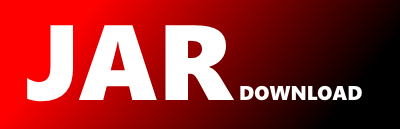
io.dapr.spring.data.repository.query.DaprPredicate Maven / Gradle / Ivy
package io.dapr.spring.data.repository.query;
import org.springframework.beans.BeanWrapper;
import org.springframework.data.mapping.PropertyPath;
import org.springframework.data.util.DirectFieldAccessFallbackBeanWrapper;
import org.springframework.util.ObjectUtils;
import java.util.function.Function;
import java.util.function.Predicate;
public class DaprPredicate implements Predicate
© 2015 - 2024 Weber Informatics LLC | Privacy Policy