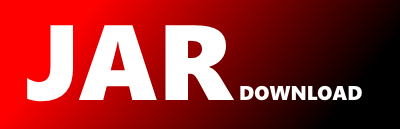
io.dangernoodle.github.repo.setup.GithubAssignTeams Maven / Gradle / Ivy
package io.dangernoodle.github.repo.setup;
import java.io.IOException;
import java.util.Map.Entry;
import org.kohsuke.github.GHOrganization;
import org.kohsuke.github.GHRepository;
import org.kohsuke.github.GHTeam;
import io.dangernoodle.github.GithubClient;
import io.dangernoodle.github.repo.setup.settings.GithubRepositorySettings;
import io.dangernoodle.github.utils.GithubUtilities;
import io.dangernoodle.scm.ScmException;
import io.dangernoodle.scm.setup.RepositorySetupException;
public class GithubAssignTeams extends GithubRepositorySetupStep
{
public GithubAssignTeams(GithubClient client)
{
super(client);
}
@Override
protected void execute(String organization, String repository, GithubRepositorySettings settings) throws ScmException
{
GHRepository ghRepository = GithubUtilities.getRepository(client, organization, repository);
for (Entry entry : settings.getTeams().entrySet())
{
GHTeam ghTeam = GithubUtilities.getTeam(client, organization, entry.getKey());
addTeampToRepository(ghTeam, entry.getValue(), ghRepository);
}
}
private void addTeampToRepository(GHTeam team, GithubRepositorySettings.Permission permission, GHRepository ghRepository)
throws RepositorySetupException
{
try
{
team.add(ghRepository, mapToOrgPermission(permission));
}
catch (IOException e)
{
logger.error("failed to add team/permission [{} / {}] to repository [{}]", team, permission, ghRepository.getName(), e);
throw new RepositorySetupException(e, "failed to add team/permission [%s / %s] to repository [%s]", team, permission,
ghRepository.getName());
}
}
private GHOrganization.Permission mapToOrgPermission(GithubRepositorySettings.Permission permission)
{
switch (permission)
{
case admin:
return GHOrganization.Permission.ADMIN;
case write:
return GHOrganization.Permission.PUSH;
default:
return GHOrganization.Permission.PULL;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy