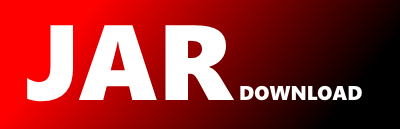
io.dapr.v1.DaprGrpc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dapr-sdk-autogen Show documentation
Show all versions of dapr-sdk-autogen Show documentation
Auto-generated SDK for Dapr
package io.dapr.v1;
import static io.grpc.MethodDescriptor.generateFullMethodName;
import static io.grpc.stub.ClientCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ClientCalls.asyncClientStreamingCall;
import static io.grpc.stub.ClientCalls.asyncServerStreamingCall;
import static io.grpc.stub.ClientCalls.asyncUnaryCall;
import static io.grpc.stub.ClientCalls.blockingServerStreamingCall;
import static io.grpc.stub.ClientCalls.blockingUnaryCall;
import static io.grpc.stub.ClientCalls.futureUnaryCall;
import static io.grpc.stub.ServerCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ServerCalls.asyncClientStreamingCall;
import static io.grpc.stub.ServerCalls.asyncServerStreamingCall;
import static io.grpc.stub.ServerCalls.asyncUnaryCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedStreamingCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall;
/**
*
* Dapr service provides APIs to user application to access Dapr building blocks.
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.25.0)",
comments = "Source: dapr.proto")
public final class DaprGrpc {
private DaprGrpc() {}
public static final String SERVICE_NAME = "dapr.proto.runtime.v1.Dapr";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getInvokeServiceMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "InvokeService",
requestType = io.dapr.v1.DaprProtos.InvokeServiceRequest.class,
responseType = io.dapr.v1.CommonProtos.InvokeResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getInvokeServiceMethod() {
io.grpc.MethodDescriptor getInvokeServiceMethod;
if ((getInvokeServiceMethod = DaprGrpc.getInvokeServiceMethod) == null) {
synchronized (DaprGrpc.class) {
if ((getInvokeServiceMethod = DaprGrpc.getInvokeServiceMethod) == null) {
DaprGrpc.getInvokeServiceMethod = getInvokeServiceMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "InvokeService"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.dapr.v1.DaprProtos.InvokeServiceRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.dapr.v1.CommonProtos.InvokeResponse.getDefaultInstance()))
.setSchemaDescriptor(new DaprMethodDescriptorSupplier("InvokeService"))
.build();
}
}
}
return getInvokeServiceMethod;
}
private static volatile io.grpc.MethodDescriptor getGetStateMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetState",
requestType = io.dapr.v1.DaprProtos.GetStateRequest.class,
responseType = io.dapr.v1.DaprProtos.GetStateResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetStateMethod() {
io.grpc.MethodDescriptor getGetStateMethod;
if ((getGetStateMethod = DaprGrpc.getGetStateMethod) == null) {
synchronized (DaprGrpc.class) {
if ((getGetStateMethod = DaprGrpc.getGetStateMethod) == null) {
DaprGrpc.getGetStateMethod = getGetStateMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetState"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.dapr.v1.DaprProtos.GetStateRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.dapr.v1.DaprProtos.GetStateResponse.getDefaultInstance()))
.setSchemaDescriptor(new DaprMethodDescriptorSupplier("GetState"))
.build();
}
}
}
return getGetStateMethod;
}
private static volatile io.grpc.MethodDescriptor getSaveStateMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "SaveState",
requestType = io.dapr.v1.DaprProtos.SaveStateRequest.class,
responseType = com.google.protobuf.Empty.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getSaveStateMethod() {
io.grpc.MethodDescriptor getSaveStateMethod;
if ((getSaveStateMethod = DaprGrpc.getSaveStateMethod) == null) {
synchronized (DaprGrpc.class) {
if ((getSaveStateMethod = DaprGrpc.getSaveStateMethod) == null) {
DaprGrpc.getSaveStateMethod = getSaveStateMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "SaveState"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.dapr.v1.DaprProtos.SaveStateRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Empty.getDefaultInstance()))
.setSchemaDescriptor(new DaprMethodDescriptorSupplier("SaveState"))
.build();
}
}
}
return getSaveStateMethod;
}
private static volatile io.grpc.MethodDescriptor getDeleteStateMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "DeleteState",
requestType = io.dapr.v1.DaprProtos.DeleteStateRequest.class,
responseType = com.google.protobuf.Empty.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getDeleteStateMethod() {
io.grpc.MethodDescriptor getDeleteStateMethod;
if ((getDeleteStateMethod = DaprGrpc.getDeleteStateMethod) == null) {
synchronized (DaprGrpc.class) {
if ((getDeleteStateMethod = DaprGrpc.getDeleteStateMethod) == null) {
DaprGrpc.getDeleteStateMethod = getDeleteStateMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "DeleteState"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.dapr.v1.DaprProtos.DeleteStateRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Empty.getDefaultInstance()))
.setSchemaDescriptor(new DaprMethodDescriptorSupplier("DeleteState"))
.build();
}
}
}
return getDeleteStateMethod;
}
private static volatile io.grpc.MethodDescriptor getPublishEventMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "PublishEvent",
requestType = io.dapr.v1.DaprProtos.PublishEventRequest.class,
responseType = com.google.protobuf.Empty.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getPublishEventMethod() {
io.grpc.MethodDescriptor getPublishEventMethod;
if ((getPublishEventMethod = DaprGrpc.getPublishEventMethod) == null) {
synchronized (DaprGrpc.class) {
if ((getPublishEventMethod = DaprGrpc.getPublishEventMethod) == null) {
DaprGrpc.getPublishEventMethod = getPublishEventMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "PublishEvent"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.dapr.v1.DaprProtos.PublishEventRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Empty.getDefaultInstance()))
.setSchemaDescriptor(new DaprMethodDescriptorSupplier("PublishEvent"))
.build();
}
}
}
return getPublishEventMethod;
}
private static volatile io.grpc.MethodDescriptor getInvokeBindingMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "InvokeBinding",
requestType = io.dapr.v1.DaprProtos.InvokeBindingRequest.class,
responseType = io.dapr.v1.DaprProtos.InvokeBindingResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getInvokeBindingMethod() {
io.grpc.MethodDescriptor getInvokeBindingMethod;
if ((getInvokeBindingMethod = DaprGrpc.getInvokeBindingMethod) == null) {
synchronized (DaprGrpc.class) {
if ((getInvokeBindingMethod = DaprGrpc.getInvokeBindingMethod) == null) {
DaprGrpc.getInvokeBindingMethod = getInvokeBindingMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "InvokeBinding"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.dapr.v1.DaprProtos.InvokeBindingRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.dapr.v1.DaprProtos.InvokeBindingResponse.getDefaultInstance()))
.setSchemaDescriptor(new DaprMethodDescriptorSupplier("InvokeBinding"))
.build();
}
}
}
return getInvokeBindingMethod;
}
private static volatile io.grpc.MethodDescriptor getGetSecretMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetSecret",
requestType = io.dapr.v1.DaprProtos.GetSecretRequest.class,
responseType = io.dapr.v1.DaprProtos.GetSecretResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetSecretMethod() {
io.grpc.MethodDescriptor getGetSecretMethod;
if ((getGetSecretMethod = DaprGrpc.getGetSecretMethod) == null) {
synchronized (DaprGrpc.class) {
if ((getGetSecretMethod = DaprGrpc.getGetSecretMethod) == null) {
DaprGrpc.getGetSecretMethod = getGetSecretMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetSecret"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.dapr.v1.DaprProtos.GetSecretRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.dapr.v1.DaprProtos.GetSecretResponse.getDefaultInstance()))
.setSchemaDescriptor(new DaprMethodDescriptorSupplier("GetSecret"))
.build();
}
}
}
return getGetSecretMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static DaprStub newStub(io.grpc.Channel channel) {
return new DaprStub(channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static DaprBlockingStub newBlockingStub(
io.grpc.Channel channel) {
return new DaprBlockingStub(channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static DaprFutureStub newFutureStub(
io.grpc.Channel channel) {
return new DaprFutureStub(channel);
}
/**
*
* Dapr service provides APIs to user application to access Dapr building blocks.
*
*/
public static abstract class DaprImplBase implements io.grpc.BindableService {
/**
*
* Invokes a method on a remote Dapr app.
*
*/
public void invokeService(io.dapr.v1.DaprProtos.InvokeServiceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getInvokeServiceMethod(), responseObserver);
}
/**
*
* Gets the state for a specific key.
*
*/
public void getState(io.dapr.v1.DaprProtos.GetStateRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getGetStateMethod(), responseObserver);
}
/**
*
* Saves the state for a specific key.
*
*/
public void saveState(io.dapr.v1.DaprProtos.SaveStateRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getSaveStateMethod(), responseObserver);
}
/**
*
* Deletes the state for a specific key.
*
*/
public void deleteState(io.dapr.v1.DaprProtos.DeleteStateRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getDeleteStateMethod(), responseObserver);
}
/**
*
* Publishes events to the specific topic.
*
*/
public void publishEvent(io.dapr.v1.DaprProtos.PublishEventRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getPublishEventMethod(), responseObserver);
}
/**
*
* Invokes binding data to specific output bindings
*
*/
public void invokeBinding(io.dapr.v1.DaprProtos.InvokeBindingRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getInvokeBindingMethod(), responseObserver);
}
/**
*
* Gets secrets from secret stores.
*
*/
public void getSecret(io.dapr.v1.DaprProtos.GetSecretRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getGetSecretMethod(), responseObserver);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getInvokeServiceMethod(),
asyncUnaryCall(
new MethodHandlers<
io.dapr.v1.DaprProtos.InvokeServiceRequest,
io.dapr.v1.CommonProtos.InvokeResponse>(
this, METHODID_INVOKE_SERVICE)))
.addMethod(
getGetStateMethod(),
asyncUnaryCall(
new MethodHandlers<
io.dapr.v1.DaprProtos.GetStateRequest,
io.dapr.v1.DaprProtos.GetStateResponse>(
this, METHODID_GET_STATE)))
.addMethod(
getSaveStateMethod(),
asyncUnaryCall(
new MethodHandlers<
io.dapr.v1.DaprProtos.SaveStateRequest,
com.google.protobuf.Empty>(
this, METHODID_SAVE_STATE)))
.addMethod(
getDeleteStateMethod(),
asyncUnaryCall(
new MethodHandlers<
io.dapr.v1.DaprProtos.DeleteStateRequest,
com.google.protobuf.Empty>(
this, METHODID_DELETE_STATE)))
.addMethod(
getPublishEventMethod(),
asyncUnaryCall(
new MethodHandlers<
io.dapr.v1.DaprProtos.PublishEventRequest,
com.google.protobuf.Empty>(
this, METHODID_PUBLISH_EVENT)))
.addMethod(
getInvokeBindingMethod(),
asyncUnaryCall(
new MethodHandlers<
io.dapr.v1.DaprProtos.InvokeBindingRequest,
io.dapr.v1.DaprProtos.InvokeBindingResponse>(
this, METHODID_INVOKE_BINDING)))
.addMethod(
getGetSecretMethod(),
asyncUnaryCall(
new MethodHandlers<
io.dapr.v1.DaprProtos.GetSecretRequest,
io.dapr.v1.DaprProtos.GetSecretResponse>(
this, METHODID_GET_SECRET)))
.build();
}
}
/**
*
* Dapr service provides APIs to user application to access Dapr building blocks.
*
*/
public static final class DaprStub extends io.grpc.stub.AbstractStub {
private DaprStub(io.grpc.Channel channel) {
super(channel);
}
private DaprStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected DaprStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new DaprStub(channel, callOptions);
}
/**
*
* Invokes a method on a remote Dapr app.
*
*/
public void invokeService(io.dapr.v1.DaprProtos.InvokeServiceRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getInvokeServiceMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Gets the state for a specific key.
*
*/
public void getState(io.dapr.v1.DaprProtos.GetStateRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getGetStateMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Saves the state for a specific key.
*
*/
public void saveState(io.dapr.v1.DaprProtos.SaveStateRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getSaveStateMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Deletes the state for a specific key.
*
*/
public void deleteState(io.dapr.v1.DaprProtos.DeleteStateRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getDeleteStateMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Publishes events to the specific topic.
*
*/
public void publishEvent(io.dapr.v1.DaprProtos.PublishEventRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getPublishEventMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Invokes binding data to specific output bindings
*
*/
public void invokeBinding(io.dapr.v1.DaprProtos.InvokeBindingRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getInvokeBindingMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Gets secrets from secret stores.
*
*/
public void getSecret(io.dapr.v1.DaprProtos.GetSecretRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getGetSecretMethod(), getCallOptions()), request, responseObserver);
}
}
/**
*
* Dapr service provides APIs to user application to access Dapr building blocks.
*
*/
public static final class DaprBlockingStub extends io.grpc.stub.AbstractStub {
private DaprBlockingStub(io.grpc.Channel channel) {
super(channel);
}
private DaprBlockingStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected DaprBlockingStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new DaprBlockingStub(channel, callOptions);
}
/**
*
* Invokes a method on a remote Dapr app.
*
*/
public io.dapr.v1.CommonProtos.InvokeResponse invokeService(io.dapr.v1.DaprProtos.InvokeServiceRequest request) {
return blockingUnaryCall(
getChannel(), getInvokeServiceMethod(), getCallOptions(), request);
}
/**
*
* Gets the state for a specific key.
*
*/
public io.dapr.v1.DaprProtos.GetStateResponse getState(io.dapr.v1.DaprProtos.GetStateRequest request) {
return blockingUnaryCall(
getChannel(), getGetStateMethod(), getCallOptions(), request);
}
/**
*
* Saves the state for a specific key.
*
*/
public com.google.protobuf.Empty saveState(io.dapr.v1.DaprProtos.SaveStateRequest request) {
return blockingUnaryCall(
getChannel(), getSaveStateMethod(), getCallOptions(), request);
}
/**
*
* Deletes the state for a specific key.
*
*/
public com.google.protobuf.Empty deleteState(io.dapr.v1.DaprProtos.DeleteStateRequest request) {
return blockingUnaryCall(
getChannel(), getDeleteStateMethod(), getCallOptions(), request);
}
/**
*
* Publishes events to the specific topic.
*
*/
public com.google.protobuf.Empty publishEvent(io.dapr.v1.DaprProtos.PublishEventRequest request) {
return blockingUnaryCall(
getChannel(), getPublishEventMethod(), getCallOptions(), request);
}
/**
*
* Invokes binding data to specific output bindings
*
*/
public io.dapr.v1.DaprProtos.InvokeBindingResponse invokeBinding(io.dapr.v1.DaprProtos.InvokeBindingRequest request) {
return blockingUnaryCall(
getChannel(), getInvokeBindingMethod(), getCallOptions(), request);
}
/**
*
* Gets secrets from secret stores.
*
*/
public io.dapr.v1.DaprProtos.GetSecretResponse getSecret(io.dapr.v1.DaprProtos.GetSecretRequest request) {
return blockingUnaryCall(
getChannel(), getGetSecretMethod(), getCallOptions(), request);
}
}
/**
*
* Dapr service provides APIs to user application to access Dapr building blocks.
*
*/
public static final class DaprFutureStub extends io.grpc.stub.AbstractStub {
private DaprFutureStub(io.grpc.Channel channel) {
super(channel);
}
private DaprFutureStub(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected DaprFutureStub build(io.grpc.Channel channel,
io.grpc.CallOptions callOptions) {
return new DaprFutureStub(channel, callOptions);
}
/**
*
* Invokes a method on a remote Dapr app.
*
*/
public com.google.common.util.concurrent.ListenableFuture invokeService(
io.dapr.v1.DaprProtos.InvokeServiceRequest request) {
return futureUnaryCall(
getChannel().newCall(getInvokeServiceMethod(), getCallOptions()), request);
}
/**
*
* Gets the state for a specific key.
*
*/
public com.google.common.util.concurrent.ListenableFuture getState(
io.dapr.v1.DaprProtos.GetStateRequest request) {
return futureUnaryCall(
getChannel().newCall(getGetStateMethod(), getCallOptions()), request);
}
/**
*
* Saves the state for a specific key.
*
*/
public com.google.common.util.concurrent.ListenableFuture saveState(
io.dapr.v1.DaprProtos.SaveStateRequest request) {
return futureUnaryCall(
getChannel().newCall(getSaveStateMethod(), getCallOptions()), request);
}
/**
*
* Deletes the state for a specific key.
*
*/
public com.google.common.util.concurrent.ListenableFuture deleteState(
io.dapr.v1.DaprProtos.DeleteStateRequest request) {
return futureUnaryCall(
getChannel().newCall(getDeleteStateMethod(), getCallOptions()), request);
}
/**
*
* Publishes events to the specific topic.
*
*/
public com.google.common.util.concurrent.ListenableFuture publishEvent(
io.dapr.v1.DaprProtos.PublishEventRequest request) {
return futureUnaryCall(
getChannel().newCall(getPublishEventMethod(), getCallOptions()), request);
}
/**
*
* Invokes binding data to specific output bindings
*
*/
public com.google.common.util.concurrent.ListenableFuture invokeBinding(
io.dapr.v1.DaprProtos.InvokeBindingRequest request) {
return futureUnaryCall(
getChannel().newCall(getInvokeBindingMethod(), getCallOptions()), request);
}
/**
*
* Gets secrets from secret stores.
*
*/
public com.google.common.util.concurrent.ListenableFuture getSecret(
io.dapr.v1.DaprProtos.GetSecretRequest request) {
return futureUnaryCall(
getChannel().newCall(getGetSecretMethod(), getCallOptions()), request);
}
}
private static final int METHODID_INVOKE_SERVICE = 0;
private static final int METHODID_GET_STATE = 1;
private static final int METHODID_SAVE_STATE = 2;
private static final int METHODID_DELETE_STATE = 3;
private static final int METHODID_PUBLISH_EVENT = 4;
private static final int METHODID_INVOKE_BINDING = 5;
private static final int METHODID_GET_SECRET = 6;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final DaprImplBase serviceImpl;
private final int methodId;
MethodHandlers(DaprImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_INVOKE_SERVICE:
serviceImpl.invokeService((io.dapr.v1.DaprProtos.InvokeServiceRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_STATE:
serviceImpl.getState((io.dapr.v1.DaprProtos.GetStateRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_SAVE_STATE:
serviceImpl.saveState((io.dapr.v1.DaprProtos.SaveStateRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_DELETE_STATE:
serviceImpl.deleteState((io.dapr.v1.DaprProtos.DeleteStateRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_PUBLISH_EVENT:
serviceImpl.publishEvent((io.dapr.v1.DaprProtos.PublishEventRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_INVOKE_BINDING:
serviceImpl.invokeBinding((io.dapr.v1.DaprProtos.InvokeBindingRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_SECRET:
serviceImpl.getSecret((io.dapr.v1.DaprProtos.GetSecretRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
private static abstract class DaprBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
DaprBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return io.dapr.v1.DaprProtos.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("Dapr");
}
}
private static final class DaprFileDescriptorSupplier
extends DaprBaseDescriptorSupplier {
DaprFileDescriptorSupplier() {}
}
private static final class DaprMethodDescriptorSupplier
extends DaprBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final String methodName;
DaprMethodDescriptorSupplier(String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (DaprGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new DaprFileDescriptorSupplier())
.addMethod(getInvokeServiceMethod())
.addMethod(getGetStateMethod())
.addMethod(getSaveStateMethod())
.addMethod(getDeleteStateMethod())
.addMethod(getPublishEventMethod())
.addMethod(getInvokeBindingMethod())
.addMethod(getGetSecretMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy