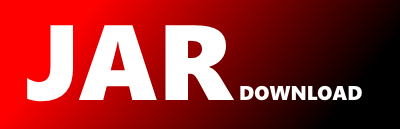
io.dapr.spring.data.AbstractDaprKeyValueAdapter Maven / Gradle / Ivy
/*
* Copyright 2024 The Dapr Authors
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
limitations under the License.
*/
package io.dapr.spring.data;
import io.dapr.client.DaprClient;
import io.dapr.client.domain.GetStateRequest;
import io.dapr.client.domain.SaveStateRequest;
import io.dapr.client.domain.State;
import io.dapr.utils.TypeRef;
import org.springframework.data.keyvalue.core.KeyValueAdapter;
import org.springframework.data.util.CloseableIterator;
import org.springframework.util.Assert;
import reactor.core.publisher.Mono;
import java.util.Collections;
import java.util.Map;
public abstract class AbstractDaprKeyValueAdapter implements KeyValueAdapter {
private static final Map CONTENT_TYPE_META = Map.of(
"contentType", "application/json");
private final DaprClient daprClient;
private final String stateStoreName;
protected AbstractDaprKeyValueAdapter(DaprClient daprClient, String stateStoreName) {
Assert.notNull(daprClient, "DaprClient must not be null");
Assert.hasText(stateStoreName, "State store name must not be empty");
this.daprClient = daprClient;
this.stateStoreName = stateStoreName;
}
@Override
public void destroy() throws Exception {
daprClient.close();
}
@Override
public void clear() {
// Ignore
}
@Override
public Object put(Object id, Object item, String keyspace) {
Assert.notNull(id, "Id must not be null");
Assert.notNull(item, "Item must not be null");
Assert.hasText(keyspace, "Keyspace must not be empty");
String key = resolveKey(keyspace, id);
State
© 2015 - 2024 Weber Informatics LLC | Privacy Policy