context.errors);
}
return builder.getResult();
}
private void addError(ParserContext context, ParserException error) {
context.errors.add(error);
if (context.errors.size() > 10)
throw new ParserException.CompositeParserException(context.errors);
}
private V handleAstError(ParserContext context, final Func action) {
return handleExternalError(context, action, null);
}
private V handleExternalError(ParserContext context, Func action, V defaultValue) {
if (stopAtFirstError) {
return action.call();
}
try {
return action.call();
} catch (ParserException.CompositeParserException compositeParserException) {
for (ParserException error : compositeParserException.errors) {
addError(context, error);
}
} catch (ParserException error) {
addError(context, error);
}
return defaultValue;
}
private void build(final ParserContext context, final Token token) {
handleAstError(context, new Func() {
public Void call() {
builder.build(token);
return null;
}
});
}
private void startRule(final ParserContext context, final RuleType ruleType) {
handleAstError(context, new Func() {
public Void call() {
builder.startRule(ruleType);
return null;
}
});
}
private void endRule(final ParserContext context, final RuleType ruleType) {
handleAstError(context, new Func() {
public Void call() {
builder.endRule(ruleType);
return null;
}
});
}
private Token readToken(ParserContext context) {
return context.tokenQueue.size() > 0 ? context.tokenQueue.remove() : context.tokenScanner.read();
}
private boolean match_EOF(final ParserContext context, final Token token) {
return handleExternalError(context, new Func() {
public Boolean call() {
return context.tokenMatcher.match_EOF(token);
}
}, false);
}
private boolean match_Empty(final ParserContext context, final Token token) {
if (token.isEOF()) return false;
return handleExternalError(context, new Func() {
public Boolean call() {
return context.tokenMatcher.match_Empty(token);
}
}, false);
}
private boolean match_Comment(final ParserContext context, final Token token) {
if (token.isEOF()) return false;
return handleExternalError(context, new Func() {
public Boolean call() {
return context.tokenMatcher.match_Comment(token);
}
}, false);
}
private boolean match_TagLine(final ParserContext context, final Token token) {
if (token.isEOF()) return false;
return handleExternalError(context, new Func() {
public Boolean call() {
return context.tokenMatcher.match_TagLine(token);
}
}, false);
}
private boolean match_FeatureLine(final ParserContext context, final Token token) {
if (token.isEOF()) return false;
return handleExternalError(context, new Func() {
public Boolean call() {
return context.tokenMatcher.match_FeatureLine(token);
}
}, false);
}
private boolean match_BackgroundLine(final ParserContext context, final Token token) {
if (token.isEOF()) return false;
return handleExternalError(context, new Func() {
public Boolean call() {
return context.tokenMatcher.match_BackgroundLine(token);
}
}, false);
}
private boolean match_ScenarioLine(final ParserContext context, final Token token) {
if (token.isEOF()) return false;
return handleExternalError(context, new Func() {
public Boolean call() {
return context.tokenMatcher.match_ScenarioLine(token);
}
}, false);
}
private boolean match_ScenarioOutlineLine(final ParserContext context, final Token token) {
if (token.isEOF()) return false;
return handleExternalError(context, new Func() {
public Boolean call() {
return context.tokenMatcher.match_ScenarioOutlineLine(token);
}
}, false);
}
private boolean match_ExamplesLine(final ParserContext context, final Token token) {
if (token.isEOF()) return false;
return handleExternalError(context, new Func() {
public Boolean call() {
return context.tokenMatcher.match_ExamplesLine(token);
}
}, false);
}
private boolean match_StepLine(final ParserContext context, final Token token) {
if (token.isEOF()) return false;
return handleExternalError(context, new Func() {
public Boolean call() {
return context.tokenMatcher.match_StepLine(token);
}
}, false);
}
private boolean match_DocStringSeparator(final ParserContext context, final Token token) {
if (token.isEOF()) return false;
return handleExternalError(context, new Func() {
public Boolean call() {
return context.tokenMatcher.match_DocStringSeparator(token);
}
}, false);
}
private boolean match_TableRow(final ParserContext context, final Token token) {
if (token.isEOF()) return false;
return handleExternalError(context, new Func() {
public Boolean call() {
return context.tokenMatcher.match_TableRow(token);
}
}, false);
}
private boolean match_Language(final ParserContext context, final Token token) {
if (token.isEOF()) return false;
return handleExternalError(context, new Func() {
public Boolean call() {
return context.tokenMatcher.match_Language(token);
}
}, false);
}
private boolean match_Other(final ParserContext context, final Token token) {
if (token.isEOF()) return false;
return handleExternalError(context, new Func() {
public Boolean call() {
return context.tokenMatcher.match_Other(token);
}
}, false);
}
private int matchToken(int state, Token token, ParserContext context) {
int newState;
switch(state) {
case 0:
newState = matchTokenAt_0(token, context);
break;
case 1:
newState = matchTokenAt_1(token, context);
break;
case 2:
newState = matchTokenAt_2(token, context);
break;
case 3:
newState = matchTokenAt_3(token, context);
break;
case 4:
newState = matchTokenAt_4(token, context);
break;
case 5:
newState = matchTokenAt_5(token, context);
break;
case 6:
newState = matchTokenAt_6(token, context);
break;
case 7:
newState = matchTokenAt_7(token, context);
break;
case 8:
newState = matchTokenAt_8(token, context);
break;
case 9:
newState = matchTokenAt_9(token, context);
break;
case 10:
newState = matchTokenAt_10(token, context);
break;
case 11:
newState = matchTokenAt_11(token, context);
break;
case 12:
newState = matchTokenAt_12(token, context);
break;
case 13:
newState = matchTokenAt_13(token, context);
break;
case 14:
newState = matchTokenAt_14(token, context);
break;
case 15:
newState = matchTokenAt_15(token, context);
break;
case 16:
newState = matchTokenAt_16(token, context);
break;
case 17:
newState = matchTokenAt_17(token, context);
break;
case 18:
newState = matchTokenAt_18(token, context);
break;
case 19:
newState = matchTokenAt_19(token, context);
break;
case 20:
newState = matchTokenAt_20(token, context);
break;
case 21:
newState = matchTokenAt_21(token, context);
break;
case 22:
newState = matchTokenAt_22(token, context);
break;
case 23:
newState = matchTokenAt_23(token, context);
break;
case 24:
newState = matchTokenAt_24(token, context);
break;
case 25:
newState = matchTokenAt_25(token, context);
break;
case 26:
newState = matchTokenAt_26(token, context);
break;
case 27:
newState = matchTokenAt_27(token, context);
break;
case 29:
newState = matchTokenAt_29(token, context);
break;
case 30:
newState = matchTokenAt_30(token, context);
break;
case 31:
newState = matchTokenAt_31(token, context);
break;
case 32:
newState = matchTokenAt_32(token, context);
break;
case 33:
newState = matchTokenAt_33(token, context);
break;
case 34:
newState = matchTokenAt_34(token, context);
break;
default:
throw new IllegalStateException("Unknown state: " + state);
}
return newState;
}
// Start
private int matchTokenAt_0(Token token, ParserContext context) {
if (match_Language(context, token))
{
startRule(context, RuleType.Feature_Header);
build(context, token);
return 1;
}
if (match_TagLine(context, token))
{
startRule(context, RuleType.Feature_Header);
startRule(context, RuleType.Tags);
build(context, token);
return 2;
}
if (match_FeatureLine(context, token))
{
startRule(context, RuleType.Feature_Header);
build(context, token);
return 3;
}
if (match_Comment(context, token))
{
build(context, token);
return 0;
}
if (match_Empty(context, token))
{
build(context, token);
return 0;
}
final String stateComment = "State: 0 - Start";
token.detach();
List expectedTokens = asList("#Language", "#TagLine", "#FeatureLine", "#Comment", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 0;
}
// Feature:0>Feature_Header:0>#Language:0
private int matchTokenAt_1(Token token, ParserContext context) {
if (match_TagLine(context, token))
{
startRule(context, RuleType.Tags);
build(context, token);
return 2;
}
if (match_FeatureLine(context, token))
{
build(context, token);
return 3;
}
if (match_Comment(context, token))
{
build(context, token);
return 1;
}
if (match_Empty(context, token))
{
build(context, token);
return 1;
}
final String stateComment = "State: 1 - Feature:0>Feature_Header:0>#Language:0";
token.detach();
List expectedTokens = asList("#TagLine", "#FeatureLine", "#Comment", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 1;
}
// Feature:0>Feature_Header:1>Tags:0>#TagLine:0
private int matchTokenAt_2(Token token, ParserContext context) {
if (match_TagLine(context, token))
{
build(context, token);
return 2;
}
if (match_FeatureLine(context, token))
{
endRule(context, RuleType.Tags);
build(context, token);
return 3;
}
if (match_Comment(context, token))
{
build(context, token);
return 2;
}
if (match_Empty(context, token))
{
build(context, token);
return 2;
}
final String stateComment = "State: 2 - Feature:0>Feature_Header:1>Tags:0>#TagLine:0";
token.detach();
List expectedTokens = asList("#TagLine", "#FeatureLine", "#Comment", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 2;
}
// Feature:0>Feature_Header:2>#FeatureLine:0
private int matchTokenAt_3(Token token, ParserContext context) {
if (match_EOF(context, token))
{
endRule(context, RuleType.Feature_Header);
build(context, token);
return 28;
}
if (match_Empty(context, token))
{
build(context, token);
return 3;
}
if (match_Comment(context, token))
{
build(context, token);
return 5;
}
if (match_BackgroundLine(context, token))
{
endRule(context, RuleType.Feature_Header);
startRule(context, RuleType.Background);
build(context, token);
return 6;
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.Feature_Header);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 11;
}
if (match_ScenarioLine(context, token))
{
endRule(context, RuleType.Feature_Header);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario);
build(context, token);
return 12;
}
if (match_ScenarioOutlineLine(context, token))
{
endRule(context, RuleType.Feature_Header);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.ScenarioOutline);
build(context, token);
return 17;
}
if (match_Other(context, token))
{
startRule(context, RuleType.Description);
build(context, token);
return 4;
}
final String stateComment = "State: 3 - Feature:0>Feature_Header:2>#FeatureLine:0";
token.detach();
List expectedTokens = asList("#EOF", "#Empty", "#Comment", "#BackgroundLine", "#TagLine", "#ScenarioLine", "#ScenarioOutlineLine", "#Other");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 3;
}
// Feature:0>Feature_Header:3>Feature_Description:0>Description_Helper:1>Description:0>#Other:0
private int matchTokenAt_4(Token token, ParserContext context) {
if (match_EOF(context, token))
{
endRule(context, RuleType.Description);
endRule(context, RuleType.Feature_Header);
build(context, token);
return 28;
}
if (match_Comment(context, token))
{
endRule(context, RuleType.Description);
build(context, token);
return 5;
}
if (match_BackgroundLine(context, token))
{
endRule(context, RuleType.Description);
endRule(context, RuleType.Feature_Header);
startRule(context, RuleType.Background);
build(context, token);
return 6;
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.Description);
endRule(context, RuleType.Feature_Header);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 11;
}
if (match_ScenarioLine(context, token))
{
endRule(context, RuleType.Description);
endRule(context, RuleType.Feature_Header);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario);
build(context, token);
return 12;
}
if (match_ScenarioOutlineLine(context, token))
{
endRule(context, RuleType.Description);
endRule(context, RuleType.Feature_Header);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.ScenarioOutline);
build(context, token);
return 17;
}
if (match_Other(context, token))
{
build(context, token);
return 4;
}
final String stateComment = "State: 4 - Feature:0>Feature_Header:3>Feature_Description:0>Description_Helper:1>Description:0>#Other:0";
token.detach();
List expectedTokens = asList("#EOF", "#Comment", "#BackgroundLine", "#TagLine", "#ScenarioLine", "#ScenarioOutlineLine", "#Other");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 4;
}
// Feature:0>Feature_Header:3>Feature_Description:0>Description_Helper:2>#Comment:0
private int matchTokenAt_5(Token token, ParserContext context) {
if (match_EOF(context, token))
{
endRule(context, RuleType.Feature_Header);
build(context, token);
return 28;
}
if (match_Comment(context, token))
{
build(context, token);
return 5;
}
if (match_BackgroundLine(context, token))
{
endRule(context, RuleType.Feature_Header);
startRule(context, RuleType.Background);
build(context, token);
return 6;
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.Feature_Header);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 11;
}
if (match_ScenarioLine(context, token))
{
endRule(context, RuleType.Feature_Header);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario);
build(context, token);
return 12;
}
if (match_ScenarioOutlineLine(context, token))
{
endRule(context, RuleType.Feature_Header);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.ScenarioOutline);
build(context, token);
return 17;
}
if (match_Empty(context, token))
{
build(context, token);
return 5;
}
final String stateComment = "State: 5 - Feature:0>Feature_Header:3>Feature_Description:0>Description_Helper:2>#Comment:0";
token.detach();
List expectedTokens = asList("#EOF", "#Comment", "#BackgroundLine", "#TagLine", "#ScenarioLine", "#ScenarioOutlineLine", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 5;
}
// Feature:1>Background:0>#BackgroundLine:0
private int matchTokenAt_6(Token token, ParserContext context) {
if (match_EOF(context, token))
{
endRule(context, RuleType.Background);
build(context, token);
return 28;
}
if (match_Empty(context, token))
{
build(context, token);
return 6;
}
if (match_Comment(context, token))
{
build(context, token);
return 8;
}
if (match_StepLine(context, token))
{
startRule(context, RuleType.Step);
build(context, token);
return 9;
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.Background);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 11;
}
if (match_ScenarioLine(context, token))
{
endRule(context, RuleType.Background);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario);
build(context, token);
return 12;
}
if (match_ScenarioOutlineLine(context, token))
{
endRule(context, RuleType.Background);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.ScenarioOutline);
build(context, token);
return 17;
}
if (match_Other(context, token))
{
startRule(context, RuleType.Description);
build(context, token);
return 7;
}
final String stateComment = "State: 6 - Feature:1>Background:0>#BackgroundLine:0";
token.detach();
List expectedTokens = asList("#EOF", "#Empty", "#Comment", "#StepLine", "#TagLine", "#ScenarioLine", "#ScenarioOutlineLine", "#Other");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 6;
}
// Feature:1>Background:1>Background_Description:0>Description_Helper:1>Description:0>#Other:0
private int matchTokenAt_7(Token token, ParserContext context) {
if (match_EOF(context, token))
{
endRule(context, RuleType.Description);
endRule(context, RuleType.Background);
build(context, token);
return 28;
}
if (match_Comment(context, token))
{
endRule(context, RuleType.Description);
build(context, token);
return 8;
}
if (match_StepLine(context, token))
{
endRule(context, RuleType.Description);
startRule(context, RuleType.Step);
build(context, token);
return 9;
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.Description);
endRule(context, RuleType.Background);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 11;
}
if (match_ScenarioLine(context, token))
{
endRule(context, RuleType.Description);
endRule(context, RuleType.Background);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario);
build(context, token);
return 12;
}
if (match_ScenarioOutlineLine(context, token))
{
endRule(context, RuleType.Description);
endRule(context, RuleType.Background);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.ScenarioOutline);
build(context, token);
return 17;
}
if (match_Other(context, token))
{
build(context, token);
return 7;
}
final String stateComment = "State: 7 - Feature:1>Background:1>Background_Description:0>Description_Helper:1>Description:0>#Other:0";
token.detach();
List expectedTokens = asList("#EOF", "#Comment", "#StepLine", "#TagLine", "#ScenarioLine", "#ScenarioOutlineLine", "#Other");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 7;
}
// Feature:1>Background:1>Background_Description:0>Description_Helper:2>#Comment:0
private int matchTokenAt_8(Token token, ParserContext context) {
if (match_EOF(context, token))
{
endRule(context, RuleType.Background);
build(context, token);
return 28;
}
if (match_Comment(context, token))
{
build(context, token);
return 8;
}
if (match_StepLine(context, token))
{
startRule(context, RuleType.Step);
build(context, token);
return 9;
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.Background);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 11;
}
if (match_ScenarioLine(context, token))
{
endRule(context, RuleType.Background);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario);
build(context, token);
return 12;
}
if (match_ScenarioOutlineLine(context, token))
{
endRule(context, RuleType.Background);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.ScenarioOutline);
build(context, token);
return 17;
}
if (match_Empty(context, token))
{
build(context, token);
return 8;
}
final String stateComment = "State: 8 - Feature:1>Background:1>Background_Description:0>Description_Helper:2>#Comment:0";
token.detach();
List expectedTokens = asList("#EOF", "#Comment", "#StepLine", "#TagLine", "#ScenarioLine", "#ScenarioOutlineLine", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 8;
}
// Feature:1>Background:2>Scenario_Step:0>Step:0>#StepLine:0
private int matchTokenAt_9(Token token, ParserContext context) {
if (match_EOF(context, token))
{
endRule(context, RuleType.Step);
endRule(context, RuleType.Background);
build(context, token);
return 28;
}
if (match_TableRow(context, token))
{
startRule(context, RuleType.DataTable);
build(context, token);
return 10;
}
if (match_DocStringSeparator(context, token))
{
startRule(context, RuleType.DocString);
build(context, token);
return 33;
}
if (match_StepLine(context, token))
{
endRule(context, RuleType.Step);
startRule(context, RuleType.Step);
build(context, token);
return 9;
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.Step);
endRule(context, RuleType.Background);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 11;
}
if (match_ScenarioLine(context, token))
{
endRule(context, RuleType.Step);
endRule(context, RuleType.Background);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario);
build(context, token);
return 12;
}
if (match_ScenarioOutlineLine(context, token))
{
endRule(context, RuleType.Step);
endRule(context, RuleType.Background);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.ScenarioOutline);
build(context, token);
return 17;
}
if (match_Comment(context, token))
{
build(context, token);
return 9;
}
if (match_Empty(context, token))
{
build(context, token);
return 9;
}
final String stateComment = "State: 9 - Feature:1>Background:2>Scenario_Step:0>Step:0>#StepLine:0";
token.detach();
List expectedTokens = asList("#EOF", "#TableRow", "#DocStringSeparator", "#StepLine", "#TagLine", "#ScenarioLine", "#ScenarioOutlineLine", "#Comment", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 9;
}
// Feature:1>Background:2>Scenario_Step:0>Step:1>Step_Arg:0>__alt1:0>DataTable:0>#TableRow:0
private int matchTokenAt_10(Token token, ParserContext context) {
if (match_EOF(context, token))
{
endRule(context, RuleType.DataTable);
endRule(context, RuleType.Step);
endRule(context, RuleType.Background);
build(context, token);
return 28;
}
if (match_TableRow(context, token))
{
build(context, token);
return 10;
}
if (match_StepLine(context, token))
{
endRule(context, RuleType.DataTable);
endRule(context, RuleType.Step);
startRule(context, RuleType.Step);
build(context, token);
return 9;
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.DataTable);
endRule(context, RuleType.Step);
endRule(context, RuleType.Background);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 11;
}
if (match_ScenarioLine(context, token))
{
endRule(context, RuleType.DataTable);
endRule(context, RuleType.Step);
endRule(context, RuleType.Background);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario);
build(context, token);
return 12;
}
if (match_ScenarioOutlineLine(context, token))
{
endRule(context, RuleType.DataTable);
endRule(context, RuleType.Step);
endRule(context, RuleType.Background);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.ScenarioOutline);
build(context, token);
return 17;
}
if (match_Comment(context, token))
{
build(context, token);
return 10;
}
if (match_Empty(context, token))
{
build(context, token);
return 10;
}
final String stateComment = "State: 10 - Feature:1>Background:2>Scenario_Step:0>Step:1>Step_Arg:0>__alt1:0>DataTable:0>#TableRow:0";
token.detach();
List expectedTokens = asList("#EOF", "#TableRow", "#StepLine", "#TagLine", "#ScenarioLine", "#ScenarioOutlineLine", "#Comment", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 10;
}
// Feature:2>Scenario_Definition:0>Tags:0>#TagLine:0
private int matchTokenAt_11(Token token, ParserContext context) {
if (match_TagLine(context, token))
{
build(context, token);
return 11;
}
if (match_ScenarioLine(context, token))
{
endRule(context, RuleType.Tags);
startRule(context, RuleType.Scenario);
build(context, token);
return 12;
}
if (match_ScenarioOutlineLine(context, token))
{
endRule(context, RuleType.Tags);
startRule(context, RuleType.ScenarioOutline);
build(context, token);
return 17;
}
if (match_Comment(context, token))
{
build(context, token);
return 11;
}
if (match_Empty(context, token))
{
build(context, token);
return 11;
}
final String stateComment = "State: 11 - Feature:2>Scenario_Definition:0>Tags:0>#TagLine:0";
token.detach();
List expectedTokens = asList("#TagLine", "#ScenarioLine", "#ScenarioOutlineLine", "#Comment", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 11;
}
// Feature:2>Scenario_Definition:1>__alt0:0>Scenario:0>#ScenarioLine:0
private int matchTokenAt_12(Token token, ParserContext context) {
if (match_EOF(context, token))
{
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
build(context, token);
return 28;
}
if (match_Empty(context, token))
{
build(context, token);
return 12;
}
if (match_Comment(context, token))
{
build(context, token);
return 14;
}
if (match_StepLine(context, token))
{
startRule(context, RuleType.Step);
build(context, token);
return 15;
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 11;
}
if (match_ScenarioLine(context, token))
{
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario);
build(context, token);
return 12;
}
if (match_ScenarioOutlineLine(context, token))
{
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.ScenarioOutline);
build(context, token);
return 17;
}
if (match_Other(context, token))
{
startRule(context, RuleType.Description);
build(context, token);
return 13;
}
final String stateComment = "State: 12 - Feature:2>Scenario_Definition:1>__alt0:0>Scenario:0>#ScenarioLine:0";
token.detach();
List expectedTokens = asList("#EOF", "#Empty", "#Comment", "#StepLine", "#TagLine", "#ScenarioLine", "#ScenarioOutlineLine", "#Other");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 12;
}
// Feature:2>Scenario_Definition:1>__alt0:0>Scenario:1>Scenario_Description:0>Description_Helper:1>Description:0>#Other:0
private int matchTokenAt_13(Token token, ParserContext context) {
if (match_EOF(context, token))
{
endRule(context, RuleType.Description);
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
build(context, token);
return 28;
}
if (match_Comment(context, token))
{
endRule(context, RuleType.Description);
build(context, token);
return 14;
}
if (match_StepLine(context, token))
{
endRule(context, RuleType.Description);
startRule(context, RuleType.Step);
build(context, token);
return 15;
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.Description);
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 11;
}
if (match_ScenarioLine(context, token))
{
endRule(context, RuleType.Description);
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario);
build(context, token);
return 12;
}
if (match_ScenarioOutlineLine(context, token))
{
endRule(context, RuleType.Description);
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.ScenarioOutline);
build(context, token);
return 17;
}
if (match_Other(context, token))
{
build(context, token);
return 13;
}
final String stateComment = "State: 13 - Feature:2>Scenario_Definition:1>__alt0:0>Scenario:1>Scenario_Description:0>Description_Helper:1>Description:0>#Other:0";
token.detach();
List expectedTokens = asList("#EOF", "#Comment", "#StepLine", "#TagLine", "#ScenarioLine", "#ScenarioOutlineLine", "#Other");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 13;
}
// Feature:2>Scenario_Definition:1>__alt0:0>Scenario:1>Scenario_Description:0>Description_Helper:2>#Comment:0
private int matchTokenAt_14(Token token, ParserContext context) {
if (match_EOF(context, token))
{
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
build(context, token);
return 28;
}
if (match_Comment(context, token))
{
build(context, token);
return 14;
}
if (match_StepLine(context, token))
{
startRule(context, RuleType.Step);
build(context, token);
return 15;
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 11;
}
if (match_ScenarioLine(context, token))
{
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario);
build(context, token);
return 12;
}
if (match_ScenarioOutlineLine(context, token))
{
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.ScenarioOutline);
build(context, token);
return 17;
}
if (match_Empty(context, token))
{
build(context, token);
return 14;
}
final String stateComment = "State: 14 - Feature:2>Scenario_Definition:1>__alt0:0>Scenario:1>Scenario_Description:0>Description_Helper:2>#Comment:0";
token.detach();
List expectedTokens = asList("#EOF", "#Comment", "#StepLine", "#TagLine", "#ScenarioLine", "#ScenarioOutlineLine", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 14;
}
// Feature:2>Scenario_Definition:1>__alt0:0>Scenario:2>Scenario_Step:0>Step:0>#StepLine:0
private int matchTokenAt_15(Token token, ParserContext context) {
if (match_EOF(context, token))
{
endRule(context, RuleType.Step);
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
build(context, token);
return 28;
}
if (match_TableRow(context, token))
{
startRule(context, RuleType.DataTable);
build(context, token);
return 16;
}
if (match_DocStringSeparator(context, token))
{
startRule(context, RuleType.DocString);
build(context, token);
return 31;
}
if (match_StepLine(context, token))
{
endRule(context, RuleType.Step);
startRule(context, RuleType.Step);
build(context, token);
return 15;
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.Step);
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 11;
}
if (match_ScenarioLine(context, token))
{
endRule(context, RuleType.Step);
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario);
build(context, token);
return 12;
}
if (match_ScenarioOutlineLine(context, token))
{
endRule(context, RuleType.Step);
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.ScenarioOutline);
build(context, token);
return 17;
}
if (match_Comment(context, token))
{
build(context, token);
return 15;
}
if (match_Empty(context, token))
{
build(context, token);
return 15;
}
final String stateComment = "State: 15 - Feature:2>Scenario_Definition:1>__alt0:0>Scenario:2>Scenario_Step:0>Step:0>#StepLine:0";
token.detach();
List expectedTokens = asList("#EOF", "#TableRow", "#DocStringSeparator", "#StepLine", "#TagLine", "#ScenarioLine", "#ScenarioOutlineLine", "#Comment", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 15;
}
// Feature:2>Scenario_Definition:1>__alt0:0>Scenario:2>Scenario_Step:0>Step:1>Step_Arg:0>__alt1:0>DataTable:0>#TableRow:0
private int matchTokenAt_16(Token token, ParserContext context) {
if (match_EOF(context, token))
{
endRule(context, RuleType.DataTable);
endRule(context, RuleType.Step);
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
build(context, token);
return 28;
}
if (match_TableRow(context, token))
{
build(context, token);
return 16;
}
if (match_StepLine(context, token))
{
endRule(context, RuleType.DataTable);
endRule(context, RuleType.Step);
startRule(context, RuleType.Step);
build(context, token);
return 15;
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.DataTable);
endRule(context, RuleType.Step);
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 11;
}
if (match_ScenarioLine(context, token))
{
endRule(context, RuleType.DataTable);
endRule(context, RuleType.Step);
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario);
build(context, token);
return 12;
}
if (match_ScenarioOutlineLine(context, token))
{
endRule(context, RuleType.DataTable);
endRule(context, RuleType.Step);
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.ScenarioOutline);
build(context, token);
return 17;
}
if (match_Comment(context, token))
{
build(context, token);
return 16;
}
if (match_Empty(context, token))
{
build(context, token);
return 16;
}
final String stateComment = "State: 16 - Feature:2>Scenario_Definition:1>__alt0:0>Scenario:2>Scenario_Step:0>Step:1>Step_Arg:0>__alt1:0>DataTable:0>#TableRow:0";
token.detach();
List expectedTokens = asList("#EOF", "#TableRow", "#StepLine", "#TagLine", "#ScenarioLine", "#ScenarioOutlineLine", "#Comment", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 16;
}
// Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:0>#ScenarioOutlineLine:0
private int matchTokenAt_17(Token token, ParserContext context) {
if (match_Empty(context, token))
{
build(context, token);
return 17;
}
if (match_Comment(context, token))
{
build(context, token);
return 19;
}
if (match_StepLine(context, token))
{
startRule(context, RuleType.Step);
build(context, token);
return 20;
}
if (match_TagLine(context, token))
{
startRule(context, RuleType.Examples_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 22;
}
if (match_ExamplesLine(context, token))
{
startRule(context, RuleType.Examples_Definition);
startRule(context, RuleType.Examples);
build(context, token);
return 23;
}
if (match_Other(context, token))
{
startRule(context, RuleType.Description);
build(context, token);
return 18;
}
final String stateComment = "State: 17 - Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:0>#ScenarioOutlineLine:0";
token.detach();
List expectedTokens = asList("#Empty", "#Comment", "#StepLine", "#TagLine", "#ExamplesLine", "#Other");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 17;
}
// Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:1>ScenarioOutline_Description:0>Description_Helper:1>Description:0>#Other:0
private int matchTokenAt_18(Token token, ParserContext context) {
if (match_Comment(context, token))
{
endRule(context, RuleType.Description);
build(context, token);
return 19;
}
if (match_StepLine(context, token))
{
endRule(context, RuleType.Description);
startRule(context, RuleType.Step);
build(context, token);
return 20;
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.Description);
startRule(context, RuleType.Examples_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 22;
}
if (match_ExamplesLine(context, token))
{
endRule(context, RuleType.Description);
startRule(context, RuleType.Examples_Definition);
startRule(context, RuleType.Examples);
build(context, token);
return 23;
}
if (match_Other(context, token))
{
build(context, token);
return 18;
}
final String stateComment = "State: 18 - Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:1>ScenarioOutline_Description:0>Description_Helper:1>Description:0>#Other:0";
token.detach();
List expectedTokens = asList("#Comment", "#StepLine", "#TagLine", "#ExamplesLine", "#Other");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 18;
}
// Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:1>ScenarioOutline_Description:0>Description_Helper:2>#Comment:0
private int matchTokenAt_19(Token token, ParserContext context) {
if (match_Comment(context, token))
{
build(context, token);
return 19;
}
if (match_StepLine(context, token))
{
startRule(context, RuleType.Step);
build(context, token);
return 20;
}
if (match_TagLine(context, token))
{
startRule(context, RuleType.Examples_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 22;
}
if (match_ExamplesLine(context, token))
{
startRule(context, RuleType.Examples_Definition);
startRule(context, RuleType.Examples);
build(context, token);
return 23;
}
if (match_Empty(context, token))
{
build(context, token);
return 19;
}
final String stateComment = "State: 19 - Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:1>ScenarioOutline_Description:0>Description_Helper:2>#Comment:0";
token.detach();
List expectedTokens = asList("#Comment", "#StepLine", "#TagLine", "#ExamplesLine", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 19;
}
// Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:2>ScenarioOutline_Step:0>Step:0>#StepLine:0
private int matchTokenAt_20(Token token, ParserContext context) {
if (match_TableRow(context, token))
{
startRule(context, RuleType.DataTable);
build(context, token);
return 21;
}
if (match_DocStringSeparator(context, token))
{
startRule(context, RuleType.DocString);
build(context, token);
return 29;
}
if (match_StepLine(context, token))
{
endRule(context, RuleType.Step);
startRule(context, RuleType.Step);
build(context, token);
return 20;
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.Step);
startRule(context, RuleType.Examples_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 22;
}
if (match_ExamplesLine(context, token))
{
endRule(context, RuleType.Step);
startRule(context, RuleType.Examples_Definition);
startRule(context, RuleType.Examples);
build(context, token);
return 23;
}
if (match_Comment(context, token))
{
build(context, token);
return 20;
}
if (match_Empty(context, token))
{
build(context, token);
return 20;
}
final String stateComment = "State: 20 - Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:2>ScenarioOutline_Step:0>Step:0>#StepLine:0";
token.detach();
List expectedTokens = asList("#TableRow", "#DocStringSeparator", "#StepLine", "#TagLine", "#ExamplesLine", "#Comment", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 20;
}
// Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:2>ScenarioOutline_Step:0>Step:1>Step_Arg:0>__alt1:0>DataTable:0>#TableRow:0
private int matchTokenAt_21(Token token, ParserContext context) {
if (match_TableRow(context, token))
{
build(context, token);
return 21;
}
if (match_StepLine(context, token))
{
endRule(context, RuleType.DataTable);
endRule(context, RuleType.Step);
startRule(context, RuleType.Step);
build(context, token);
return 20;
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.DataTable);
endRule(context, RuleType.Step);
startRule(context, RuleType.Examples_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 22;
}
if (match_ExamplesLine(context, token))
{
endRule(context, RuleType.DataTable);
endRule(context, RuleType.Step);
startRule(context, RuleType.Examples_Definition);
startRule(context, RuleType.Examples);
build(context, token);
return 23;
}
if (match_Comment(context, token))
{
build(context, token);
return 21;
}
if (match_Empty(context, token))
{
build(context, token);
return 21;
}
final String stateComment = "State: 21 - Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:2>ScenarioOutline_Step:0>Step:1>Step_Arg:0>__alt1:0>DataTable:0>#TableRow:0";
token.detach();
List expectedTokens = asList("#TableRow", "#StepLine", "#TagLine", "#ExamplesLine", "#Comment", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 21;
}
// Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:3>Examples_Definition:0>Tags:0>#TagLine:0
private int matchTokenAt_22(Token token, ParserContext context) {
if (match_TagLine(context, token))
{
build(context, token);
return 22;
}
if (match_ExamplesLine(context, token))
{
endRule(context, RuleType.Tags);
startRule(context, RuleType.Examples);
build(context, token);
return 23;
}
if (match_Comment(context, token))
{
build(context, token);
return 22;
}
if (match_Empty(context, token))
{
build(context, token);
return 22;
}
final String stateComment = "State: 22 - Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:3>Examples_Definition:0>Tags:0>#TagLine:0";
token.detach();
List expectedTokens = asList("#TagLine", "#ExamplesLine", "#Comment", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 22;
}
// Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:3>Examples_Definition:1>Examples:0>#ExamplesLine:0
private int matchTokenAt_23(Token token, ParserContext context) {
if (match_Empty(context, token))
{
build(context, token);
return 23;
}
if (match_Comment(context, token))
{
build(context, token);
return 25;
}
if (match_TableRow(context, token))
{
build(context, token);
return 26;
}
if (match_Other(context, token))
{
startRule(context, RuleType.Description);
build(context, token);
return 24;
}
final String stateComment = "State: 23 - Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:3>Examples_Definition:1>Examples:0>#ExamplesLine:0";
token.detach();
List expectedTokens = asList("#Empty", "#Comment", "#TableRow", "#Other");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 23;
}
// Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:3>Examples_Definition:1>Examples:1>Examples_Description:0>Description_Helper:1>Description:0>#Other:0
private int matchTokenAt_24(Token token, ParserContext context) {
if (match_Comment(context, token))
{
endRule(context, RuleType.Description);
build(context, token);
return 25;
}
if (match_TableRow(context, token))
{
endRule(context, RuleType.Description);
build(context, token);
return 26;
}
if (match_Other(context, token))
{
build(context, token);
return 24;
}
final String stateComment = "State: 24 - Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:3>Examples_Definition:1>Examples:1>Examples_Description:0>Description_Helper:1>Description:0>#Other:0";
token.detach();
List expectedTokens = asList("#Comment", "#TableRow", "#Other");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 24;
}
// Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:3>Examples_Definition:1>Examples:1>Examples_Description:0>Description_Helper:2>#Comment:0
private int matchTokenAt_25(Token token, ParserContext context) {
if (match_Comment(context, token))
{
build(context, token);
return 25;
}
if (match_TableRow(context, token))
{
build(context, token);
return 26;
}
if (match_Empty(context, token))
{
build(context, token);
return 25;
}
final String stateComment = "State: 25 - Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:3>Examples_Definition:1>Examples:1>Examples_Description:0>Description_Helper:2>#Comment:0";
token.detach();
List expectedTokens = asList("#Comment", "#TableRow", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 25;
}
// Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:3>Examples_Definition:1>Examples:2>#TableRow:0
private int matchTokenAt_26(Token token, ParserContext context) {
if (match_TableRow(context, token))
{
build(context, token);
return 27;
}
if (match_Comment(context, token))
{
build(context, token);
return 26;
}
if (match_Empty(context, token))
{
build(context, token);
return 26;
}
final String stateComment = "State: 26 - Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:3>Examples_Definition:1>Examples:2>#TableRow:0";
token.detach();
List expectedTokens = asList("#TableRow", "#Comment", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 26;
}
// Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:3>Examples_Definition:1>Examples:3>#TableRow:0
private int matchTokenAt_27(Token token, ParserContext context) {
if (match_EOF(context, token))
{
endRule(context, RuleType.Examples);
endRule(context, RuleType.Examples_Definition);
endRule(context, RuleType.ScenarioOutline);
endRule(context, RuleType.Scenario_Definition);
build(context, token);
return 28;
}
if (match_TableRow(context, token))
{
build(context, token);
return 27;
}
if (match_TagLine(context, token))
{
if (lookahead_0(context, token))
{
endRule(context, RuleType.Examples);
endRule(context, RuleType.Examples_Definition);
startRule(context, RuleType.Examples_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 22;
}
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.Examples);
endRule(context, RuleType.Examples_Definition);
endRule(context, RuleType.ScenarioOutline);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 11;
}
if (match_ExamplesLine(context, token))
{
endRule(context, RuleType.Examples);
endRule(context, RuleType.Examples_Definition);
startRule(context, RuleType.Examples_Definition);
startRule(context, RuleType.Examples);
build(context, token);
return 23;
}
if (match_ScenarioLine(context, token))
{
endRule(context, RuleType.Examples);
endRule(context, RuleType.Examples_Definition);
endRule(context, RuleType.ScenarioOutline);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario);
build(context, token);
return 12;
}
if (match_ScenarioOutlineLine(context, token))
{
endRule(context, RuleType.Examples);
endRule(context, RuleType.Examples_Definition);
endRule(context, RuleType.ScenarioOutline);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.ScenarioOutline);
build(context, token);
return 17;
}
if (match_Comment(context, token))
{
build(context, token);
return 27;
}
if (match_Empty(context, token))
{
build(context, token);
return 27;
}
final String stateComment = "State: 27 - Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:3>Examples_Definition:1>Examples:3>#TableRow:0";
token.detach();
List expectedTokens = asList("#EOF", "#TableRow", "#TagLine", "#ExamplesLine", "#ScenarioLine", "#ScenarioOutlineLine", "#Comment", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 27;
}
// Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:2>ScenarioOutline_Step:0>Step:1>Step_Arg:0>__alt1:1>DocString:0>#DocStringSeparator:0
private int matchTokenAt_29(Token token, ParserContext context) {
if (match_DocStringSeparator(context, token))
{
build(context, token);
return 30;
}
if (match_Other(context, token))
{
build(context, token);
return 29;
}
final String stateComment = "State: 29 - Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:2>ScenarioOutline_Step:0>Step:1>Step_Arg:0>__alt1:1>DocString:0>#DocStringSeparator:0";
token.detach();
List expectedTokens = asList("#DocStringSeparator", "#Other");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 29;
}
// Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:2>ScenarioOutline_Step:0>Step:1>Step_Arg:0>__alt1:1>DocString:2>#DocStringSeparator:0
private int matchTokenAt_30(Token token, ParserContext context) {
if (match_StepLine(context, token))
{
endRule(context, RuleType.DocString);
endRule(context, RuleType.Step);
startRule(context, RuleType.Step);
build(context, token);
return 20;
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.DocString);
endRule(context, RuleType.Step);
startRule(context, RuleType.Examples_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 22;
}
if (match_ExamplesLine(context, token))
{
endRule(context, RuleType.DocString);
endRule(context, RuleType.Step);
startRule(context, RuleType.Examples_Definition);
startRule(context, RuleType.Examples);
build(context, token);
return 23;
}
if (match_Comment(context, token))
{
build(context, token);
return 30;
}
if (match_Empty(context, token))
{
build(context, token);
return 30;
}
final String stateComment = "State: 30 - Feature:2>Scenario_Definition:1>__alt0:1>ScenarioOutline:2>ScenarioOutline_Step:0>Step:1>Step_Arg:0>__alt1:1>DocString:2>#DocStringSeparator:0";
token.detach();
List expectedTokens = asList("#StepLine", "#TagLine", "#ExamplesLine", "#Comment", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 30;
}
// Feature:2>Scenario_Definition:1>__alt0:0>Scenario:2>Scenario_Step:0>Step:1>Step_Arg:0>__alt1:1>DocString:0>#DocStringSeparator:0
private int matchTokenAt_31(Token token, ParserContext context) {
if (match_DocStringSeparator(context, token))
{
build(context, token);
return 32;
}
if (match_Other(context, token))
{
build(context, token);
return 31;
}
final String stateComment = "State: 31 - Feature:2>Scenario_Definition:1>__alt0:0>Scenario:2>Scenario_Step:0>Step:1>Step_Arg:0>__alt1:1>DocString:0>#DocStringSeparator:0";
token.detach();
List expectedTokens = asList("#DocStringSeparator", "#Other");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 31;
}
// Feature:2>Scenario_Definition:1>__alt0:0>Scenario:2>Scenario_Step:0>Step:1>Step_Arg:0>__alt1:1>DocString:2>#DocStringSeparator:0
private int matchTokenAt_32(Token token, ParserContext context) {
if (match_EOF(context, token))
{
endRule(context, RuleType.DocString);
endRule(context, RuleType.Step);
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
build(context, token);
return 28;
}
if (match_StepLine(context, token))
{
endRule(context, RuleType.DocString);
endRule(context, RuleType.Step);
startRule(context, RuleType.Step);
build(context, token);
return 15;
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.DocString);
endRule(context, RuleType.Step);
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 11;
}
if (match_ScenarioLine(context, token))
{
endRule(context, RuleType.DocString);
endRule(context, RuleType.Step);
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario);
build(context, token);
return 12;
}
if (match_ScenarioOutlineLine(context, token))
{
endRule(context, RuleType.DocString);
endRule(context, RuleType.Step);
endRule(context, RuleType.Scenario);
endRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.ScenarioOutline);
build(context, token);
return 17;
}
if (match_Comment(context, token))
{
build(context, token);
return 32;
}
if (match_Empty(context, token))
{
build(context, token);
return 32;
}
final String stateComment = "State: 32 - Feature:2>Scenario_Definition:1>__alt0:0>Scenario:2>Scenario_Step:0>Step:1>Step_Arg:0>__alt1:1>DocString:2>#DocStringSeparator:0";
token.detach();
List expectedTokens = asList("#EOF", "#StepLine", "#TagLine", "#ScenarioLine", "#ScenarioOutlineLine", "#Comment", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 32;
}
// Feature:1>Background:2>Scenario_Step:0>Step:1>Step_Arg:0>__alt1:1>DocString:0>#DocStringSeparator:0
private int matchTokenAt_33(Token token, ParserContext context) {
if (match_DocStringSeparator(context, token))
{
build(context, token);
return 34;
}
if (match_Other(context, token))
{
build(context, token);
return 33;
}
final String stateComment = "State: 33 - Feature:1>Background:2>Scenario_Step:0>Step:1>Step_Arg:0>__alt1:1>DocString:0>#DocStringSeparator:0";
token.detach();
List expectedTokens = asList("#DocStringSeparator", "#Other");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 33;
}
// Feature:1>Background:2>Scenario_Step:0>Step:1>Step_Arg:0>__alt1:1>DocString:2>#DocStringSeparator:0
private int matchTokenAt_34(Token token, ParserContext context) {
if (match_EOF(context, token))
{
endRule(context, RuleType.DocString);
endRule(context, RuleType.Step);
endRule(context, RuleType.Background);
build(context, token);
return 28;
}
if (match_StepLine(context, token))
{
endRule(context, RuleType.DocString);
endRule(context, RuleType.Step);
startRule(context, RuleType.Step);
build(context, token);
return 9;
}
if (match_TagLine(context, token))
{
endRule(context, RuleType.DocString);
endRule(context, RuleType.Step);
endRule(context, RuleType.Background);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Tags);
build(context, token);
return 11;
}
if (match_ScenarioLine(context, token))
{
endRule(context, RuleType.DocString);
endRule(context, RuleType.Step);
endRule(context, RuleType.Background);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.Scenario);
build(context, token);
return 12;
}
if (match_ScenarioOutlineLine(context, token))
{
endRule(context, RuleType.DocString);
endRule(context, RuleType.Step);
endRule(context, RuleType.Background);
startRule(context, RuleType.Scenario_Definition);
startRule(context, RuleType.ScenarioOutline);
build(context, token);
return 17;
}
if (match_Comment(context, token))
{
build(context, token);
return 34;
}
if (match_Empty(context, token))
{
build(context, token);
return 34;
}
final String stateComment = "State: 34 - Feature:1>Background:2>Scenario_Step:0>Step:1>Step_Arg:0>__alt1:1>DocString:2>#DocStringSeparator:0";
token.detach();
List expectedTokens = asList("#EOF", "#StepLine", "#TagLine", "#ScenarioLine", "#ScenarioOutlineLine", "#Comment", "#Empty");
ParserException error = token.isEOF()
? new ParserException.UnexpectedEOFException(token, expectedTokens, stateComment)
: new ParserException.UnexpectedTokenException(token, expectedTokens, stateComment);
if (stopAtFirstError)
throw error;
addError(context, error);
return 34;
}
private boolean lookahead_0(ParserContext context, Token currentToken) {
currentToken.detach();
Token token;
Queue queue = new ArrayDeque();
boolean match = false;
do
{
token = readToken(context);
token.detach();
queue.add(token);
if (false
|| match_ExamplesLine(context, token)
)
{
match = true;
break;
}
} while (false
|| match_Empty(context, token)
|| match_Comment(context, token)
|| match_TagLine(context, token)
);
context.tokenQueue.addAll(queue);
return match;
}
public interface Builder {
void build(Token token);
void startRule(RuleType ruleType);
void endRule(RuleType ruleType);
T getResult();
void reset();
}
public interface ITokenScanner {
Token read();
}
public interface ITokenMatcher {
boolean match_EOF(Token token);
boolean match_Empty(Token token);
boolean match_Comment(Token token);
boolean match_TagLine(Token token);
boolean match_FeatureLine(Token token);
boolean match_BackgroundLine(Token token);
boolean match_ScenarioLine(Token token);
boolean match_ScenarioOutlineLine(Token token);
boolean match_ExamplesLine(Token token);
boolean match_StepLine(Token token);
boolean match_DocStringSeparator(Token token);
boolean match_TableRow(Token token);
boolean match_Language(Token token);
boolean match_Other(Token token);
void reset();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy